Static versus dynamic correlation correlation#
Bond-breaking#
The most commonly used example to illustrate static vs dynamical correlation is H\(_2\) dissociation:
import multipsi as mtp
import numpy as np
import matplotlib.pyplot as plt
import veloxchem as vlx
bohr_to_Å = 0.529177
# HF calculation of H, equivalent to CI
mol_str = "H 0.0000 0.0000 0.0000"
molecule = vlx.Molecule.read_molecule_string(mol_str, units='angstrom')
molecule.set_multiplicity(2)
basis = vlx.MolecularBasis.read(molecule, "cc-pvdz")
scf_drv = vlx.ScfUnrestrictedDriver()
scf_results = scf_drv.compute(molecule, basis)
E_H_hf = scf_drv.get_scf_energy()
# H2 scan
mol_template = """
H 0.0000 0.0000 0.0000
H 0.0000 0.0000 dist
"""
scf_drv = vlx.ScfRestrictedDriver()
ci_drv = mtp.CIDriver()
distlist = [0.5, 0.6, 0.7, 0.75, 0.8,0.9,1.0,1.2,1.5,2,2.5,3,3.5,4,4.5,5]
E_hf = []
E_FCI = []
# Scan over O-H distances
for dist in distlist:
mol_str = mol_template.replace("dist", str(dist))
molecule = vlx.Molecule.read_molecule_string(mol_str, units='angstrom')
basis = vlx.MolecularBasis.read(molecule, "cc-pvdz")
scf_results = scf_drv.compute(molecule, basis)
E_hf.append(scf_drv.get_scf_energy() - 2*E_H_hf)
space=mtp.OrbSpace(molecule,scf_drv.mol_orbs)
space.fci()
ci_results = ci_drv.compute(molecule,basis,space)
E_FCI.append(ci_drv.get_energy() - 2*E_H_hf)
# Save orbitals and densities for equilibrium value
if dist == 0.75:
eq_molecule = molecule
eq_orbitals = scf_drv.mol_orbs
eq_2density = ci_drv.get_active_2body_density(0)
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 5
Primitive Basis Functions : 7
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Unrestricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.0000000000 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.499278403420 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.499278403420 0.0000000000 0.00000005 0.00000003 0.00000000
*** SCF converged in 1 iterations. Time: 0.01 sec.
Spin-Unrestricted Hartree-Fock:
-------------------------------
Total Energy : -0.4992784034 a.u.
Electronic Energy : -0.4992784034 a.u.
Nuclear Repulsion Energy : 0.0000000000 a.u.
------------------------------------
Gradient Norm : 0.0000000462 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Magnetic Quantum Number (M_S) : 0.5
Expectation value of S**2 : 0.7500
Spin Unrestricted Alpha Orbitals
--------------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 1.000 Energy: -0.49928 a.u.
( 1 H 1s : 0.59) ( 1 H 2s : 0.50)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.57244 a.u.
( 1 H 1s : 1.24) ( 1 H 2s : -1.28)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 1.44303 a.u.
( 1 H 1p+1: 0.50) ( 1 H 1p-1: 0.50) ( 1 H 1p0 : -0.71)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 1.44303 a.u.
( 1 H 1p+1: -0.50) ( 1 H 1p-1: -0.50) ( 1 H 1p0 : -0.71)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.44303 a.u.
( 1 H 1p+1: 0.71) ( 1 H 1p-1: -0.71)
Spin Unrestricted Beta Orbitals
-------------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 0.000 Energy: 0.07335 a.u.
( 1 H 1s : 0.22) ( 1 H 2s : 0.84)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.75329 a.u.
( 1 H 1s : -1.35) ( 1 H 2s : 1.09)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 1.57364 a.u.
( 1 H 1p0 : 1.00)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 1.57364 a.u.
( 1 H 1p+1: 1.00)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.57364 a.u.
( 1 H 1p-1: -1.00)
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 1.0583544218 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.044195881437 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.048687184260 0.0000000000 0.03228141 0.00976213 0.00000000
2 -1.048800398179 -0.0001132139 0.00075218 0.00019687 0.00838820
3 -1.048800556215 -0.0000001580 0.00000986 0.00000323 0.00041723
4 -1.048800556227 -0.0000000000 0.00000001 0.00000000 0.00000326
*** SCF converged in 4 iterations. Time: 0.04 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.0488005562 a.u.
Electronic Energy : -2.1071549780 a.u.
Nuclear Repulsion Energy : 1.0583544218 a.u.
------------------------------------
Gradient Norm : 0.0000000051 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.67746 a.u.
( 1 H 1s : -0.43) ( 2 H 1s : -0.43)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.23819 a.u.
( 1 H 2s : 2.99) ( 2 H 2s : -2.99)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.42154 a.u.
( 1 H 1s : -0.62) ( 1 H 2s : 0.72) ( 2 H 1s : -0.62)
( 2 H 2s : 0.72)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 1.16876 a.u.
( 1 H 1s : -0.50) ( 1 H 2s : 1.87) ( 1 H 1p0 : 0.52)
( 2 H 1s : 0.50) ( 2 H 2s : -1.87) ( 2 H 1p0 : 0.52)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.33275 a.u.
( 1 H 1p+1: 0.54) ( 2 H 1p+1: 0.54)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.33275 a.u.
( 1 H 1p-1: -0.54) ( 2 H 1p-1: -0.54)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.079370051 0.0e+00 4.4e-27 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.0793700508928037
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.97719 0.00008 0.00501 0.00970 0.00380 0.00380 0.00013 0.00013 0.00013 0.00001
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.8819620182 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.102601746873 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.106788495584 0.0000000000 0.03045659 0.00841139 0.00000000
2 -1.106892338747 -0.0001038432 0.00069812 0.00020665 0.00725834
3 -1.106892475138 -0.0000001364 0.00000920 0.00000317 0.00039011
4 -1.106892475152 -0.0000000000 0.00000001 0.00000000 0.00000386
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1068924752 a.u.
Electronic Energy : -1.9888544933 a.u.
Nuclear Repulsion Energy : 0.8819620182 a.u.
------------------------------------
Gradient Norm : 0.0000000057 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.63879 a.u.
( 1 H 1s : 0.42) ( 2 H 1s : 0.42)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.22260 a.u.
( 1 H 2s : 2.47) ( 2 H 2s : -2.47)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.44547 a.u.
( 1 H 1s : 0.66) ( 1 H 2s : -0.72) ( 2 H 1s : 0.66)
( 2 H 2s : -0.72)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 1.05754 a.u.
( 1 H 1s : 0.58) ( 1 H 2s : -1.73) ( 1 H 1p0 : -0.50)
( 2 H 1s : -0.58) ( 2 H 2s : 1.73) ( 2 H 1p0 : -0.50)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.31355 a.u.
( 1 H 1p+1: 0.55) ( 2 H 1p+1: 0.55)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.31355 a.u.
( 1 H 1p-1: -0.55) ( 2 H 1p-1: -0.55)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.139173293 0.0e+00 3.6e-28 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.139173293295147
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.97340 0.01319 0.00564 0.00001 0.00360 0.00360 0.00016 0.00015 0.00015 0.00011
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.7559674441 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.122770998276 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.126826026762 0.0000000000 0.02918562 0.00724027 0.00000000
2 -1.126924576362 -0.0000985496 0.00064391 0.00020404 0.00640409
3 -1.126924692283 -0.0000001159 0.00000970 0.00000342 0.00036324
4 -1.126924692303 -0.0000000000 0.00000002 0.00000001 0.00000477
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1269246923 a.u.
Electronic Energy : -1.8828921364 a.u.
Nuclear Repulsion Energy : 0.7559674441 a.u.
------------------------------------
Gradient Norm : 0.0000000192 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.60482 a.u.
( 1 H 1s : -0.41) ( 1 H 2s : -0.16) ( 2 H 1s : -0.41)
( 2 H 2s : -0.16)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.20498 a.u.
( 1 H 2s : -2.09) ( 2 H 2s : 2.09)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.46968 a.u.
( 1 H 1s : -0.69) ( 1 H 2s : 0.73) ( 2 H 1s : -0.69)
( 2 H 2s : 0.73)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.96759 a.u.
( 1 H 1s : -0.71) ( 1 H 2s : 1.64) ( 1 H 1p0 : 0.44)
( 2 H 1s : 0.71) ( 2 H 2s : -1.64) ( 2 H 1p0 : 0.44)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.29782 a.u.
( 1 H 1p+1: -0.57) ( 2 H 1p+1: -0.57)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.29782 a.u.
( 1 H 1p-1: 0.57) ( 2 H 1p-1: 0.57)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.160904682 0.0e+00 9.9e-29 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1609046824806657
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.96871 0.01801 0.00602 0.00001 0.00331 0.00331 0.00019 0.00015 0.00015 0.00014
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.7055696145 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.124608367719 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.128645660151 0.0000000000 0.02872496 0.00752087 0.00000000
2 -1.128743027026 -0.0000973669 0.00062088 0.00020101 0.00607432
3 -1.128743134729 -0.0000001077 0.00001024 0.00000362 0.00035226
4 -1.128743134753 -0.0000000000 0.00000002 0.00000001 0.00000526
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1287431348 a.u.
Electronic Energy : -1.8343127493 a.u.
Nuclear Repulsion Energy : 0.7055696145 a.u.
------------------------------------
Gradient Norm : 0.0000000210 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.58941 a.u.
( 1 H 1s : 0.40) ( 1 H 2s : 0.18) ( 2 H 1s : 0.40)
( 2 H 2s : 0.18)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.19551 a.u.
( 1 H 1s : -0.15) ( 1 H 2s : -1.93) ( 2 H 1s : 0.15)
( 2 H 2s : 1.93)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.48172 a.u.
( 1 H 1s : 0.70) ( 1 H 2s : -0.73) ( 2 H 1s : 0.70)
( 2 H 2s : -0.73)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.93020 a.u.
( 1 H 1s : 0.77) ( 1 H 2s : -1.61) ( 1 H 1p0 : -0.40)
( 2 H 1s : -0.77) ( 2 H 2s : 1.61) ( 2 H 1p0 : -0.40)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.29182 a.u.
( 1 H 1p+1: -0.58) ( 2 H 1p+1: -0.58)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.29182 a.u.
( 1 H 1p-1: -0.58) ( 2 H 1p-1: -0.58)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.163593561 0.0e+00 1.3e-29 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1635935606538292
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.96588 0.02104 0.00611 0.00001 0.00314 0.00314 0.00020 0.00016 0.00016 0.00016
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.6614715136 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.122863755794 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.126903786231 0.0000000000 0.02833405 0.00776828 0.00000000
2 -1.127000620590 -0.0000968344 0.00059929 0.00019720 0.00578585
3 -1.127000720853 -0.0000001003 0.00001092 0.00000385 0.00034224
4 -1.127000720883 -0.0000000000 0.00000001 0.00000000 0.00000582
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1270007209 a.u.
Electronic Energy : -1.7884722345 a.u.
Nuclear Repulsion Energy : 0.6614715136 a.u.
------------------------------------
Gradient Norm : 0.0000000052 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.57494 a.u.
( 1 H 1s : 0.40) ( 1 H 2s : 0.19) ( 2 H 1s : 0.40)
( 2 H 2s : 0.19)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.18567 a.u.
( 1 H 1s : 0.16) ( 1 H 2s : 1.79) ( 2 H 1s : -0.16)
( 2 H 2s : -1.79)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.49365 a.u.
( 1 H 1s : 0.72) ( 1 H 2s : -0.73) ( 2 H 1s : 0.72)
( 2 H 2s : -0.73)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.89695 a.u.
( 1 H 1s : 0.83) ( 1 H 2s : -1.58) ( 1 H 1p0 : -0.36)
( 2 H 1s : -0.83) ( 2 H 2s : 1.58) ( 2 H 1p0 : -0.36)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.28732 a.u.
( 1 H 1p+1: 0.59) ( 2 H 1p+1: 0.59)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.28732 a.u.
( 1 H 1p-1: -0.59) ( 2 H 1p-1: -0.59)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.162750763 0.0e+00 5.3e-29 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1627507630215634
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.96266 0.02457 0.00613 0.00017 0.00296 0.00296 0.00022 0.00016 0.00016 0.00001
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.5879746788 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.112220769289 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.116294268816 0.0000000000 0.02764470 0.00815308 0.00000000
2 -1.116391213357 -0.0000969445 0.00055877 0.00018803 0.00530657
3 -1.116391300399 -0.0000000870 0.00001254 0.00000437 0.00032420
4 -1.116391300440 -0.0000000000 0.00000000 0.00000000 0.00000699
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1163913004 a.u.
Electronic Energy : -1.7043659792 a.u.
Nuclear Repulsion Energy : 0.5879746788 a.u.
------------------------------------
Gradient Norm : 0.0000000013 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.54852 a.u.
( 1 H 1s : 0.39) ( 1 H 2s : 0.21) ( 2 H 1s : 0.39)
( 2 H 2s : 0.21)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.16505 a.u.
( 1 H 1s : -0.18) ( 1 H 2s : -1.55) ( 2 H 1s : 0.18)
( 2 H 2s : 1.55)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.51701 a.u.
( 1 H 1s : -0.75) ( 1 H 2s : 0.73) ( 2 H 1s : -0.75)
( 2 H 2s : 0.73)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.84044 a.u.
( 1 H 1s : -0.91) ( 1 H 2s : 1.52) ( 1 H 1p0 : 0.27)
( 2 H 1s : 0.91) ( 2 H 2s : -1.52) ( 2 H 1p0 : 0.27)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.28349 a.u.
( 1 H 1p+1: -0.61) ( 2 H 1p+1: -0.61)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.28349 a.u.
( 1 H 1p-1: -0.61) ( 2 H 1p-1: -0.61)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.154081706 0.0e+00 5.4e-30 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1540817061165305
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.95470 0.03339 0.00599 0.00020 0.00257 0.00257 0.00025 0.00016 0.00016 0.00002
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.5291772109 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.095959327021 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.100056242572 0.0000000000 0.02692675 0.00838888 0.00000000
2 -1.100153689745 -0.0000974472 0.00051916 0.00017723 0.00493758
3 -1.100153764817 -0.0000000751 0.00001420 0.00000490 0.00030702
4 -1.100153764873 -0.0000000001 0.00000000 0.00000000 0.00000815
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.1001537649 a.u.
Electronic Energy : -1.6293309758 a.u.
Nuclear Repulsion Energy : 0.5291772109 a.u.
------------------------------------
Gradient Norm : 0.0000000003 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.52504 a.u.
( 1 H 1s : 0.38) ( 1 H 2s : 0.23) ( 2 H 1s : 0.38)
( 2 H 2s : 0.23)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.14359 a.u.
( 1 H 1s : 0.20) ( 1 H 2s : 1.36) ( 2 H 1s : -0.20)
( 2 H 2s : -1.36)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.53961 a.u.
( 1 H 1s : 0.78) ( 1 H 2s : -0.74) ( 2 H 1s : 0.78)
( 2 H 2s : -0.74)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.79480 a.u.
( 1 H 1s : 0.95) ( 1 H 2s : -1.47) ( 1 H 1p0 : -0.20)
( 2 H 1s : -0.95) ( 2 H 2s : 1.47) ( 2 H 1p0 : -0.20)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.28713 a.u.
( 1 H 1p+1: -0.63) ( 2 H 1p+1: -0.63)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.28713 a.u.
( 1 H 1p-1: 0.63) ( 2 H 1p-1: 0.63)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.140073481 0.0e+00 4.2e-30 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1400734808710455
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.94416 0.04503 0.00565 0.00022 0.00217 0.00217 0.00027 0.00016 0.00016 0.00002
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.4409810091 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -1.057057778249 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.061017008512 0.0000000000 0.02498883 0.00837395 0.00000000
2 -1.061111945190 -0.0000949367 0.00043469 0.00015110 0.00450475
3 -1.061111997775 -0.0000000526 0.00001623 0.00000553 0.00026831
4 -1.061111997850 -0.0000000001 0.00000000 0.00000000 0.00000968
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.0611119978 a.u.
Electronic Energy : -1.5020930069 a.u.
Nuclear Repulsion Energy : 0.4409810091 a.u.
------------------------------------
Gradient Norm : 0.0000000004 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.48526 a.u.
( 1 H 1s : -0.36) ( 1 H 2s : -0.27) ( 2 H 1s : -0.36)
( 2 H 2s : -0.27)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.10025 a.u.
( 1 H 1s : 0.24) ( 1 H 2s : 1.08) ( 2 H 1s : -0.24)
( 2 H 2s : -1.08)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.58296 a.u.
( 1 H 1s : 0.83) ( 1 H 2s : -0.75) ( 2 H 1s : 0.83)
( 2 H 2s : -0.75)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.72928 a.u.
( 1 H 1s : -0.96) ( 1 H 2s : 1.37) ( 2 H 1s : 0.96)
( 2 H 2s : -1.37)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.31531 a.u.
( 1 H 1p+1: -0.45) ( 1 H 1p-1: 0.48) ( 2 H 1p+1: -0.45)
( 2 H 1p-1: 0.48)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.31531 a.u.
( 1 H 1p+1: 0.48) ( 1 H 1p-1: 0.45) ( 2 H 1p+1: 0.48)
( 2 H 1p-1: 0.45)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.106855193 0.0e+00 2.4e-30 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.1068551925482597
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.91211 0.07966 0.00454 0.00024 0.00142 0.00142 0.00030 0.00015 0.00015 0.00002
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.3527848073 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.999019668155 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -1.002120347046 0.0000000000 0.02044695 0.00718048 0.00000000
2 -1.002192720639 -0.0000723736 0.00029841 0.00010488 0.00418857
3 -1.002192745431 -0.0000000248 0.00001282 0.00000447 0.00019427
4 -1.002192745478 -0.0000000000 0.00000000 0.00000000 0.00000804
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -1.0021927455 a.u.
Electronic Energy : -1.3549775527 a.u.
Nuclear Repulsion Energy : 0.3527848073 a.u.
------------------------------------
Gradient Norm : 0.0000000003 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.43916 a.u.
( 1 H 1s : 0.33) ( 1 H 2s : 0.32) ( 2 H 1s : 0.33)
( 2 H 2s : 0.32)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: 0.04030 a.u.
( 1 H 1s : -0.28) ( 1 H 2s : -0.81) ( 2 H 1s : 0.28)
( 2 H 2s : 0.81)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.64366 a.u.
( 1 H 1s : -0.91) ( 1 H 2s : 0.76) ( 2 H 1s : -0.91)
( 2 H 2s : 0.76)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.67571 a.u.
( 1 H 1s : 0.94) ( 1 H 2s : -1.25) ( 2 H 1s : -0.94)
( 2 H 2s : 1.25)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.27713 a.u.
( 1 H 1s : 0.24) ( 1 H 1p0 : -0.63) ( 2 H 1s : 0.24)
( 2 H 1p0 : 0.63)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.38302 a.u.
( 1 H 1p-1: -0.68) ( 2 H 1p-1: -0.68)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.061534950 0.0e+00 1.0e-29 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.0615349496254
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.82407 0.17146 0.00246 0.00020 0.00026 0.00065 0.00065 0.00011 0.00011 0.00003
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.2645886055 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.920706243669 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.921886356635 0.0000000000 0.01150627 0.00406041 0.00000000
2 -0.921908589697 -0.0000222331 0.00012300 0.00004281 0.00260878
3 -0.921908594102 -0.0000000044 0.00000367 0.00000128 0.00008607
4 -0.921908594106 -0.0000000000 0.00000000 0.00000000 0.00000249
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.9219085941 a.u.
Electronic Energy : -1.1864971996 a.u.
Nuclear Repulsion Energy : 0.2645886055 a.u.
------------------------------------
Gradient Norm : 0.0000000000 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.38571 a.u.
( 1 H 1s : -0.31) ( 1 H 2s : -0.38) ( 2 H 1s : -0.31)
( 2 H 2s : -0.38)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.03585 a.u.
( 1 H 1s : 0.31) ( 1 H 2s : 0.60) ( 2 H 1s : -0.31)
( 2 H 2s : -0.60)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.65320 a.u.
( 1 H 1s : 0.93) ( 1 H 2s : -1.11) ( 2 H 1s : -0.93)
( 2 H 2s : 1.11)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.67021 a.u.
( 1 H 1s : -0.94) ( 1 H 2s : 0.75) ( 2 H 1s : -0.94)
( 2 H 2s : 0.75)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.34213 a.u.
( 1 H 1p0 : 0.69) ( 2 H 1p0 : -0.69)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.45332 a.u.
( 1 H 1p+1: 0.64) ( 1 H 1p-1: -0.29) ( 2 H 1p+1: 0.64)
( 2 H 1p-1: -0.29)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.017594114 0.0e+00 3.9e-29 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.0175941140438796
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.56605 0.43300 0.00006 0.00010 0.00041 0.00014 0.00014 0.00004 0.00004 0.00001
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.2116708844 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.864890569654 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.865323130286 0.0000000000 0.00662539 0.00233203 0.00000000
2 -0.865330119957 -0.0000069897 0.00002402 0.00000842 0.00153364
3 -0.865330120137 -0.0000000002 0.00000130 0.00000045 0.00001924
4 -0.865330120138 -0.0000000000 0.00000000 0.00000000 0.00000098
*** SCF converged in 4 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.8653301201 a.u.
Electronic Energy : -1.0770010045 a.u.
Nuclear Repulsion Energy : 0.2116708844 a.u.
------------------------------------
Gradient Norm : 0.0000000000 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.34934 a.u.
( 1 H 1s : 0.30) ( 1 H 2s : 0.42) ( 2 H 1s : 0.30)
( 2 H 2s : 0.42)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.08466 a.u.
( 1 H 1s : -0.32) ( 1 H 2s : -0.51) ( 2 H 1s : 0.32)
( 2 H 2s : 0.51)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.62463 a.u.
( 1 H 1s : 0.91) ( 1 H 2s : -0.75) ( 2 H 1s : 0.91)
( 2 H 2s : -0.75)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.66777 a.u.
( 1 H 1s : 0.94) ( 1 H 2s : -1.02) ( 2 H 1s : -0.94)
( 2 H 2s : 1.02)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.45319 a.u.
( 1 H 1p0 : -0.71) ( 2 H 1p0 : 0.71)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46317 a.u.
( 1 H 1p-1: -0.71) ( 2 H 1p-1: -0.71)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -1.003129251 0.0e+00 3.0e-28 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -1.0031292512270575
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.30747 0.69238 0.00002 0.00001 0.00006 0.00003 0.00003 0.00001 0.00001 0.00000
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.1763924036 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.826215752307 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.826444448804 0.0000000000 0.00461717 0.00162670 0.00000000
2 -0.826447843878 -0.0000033951 0.00000214 0.00000076 0.00110952
3 -0.826447843880 -0.0000000000 0.00000021 0.00000007 0.00000161
*** SCF converged in 3 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.8264478439 a.u.
Electronic Energy : -1.0028402475 a.u.
Nuclear Repulsion Energy : 0.1763924036 a.u.
------------------------------------
Gradient Norm : 0.0000002057 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.32328 a.u.
( 1 H 1s : -0.30) ( 1 H 2s : -0.44) ( 2 H 1s : -0.30)
( 2 H 2s : -0.44)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.11576 a.u.
( 1 H 1s : 0.32) ( 1 H 2s : 0.48) ( 2 H 1s : -0.32)
( 2 H 2s : -0.48)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.59484 a.u.
( 1 H 1s : -0.90) ( 1 H 2s : 0.77) ( 2 H 1s : -0.90)
( 2 H 2s : 0.77)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.67845 a.u.
( 1 H 1s : 0.94) ( 1 H 2s : -0.96) ( 2 H 1s : -0.94)
( 2 H 2s : 0.96)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.46177 a.u.
( 1 H 1p+1: 0.70) ( 2 H 1p+1: 0.70)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46177 a.u.
( 1 H 1p-1: -0.70) ( 2 H 1p-1: -0.70)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -0.999550619 0.0e+00 2.9e-28 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -0.9995506185969378
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.15006 0.84991 0.00000 0.00000 0.00001 0.00001 0.00001 0.00000 0.00000 0.00000
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.1511934888 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.799871798054 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.799991332224 0.0000000000 0.00320033 0.00112755 0.00000000
2 -0.799992972433 -0.0000016402 0.00000479 0.00000169 0.00078939
3 -0.799992972441 -0.0000000000 0.00000017 0.00000006 0.00000407
*** SCF converged in 3 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.7999929724 a.u.
Electronic Energy : -0.9511864613 a.u.
Nuclear Repulsion Energy : 0.1511934888 a.u.
------------------------------------
Gradient Norm : 0.0000001692 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.30398 a.u.
( 1 H 1s : 0.30) ( 1 H 2s : 0.45) ( 2 H 1s : 0.30)
( 2 H 2s : 0.45)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.13570 a.u.
( 1 H 1s : -0.32) ( 1 H 2s : -0.46) ( 2 H 1s : 0.32)
( 2 H 2s : 0.46)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.59106 a.u.
( 1 H 1s : 0.91) ( 1 H 2s : -0.80) ( 2 H 1s : 0.91)
( 2 H 2s : -0.80)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.67000 a.u.
( 1 H 1s : -0.94) ( 1 H 2s : 0.92) ( 2 H 1s : 0.94)
( 2 H 2s : -0.92)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.46240 a.u.
( 1 H 1p-1: -0.70) ( 2 H 1p-1: -0.70)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46240 a.u.
( 1 H 1p+1: -0.70) ( 2 H 1p+1: -0.70)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -0.998771056 0.0e+00 3.3e-26 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -0.9987710557416052
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.07018 0.92981 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.1322943027 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.782139853255 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.782197472959 0.0000000000 0.00214017 0.00075393 0.00000000
2 -0.782198208427 -0.0000007355 0.00000243 0.00000086 0.00053449
3 -0.782198208429 -0.0000000000 0.00000007 0.00000003 0.00000212
*** SCF converged in 3 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.7821982084 a.u.
Electronic Energy : -0.9144925112 a.u.
Nuclear Repulsion Energy : 0.1322943027 a.u.
------------------------------------
Gradient Norm : 0.0000000737 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.28967 a.u.
( 1 H 1s : -0.30) ( 1 H 2s : -0.46) ( 2 H 1s : -0.30)
( 2 H 2s : -0.46)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.14868 a.u.
( 1 H 1s : 0.32) ( 1 H 2s : 0.46) ( 2 H 1s : -0.32)
( 2 H 2s : -0.46)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.60146 a.u.
( 1 H 1s : -0.91) ( 1 H 2s : 0.83) ( 2 H 1s : -0.91)
( 2 H 2s : 0.83)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.65436 a.u.
( 1 H 1s : -0.93) ( 1 H 2s : 0.88) ( 2 H 1s : 0.93)
( 2 H 2s : -0.88)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.46422 a.u.
( 1 H 1p0 : -0.71) ( 2 H 1p0 : 0.71)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46443 a.u.
( 1 H 1p-1: 0.71) ( 2 H 1p-1: 0.71)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -0.998606186 0.0e+00 2.2e-25 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -0.9986061861413559
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.03178 0.96822 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.1175949358 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.770215954354 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.770245883432 0.0000000000 0.00149442 0.00052645 0.00000000
2 -0.770246242419 -0.0000003590 0.00000095 0.00000034 0.00037504
*** SCF converged in 2 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.7702462424 a.u.
Electronic Energy : -0.8878411782 a.u.
Nuclear Repulsion Energy : 0.1175949358 a.u.
------------------------------------
Gradient Norm : 0.0000009489 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.27925 a.u.
( 1 H 1s : -0.31) ( 1 H 2s : -0.46) ( 2 H 1s : -0.31)
( 2 H 2s : -0.46)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.15756 a.u.
( 1 H 1s : 0.31) ( 1 H 2s : 0.46) ( 2 H 1s : -0.31)
( 2 H 2s : -0.46)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.61352 a.u.
( 1 H 1s : 0.92) ( 1 H 2s : -0.84) ( 2 H 1s : 0.92)
( 2 H 2s : -0.84)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.64164 a.u.
( 1 H 1s : 0.92) ( 1 H 2s : -0.87) ( 2 H 1s : -0.92)
( 2 H 2s : 0.87)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.46607 a.u.
( 1 H 1p0 : 0.71) ( 2 H 1p0 : -0.71)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46635 a.u.
( 1 H 1p-1: -0.71) ( 2 H 1p-1: -0.71)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -0.998569095 0.0e+00 2.4e-24 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -0.9985690947312202
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.01383 0.98617 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/CC-PVDZ
Molecular Basis (Atomic Basis)
================================
Basis: CC-PVDZ
Atom Contracted GTOs Primitive GTOs
H (2S,1P) (4S,1P)
Contracted Basis Functions : 10
Primitive Basis Functions : 14
Self Consistent Field Driver Setup
====================================
Wave Function Model : Spin-Restricted Hartree-Fock
Initial Guess Model : Superposition of Atomic Densities
Convergence Accelerator : Two Level Direct Inversion of Iterative Subspace
Max. Number of Iterations : 50
Max. Number of Error Vectors : 10
Convergence Threshold : 1.0e-06
ERI Screening Scheme : Cauchy Schwarz + Density
ERI Screening Mode : Dynamic
ERI Screening Threshold : 1.0e-12
Linear Dependence Threshold : 1.0e-06
* Info * Nuclear repulsion energy: 0.1058354422 a.u.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
* Info * SAD initial guess computed in 0.00 sec.
* Info * Starting Reduced Basis SCF calculation...
* Info * ...done. SCF energy in reduced basis set: -0.762026297205 a.u. Time: 0.01 sec.
* Info * Overlap matrix computed in 0.00 sec.
* Info * Kinetic energy matrix computed in 0.00 sec.
* Info * Nuclear potential matrix computed in 0.00 sec.
* Info * Orthogonalization matrix computed in 0.00 sec.
Iter. | Hartree-Fock Energy | Energy Change | Gradient Norm | Max. Gradient | Density Change
--------------------------------------------------------------------------------------------
1 -0.762044195778 0.0000000000 0.00112546 0.00039650 0.00000000
2 -0.762044399480 -0.0000002037 0.00000038 0.00000013 0.00028293
*** SCF converged in 2 iterations. Time: 0.01 sec.
Spin-Restricted Hartree-Fock:
-----------------------------
Total Energy : -0.7620443995 a.u.
Electronic Energy : -0.8678798417 a.u.
Nuclear Repulsion Energy : 0.1058354422 a.u.
------------------------------------
Gradient Norm : 0.0000003765 a.u.
Ground State Information
------------------------
Charge of Molecule : 0.0
Multiplicity (2S+1) : 1.0
Magnetic Quantum Number (M_S) : 0.0
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 1:
--------------------------
Occupation: 2.000 Energy: -0.27168 a.u.
( 1 H 1s : -0.31) ( 1 H 2s : -0.46) ( 2 H 1s : -0.31)
( 2 H 2s : -0.46)
Molecular Orbital No. 2:
--------------------------
Occupation: 0.000 Energy: -0.16408 a.u.
( 1 H 1s : -0.31) ( 1 H 2s : -0.46) ( 2 H 1s : 0.31)
( 2 H 2s : 0.46)
Molecular Orbital No. 3:
--------------------------
Occupation: 0.000 Energy: 0.62166 a.u.
( 1 H 1s : 0.92) ( 1 H 2s : -0.85) ( 2 H 1s : 0.92)
( 2 H 2s : -0.85)
Molecular Orbital No. 4:
--------------------------
Occupation: 0.000 Energy: 0.63412 a.u.
( 1 H 1s : 0.92) ( 1 H 2s : -0.86) ( 2 H 1s : -0.92)
( 2 H 2s : 0.86)
Molecular Orbital No. 5:
--------------------------
Occupation: 0.000 Energy: 1.46736 a.u.
( 1 H 1p0 : 0.71) ( 2 H 1p0 : -0.71)
Molecular Orbital No. 6:
--------------------------
Occupation: 0.000 Energy: 1.46760 a.u.
( 1 H 1p+1: 0.57) ( 1 H 1p-1: -0.41) ( 2 H 1p+1: 0.57)
( 2 H 1p-1: -0.41)
Configuration Interaction Driver
==================================
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 0
Number of active orbitals: 10
Number of virtual orbitals: 0
This is a CASSCF wavefunction: CAS(2,10)
CI expansion:
-------------
Number of determinants: 55
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
Solved by explicit diagonalization
CI Iterations
-------------
Iter. | Average Energy | E. Change | Grad. Norm | Time
----------------------------------------------------------
1 -0.998559877 0.0e+00 2.7e-24 0:00:00
** Convergence reached in 1 iterations
Final results
-------------
* State 1
- Energy: -0.9985598772014368
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.00568 0.99432 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000 0.00000
plt.figure(figsize=(6,4))
plt.title('Energy during H$_2$ dissociation')
x = np.array(distlist)
y = np.array(E_FCI)
z = np.array(E_hf)
plt.axhline(y = 0, color = "k", linestyle = 'dashed')
plt.plot(x,y, label='FullCI')
plt.plot(x,z, label='RHF')
plt.arrow(x[3], z[3], 0, y[3]-z[3], length_includes_head = True, head_width = 0.05, head_length = 0.01, facecolor='r', edgecolor='r')
plt.annotate('Dynamical correlation', xy = (x[3]+0.1, 0.5*(z[3]+y[3])), color = 'r')
plt.arrow(x[-1], z[-1], 0, y[-1]-z[-1], length_includes_head = True, head_width = 0.05, head_length = 0.01, facecolor='r', edgecolor='r')
plt.annotate('Static correlation', xy = (x[-1]-1.25, 0.5*(z[-1]+y[-1])), color = 'r')
plt.xlabel("H-H distance (Å)")
plt.ylabel("Energy (Ha)")
plt.legend()
plt.tight_layout(); plt.show()

Following Löwdin, we usually call correlation the difference between HF and FullCI. At short distance, this corresponds to our intuitive idea of electrons dynamically avoiding each other. However, at long distance, we expect one electron on each hydrogen atom, and there is no dynamical correlation. The error of Hartree-Fock is that even if one electron is on the first hydrogen, the second one is still equally shared between both hydrogens. We can visualize this using the functions written in “Electron correlation” chapter:
n=100
norb = space.n_active
origin = np.zeros((n, 3))
coords = np.zeros((n, 3))
def get_orbital_values(coords, molecule, basis, mol_orbs):
orbital_values = []
for i in range(norb):
values = np.array(vis_drv.get_mo(coords, molecule, basis, mol_orbs, i, "alpha"))
orbital_values.append(values)
return orbital_values
def get_n12(ci_twopart_denmat, orbital_values_1, orbital_values_2):
hf_twopart_denmat = np.zeros((norb,norb,norb,norb))
hf_twopart_denmat[0,0,0,0] = 2.0 # first MO is doubly occupied
# Two-particle densities for FCI
n12_hf = np.zeros(n)
n12_fci = np.zeros(n)
for i in range(norb):
for j in range(norb):
for k in range(norb):
for l in range(norb):
n12_hf += (
hf_twopart_denmat[i, j, k, l]
* orbital_values_1[i]
* orbital_values_1[j]
* orbital_values_2[k]
* orbital_values_2[l]
)
n12_fci += (
ci_twopart_denmat[i, j, k, l]
* orbital_values_1[i]
* orbital_values_1[j]
* orbital_values_2[k]
* orbital_values_2[l]
)
return n12_hf, n12_fci
z = np.linspace(-1, 2, n)
coords[:, 2] = z
vis_drv = vlx.VisualizationDriver()
orbital_values = get_orbital_values(coords/bohr_to_Å, eq_molecule, basis, eq_orbitals)
orbital_values_at_H1 = get_orbital_values([[0, 0, 0]], eq_molecule, basis, eq_orbitals)
n12_hf, n12_fci = get_n12(eq_2density, orbital_values_at_H1, orbital_values)
plt.figure(figsize=(6,4))
plt.title('Pair density at equilibrium distance')
plt.plot(z,n12_hf, label='HF')
plt.plot(z,n12_fci, label='FullCI')
plt.axvline(x = 0, color = 'k', linestyle = ':')
plt.xlabel("Internuclear axis coordinate (Å)")
plt.ylabel("Two-particle density (a.u.)")
plt.legend()
plt.tight_layout(); plt.show()
z = np.linspace(-2, 8, n)
coords[:, 2] = z
orbital_values = get_orbital_values(coords/bohr_to_Å, molecule, basis, scf_drv.mol_orbs)
orbital_values_at_H1 = get_orbital_values([[0, 0, 0]], molecule, basis, scf_drv.mol_orbs)
ci_twopart_denmat = ci_drv.get_active_2body_density(0)
n12_hf, n12_fci = get_n12(ci_twopart_denmat, orbital_values_at_H1, orbital_values)
plt.figure(figsize=(6,4))
plt.title('Pair density at dissociation')
plt.plot(z,n12_hf, label='HF')
plt.plot(z,n12_fci, label='FullCI')
plt.axvline(x = 0, color = 'k', linestyle = ':')
plt.xlabel("Internuclear axis coordinate (Å)")
plt.ylabel("Two-particle density (a.u.)")
plt.legend()
plt.tight_layout(); plt.show()

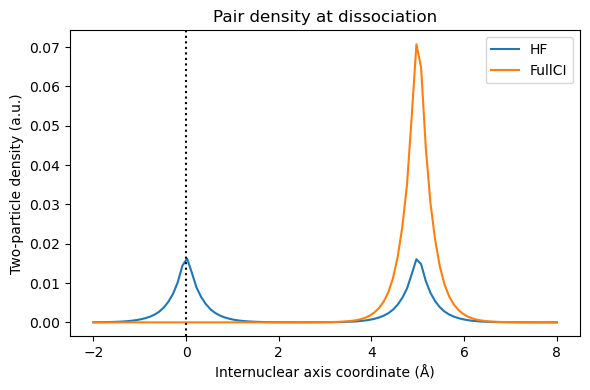
This correlation is called by contrast “static” or “non-dynamical” or sometimes simply “strong” correlation. In practice both type of correlation have the same physical origin. If one electron is on one atom, the second electron is more likely to be on the second one. However, at long distances, the presence of one electron on one nucleus guarantees that the second electron is on the other one. Both types of correlation are also treated with the same excited determinants. But the distinction is useful as they may guide the way we calculate such systems.
While H\(_2\) is the most used example, in practice whenever one breaks a bond homolytically (i.e. at dissociation each fragment gets one electron), static correlation will be a problem. However, in chemistry, we rarely break bonds in this way since the energy cost of doing so is simply too high. Even transition states typically form a bond while breaking another one, rarely getting a truly homolytic stage. Some exceptions are weaker bonds, like \(\pi\) bonds that can be broken by a twisting motion for example, as well as excited states reaction where the energies are typically higher.
At equilibrium distance#
While true bond breakings are rare in chemistry, it turns out that static/strong correlation can occur even at equilibrium distance. One way to easily detect such thing is by looking at occupation numbers in a CI (or MCSCF) calculations. As explained in the MCSCF chapter, correlation will show as a difference in occupation number from the integers we expect. For standard dynamical correlation, the occupation numbers will typically be around 1.98 for occupied orbitals and 0.02 for virtual orbitals, showing that the CI expansion contains some configurations exciting from the occupied to the virtual, but that the dominant configuration is still the Hartree-Fock reference.
However, in some cases, we get higher deviations from 2 and 0, for example let’s look at ethene, including the carbon-carbon \(\sigma\) and \(\pi\) bonds in the active space:
mol_str = """
H 0.000000 0.923274 1.238289
H 0.000000 -0.923274 1.238289
H 0.000000 0.923274 -1.238289
H 0.000000 -0.923274 -1.238289
C 0.000000 0.000000 0.668188
C 0.000000 0.000000 -0.668188
"""
molecule = vlx.Molecule.read_str(mol_str)
molecule.set_charge(0)
molecule.set_multiplicity(1)
basis = vlx.MolecularBasis.read(molecule,"def2-sv(p)")
eht = mtp.OrbitalGuess()
guessorb = eht.compute(molecule,basis)
space = mtp.OrbSpace(molecule,guessorb)
space.cas_orbitals([5,6,8,13])
mcscfdrv = mtp.McscfDriver()
mcresults = mcscfdrv.compute(molecule,basis,space)
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/DEF2-SV_P_
Molecular Basis (Atomic Basis)
================================
Basis: DEF2-SV(P)
Atom Contracted GTOs Primitive GTOs
H (2S) (4S)
C (3S,2P,1D) (7S,4P,1D)
Contracted Basis Functions : 36
Primitive Basis Functions : 64
Multi-Configurational Self-Consistent Field Driver
====================================================
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
State-specific calculation
Max. iterations : 50
BFGS window : 5
Convergence thresholds:
- Energy change : 1e-08
- Gradient norm : 0.0001
Integrals in memory
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 6
Number of active orbitals: 4
Number of virtual orbitals: 26
This is a CASSCF wavefunction: CAS(4,4)
CI expansion:
-------------
Number of determinants: 21
MCSCF Iterations
---------------------------------------------------------------------
Iter. | Average Energy | E. Change | Grad. Norm | CI Iter. | Time
---------------------------------------------------------------------
1 -77.737843601 0.0e+00 1.4e+00 1 0:00:00
2 -77.976388879 -2.4e-01 4.4e-01 1 0:00:00
3 -78.016080942 -4.0e-02 1.0e-01 1 0:00:00
4 -78.019128794 -3.0e-03 2.3e-02 1 0:00:00
5 -78.019331249 -2.0e-04 7.0e-03 1 0:00:00
6 -78.019362607 -3.1e-05 2.9e-03 1 0:00:00
7 -78.019365983 -3.4e-06 1.3e-03 1 0:00:00
8 -78.019366505 -5.2e-07 3.4e-04 1 0:00:00
9 -78.019366536 -3.1e-08 8.9e-05 1 0:00:00
10 -78.019366538 -2.1e-09 1.8e-05 1 0:00:00
** Convergence reached in 10 iterations
11 -78.019366538 -6.7e-11 4.0e-06 1 0:00:00
Final results
-------------
* State 1
- Energy: -78.01936653764186
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.98365 1.92629 0.07350 0.01656
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 4:
--------------------------
Occupation: 2.000 Energy: -0.77861 a.u.
( 1 H 1s : -0.19) ( 2 H 1s : -0.19) ( 3 H 1s : 0.19)
( 4 H 1s : 0.19) ( 5 C 1s : 0.15) ( 5 C 2s : -0.32)
( 5 C 1p0 : -0.17) ( 6 C 1s : -0.15) ( 6 C 2s : 0.32)
( 6 C 1p0 : -0.17)
Molecular Orbital No. 5:
--------------------------
Occupation: 2.000 Energy: -0.63704 a.u.
( 1 H 1s : -0.20) ( 2 H 1s : 0.20) ( 3 H 1s : -0.20)
( 4 H 1s : 0.20) ( 5 C 1p-1: -0.32) ( 6 C 1p-1: -0.32)
Molecular Orbital No. 6:
--------------------------
Occupation: 2.000 Energy: -0.50339 a.u.
( 1 H 1s : 0.25) ( 2 H 1s : -0.25) ( 3 H 1s : -0.25)
( 4 H 1s : 0.25) ( 5 C 1p-1: 0.30) ( 5 C 2p-1: 0.16)
( 6 C 1p-1: -0.30) ( 6 C 2p-1: -0.16)
Molecular Orbital No. 7:
--------------------------
Occupation: 1.984 Energy: -0.74951 a.u.
( 5 C 2s : 0.28) ( 5 C 1p0 : -0.39) ( 6 C 2s : 0.28)
( 6 C 1p0 : 0.39)
Molecular Orbital No. 8:
--------------------------
Occupation: 1.926 Energy: -0.36398 a.u.
( 5 C 1p+1: -0.40) ( 5 C 2p+1: -0.29) ( 6 C 1p+1: -0.40)
( 6 C 2p+1: -0.29)
Molecular Orbital No. 9:
--------------------------
Occupation: 0.074 Energy: 0.26140 a.u.
( 5 C 1p+1: 0.63) ( 5 C 2p+1: 0.31) ( 6 C 1p+1: -0.63)
( 6 C 2p+1: -0.31)
Molecular Orbital No. 10:
--------------------------
Occupation: 0.017 Energy: 0.96850 a.u.
( 5 C 1s : -0.22) ( 5 C 2s : 0.91) ( 5 C 1p0 : -0.84)
( 5 C 2p0 : -0.24) ( 6 C 1s : 0.22) ( 6 C 2s : -0.91)
( 6 C 1p0 : -0.84) ( 6 C 2p0 : -0.24)
Molecular Orbital No. 11:
--------------------------
Occupation: 0.000 Energy: 0.20754 a.u.
( 1 H 2s : -1.12) ( 2 H 2s : -1.12) ( 3 H 2s : -1.12)
( 4 H 2s : -1.12) ( 5 C 3s : 1.60) ( 5 C 2p0 : 0.57)
( 6 C 3s : 1.60) ( 6 C 2p0 : -0.57)
Molecular Orbital No. 12:
--------------------------
Occupation: 0.000 Energy: 0.23435 a.u.
( 1 H 2s : -1.16) ( 2 H 2s : 1.16) ( 3 H 2s : -1.16)
( 4 H 2s : 1.16) ( 5 C 1p-1: 0.22) ( 5 C 2p-1: 0.76)
( 6 C 1p-1: 0.22) ( 6 C 2p-1: 0.76)
Molecular Orbital No. 13:
--------------------------
Occupation: 0.000 Energy: 0.25745 a.u.
( 1 H 2s : -1.29) ( 2 H 2s : -1.29) ( 3 H 2s : 1.29)
( 4 H 2s : 1.29) ( 5 C 3s : 1.52) ( 5 C 1p0 : 0.18)
( 5 C 2p0 : 0.70) ( 6 C 3s : -1.52) ( 6 C 1p0 : 0.18)
( 6 C 2p0 : 0.70)
Dipole moment for state 1
---------------------------
X : 0.000000 a.u. 0.000000 Debye
Y : -0.000001 a.u. -0.000004 Debye
Z : 0.000001 a.u. 0.000002 Debye
Total : 0.000002 a.u. 0.000004 Debye
Total MCSCF time: 00:00:00
viewer = mtp.OrbitalViewer()
viewer.plot(molecule, basis, space)
print(mcscfdrv.get_natural_occupations())
[1.98365468 1.92628668 0.07349929 0.01655935]
The first and last orbitals are the \(\sigma\) - \(\sigma^*\) pairs and have an occupation number around 1.98 as expected. However, the second and third, the \(\pi\) - \(\pi^*\), have an occupation number of 1.93, which, while still far from a fully broken bond, differ more than expected from 2 and 0. Were we to perform a scan elongating the C-C bond, we would see that the \(\pi\) bond occupation numbers are simply shifted by around 0.55 Å.
from IPython.display import Image
Image(filename="../../img/tutorials/ethene_bond_breaking.png", width=400)
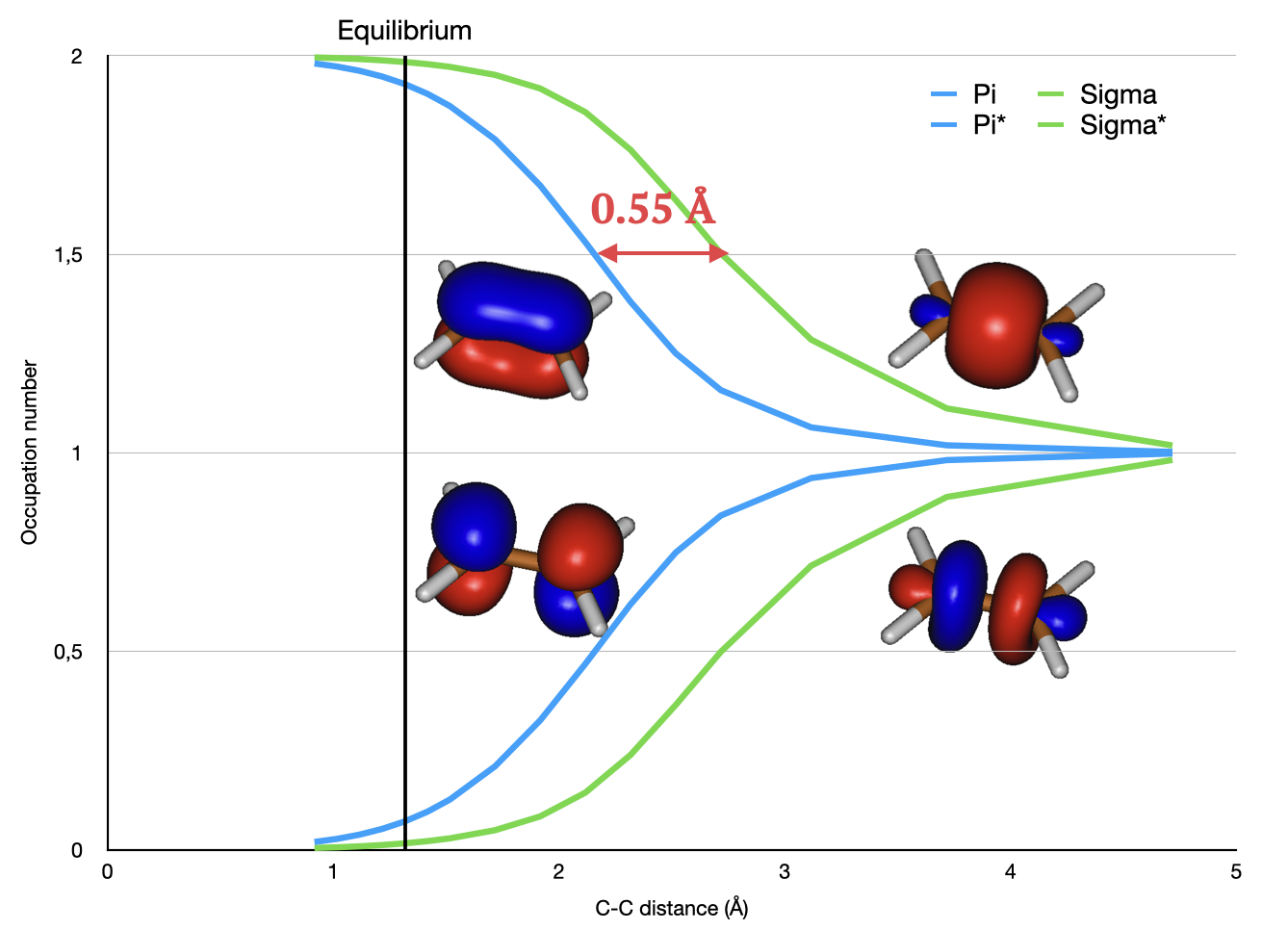
The reason for this is that at the equilibrium distance, the \(\pi\) bond is indeed stretched. It is visible if we look at the radial profile of the orbitals:
coords = np.zeros((n, 3))
z = np.linspace(-1.5, 1.5, n)
coords[:, 2] = z
coords_shifted = np.zeros((n, 3))
coords_shifted[:, 0] = 0.35 # Pi orbitals are 0 in the xy plane, so we compute them at shifted x value
coords_shifted[:, 2] = z
sigma = np.array(vis_drv.get_mo(coords/bohr_to_Å, molecule, basis, space.molecular_orbitals, 6, "alpha"))
pi = np.array(vis_drv.get_mo(coords_shifted/bohr_to_Å, molecule, basis, space.molecular_orbitals, 7, "alpha"))
# Make sure the sign is the same in the middle of the bond
sigma*= np.sign(sigma[50])
pi*= np.sign(pi[50])
sigma_max = abs(z[np.argmax(sigma)])
pi_max = abs(z[np.argmax(pi)])
plt.figure(figsize=(6,4))
plt.title('Absolute value of the orbital profiles')
plt.plot(z,sigma, label='$\sigma$')
plt.plot(z,pi, label='$\pi$')
plt.xlabel("Internuclear axis coordinate (Å)")
plt.ylabel("Two-particle density (a.u.)")
plt.axhline(y = 0, color = "k")
plt.axvline(x = 0.668188, color = 'k', linestyle = ':')
plt.axvline(x = -0.668188, color = 'k', linestyle = ':')
plt.legend()
plt.tight_layout(); plt.show()
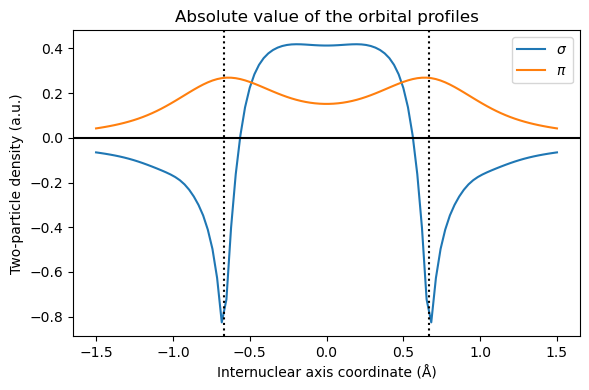
It is clear in this orbital profile that the distance between the maxima of the \(\sigma\) is shorter than that of the \(\pi\). This also explains why double bonds are shorter than single bonds. The \(\pi\) bond wants to be much shorter but is prevented by the Fermi repulsion in the \(\sigma\) bond. The resulting bond length is shorter than a pure \(\sigma\) but still too long for the \(\pi\) bond that becomes stretched and thus displays some light amount of static correlation.
This situation is very general, and whenever bonds get “frustrated” due to the electronic repulsion of other bonds, they may be stretched and display static correlation even in the equilibrium structure. While the \(\pi\) bond of ethene is still arguably a mild case, some can get much more pronounced such as the N-N bond is NO dimer or most metal-metal bonds, including the famous chromium dimer:
mol_str = """
Cr 0.0 0.0 -0.825
Cr 0.0 0.0 0.825
"""
molecule = vlx.Molecule.read_str(mol_str)
molecule.set_charge(0)
molecule.set_multiplicity(1)
basis = vlx.MolecularBasis.read(molecule,"def2-sv(p)")
eht = mtp.OrbitalGuess()
guessorb = eht.compute(molecule,basis)
space = mtp.OrbSpace(molecule,guessorb)
space.cas(12,12)
mcscfdrv = mtp.McscfDriver()
mcresults = mcscfdrv.compute(molecule,basis,space)
* Info * Reading basis set from file: /opt/miniconda3/envs/echem/lib/python3.10/site-packages/veloxchem/basis/DEF2-SV_P_
Molecular Basis (Atomic Basis)
================================
Basis: DEF2-SV(P)
Atom Contracted GTOs Primitive GTOs
Cr (5S,3P,2D) (14S,9P,5D)
Contracted Basis Functions : 48
Primitive Basis Functions : 132
Multi-Configurational Self-Consistent Field Driver
====================================================
╭────────────────────────────────────╮
│ Driver settings │
╰────────────────────────────────────╯
State-specific calculation
Max. iterations : 50
BFGS window : 5
Convergence thresholds:
- Energy change : 1e-08
- Gradient norm : 0.0001
Integrals in memory
Active space definition:
------------------------
Number of inactive (occupied) orbitals: 18
Number of active orbitals: 12
Number of virtual orbitals: 18
This is a CASSCF wavefunction: CAS(12,12)
CI expansion:
-------------
Number of determinants: 427350
MCSCF Iterations
---------------------------------------------------------------------
Iter. | Average Energy | E. Change | Grad. Norm | CI Iter. | Time
---------------------------------------------------------------------
Convergence difficulties in CI, increasing the max number of iteration to 60
1 -2086.226771556 0.0e+00 1.0e+00 40 0:00:08
2 -2086.292283561 -6.6e-02 2.2e-01 31 0:00:15
3 -2086.303171093 -1.1e-02 1.1e-01 3 0:00:01
4 -2086.305468123 -2.3e-03 3.6e-02 5 0:00:02
5 -2086.305826484 -3.6e-04 8.9e-03 9 0:00:03
6 -2086.305862979 -3.6e-05 2.9e-03 9 0:00:03
7 -2086.305869470 -6.5e-06 1.1e-03 12 0:00:04
8 -2086.305870546 -1.1e-06 5.6e-04 13 0:00:04
9 -2086.305870683 -1.4e-07 2.4e-04 13 0:00:04
10 -2086.305870726 -4.3e-08 8.9e-05 17 0:00:05
11 -2086.305870729 -3.5e-09 3.8e-05 21 0:00:07
** Convergence reached in 11 iterations
Convergence difficulties in CI, increasing the max number of iteration to 80
12 -2086.305870729 4.1e-10 1.5e-05 60 0:00:20
Final results
-------------
* State 1
- Energy: -2086.3058707286036
- S^2 : 0.00 (multiplicity = 1.0 )
- Natural orbitals
1.91211 1.81416 1.81416 1.78608 1.59981 1.59981 0.40095 0.40095 0.20939 0.18598 0.18598 0.09065
Spin Restricted Orbitals
------------------------
Molecular Orbital No. 17:
--------------------------
Occupation: 2.000 Energy: -2.11529 a.u.
( 1 Cr 1p-1: -0.27) ( 1 Cr 2p-1: 0.76) ( 2 Cr 1p-1: 0.27)
( 2 Cr 2p-1: -0.76)
Molecular Orbital No. 18:
--------------------------
Occupation: 2.000 Energy: -2.03812 a.u.
( 1 Cr 1p0 : -0.28) ( 1 Cr 2p0 : 0.79) ( 2 Cr 1p0 : -0.28)
( 2 Cr 2p0 : 0.79)
Molecular Orbital No. 19:
--------------------------
Occupation: 1.912 Energy: -0.20539 a.u.
( 1 Cr 3s : 0.33) ( 1 Cr 4s : -0.41) ( 1 Cr 5s : -0.19)
( 2 Cr 3s : 0.33) ( 2 Cr 4s : -0.41) ( 2 Cr 5s : -0.19)
Molecular Orbital No. 20:
--------------------------
Occupation: 1.814 Energy: -0.28978 a.u.
( 1 Cr 1d+1: -0.54) ( 1 Cr 2d+1: -0.21) ( 2 Cr 1d+1: 0.54)
( 2 Cr 2d+1: 0.21)
Molecular Orbital No. 21:
--------------------------
Occupation: 1.814 Energy: -0.28978 a.u.
( 1 Cr 1d-1: 0.54) ( 1 Cr 2d-1: 0.21) ( 2 Cr 1d-1: -0.54)
( 2 Cr 2d-1: -0.21)
Molecular Orbital No. 22:
--------------------------
Occupation: 1.786 Energy: -0.26153 a.u.
( 1 Cr 1d0 : -0.59) ( 1 Cr 2d0 : -0.18) ( 2 Cr 1d0 : -0.59)
( 2 Cr 2d0 : -0.18)
Molecular Orbital No. 23:
--------------------------
Occupation: 1.600 Energy: -0.15529 a.u.
( 1 Cr 1d+2: -0.53) ( 1 Cr 1d-2: 0.16) ( 1 Cr 2d+2: -0.23)
( 2 Cr 1d+2: -0.53) ( 2 Cr 1d-2: 0.16) ( 2 Cr 2d+2: -0.23)
Molecular Orbital No. 24:
--------------------------
Occupation: 1.600 Energy: -0.15529 a.u.
( 1 Cr 1d+2: 0.16) ( 1 Cr 1d-2: 0.53) ( 1 Cr 2d-2: 0.23)
( 2 Cr 1d+2: 0.16) ( 2 Cr 1d-2: 0.53) ( 2 Cr 2d-2: 0.23)
Molecular Orbital No. 25:
--------------------------
Occupation: 0.401 Energy: 0.12626 a.u.
( 1 Cr 1d-2: 0.63) ( 1 Cr 2d-2: 0.18) ( 2 Cr 1d-2: -0.63)
( 2 Cr 2d-2: -0.18)
Molecular Orbital No. 26:
--------------------------
Occupation: 0.401 Energy: 0.12626 a.u.
( 1 Cr 1d+2: 0.63) ( 1 Cr 2d+2: 0.18) ( 2 Cr 1d+2: -0.63)
( 2 Cr 2d+2: -0.18)
Molecular Orbital No. 27:
--------------------------
Occupation: 0.209 Energy: 0.28096 a.u.
( 1 Cr 1d0 : 0.70) ( 2 Cr 1d0 : -0.70)
Molecular Orbital No. 28:
--------------------------
Occupation: 0.186 Energy: 0.27552 a.u.
( 1 Cr 1d-1: 0.69) ( 1 Cr 2d-1: 0.18) ( 2 Cr 1d-1: 0.69)
( 2 Cr 2d-1: 0.18)
Molecular Orbital No. 29:
--------------------------
Occupation: 0.186 Energy: 0.27552 a.u.
( 1 Cr 1d+1: 0.69) ( 1 Cr 2d+1: 0.18) ( 2 Cr 1d+1: 0.69)
( 2 Cr 2d+1: 0.18)
Molecular Orbital No. 30:
--------------------------
Occupation: 0.091 Energy: 0.07388 a.u.
( 1 Cr 3s : -0.34) ( 1 Cr 4s : 0.51) ( 1 Cr 5s : 0.19)
( 1 Cr 3p0 : -0.46) ( 2 Cr 3s : 0.34) ( 2 Cr 4s : -0.51)
( 2 Cr 5s : -0.19) ( 2 Cr 3p0 : -0.46)
Molecular Orbital No. 31:
--------------------------
Occupation: 0.000 Energy: 0.10910 a.u.
( 1 Cr 4s : 3.68) ( 1 Cr 5s : -3.87) ( 1 Cr 3p0 : 0.91)
( 1 Cr 2d0 : 0.17) ( 2 Cr 4s : -3.68) ( 2 Cr 5s : 3.87)
( 2 Cr 3p0 : 0.91) ( 2 Cr 2d0 : -0.17)
Molecular Orbital No. 32:
--------------------------
Occupation: 0.000 Energy: 0.12124 a.u.
( 1 Cr 3s : -0.30) ( 1 Cr 4s : 1.24) ( 1 Cr 5s : -1.12)
( 2 Cr 3s : -0.30) ( 2 Cr 4s : 1.24) ( 2 Cr 5s : -1.12)
Dipole moment for state 1
---------------------------
X : 0.000001 a.u. 0.000002 Debye
Y : -0.000000 a.u. -0.000001 Debye
Z : 0.000042 a.u. 0.000106 Debye
Total : 0.000042 a.u. 0.000106 Debye
Total MCSCF time: 00:01:22
print(mcscfdrv.get_natural_occupations())
[1.91210501 1.81415618 1.81415604 1.78607583 1.59980801 1.59980765
0.40094968 0.40094931 0.2093917 0.18597598 0.18597582 0.09064879]