Optimization and normal modes#
In this section we will see how to visualize geometry optimization trajectories, as well as molecular vibration modes using py3Dmol.
Geometry optimization#
import veloxchem as vlx
import py3Dmol as p3d
from matplotlib import pyplot as plt
import numpy as np
import ipywidgets
from veloxchem.veloxchemlib import bohr_in_angstroms
import h5py
Let’s assume we have run the geometry optimization and saved all the steps in xyz
format in a file. To animate the optimization trajectory we first need a routine which reads the configurations from file.
def read_xyz_file(file_name):
"""Reads all the configurations from an xyz geometry optimization file."""
xyz_file = open(file_name, "r")
data = xyz_file.read()
xyz_file.close()
xyz_file = open(file_name, "r")
i = 0
steps = []
energies = []
for line in xyz_file:
if "Energy" in line:
steps.append(i)
i += 1
parts = line.split()
energy = float(parts[-1])
energies.append(energy)
xyz_file.close()
return data, steps, energies
Using this routine, we can plot the energies during optimization, as well as animate the trajectory.
xyz_file_name = '../../data/md/kahweol_optim.xyz'
xyz_data, steps, energies = read_xyz_file(xyz_file_name)
# Plot the energies
plt.figure(figsize=(7,4))
plt.plot(steps, energies,'o--')
plt.xlabel('Iteration')
plt.ylabel('Energy (H)')
plt.title("Kahweol XTB optimization")
plt.tight_layout(); plt.show()
# and animate the optimization
viewer = p3d.view(width=600, height=300)
viewer.addModelsAsFrames(xyz_data)
viewer.animate({"loop": "forward"})
viewer.setViewStyle({"style": "outline", "width": 0.05})
viewer.setStyle({"stick": {}, "sphere": {"scale": 0.25}})
viewer.show()
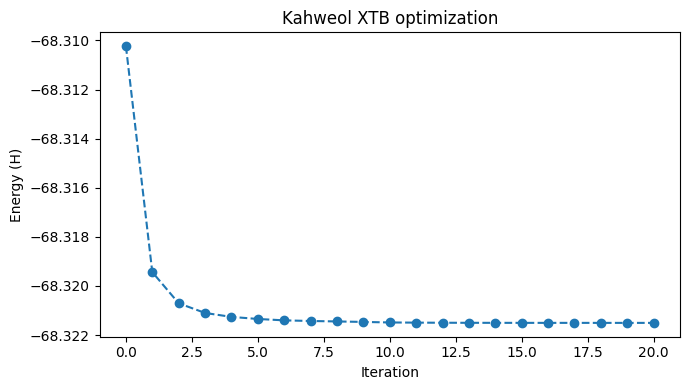
3Dmol.js failed to load for some reason. Please check your browser console for error messages.
If instead we would like to see each configuration individually, we can create an interactive widget with a slider. As in the previous section, we need a routine which reads an individual configuration at a time and returns the corresponding py3Dmol viewer.
def read_xyz_index(file_name, index=0):
"""Reads one configuration defined by its index from the xyz optimization file."""
xyz_file = open(file_name, "r")
current_index = 0
data = ""
# Read the number of atoms from the first line
line = xyz_file.readline()
natm = line.split()[0]
data += line
for line in xyz_file:
if index == current_index:
data += line
if 'Energy' in line:
parts = line.split()
energy = float(parts[-1])
if natm in line:
parts = line.split()
if len(parts) == 1:
if index == current_index:
break
current_index += 1
xyz_file.close()
return data, energy
def return_viewer(file_name, step=0):
xyz_data_i, energy_i = read_xyz_index(file_name, step)
# Uncomment if you would also like to see the energy plot
# or comment out if you would like to skip this.
plt.figure(figsize=(7,4))
plt.plot(steps, energies, 'o--')
plt.plot(step, energy_i, 'o', markersize=15)
plt.xlabel('Iteration')
plt.ylabel('Energy (H)')
plt.title("Kahweol XTB optimization")
plt.tight_layout(); plt.show()
viewer = p3d.view(width=600, height=300)
viewer.addModel(xyz_data_i)
viewer.setViewStyle({"style": "outline", "width": 0.05})
viewer.setStyle({"stick": {}, "sphere": {"scale": 0.25}})
viewer.show()
total_steps = steps[-1] # number of configurations in the trajectory file
# Note that the slider only works in a Jupyter notebook.
ipywidgets.interact(return_viewer, file_name=xyz_file_name,
step=ipywidgets.IntSlider(min=0, max=total_steps, step=1, value=3))
<function __main__.return_viewer(file_name, step=0)>
Vibrational normal modes#
Now let’s see how to animate and visualize vibrational normal modes. For this, we will need to determine the molecular Hessian and perform a vibrational analysis. Let’s assume we have already carried out the Hessian calculation and we saved the result in a h5 checkpoint file. We will then start by reading the Hessian from file.
xtb_drv = vlx.XtbDriver()
xtb_hessian_drv = vlx.XtbHessianDriver(xtb_drv)
fname = '../../data/md/kahweol_xtb_hessian.h5'
hf = h5py.File(fname, "r")
xtb_hessian_drv.hessian = np.array(hf.get('kahweol_hessian'))
xtb_hessian_drv.dipole_gradient = np.array(hf.get('kahweol_dipolegrad'))
hf.close()
In order to perform a vibrational analysis we need a Molecule
object which stores the optimized geometry. To create the Molecule
object we will read the last geometry from the xyz
file we used in the previous subsection.
# read the last configuration from file
kahweol_xyz, opt_energy = read_xyz_index(file_name=xyz_file_name, index=total_steps)
# create the Mlecul object
xtb_opt_kahweol = vlx.Molecule.read_xyz_string(kahweol_xyz)
# perform vibrational analysis -- diagonalize Hessian, extract frequencies and normal modes
# and calculate IR intensities.
xtb_hessian_drv.vibrational_analysis(xtb_opt_kahweol)
Show code cell output
Vibrational Analysis
======================
Vibrational Mode 1
----------------------------------------------------
Harmonic frequency: 44.72 cm**-1
Reduced mass: 4.8483 amu
Force constant: 0.0057 mdyne/A
IR intensity: 1.4717 km/mol
Normal mode:
X Y Z
1 O -0.0064 -0.0451 0.0007
2 O 0.2050 0.2565 0.2331
3 O -0.0411 0.0481 0.1018
4 C -0.0021 -0.0344 -0.0574
5 C -0.0149 -0.0290 -0.0615
6 C 0.0207 -0.0511 -0.0214
7 C 0.0288 -0.0421 -0.0461
8 C -0.0208 -0.0454 -0.0309
9 C -0.0165 -0.0166 -0.0613
10 C -0.0031 -0.0486 -0.0059
11 C 0.0080 -0.0214 -0.0762
12 C -0.0153 -0.0333 -0.0498
13 C -0.0076 -0.0405 -0.0439
14 C 0.0031 -0.0111 -0.0421
15 C 0.0029 -0.0194 -0.0646
16 C -0.0231 -0.0019 -0.0622
17 C -0.0033 -0.0293 0.0341
18 C -0.0219 -0.0199 -0.0744
19 C -0.0134 0.0115 0.0143
20 C -0.0215 -0.0032 -0.0387
21 C -0.0238 0.0177 0.0195
22 C -0.0252 0.0408 0.1013
23 C -0.0421 0.0622 0.1511
24 H -0.0200 -0.0270 -0.0620
25 H 0.0405 -0.0555 -0.0062
26 H 0.0425 -0.0374 -0.0606
27 H 0.0394 -0.0451 -0.0394
28 H -0.0460 -0.0492 -0.0357
29 H -0.0202 -0.0484 -0.0208
30 H 0.0135 -0.0016 -0.0763
31 H 0.0153 -0.0314 -0.0936
32 H -0.0180 -0.0270 -0.0460
33 H -0.0148 -0.0336 -0.0494
34 H -0.0097 -0.0140 -0.0473
35 H -0.0289 -0.0642 -0.0538
36 H 0.0394 -0.0091 -0.0426
37 H -0.0081 -0.0317 -0.0655
38 H 0.0113 -0.0127 -0.0676
39 H -0.0346 0.0589 -0.0651
40 H -0.0704 -0.0354 -0.0622
41 H 0.0308 -0.0190 -0.0575
42 H -0.2599 -0.1415 0.1614
43 H 0.0965 -0.1245 -0.2226
44 H -0.0258 -0.0410 -0.1169
45 H 0.0312 -0.0643 0.0273
46 H -0.0204 -0.0076 -0.0467
47 H -0.0225 0.0459 0.1245
48 H 0.0691 0.3616 0.4350
49 H -0.0555 0.0872 0.2197
Vibrational Mode 2
----------------------------------------------------
Harmonic frequency: 50.43 cm**-1
Reduced mass: 5.0837 amu
Force constant: 0.0076 mdyne/A
IR intensity: 1.2128 km/mol
Normal mode:
X Y Z
1 O 0.0689 0.0035 0.0792
2 O -0.1582 -0.2381 0.0951
3 O -0.0115 -0.0384 0.1324
4 C 0.0025 0.0477 -0.0751
5 C 0.0142 0.0400 -0.0746
6 C 0.0659 0.0388 0.0107
7 C 0.0615 0.0291 -0.0519
8 C -0.0304 0.0856 -0.0260
9 C 0.0178 0.0169 -0.0801
10 C 0.0066 0.0374 0.0454
11 C -0.0225 0.0455 -0.1245
12 C 0.0195 0.0371 -0.0534
13 C 0.0746 0.0353 -0.0095
14 C 0.0031 0.0023 -0.0646
15 C -0.0293 0.0048 -0.1063
16 C 0.0272 0.0332 -0.0823
17 C -0.0789 0.0112 0.1196
18 C 0.0497 -0.0096 -0.0953
19 C -0.0144 -0.0190 0.0103
20 C 0.0527 -0.0328 -0.0501
21 C 0.0139 -0.0314 0.0211
22 C -0.0622 -0.0178 0.1252
23 C -0.0580 -0.0294 0.1950
24 H 0.0044 0.0487 -0.0757
25 H 0.1059 0.0399 0.0417
26 H 0.0951 0.0099 -0.0773
27 H 0.0614 0.0290 -0.0526
28 H -0.0670 0.1318 0.0022
29 H -0.0399 0.1006 -0.0694
30 H -0.0164 0.0865 -0.1254
31 H -0.0384 0.0417 -0.1623
32 H -0.0275 0.0161 -0.0272
33 H 0.0204 0.0458 -0.0944
34 H 0.1181 0.0228 -0.0329
35 H 0.0813 0.0457 0.0364
36 H 0.0497 0.0163 -0.0655
37 H -0.0458 -0.0181 -0.1078
38 H -0.0390 0.0018 -0.1174
39 H 0.0233 0.0630 -0.0914
40 H 0.0094 0.0205 -0.0832
41 H 0.0588 0.0283 -0.0717
42 H 0.0207 0.1036 0.0499
43 H -0.1864 0.0942 0.2741
44 H 0.0704 -0.0151 -0.1426
45 H 0.1185 -0.0247 0.1164
46 H 0.0816 -0.0542 -0.0573
47 H -0.0958 -0.0087 0.1535
48 H -0.0321 -0.3294 -0.0118
49 H -0.0847 -0.0321 0.2868
Vibrational Mode 3
----------------------------------------------------
Harmonic frequency: 80.30 cm**-1
Reduced mass: 3.6274 amu
Force constant: 0.0138 mdyne/A
IR intensity: 0.3441 km/mol
Normal mode:
X Y Z
1 O 0.0917 -0.0903 0.0475
2 O 0.0163 -0.0261 0.1117
3 O 0.0282 -0.0635 0.0215
4 C 0.0042 0.0459 -0.0139
5 C 0.0149 0.0351 -0.0346
6 C -0.0162 0.0664 -0.0569
7 C 0.0084 0.1001 -0.0088
8 C 0.0084 0.0171 -0.0232
9 C -0.0033 0.0317 0.0257
10 C -0.0075 -0.0383 -0.0178
11 C -0.0080 0.0288 0.0193
12 C -0.0176 0.0368 -0.1199
13 C -0.0622 0.0822 -0.1463
14 C -0.0086 0.0289 0.0190
15 C -0.0101 0.0289 0.0183
16 C -0.0452 0.0018 0.0346
17 C -0.0986 -0.1293 -0.0184
18 C 0.0344 0.0449 0.0935
19 C -0.0037 -0.0071 0.0064
20 C 0.0374 0.0299 0.1407
21 C 0.0168 -0.0076 0.0794
22 C -0.0057 -0.0683 -0.1121
23 C 0.0143 -0.1004 -0.0962
24 H 0.0626 0.0027 -0.0292
25 H -0.0092 0.1032 -0.0493
26 H 0.0016 0.1597 -0.0239
27 H 0.0339 0.0998 0.0434
28 H -0.0093 0.0373 -0.0107
29 H 0.0447 0.0153 -0.0447
30 H -0.0064 0.0023 0.0206
31 H -0.0198 0.0422 0.0393
32 H 0.0087 -0.0147 -0.1518
33 H -0.0401 0.0563 -0.1380
34 H -0.1078 0.1741 -0.1353
35 H -0.0905 0.0467 -0.2344
36 H -0.0148 0.0324 0.0188
37 H -0.0090 0.0260 0.0183
38 H -0.0101 0.0294 0.0171
39 H -0.0665 0.0926 0.0496
40 H -0.1302 -0.0613 0.0473
41 H 0.0365 -0.0424 0.0136
42 H -0.2353 -0.2531 0.0760
43 H -0.1111 -0.1530 -0.2103
44 H 0.0594 0.0749 0.1219
45 H 0.1378 -0.1234 0.0827
46 H 0.0578 0.0439 0.2002
47 H -0.0192 -0.0868 -0.1978
48 H 0.0146 -0.0120 0.2828
49 H 0.0206 -0.1474 -0.1580
Vibrational Mode 4
----------------------------------------------------
Harmonic frequency: 92.90 cm**-1
Reduced mass: 4.4744 amu
Force constant: 0.0227 mdyne/A
IR intensity: 0.5228 km/mol
Normal mode:
X Y Z
1 O 0.0616 -0.0843 -0.1626
2 O 0.0187 -0.0318 0.1283
3 O 0.0465 -0.0751 -0.1712
4 C 0.0045 0.0403 -0.0337
5 C 0.0065 0.0554 0.0399
6 C -0.0408 -0.0539 -0.0319
7 C -0.0258 -0.0310 -0.0488
8 C 0.0351 0.0905 -0.0832
9 C 0.0032 0.0528 0.0555
10 C 0.0020 -0.0174 -0.0698
11 C 0.0120 0.0434 -0.0114
12 C -0.0201 0.0270 0.0757
13 C -0.0494 -0.0433 0.0437
14 C -0.0194 0.0181 0.1241
15 C -0.0547 0.0412 0.1253
16 C -0.0072 0.1491 0.0389
17 C -0.0480 -0.0086 0.0208
18 C 0.0306 -0.0039 -0.0210
19 C -0.0128 -0.0035 0.0987
20 C 0.0313 -0.0627 -0.1219
21 C 0.0280 -0.0509 -0.0875
22 C -0.0269 0.0035 0.1453
23 C 0.0123 -0.0402 -0.0275
24 H 0.0210 0.0999 0.0428
25 H -0.0637 -0.0967 -0.0519
26 H -0.0416 -0.0471 -0.0279
27 H -0.0035 -0.0482 -0.0967
28 H 0.0500 0.1766 -0.0229
29 H 0.0636 0.1150 -0.1875
30 H 0.1123 0.0714 0.0003
31 H -0.0231 0.0392 -0.0826
32 H -0.0047 0.0605 0.0734
33 H -0.0350 0.0270 0.1273
34 H -0.0694 -0.0865 0.0632
35 H -0.0539 -0.0473 0.0619
36 H -0.0079 -0.0086 0.1232
37 H -0.1605 0.0882 0.1162
38 H -0.0308 0.0275 0.2156
39 H 0.0132 0.0385 0.0464
40 H 0.0917 0.2441 -0.0372
41 H -0.1340 0.2320 0.0935
42 H -0.1254 0.0165 0.0352
43 H -0.0588 -0.0045 0.0063
44 H 0.0400 0.0111 -0.0048
45 H 0.0638 -0.1874 -0.1379
46 H 0.0338 -0.0989 -0.2004
47 H -0.0592 0.0375 0.2831
48 H 0.0129 -0.0269 0.1359
49 H 0.0207 -0.0510 -0.0664
Vibrational Mode 5
----------------------------------------------------
Harmonic frequency: 134.98 cm**-1
Reduced mass: 2.7642 amu
Force constant: 0.0297 mdyne/A
IR intensity: 1.4207 km/mol
Normal mode:
X Y Z
1 O -0.0561 0.0708 -0.0249
2 O -0.0101 -0.0323 0.0900
3 O 0.0035 0.0266 0.0317
4 C -0.0079 -0.0468 -0.0397
5 C 0.0015 -0.0519 -0.0579
6 C 0.0327 -0.0117 -0.0174
7 C -0.0212 -0.0693 -0.0459
8 C -0.0225 -0.0245 -0.0272
9 C -0.0177 -0.0048 -0.0182
10 C 0.0009 0.0346 -0.0161
11 C 0.0211 -0.0387 0.0067
12 C -0.0163 -0.0604 -0.1086
13 C 0.0926 -0.0372 -0.0254
14 C 0.0090 -0.0137 0.0586
15 C -0.0157 0.0086 0.0912
16 C -0.0659 0.1001 -0.0332
17 C 0.0075 0.0980 0.0646
18 C -0.0099 -0.0126 -0.0191
19 C 0.0113 0.0049 0.0529
20 C -0.0089 0.0202 0.0880
21 C -0.0125 0.0261 0.1028
22 C 0.0536 -0.0100 -0.0737
23 C 0.0453 0.0028 -0.0799
24 H 0.0269 -0.0856 -0.0556
25 H 0.0403 0.0132 -0.0105
26 H -0.0223 -0.1541 -0.0186
27 H -0.0787 -0.0576 -0.1000
28 H -0.0156 -0.0539 -0.0463
29 H -0.0591 -0.0262 0.0060
30 H 0.0826 -0.0536 0.0154
31 H 0.0263 -0.0460 -0.0060
32 H -0.0871 -0.1556 -0.0833
33 H -0.0438 -0.0183 -0.2165
34 H 0.1914 -0.0906 -0.0751
35 H 0.1248 0.0030 0.0708
36 H 0.0280 -0.0611 0.0579
37 H -0.0831 0.0777 0.0856
38 H 0.0050 -0.0074 0.1817
39 H -0.0270 -0.1630 0.0176
40 H 0.1577 0.3008 -0.1669
41 H -0.3511 0.2545 0.0362
42 H 0.0291 0.2108 0.0114
43 H -0.0215 0.1327 0.1909
44 H -0.0039 -0.0309 -0.0711
45 H -0.0084 0.0738 0.0039
46 H 0.0023 0.0252 0.1149
47 H 0.0862 -0.0303 -0.1511
48 H 0.0172 -0.0586 -0.0177
49 H 0.0644 -0.0026 -0.1519
Vibrational Mode 6
----------------------------------------------------
Harmonic frequency: 147.48 cm**-1
Reduced mass: 3.6993 amu
Force constant: 0.0474 mdyne/A
IR intensity: 1.2818 km/mol
Normal mode:
X Y Z
1 O 0.0090 -0.0630 0.0205
2 O 0.0379 0.0387 -0.0880
3 O -0.0062 -0.0738 0.1197
4 C 0.0177 0.0171 0.0216
5 C -0.0300 0.0520 0.0073
6 C -0.0045 -0.0645 0.0469
7 C 0.0254 -0.0306 0.0232
8 C 0.0153 -0.0431 0.0383
9 C 0.0001 0.0854 -0.0695
10 C 0.0084 -0.0601 0.0334
11 C 0.0221 0.0278 0.0161
12 C -0.0440 0.0201 0.0879
13 C -0.0282 -0.0503 0.0929
14 C -0.0297 0.0642 -0.0526
15 C 0.0072 0.0760 0.0165
16 C 0.0270 0.1882 -0.0890
17 C 0.0487 -0.0676 -0.0299
18 C 0.0460 0.0431 -0.1043
19 C -0.0347 0.0123 -0.0660
20 C 0.0545 0.0257 0.0171
21 C 0.0078 0.0060 0.0597
22 C -0.0906 -0.0730 -0.1146
23 C -0.0680 -0.1202 0.0076
24 H -0.0958 0.0745 -0.0004
25 H -0.0034 -0.1070 0.0452
26 H 0.0159 -0.0320 0.0333
27 H 0.0562 -0.0472 -0.0025
28 H -0.0147 -0.0614 0.0229
29 H 0.0470 -0.0583 0.0648
30 H 0.0228 0.0200 0.0166
31 H 0.0328 0.0249 0.0249
32 H -0.0589 0.0595 0.1084
33 H -0.0453 0.0165 0.1147
34 H -0.0152 -0.0809 0.0908
35 H -0.0429 -0.0633 0.1301
36 H -0.0589 0.0371 -0.0516
37 H 0.0092 0.1434 0.0172
38 H 0.0289 0.0752 0.0652
39 H 0.0672 -0.0333 -0.0794
40 H 0.2230 0.3584 -0.1867
41 H -0.2015 0.3231 -0.0153
42 H 0.0608 -0.1170 -0.0135
43 H 0.0926 -0.0986 -0.0769
44 H 0.0746 0.0358 -0.1719
45 H -0.0933 -0.0204 -0.0517
46 H 0.0971 0.0075 0.0351
47 H -0.1413 -0.0928 -0.2206
48 H -0.0263 0.0824 -0.0751
49 H -0.0892 -0.1815 0.0305
Vibrational Mode 7
----------------------------------------------------
Harmonic frequency: 162.66 cm**-1
Reduced mass: 4.1264 amu
Force constant: 0.0643 mdyne/A
IR intensity: 0.1523 km/mol
Normal mode:
X Y Z
1 O 0.0388 -0.0276 -0.1683
2 O -0.0078 -0.0542 0.1177
3 O -0.0399 0.0663 0.1333
4 C -0.0154 0.0103 0.0763
5 C 0.0147 -0.0187 0.0469
6 C -0.0887 0.0451 -0.0347
7 C -0.0953 0.0523 0.0503
8 C 0.0439 0.0759 -0.0255
9 C 0.0424 -0.0445 -0.0450
10 C -0.0085 0.0313 -0.0748
11 C -0.0384 -0.0393 0.1756
12 C 0.0534 0.0142 0.0649
13 C -0.0389 0.0291 -0.0016
14 C 0.0155 -0.0572 -0.0705
15 C 0.0173 -0.0119 0.0667
16 C 0.1040 -0.0581 -0.0492
17 C -0.0592 0.0326 0.0050
18 C -0.0028 -0.0467 -0.0915
19 C 0.0325 -0.0333 -0.1247
20 C -0.0062 -0.0054 -0.0397
21 C -0.0127 0.0100 0.0100
22 C 0.0526 -0.0108 -0.1225
23 C 0.0020 0.0506 0.0494
24 H -0.0331 -0.0375 0.0401
25 H -0.1492 0.0703 -0.0804
26 H -0.1278 0.0783 0.0745
27 H -0.0945 0.0542 0.0611
28 H 0.1167 0.1337 0.0212
29 H 0.0448 0.1008 -0.1098
30 H -0.1031 -0.1700 0.1729
31 H -0.0366 0.0053 0.3096
32 H 0.1127 0.0934 0.0430
33 H 0.0916 -0.0299 0.1448
34 H -0.0901 0.0022 0.0343
35 H -0.0052 0.0609 -0.0473
36 H -0.0567 -0.1128 -0.0662
37 H 0.1061 0.0702 0.0748
38 H -0.0308 -0.0510 0.0845
39 H 0.1307 -0.1896 -0.0573
40 H 0.2119 0.0169 -0.0439
41 H -0.0125 -0.0139 -0.0483
42 H -0.1156 0.0786 0.0047
43 H -0.0913 0.0542 0.0270
44 H -0.0276 -0.0975 -0.1665
45 H 0.0559 -0.1386 -0.1323
46 H -0.0049 -0.0139 -0.0557
47 H 0.0944 -0.0364 -0.2201
48 H 0.0208 -0.0741 0.0987
49 H -0.0109 0.0866 0.1254
Vibrational Mode 8
----------------------------------------------------
Harmonic frequency: 190.61 cm**-1
Reduced mass: 1.9813 amu
Force constant: 0.0424 mdyne/A
IR intensity: 0.6579 km/mol
Normal mode:
X Y Z
1 O -0.0005 0.0089 0.0020
2 O 0.0149 -0.0129 0.0227
3 O 0.0103 -0.0303 -0.0389
4 C 0.0068 -0.0116 0.0263
5 C 0.0093 -0.0086 0.0017
6 C -0.0060 0.0154 -0.0108
7 C -0.0428 -0.0218 0.0089
8 C 0.0138 -0.0223 0.0100
9 C 0.0039 0.0165 0.0143
10 C 0.0049 0.0032 -0.0114
11 C 0.0062 -0.0198 0.0487
12 C -0.0220 -0.0213 -0.0631
13 C 0.0340 -0.0015 -0.0230
14 C -0.0097 0.0354 -0.0582
15 C 0.0402 0.0206 -0.0312
16 C 0.0088 -0.0400 0.0200
17 C -0.0028 -0.0035 -0.0070
18 C 0.0176 0.0662 0.1133
19 C -0.0078 0.0158 -0.0877
20 C 0.0172 0.0380 0.0115
21 C 0.0282 -0.0093 -0.1197
22 C -0.0693 0.0173 0.0545
23 C -0.0526 -0.0108 0.0744
24 H 0.0283 -0.0433 0.0030
25 H -0.0185 0.0462 -0.0189
26 H -0.0745 -0.0845 0.0566
27 H -0.0902 -0.0134 -0.0424
28 H 0.0254 -0.0495 -0.0072
29 H 0.0121 -0.0301 0.0371
30 H -0.0466 -0.0723 0.0442
31 H 0.0317 -0.0107 0.1179
32 H -0.0552 -0.1024 -0.0603
33 H -0.0585 0.0176 -0.1303
34 H 0.0943 -0.0296 -0.0538
35 H 0.0540 0.0226 0.0233
36 H -0.0596 0.0622 -0.0558
37 H 0.1109 0.0235 -0.0250
38 H 0.0433 0.0348 -0.0676
39 H 0.0822 -0.4789 0.0738
40 H 0.3546 0.2188 -0.0267
41 H -0.3942 0.1064 0.0090
42 H -0.0213 -0.0056 -0.0002
43 H -0.0137 0.0008 -0.0148
44 H 0.0231 0.1434 0.2820
45 H 0.0176 0.0114 0.0125
46 H 0.0004 0.0828 0.0880
47 H -0.1204 0.0398 0.1339
48 H 0.0231 -0.0176 0.0346
49 H -0.0771 -0.0198 0.1530
Vibrational Mode 9
----------------------------------------------------
Harmonic frequency: 194.86 cm**-1
Reduced mass: 2.7886 amu
Force constant: 0.0624 mdyne/A
IR intensity: 1.8599 km/mol
Normal mode:
X Y Z
1 O 0.0229 0.0386 -0.0924
2 O 0.0980 -0.0820 0.0506
3 O -0.0600 -0.0387 -0.0043
4 C 0.0435 -0.0187 0.0115
5 C -0.0071 0.0006 -0.0117
6 C 0.0507 -0.0093 -0.0005
7 C 0.0746 0.0267 0.0239
8 C 0.0677 -0.0392 -0.0030
9 C -0.0342 0.0304 0.0122
10 C 0.0828 0.0123 -0.0266
11 C 0.0188 -0.0479 0.0634
12 C -0.0266 -0.0084 -0.0386
13 C 0.0084 0.0067 -0.0110
14 C -0.0618 0.0274 -0.0065
15 C 0.0165 0.0049 0.0367
16 C -0.0389 0.0093 0.0189
17 C 0.1271 0.0854 0.0350
18 C -0.0405 0.0376 0.0169
19 C -0.0758 0.0209 -0.0334
20 C -0.0406 0.0229 -0.0095
21 C -0.0532 0.0059 -0.0243
22 C -0.1213 -0.0150 -0.0192
23 C -0.1032 -0.0468 -0.0014
24 H 0.0080 -0.0088 -0.0098
25 H 0.0317 -0.0232 -0.0162
26 H 0.0923 0.0925 -0.0140
27 H 0.1103 0.0247 0.0883
28 H 0.0905 -0.0820 -0.0299
29 H 0.0477 -0.0476 0.0405
30 H 0.0023 -0.1209 0.0648
31 H 0.0099 -0.0197 0.1311
32 H -0.0436 -0.0495 -0.0361
33 H -0.0361 0.0076 -0.0808
34 H 0.0178 0.0324 -0.0216
35 H -0.0082 -0.0104 0.0009
36 H -0.1020 0.0172 -0.0048
37 H 0.0418 0.0124 0.0387
38 H 0.0548 0.0324 0.0350
39 H -0.1008 0.3870 -0.0281
40 H -0.3423 -0.2292 0.0946
41 H 0.3258 -0.1420 -0.0016
42 H 0.1731 0.2189 -0.0348
43 H 0.0955 0.1246 0.1918
44 H -0.0398 0.0463 0.0374
45 H 0.0998 -0.0213 -0.0315
46 H -0.0368 0.0207 -0.0089
47 H -0.1611 -0.0118 -0.0216
48 H 0.1624 -0.1331 -0.0589
49 H -0.1139 -0.0741 0.0133
Vibrational Mode 10
----------------------------------------------------
Harmonic frequency: 207.20 cm**-1
Reduced mass: 3.6430 amu
Force constant: 0.0921 mdyne/A
IR intensity: 61.8983 km/mol
Normal mode:
X Y Z
1 O -0.2647 0.0691 -0.0766
2 O -0.0225 -0.0392 0.0420
3 O 0.1029 -0.0370 -0.0002
4 C -0.0091 0.0010 0.0321
5 C -0.0060 0.0060 0.0014
6 C -0.0770 -0.0529 -0.0048
7 C -0.0206 0.0094 0.0301
8 C -0.0369 -0.0792 0.0473
9 C 0.0323 0.0357 -0.0344
10 C -0.0751 -0.0251 -0.0088
11 C 0.0307 0.0283 0.0216
12 C -0.0501 -0.0206 0.0014
13 C -0.1099 -0.0353 -0.0365
14 C 0.0596 0.0490 -0.0325
15 C 0.0658 0.0432 -0.0466
16 C 0.0061 0.0192 -0.0297
17 C -0.0252 0.0433 0.0149
18 C 0.0825 0.0253 -0.0162
19 C 0.0611 0.0249 0.0026
20 C 0.0912 0.0060 -0.0001
21 C 0.1001 0.0037 -0.0072
22 C 0.0307 -0.0017 0.0300
23 C 0.0568 -0.0404 0.0245
24 H -0.0349 -0.0070 -0.0018
25 H -0.0699 -0.0399 0.0037
26 H -0.0388 0.0883 0.0244
27 H 0.0527 -0.0141 0.0647
28 H -0.0502 -0.1581 -0.0080
29 H -0.0084 -0.1114 0.1330
30 H -0.0151 0.0308 0.0155
31 H 0.0787 0.0078 0.0432
32 H -0.0196 -0.0269 -0.0210
33 H -0.0747 -0.0088 0.0325
34 H -0.1613 0.0134 -0.0140
35 H -0.1295 -0.0607 -0.0932
36 H 0.0812 0.0608 -0.0328
37 H 0.1172 0.0138 -0.0414
38 H 0.0526 0.0497 -0.0939
39 H -0.0362 0.2301 -0.0275
40 H -0.1774 -0.1205 0.0053
41 H 0.1917 -0.0730 -0.0613
42 H -0.0287 0.0853 -0.0036
43 H -0.0423 0.0572 0.0613
44 H 0.1052 0.0484 -0.0047
45 H 0.3200 -0.1959 0.3378
46 H 0.0905 0.0139 0.0149
47 H -0.0055 0.0053 0.0488
48 H 0.2104 -0.1799 0.2651
49 H 0.0466 -0.0708 0.0348
Vibrational Mode 11
----------------------------------------------------
Harmonic frequency: 209.80 cm**-1
Reduced mass: 1.2470 amu
Force constant: 0.0323 mdyne/A
IR intensity: 211.0478 km/mol
Normal mode:
X Y Z
1 O 0.0092 -0.0315 -0.0211
2 O -0.0272 0.0106 0.0491
3 O 0.0320 -0.0118 -0.0044
4 C -0.0009 -0.0003 0.0124
5 C 0.0003 -0.0028 -0.0095
6 C -0.0177 -0.0096 0.0062
7 C -0.0022 0.0100 0.0127
8 C -0.0094 -0.0226 0.0175
9 C 0.0083 0.0095 -0.0136
10 C -0.0183 -0.0189 0.0036
11 C 0.0073 0.0026 0.0175
12 C -0.0209 -0.0126 -0.0245
13 C -0.0281 -0.0055 -0.0235
14 C 0.0177 0.0137 -0.0120
15 C 0.0223 0.0116 -0.0120
16 C -0.0082 -0.0035 -0.0105
17 C -0.0146 0.0184 0.0439
18 C 0.0252 0.0105 0.0019
19 C 0.0178 0.0085 -0.0007
20 C 0.0278 0.0045 0.0037
21 C 0.0309 0.0008 -0.0067
22 C 0.0072 0.0013 0.0129
23 C 0.0160 -0.0118 0.0089
24 H -0.0030 -0.0160 -0.0104
25 H -0.0152 -0.0190 0.0039
26 H -0.0055 0.0286 0.0099
27 H 0.0023 0.0110 0.0217
28 H -0.0159 -0.0469 0.0001
29 H -0.0002 -0.0345 0.0501
30 H -0.0121 -0.0111 0.0153
31 H 0.0209 0.0028 0.0398
32 H -0.0174 -0.0343 -0.0329
33 H -0.0348 0.0000 -0.0347
34 H -0.0312 0.0169 -0.0255
35 H -0.0300 -0.0082 -0.0347
36 H 0.0241 0.0145 -0.0121
37 H 0.0436 0.0028 -0.0102
38 H 0.0182 0.0142 -0.0289
39 H -0.0281 0.0940 -0.0073
40 H -0.0938 -0.0690 0.0065
41 H 0.0772 -0.0474 -0.0284
42 H -0.0060 0.0932 0.0140
43 H 0.0057 0.0187 0.1101
44 H 0.0330 0.0227 0.0158
45 H -0.5900 0.2696 -0.4528
46 H 0.0270 0.0107 0.0157
47 H -0.0057 0.0047 0.0236
48 H -0.3196 0.1764 -0.3725
49 H 0.0126 -0.0210 0.0134
Vibrational Mode 12
----------------------------------------------------
Harmonic frequency: 267.88 cm**-1
Reduced mass: 2.5902 amu
Force constant: 0.1095 mdyne/A
IR intensity: 7.1405 km/mol
Normal mode:
X Y Z
1 O 0.0077 0.0307 -0.0084
2 O 0.0971 -0.0907 0.0556
3 O -0.0027 -0.0057 -0.0193
4 C 0.0025 0.0043 0.0608
5 C -0.0295 0.0097 0.0386
6 C -0.0223 0.0530 -0.0172
7 C -0.0346 0.0392 0.0503
8 C 0.0350 -0.0595 0.0317
9 C -0.0308 -0.0101 0.0161
10 C 0.0356 0.0027 -0.0305
11 C -0.0094 0.0224 -0.0398
12 C 0.0154 0.0508 0.0364
13 C -0.0541 0.0681 -0.0167
14 C 0.0044 0.0130 0.0076
15 C 0.0502 -0.0689 -0.1667
16 C -0.0495 0.0307 0.0051
17 C 0.0594 -0.0017 -0.0305
18 C -0.0313 -0.0511 -0.0550
19 C -0.0202 0.0112 0.1028
20 C -0.0310 -0.0339 -0.0100
21 C -0.0237 0.0098 0.0819
22 C -0.0016 -0.0006 0.0346
23 C 0.0129 -0.0138 -0.0547
24 H -0.0483 -0.0058 0.0358
25 H -0.0639 0.0642 -0.0493
26 H -0.0628 0.0344 0.0757
27 H -0.0655 0.0519 0.0560
28 H 0.0572 -0.1292 -0.0142
29 H 0.0386 -0.0835 0.1097
30 H -0.1048 0.0995 -0.0559
31 H 0.0029 0.0078 -0.0619
32 H 0.0719 0.1095 0.0114
33 H 0.0591 0.0083 0.0897
34 H -0.1108 0.1056 0.0104
35 H -0.0717 0.0445 -0.0809
36 H 0.0862 0.1091 0.0015
37 H 0.1615 -0.2568 -0.1559
38 H 0.0342 -0.0058 -0.3882
39 H -0.0075 -0.2521 0.0578
40 H 0.1749 0.2136 -0.0723
41 H -0.3304 0.1432 0.0151
42 H 0.0341 0.0353 -0.0362
43 H 0.0370 0.0135 -0.0030
44 H -0.0319 -0.1020 -0.1719
45 H -0.0964 0.1204 -0.0915
46 H -0.0327 -0.0666 -0.0862
47 H 0.0055 -0.0025 0.0283
48 H 0.0727 -0.0829 -0.0653
49 H 0.0325 -0.0266 -0.1347
Vibrational Mode 13
----------------------------------------------------
Harmonic frequency: 271.09 cm**-1
Reduced mass: 2.8853 amu
Force constant: 0.1249 mdyne/A
IR intensity: 7.8556 km/mol
Normal mode:
X Y Z
1 O -0.0520 0.0558 0.0946
2 O 0.0709 -0.0848 0.0596
3 O 0.0073 -0.0060 -0.0265
4 C -0.0056 0.0170 -0.0715
5 C -0.0266 0.0428 -0.0565
6 C 0.0059 0.0166 -0.0644
7 C -0.0082 0.0105 -0.0764
8 C -0.0221 -0.0983 -0.0428
9 C -0.0094 -0.0164 -0.0831
10 C 0.0057 -0.0220 -0.0498
11 C 0.0292 0.0172 0.0146
12 C 0.0936 0.0942 0.1224
13 C -0.0034 0.0218 0.0358
14 C 0.0005 -0.0238 -0.0144
15 C -0.0301 0.0469 0.1273
16 C -0.0525 -0.1113 -0.0668
17 C 0.0144 -0.0324 -0.0442
18 C 0.0107 0.0183 0.0057
19 C -0.0042 0.0111 -0.0069
20 C 0.0116 0.0325 0.0447
21 C 0.0059 0.0047 -0.0199
22 C -0.0160 0.0134 0.0241
23 C -0.0070 0.0007 0.0049
24 H -0.0920 0.1152 -0.0616
25 H -0.0037 -0.0158 -0.0729
26 H -0.0215 -0.0184 -0.0581
27 H -0.0311 0.0147 -0.1018
28 H -0.0670 -0.1731 -0.0992
29 H -0.0025 -0.1347 0.0674
30 H 0.1204 -0.0194 0.0278
31 H 0.0017 0.0278 0.0011
32 H 0.1651 0.3337 0.1314
33 H 0.2007 -0.0232 0.3128
34 H -0.0855 -0.0250 0.0929
35 H -0.0057 0.0178 0.0129
36 H 0.0044 -0.1350 -0.0105
37 H -0.1189 0.1906 0.1179
38 H -0.0290 -0.0088 0.2961
39 H -0.0526 -0.1682 -0.0211
40 H -0.0278 -0.1124 -0.0161
41 H -0.0959 -0.1319 -0.1283
42 H -0.0402 -0.0111 -0.0335
43 H 0.0119 -0.0306 -0.0467
44 H 0.0194 0.0451 0.0528
45 H -0.1543 0.2673 -0.0156
46 H 0.0147 0.0662 0.1250
47 H -0.0292 0.0213 0.0537
48 H 0.0051 -0.0506 -0.0739
49 H -0.0091 -0.0049 0.0075
Vibrational Mode 14
----------------------------------------------------
Harmonic frequency: 271.46 cm**-1
Reduced mass: 2.8588 amu
Force constant: 0.1241 mdyne/A
IR intensity: 4.3370 km/mol
Normal mode:
X Y Z
1 O 0.0999 -0.0272 -0.0110
2 O -0.0361 0.0418 -0.0287
3 O 0.0543 -0.0288 -0.0469
4 C -0.0109 -0.0169 0.0424
5 C -0.0213 -0.0251 -0.0406
6 C -0.0169 0.0238 0.0241
7 C -0.0831 -0.0481 0.0209
8 C 0.0146 0.0606 -0.0004
9 C -0.0191 0.0025 -0.0996
10 C 0.0071 0.0402 0.0044
11 C -0.0115 -0.0407 0.1016
12 C 0.0222 -0.0128 0.0321
13 C 0.0776 -0.0159 0.0760
14 C 0.0240 0.0192 -0.0409
15 C 0.0619 -0.0024 -0.0420
16 C -0.1989 -0.0360 -0.0918
17 C -0.0325 0.0012 -0.0067
18 C 0.0241 0.0083 -0.0512
19 C -0.0027 0.0532 0.0594
20 C 0.0312 0.0341 0.0414
21 C 0.0342 0.0317 0.0323
22 C -0.0292 0.0237 0.0597
23 C 0.0123 -0.0326 -0.0277
24 H -0.1011 -0.0538 -0.0504
25 H -0.0456 0.0463 0.0038
26 H -0.1098 -0.1723 0.0853
27 H -0.1652 -0.0358 -0.0830
28 H 0.0549 0.1129 0.0399
29 H -0.0173 0.0885 -0.0706
30 H -0.1057 -0.1512 0.0944
31 H 0.0257 -0.0153 0.2375
32 H -0.0273 0.0286 0.0770
33 H 0.0555 -0.0322 0.0151
34 H 0.1198 -0.1079 0.0661
35 H 0.1268 0.0419 0.1475
36 H 0.0909 -0.0022 -0.0418
37 H 0.1840 -0.0532 -0.0309
38 H 0.0467 0.0193 -0.1393
39 H -0.2657 0.1406 0.0379
40 H -0.3831 -0.1633 -0.1073
41 H -0.1044 -0.1302 -0.1842
42 H -0.0320 -0.0430 0.0103
43 H -0.0358 -0.0017 -0.0452
44 H 0.0426 0.0127 -0.0744
45 H 0.1596 -0.1364 0.0504
46 H 0.0305 0.0556 0.0876
47 H -0.0700 0.0349 0.0947
48 H 0.0286 0.0095 0.1182
49 H 0.0164 -0.0754 -0.0782
Vibrational Mode 15
----------------------------------------------------
Harmonic frequency: 294.51 cm**-1
Reduced mass: 3.1302 amu
Force constant: 0.1600 mdyne/A
IR intensity: 8.9861 km/mol
Normal mode:
X Y Z
1 O -0.0007 0.0763 0.0770
2 O 0.0164 -0.0356 0.0366
3 O 0.0107 0.0101 0.0853
4 C 0.0015 -0.0224 -0.0010
5 C 0.0123 -0.0059 0.0558
6 C 0.0005 0.0284 -0.0391
7 C -0.0822 -0.0734 -0.0388
8 C 0.0099 -0.0206 -0.0278
9 C -0.0025 0.0270 0.1091
10 C 0.0095 0.0354 -0.0475
11 C 0.0433 0.0054 -0.0130
12 C 0.0151 -0.0015 0.0203
13 C 0.0436 0.0056 0.0313
14 C 0.0046 0.0481 0.0239
15 C 0.0456 0.0193 -0.0151
16 C -0.1445 0.0869 0.1112
17 C -0.0495 -0.0342 -0.0655
18 C 0.0056 -0.0187 0.0281
19 C 0.0433 -0.0227 -0.1126
20 C 0.0040 -0.0713 -0.1438
21 C 0.0397 -0.0207 -0.0640
22 C 0.0358 -0.0329 -0.1078
23 C 0.0049 -0.0014 0.0584
24 H 0.0485 -0.0004 0.0601
25 H -0.0281 0.0328 -0.0603
26 H -0.0997 -0.2168 0.0259
27 H -0.2005 -0.0481 -0.1525
28 H 0.0292 -0.0510 -0.0471
29 H -0.0022 -0.0285 0.0084
30 H 0.0486 0.0315 -0.0138
31 H 0.0684 -0.0162 -0.0346
32 H -0.0127 -0.0463 0.0275
33 H 0.0081 0.0140 -0.0291
34 H 0.0485 -0.0507 0.0369
35 H 0.0608 0.0270 0.0612
36 H -0.1014 0.1320 0.0230
37 H 0.0571 -0.0169 -0.0137
38 H 0.0633 0.0441 -0.0517
39 H -0.2049 0.3182 0.1786
40 H -0.3483 -0.0348 0.0424
41 H -0.0093 0.0283 0.1021
42 H -0.1218 -0.1123 -0.0085
43 H -0.0934 -0.0257 -0.1675
44 H 0.0075 -0.0332 -0.0111
45 H -0.0939 0.2419 -0.0173
46 H -0.0288 -0.1155 -0.2909
47 H 0.0437 -0.0487 -0.1734
48 H -0.0189 -0.0126 0.0246
49 H -0.0198 0.0145 0.1593
Vibrational Mode 16
----------------------------------------------------
Harmonic frequency: 301.77 cm**-1
Reduced mass: 2.9888 amu
Force constant: 0.1604 mdyne/A
IR intensity: 3.0035 km/mol
Normal mode:
X Y Z
1 O 0.0280 -0.0551 -0.0173
2 O 0.0214 0.0112 -0.0322
3 O -0.0069 0.0284 0.0130
4 C 0.0079 0.0406 -0.0005
5 C -0.0191 0.0334 -0.0426
6 C 0.0055 -0.0212 0.0479
7 C 0.0554 0.0427 0.0224
8 C 0.0024 0.0296 0.0285
9 C -0.0186 -0.0308 -0.0330
10 C 0.0126 -0.0279 0.0573
11 C -0.0189 0.0297 -0.0144
12 C -0.0272 0.0329 0.0095
13 C -0.1025 0.0232 -0.0328
14 C 0.0073 -0.0545 -0.0161
15 C -0.0308 -0.0253 0.0158
16 C -0.2217 0.0320 -0.0368
17 C 0.0957 0.0490 0.0725
18 C 0.0104 -0.0105 0.0615
19 C 0.0531 -0.0927 -0.0909
20 C 0.0064 -0.0480 0.0087
21 C 0.0138 -0.0743 -0.0860
22 C 0.1039 -0.0237 -0.0281
23 C 0.0492 0.0552 0.0601
24 H -0.0529 0.0500 -0.0450
25 H 0.0307 -0.0415 0.0659
26 H 0.0425 0.0769 0.0156
27 H 0.1062 0.0256 0.0425
28 H -0.0269 0.0638 0.0495
29 H 0.0178 0.0371 -0.0082
30 H 0.0014 0.0537 -0.0129
31 H -0.0567 0.0385 -0.0504
32 H 0.0268 0.0901 -0.0127
33 H -0.0245 0.0167 0.0748
34 H -0.1815 0.1517 -0.0086
35 H -0.1657 -0.0511 -0.1350
36 H -0.0410 -0.0630 -0.0145
37 H -0.0499 0.0119 0.0142
38 H -0.0615 -0.0578 0.0503
39 H -0.2367 -0.0702 0.1133
40 H -0.1663 0.1219 -0.1947
41 H -0.4000 0.1134 -0.0223
42 H 0.1867 0.1595 -0.0038
43 H 0.1388 0.0460 0.2117
44 H 0.0258 0.0611 0.2003
45 H 0.1092 -0.1727 0.0589
46 H 0.0120 -0.0131 0.0940
47 H 0.1684 -0.0266 -0.0149
48 H 0.0600 -0.0175 -0.0689
49 H 0.0428 0.1259 0.1429
Vibrational Mode 17
----------------------------------------------------
Harmonic frequency: 319.21 cm**-1
Reduced mass: 2.5694 amu
Force constant: 0.1543 mdyne/A
IR intensity: 1.4968 km/mol
Normal mode:
X Y Z
1 O 0.0270 -0.0262 -0.0125
2 O 0.0164 0.0075 -0.0148
3 O 0.0255 -0.0048 0.0456
4 C 0.0073 0.0513 0.0231
5 C -0.0287 0.0545 -0.0092
6 C -0.0081 -0.0023 0.0281
7 C -0.0190 -0.0118 0.0150
8 C 0.0136 -0.0026 0.0302
9 C -0.0237 0.0228 -0.0008
10 C 0.0091 -0.0049 0.0220
11 C 0.0514 0.1037 -0.0162
12 C -0.1078 0.0152 -0.0737
13 C -0.0087 0.0020 0.0026
14 C 0.0084 0.0401 0.0188
15 C 0.0019 0.0770 0.0732
16 C -0.0373 -0.2135 0.0299
17 C 0.0382 0.0148 0.0205
18 C 0.0013 -0.0270 -0.0602
19 C 0.0025 0.0392 0.0340
20 C 0.0033 -0.0457 -0.1207
21 C 0.0223 0.0283 0.0467
22 C -0.0090 -0.0041 -0.0328
23 C 0.0060 -0.0295 -0.0155
24 H -0.0189 0.0262 -0.0090
25 H -0.0084 0.0035 0.0276
26 H -0.0614 -0.0721 0.0697
27 H -0.0374 -0.0206 -0.0670
28 H -0.0050 -0.0439 0.0009
29 H 0.0343 -0.0213 0.0755
30 H 0.1279 0.2093 -0.0115
31 H 0.0470 0.0633 -0.1407
32 H -0.1764 -0.1290 -0.0612
33 H -0.1750 0.0888 -0.1866
34 H 0.0778 -0.0110 -0.0465
35 H -0.0134 0.0022 0.0829
36 H 0.0297 -0.0092 0.0198
37 H -0.0582 0.1218 0.0652
38 H -0.0010 0.0502 0.1472
39 H -0.0137 -0.4059 0.0882
40 H 0.0721 -0.1816 0.1645
41 H -0.1668 -0.2664 -0.1323
42 H 0.0686 0.0472 -0.0035
43 H 0.0448 0.0171 0.0666
44 H -0.0003 -0.0975 -0.2243
45 H 0.0522 -0.0846 0.0163
46 H -0.0151 -0.1122 -0.2970
47 H -0.0268 -0.0149 -0.0866
48 H 0.0418 -0.0083 0.0025
49 H 0.0034 -0.0602 -0.0323
Vibrational Mode 18
----------------------------------------------------
Harmonic frequency: 333.42 cm**-1
Reduced mass: 2.4191 amu
Force constant: 0.1584 mdyne/A
IR intensity: 2.8198 km/mol
Normal mode:
X Y Z
1 O -0.0317 -0.0490 0.0357
2 O -0.0667 0.0517 -0.0167
3 O 0.0062 -0.0127 0.0166
4 C 0.0157 -0.0352 -0.0155
5 C 0.0456 -0.0399 0.0047
6 C 0.0273 -0.0335 -0.0256
7 C 0.0092 -0.0501 -0.0246
8 C -0.0109 0.0281 -0.0118
9 C 0.0087 0.0050 0.0476
10 C -0.0318 -0.0482 0.0305
11 C 0.0422 -0.0378 0.0158
12 C 0.1555 0.0240 0.0116
13 C -0.0349 -0.0069 -0.1383
14 C -0.0001 0.0395 0.0194
15 C 0.0508 0.0120 0.0073
16 C -0.0343 -0.0138 0.0555
17 C 0.0079 0.0435 0.0866
18 C -0.0086 -0.0102 -0.0165
19 C -0.0249 0.0708 0.0045
20 C -0.0095 0.0180 -0.0635
21 C 0.0059 0.0565 0.0182
22 C -0.0782 0.0147 -0.0248
23 C -0.0481 -0.0362 -0.0175
24 H 0.0721 -0.0610 0.0083
25 H 0.0685 0.0224 0.0105
26 H 0.0009 -0.0860 -0.0066
27 H -0.0229 -0.0427 -0.0492
28 H -0.0184 0.1370 0.0618
29 H 0.0032 0.0612 -0.1311
30 H 0.0422 -0.0774 0.0178
31 H 0.0657 -0.0384 0.0522
32 H 0.3317 0.2679 -0.0500
33 H 0.2572 -0.1023 0.2593
34 H -0.1827 0.1259 -0.0728
35 H -0.0883 -0.0749 -0.2991
36 H -0.0184 0.0618 0.0184
37 H 0.0696 -0.0059 0.0086
38 H 0.0789 0.0392 -0.0153
39 H -0.0230 -0.1269 0.1001
40 H 0.0427 0.0431 0.0455
41 H -0.1514 0.0146 0.0298
42 H 0.0742 0.1627 0.0158
43 H 0.0811 0.0256 0.2148
44 H -0.0281 -0.0696 -0.1217
45 H 0.0959 -0.1048 0.1255
46 H -0.0437 -0.0108 -0.1753
47 H -0.1344 0.0131 -0.0547
48 H -0.0945 0.0639 -0.0979
49 H -0.0571 -0.0857 -0.0282
Vibrational Mode 19
----------------------------------------------------
Harmonic frequency: 357.10 cm**-1
Reduced mass: 2.8192 amu
Force constant: 0.2118 mdyne/A
IR intensity: 0.5149 km/mol
Normal mode:
X Y Z
1 O 0.0243 0.0653 0.0204
2 O -0.0840 0.0434 -0.0018
3 O 0.0168 -0.0054 0.0142
4 C 0.0517 -0.0103 -0.1116
5 C -0.0306 0.0434 -0.0302
6 C 0.0476 -0.0269 -0.0091
7 C 0.1388 0.0729 -0.0903
8 C 0.0045 -0.0121 -0.0668
9 C -0.0361 -0.0137 0.0189
10 C 0.0226 0.0473 -0.0262
11 C 0.0602 -0.0939 0.0865
12 C -0.1135 -0.0040 0.0553
13 C -0.0966 0.0171 0.0754
14 C 0.0056 0.0029 0.0118
15 C 0.1111 -0.0520 -0.0010
16 C -0.0197 -0.0275 0.0209
17 C -0.0757 0.0222 -0.0000
18 C -0.0191 -0.0380 0.0042
19 C 0.0060 0.0118 0.0178
20 C -0.0200 -0.0329 -0.0291
21 C 0.0126 0.0056 0.0207
22 C 0.0030 -0.0033 -0.0004
23 C 0.0103 -0.0147 -0.0052
24 H -0.0150 0.1728 -0.0246
25 H 0.0798 -0.1811 0.0096
26 H 0.1787 0.1975 -0.1736
27 H 0.2399 0.0576 0.0421
28 H -0.0116 -0.0492 -0.0938
29 H -0.0363 -0.0184 -0.0137
30 H 0.0152 -0.3394 0.0918
31 H 0.0395 -0.0089 0.3037
32 H -0.1627 -0.0660 0.0737
33 H -0.1411 0.0310 -0.0107
34 H -0.1282 0.0797 0.0819
35 H -0.1572 -0.0505 0.0301
36 H -0.0005 0.0193 0.0116
37 H 0.2189 -0.1164 0.0085
38 H 0.1286 -0.0037 -0.1086
39 H 0.0178 -0.2066 0.0158
40 H 0.1294 0.0813 0.0092
41 H -0.1531 0.0349 0.0364
42 H -0.0943 -0.0480 0.0349
43 H -0.1289 0.0382 -0.0783
44 H -0.0256 -0.0580 -0.0343
45 H 0.0411 0.0998 0.0227
46 H -0.0463 -0.0448 -0.0936
47 H -0.0017 -0.0059 -0.0137
48 H -0.0647 0.0359 0.0661
49 H 0.0107 -0.0264 -0.0167
Vibrational Mode 20
----------------------------------------------------
Harmonic frequency: 367.61 cm**-1
Reduced mass: 3.3827 amu
Force constant: 0.2693 mdyne/A
IR intensity: 4.3512 km/mol
Normal mode:
X Y Z
1 O -0.1199 -0.0084 -0.1745
2 O -0.0760 0.0193 0.0034
3 O -0.0520 0.0054 0.0019
4 C 0.0618 0.0519 0.0300
5 C 0.0508 0.0269 -0.0522
6 C 0.0547 0.0058 0.0251
7 C 0.1227 0.0802 0.0540
8 C 0.0243 -0.0426 0.0927
9 C 0.0030 -0.0079 0.0068
10 C -0.0156 -0.0065 0.0354
11 C 0.0434 0.0498 -0.0106
12 C 0.1216 0.0448 -0.0059
13 C 0.1691 -0.0141 0.0228
14 C -0.0039 -0.0129 0.0203
15 C 0.0280 -0.0245 0.0108
16 C -0.1040 -0.0154 0.0135
17 C -0.0975 -0.0875 -0.0078
18 C -0.0165 0.0028 0.0223
19 C -0.0219 -0.0142 0.0035
20 C -0.0239 -0.0135 -0.0308
21 C -0.0433 -0.0102 -0.0041
22 C -0.0193 -0.0129 -0.0164
23 C -0.0334 0.0066 -0.0103
24 H 0.0455 0.0030 -0.0516
25 H 0.0944 0.0455 0.0568
26 H 0.0974 0.1982 0.0316
27 H 0.2307 0.0513 0.1306
28 H -0.0066 -0.1485 0.0171
29 H 0.0509 -0.0856 0.2141
30 H 0.0658 0.1193 -0.0112
31 H 0.0064 0.0446 -0.0864
32 H 0.0869 0.1089 0.0329
33 H 0.1455 0.0180 0.0323
34 H 0.2327 -0.1278 0.0044
35 H 0.2293 0.0569 0.1203
36 H -0.0141 -0.0121 0.0196
37 H 0.0341 -0.0615 0.0105
38 H 0.0229 -0.0139 -0.0292
39 H -0.1046 -0.0980 0.0885
40 H -0.0459 0.0384 -0.0334
41 H -0.1971 0.0117 -0.0043
42 H -0.1523 -0.2808 0.0899
43 H -0.1410 -0.0941 -0.2077
44 H -0.0247 -0.0056 0.0201
45 H -0.1668 -0.1081 -0.1808
46 H -0.0148 -0.0326 -0.0595
47 H -0.0081 -0.0164 -0.0302
48 H -0.0765 0.0309 0.1509
49 H -0.0292 0.0225 -0.0120
Vibrational Mode 21
----------------------------------------------------
Harmonic frequency: 377.12 cm**-1
Reduced mass: 2.3421 amu
Force constant: 0.1963 mdyne/A
IR intensity: 3.4504 km/mol
Normal mode:
X Y Z
1 O -0.0342 0.1178 -0.0148
2 O 0.0129 -0.0389 0.0088
3 O -0.0006 -0.0100 -0.0140
4 C 0.0453 -0.0323 0.0483
5 C 0.0207 -0.0403 0.0303
6 C 0.0395 -0.0299 0.0730
7 C 0.0308 -0.0622 0.0441
8 C 0.0580 0.1370 0.0314
9 C 0.0196 -0.0293 -0.0356
10 C 0.0451 0.0695 0.0413
11 C 0.0570 -0.0014 -0.0527
12 C -0.0221 -0.0583 0.0005
13 C -0.0911 0.0058 -0.0345
14 C 0.0096 -0.0369 -0.0073
15 C 0.0121 -0.0222 0.0473
16 C -0.0265 -0.0210 -0.0433
17 C -0.0240 -0.0507 -0.0410
18 C -0.0008 -0.0161 -0.0325
19 C -0.0089 0.0251 -0.0196
20 C -0.0010 0.0431 0.0316
21 C -0.0006 0.0236 -0.0131
22 C -0.0415 0.0111 0.0024
23 C -0.0286 -0.0117 0.0000
24 H -0.0102 -0.0609 0.0244
25 H 0.0543 -0.0574 0.0818
26 H 0.0133 -0.1103 0.0754
27 H -0.0122 -0.0540 -0.0014
28 H 0.1074 0.2860 0.1377
29 H 0.0174 0.2030 -0.1616
30 H 0.1310 0.1487 -0.0497
31 H 0.0464 -0.0481 -0.2096
32 H 0.0072 -0.0904 -0.0272
33 H -0.0437 -0.0402 -0.0128
34 H -0.2002 0.2355 -0.0109
35 H -0.1936 -0.1153 -0.2367
36 H 0.0038 -0.0871 -0.0062
37 H -0.0645 0.0391 0.0396
38 H 0.0261 -0.0401 0.1326
39 H -0.0367 -0.0435 0.0131
40 H -0.0276 -0.0076 -0.0851
41 H -0.0819 -0.0048 -0.0532
42 H -0.0649 -0.2753 0.0651
43 H -0.1326 -0.0308 -0.2711
44 H -0.0248 -0.0264 -0.0169
45 H -0.1777 0.2265 -0.1273
46 H -0.0133 0.0737 0.0840
47 H -0.0742 0.0170 0.0146
48 H 0.0282 -0.0434 0.0724
49 H -0.0342 -0.0291 0.0048
Vibrational Mode 22
----------------------------------------------------
Harmonic frequency: 381.44 cm**-1
Reduced mass: 2.7143 amu
Force constant: 0.2327 mdyne/A
IR intensity: 3.4784 km/mol
Normal mode:
X Y Z
1 O -0.1342 0.0102 0.0371
2 O 0.1000 -0.0599 -0.0001
3 O -0.0232 0.0221 0.0101
4 C 0.0024 0.0694 -0.0341
5 C 0.0407 0.0541 -0.0388
6 C 0.0082 -0.0512 0.0226
7 C 0.0273 -0.0052 -0.0322
8 C -0.0036 0.1390 -0.0304
9 C 0.0188 0.0507 0.0100
10 C -0.0141 -0.0611 0.0400
11 C -0.1096 -0.0357 0.1004
12 C -0.0069 0.0155 -0.0421
13 C 0.0363 -0.0592 -0.0131
14 C -0.0222 0.0195 0.0040
15 C -0.0412 0.0026 -0.0405
16 C -0.0150 -0.0264 0.0244
17 C 0.0649 -0.0794 -0.0193
18 C 0.0370 0.0441 0.0031
19 C -0.0140 -0.0203 0.0473
20 C 0.0350 -0.0226 -0.0532
21 C -0.0236 -0.0148 0.0393
22 C 0.0304 -0.0002 0.0034
23 C 0.0190 0.0235 -0.0241
24 H 0.0704 0.0480 -0.0344
25 H 0.0467 -0.0400 0.0507
26 H 0.0283 -0.0358 -0.0253
27 H 0.0577 -0.0274 -0.0862
28 H -0.0532 0.3676 0.1204
29 H 0.0665 0.2013 -0.2897
30 H -0.2223 -0.3020 0.0982
31 H -0.1330 0.0690 0.3690
32 H -0.0247 0.0091 -0.0299
33 H -0.0475 0.0420 -0.0289
34 H 0.0943 -0.0988 -0.0402
35 H 0.0446 -0.0449 0.0613
36 H 0.0014 0.0438 0.0034
37 H 0.0666 -0.0656 -0.0305
38 H -0.0576 0.0235 -0.1408
39 H -0.0144 -0.0769 0.0626
40 H 0.0062 -0.0248 0.0629
41 H -0.0539 -0.0545 -0.0422
42 H 0.0560 -0.1177 -0.0001
43 H 0.0834 -0.0960 -0.0569
44 H 0.0468 0.0341 -0.0362
45 H -0.1730 0.1347 -0.0165
46 H 0.0829 -0.0830 -0.1200
47 H 0.0726 -0.0084 -0.0153
48 H 0.0013 -0.0038 -0.1299
49 H 0.0325 0.0412 -0.0565
Vibrational Mode 23
----------------------------------------------------
Harmonic frequency: 416.84 cm**-1
Reduced mass: 3.9118 amu
Force constant: 0.4005 mdyne/A
IR intensity: 1.4658 km/mol
Normal mode:
X Y Z
1 O -0.0139 -0.0278 -0.0183
2 O 0.0670 -0.0347 -0.0043
3 O 0.1617 -0.0046 0.0287
4 C -0.0719 -0.0265 -0.0377
5 C -0.0134 -0.0646 -0.0053
6 C -0.0293 0.0331 0.0117
7 C 0.0470 0.1249 0.0217
8 C -0.0671 0.0213 -0.0281
9 C -0.0246 -0.0811 0.0541
10 C -0.0178 -0.0152 0.0093
11 C -0.1070 -0.0383 -0.0533
12 C 0.0047 -0.0653 0.0196
13 C 0.0405 0.0090 0.0601
14 C -0.0134 -0.0376 0.0438
15 C -0.1066 -0.0491 0.0050
16 C -0.0564 0.0012 0.0531
17 C 0.0406 -0.0206 -0.0249
18 C 0.0226 -0.1285 -0.0079
19 C 0.0245 0.1635 -0.0760
20 C 0.0266 0.0760 -0.0046
21 C 0.1509 0.1320 -0.0390
22 C -0.0738 0.0884 -0.0510
23 C 0.0030 -0.0423 0.0346
24 H 0.0200 -0.0273 0.0005
25 H -0.0289 0.0100 0.0089
26 H 0.1106 0.3551 -0.1168
27 H 0.1896 0.1145 0.2630
28 H -0.0665 0.0938 0.0206
29 H -0.0859 0.0502 -0.1089
30 H -0.0982 -0.0254 -0.0518
31 H -0.1168 -0.0362 -0.0647
32 H -0.0393 -0.0917 0.0432
33 H 0.0219 -0.0605 -0.0528
34 H 0.0851 -0.0750 0.0474
35 H 0.1149 0.0903 0.1048
36 H -0.0610 -0.0330 0.0424
37 H -0.1951 -0.0358 -0.0023
38 H -0.1332 -0.0835 0.0559
39 H -0.0456 -0.0458 0.0533
40 H 0.0012 0.0686 -0.0303
41 H -0.1028 0.0674 0.1251
42 H 0.0395 -0.0071 -0.0305
43 H 0.0590 -0.0275 -0.0060
44 H -0.0326 -0.2022 -0.0982
45 H -0.0789 -0.0287 -0.0577
46 H -0.1483 0.1524 -0.0783
47 H -0.2144 0.0951 -0.0693
48 H 0.0736 -0.0396 -0.0076
49 H -0.0430 -0.1683 0.0896
Vibrational Mode 24
----------------------------------------------------
Harmonic frequency: 434.14 cm**-1
Reduced mass: 2.4453 amu
Force constant: 0.2715 mdyne/A
IR intensity: 5.8704 km/mol
Normal mode:
X Y Z
1 O 0.0702 0.0261 -0.0613
2 O -0.0185 -0.0060 -0.0193
3 O 0.0041 0.0153 0.0192
4 C -0.0357 -0.0696 -0.0184
5 C 0.0011 -0.0605 -0.0003
6 C 0.0465 -0.0014 0.0489
7 C 0.0323 -0.0260 0.0118
8 C -0.0428 -0.0592 0.0282
9 C 0.0079 0.0663 -0.0005
10 C 0.0312 0.0638 0.0284
11 C -0.1017 -0.0931 -0.0332
12 C 0.0415 -0.0485 0.0316
13 C -0.0291 0.0196 -0.0085
14 C -0.0204 0.0694 -0.0275
15 C -0.1163 0.1045 -0.0410
16 C -0.0135 -0.0276 0.0186
17 C -0.0043 0.0108 -0.0025
18 C 0.0422 0.0851 0.0246
19 C -0.0058 -0.0105 0.0435
20 C 0.0480 -0.0254 -0.0668
21 C 0.0013 -0.0120 0.0340
22 C 0.0343 0.0012 0.0042
23 C 0.0316 0.0121 -0.0170
24 H -0.0084 -0.0526 -0.0006
25 H 0.0592 -0.0301 0.0567
26 H 0.0708 -0.0121 -0.0306
27 H 0.0093 -0.0055 0.0727
28 H -0.0326 -0.1914 -0.0602
29 H -0.1289 -0.0879 0.1870
30 H -0.1749 -0.2102 -0.0348
31 H -0.0348 -0.0766 0.1228
32 H 0.0826 0.0253 0.0207
33 H 0.1006 -0.1000 0.0753
34 H -0.1269 0.1731 0.0214
35 H -0.0856 -0.0502 -0.1682
36 H 0.0231 0.0966 -0.0270
37 H -0.2055 0.2115 -0.0466
38 H -0.0526 0.0924 0.1202
39 H -0.0043 -0.1147 0.0520
40 H 0.0345 -0.0118 0.0718
41 H -0.0744 -0.0422 -0.0417
42 H -0.0007 -0.0973 0.0370
43 H -0.0713 0.0291 -0.0986
44 H 0.0809 0.1010 -0.0035
45 H -0.1778 0.0303 -0.2114
46 H 0.0994 -0.0871 -0.1339
47 H 0.0707 -0.0071 -0.0159
48 H 0.2395 -0.1497 0.3641
49 H 0.0405 0.0173 -0.0437
Vibrational Mode 25
----------------------------------------------------
Harmonic frequency: 447.30 cm**-1
Reduced mass: 2.5916 amu
Force constant: 0.3055 mdyne/A
IR intensity: 4.5664 km/mol
Normal mode:
X Y Z
1 O -0.0129 0.0083 0.0330
2 O 0.0292 -0.0116 0.0173
3 O -0.0087 -0.0239 -0.0049
4 C -0.0311 -0.0606 0.0127
5 C -0.0143 -0.0989 -0.0071
6 C -0.0446 0.0094 -0.0225
7 C 0.0303 0.0960 0.0543
8 C -0.0045 0.0592 -0.0426
9 C -0.0359 -0.0018 -0.0104
10 C -0.0125 -0.0119 -0.0286
11 C 0.0560 0.0181 -0.0243
12 C 0.0289 -0.0984 0.0256
13 C 0.0231 -0.0286 0.0267
14 C 0.0504 0.0930 -0.0828
15 C 0.0405 0.1694 -0.0238
16 C -0.0252 -0.0072 -0.0118
17 C -0.0018 -0.0018 -0.0281
18 C -0.0927 0.0187 0.0281
19 C 0.0257 -0.0426 0.0231
20 C -0.0898 -0.0713 -0.0016
21 C -0.0207 -0.0354 0.0245
22 C 0.0317 -0.0445 0.0350
23 C 0.0191 -0.0218 -0.0011
24 H -0.0182 -0.1025 -0.0074
25 H -0.0735 0.0107 -0.0450
26 H 0.0528 0.3579 -0.0543
27 H 0.1834 0.0822 0.2960
28 H 0.0574 0.2323 0.0803
29 H -0.0161 0.1260 -0.2548
30 H 0.0534 0.0722 -0.0266
31 H 0.1695 -0.0524 -0.0446
32 H 0.0253 -0.0481 0.0406
33 H 0.0670 -0.1257 0.0272
34 H 0.0311 -0.0938 0.0331
35 H 0.0941 0.0466 0.0336
36 H 0.0927 0.1359 -0.0810
37 H -0.0019 0.3326 -0.0256
38 H 0.0715 0.1339 0.1428
39 H -0.0102 -0.0489 -0.0343
40 H 0.0265 0.0324 -0.0221
41 H -0.0440 0.0290 0.0295
42 H -0.0188 0.0337 -0.0342
43 H 0.0213 -0.0082 -0.0031
44 H -0.0551 0.0419 0.0249
45 H 0.1121 0.0143 0.1069
46 H -0.0842 -0.0836 -0.0274
47 H 0.0547 -0.0438 0.0454
48 H -0.1393 0.0814 -0.2480
49 H 0.0307 0.0041 -0.0193
Vibrational Mode 26
----------------------------------------------------
Harmonic frequency: 454.30 cm**-1
Reduced mass: 2.5593 amu
Force constant: 0.3112 mdyne/A
IR intensity: 9.2887 km/mol
Normal mode:
X Y Z
1 O 0.0224 0.0277 0.0579
2 O -0.0173 0.0159 0.0358
3 O -0.0563 0.0269 0.0135
4 C 0.0485 -0.0083 0.0400
5 C 0.0792 -0.0186 0.0190
6 C -0.0544 -0.0233 -0.0503
7 C 0.0133 0.0537 0.0409
8 C 0.0633 -0.0247 0.0016
9 C 0.0842 0.0446 -0.0216
10 C 0.0033 0.0007 -0.0711
11 C -0.0319 -0.0351 -0.0488
12 C 0.0009 -0.0931 0.0364
13 C -0.0245 -0.0573 0.0232
14 C -0.0242 -0.0414 0.0347
15 C -0.0619 -0.0687 0.0009
16 C -0.0482 -0.0259 -0.0105
17 C -0.0417 0.0301 -0.0275
18 C 0.1104 0.0899 -0.0032
19 C -0.0423 0.0059 0.0358
20 C 0.1085 0.0495 -0.0708
21 C -0.0380 0.0070 0.0098
22 C -0.0231 0.0139 -0.0205
23 C -0.0301 0.0259 -0.0334
24 H 0.0461 -0.0129 0.0148
25 H -0.1059 -0.0398 -0.0893
26 H -0.0515 0.2159 0.0453
27 H 0.1243 0.0282 0.1347
28 H 0.1061 -0.0839 -0.0348
29 H 0.0860 -0.0452 0.0514
30 H -0.0174 0.0645 -0.0509
31 H -0.0697 -0.0429 -0.1371
32 H -0.0266 -0.1739 0.0338
33 H -0.0616 -0.0371 -0.0137
34 H -0.0571 -0.0869 0.0471
35 H 0.0140 -0.0184 -0.0075
36 H -0.0096 -0.0761 0.0338
37 H -0.1214 -0.1115 -0.0046
38 H -0.0347 -0.0489 -0.0017
39 H -0.0716 -0.0935 0.1368
40 H -0.0472 -0.0194 -0.0292
41 H -0.1545 -0.0559 -0.1199
42 H -0.0706 0.0943 -0.0388
43 H -0.0268 0.0315 0.0120
44 H 0.0886 0.0903 0.0321
45 H 0.3199 -0.0054 0.2457
46 H 0.1898 -0.0056 -0.0733
47 H -0.0049 0.0058 -0.0509
48 H -0.2958 0.1702 -0.4011
49 H -0.0214 0.0324 -0.0592
Vibrational Mode 27
----------------------------------------------------
Harmonic frequency: 475.53 cm**-1
Reduced mass: 1.4137 amu
Force constant: 0.1883 mdyne/A
IR intensity: 16.0138 km/mol
Normal mode:
X Y Z
1 O 0.0106 -0.0252 0.0671
2 O -0.0157 0.0115 -0.0319
3 O -0.0296 0.0011 0.0026
4 C 0.0122 0.0179 0.0068
5 C 0.0349 -0.0076 -0.0107
6 C -0.0587 -0.0182 -0.0324
7 C -0.0082 0.0482 0.0046
8 C 0.0062 0.0195 -0.0148
9 C 0.0269 -0.0037 -0.0064
10 C -0.0289 -0.0223 -0.0316
11 C 0.0306 0.0290 0.0029
12 C 0.0079 -0.0434 0.0039
13 C -0.0047 -0.0524 -0.0034
14 C 0.0136 -0.0163 0.0105
15 C 0.0321 -0.0236 0.0097
16 C -0.0095 0.0013 -0.0078
17 C 0.0093 0.0472 0.0036
18 C 0.0108 0.0185 0.0074
19 C -0.0083 -0.0025 0.0123
20 C 0.0080 0.0100 -0.0187
21 C -0.0220 -0.0005 -0.0012
22 C -0.0133 -0.0075 -0.0041
23 C -0.0194 0.0005 -0.0106
24 H 0.0341 -0.0071 -0.0105
25 H -0.0690 -0.0285 -0.0414
26 H -0.0365 0.1509 -0.0033
27 H 0.0714 0.0280 0.0568
28 H 0.0066 0.0643 0.0159
29 H 0.0422 0.0273 -0.0668
30 H 0.0582 0.0887 0.0029
31 H 0.0185 0.0130 -0.0638
32 H 0.0107 -0.0453 0.0012
33 H -0.0216 -0.0258 0.0213
34 H 0.0111 -0.1208 -0.0002
35 H 0.0521 0.0106 0.0317
36 H 0.0153 -0.0283 0.0102
37 H 0.0550 -0.0612 0.0109
38 H 0.0175 -0.0166 -0.0399
39 H -0.0168 -0.0019 0.0246
40 H -0.0132 0.0064 -0.0308
41 H -0.0294 0.0025 -0.0173
42 H 0.0298 0.1571 -0.0553
43 H 0.0324 0.0518 0.1278
44 H -0.0039 0.0205 0.0375
45 H -0.3607 0.2165 -0.2134
46 H 0.0259 0.0010 -0.0117
47 H -0.0133 -0.0091 -0.0136
48 H 0.4165 -0.2321 0.5989
49 H -0.0151 0.0084 -0.0192
Vibrational Mode 28
----------------------------------------------------
Harmonic frequency: 506.43 cm**-1
Reduced mass: 3.4981 amu
Force constant: 0.5286 mdyne/A
IR intensity: 0.4331 km/mol
Normal mode:
X Y Z
1 O 0.0490 0.0521 0.0467
2 O -0.0388 0.0168 0.0070
3 O 0.0170 -0.0117 -0.0745
4 C 0.0284 0.1073 0.0390
5 C 0.0657 0.0436 0.0349
6 C 0.0181 -0.1177 -0.0388
7 C 0.0600 -0.0353 0.0247
8 C 0.0667 -0.0249 0.0092
9 C 0.0265 -0.0250 0.0621
10 C 0.0303 0.0153 -0.0657
11 C -0.0957 0.0901 0.0460
12 C 0.0222 -0.0060 -0.0224
13 C 0.0001 -0.1404 -0.0627
14 C -0.0049 0.0218 -0.0733
15 C -0.0798 0.0999 -0.0444
16 C -0.0375 -0.0059 0.0817
17 C -0.0372 0.0362 -0.0191
18 C -0.0312 -0.1302 -0.1221
19 C 0.0169 -0.0314 -0.0937
20 C -0.0344 0.0155 0.1413
21 C -0.0022 -0.0010 0.0285
22 C -0.0005 0.0119 0.0427
23 C 0.0007 0.0165 0.0270
24 H 0.1030 0.0078 0.0384
25 H -0.0123 -0.0991 -0.0602
26 H -0.0060 0.0423 0.0565
27 H 0.1767 -0.0857 -0.0123
28 H 0.0707 -0.1943 -0.1042
29 H 0.1336 -0.0987 0.2010
30 H -0.1965 -0.0465 0.0394
31 H -0.1066 0.1487 0.2034
32 H 0.0483 -0.0394 -0.0489
33 H -0.0597 0.0381 0.0438
34 H -0.0236 -0.1091 -0.0533
35 H -0.0401 -0.1807 -0.0617
36 H -0.0553 0.1020 -0.0723
37 H -0.0615 0.2114 -0.0408
38 H -0.1420 0.0287 0.0375
39 H -0.0565 -0.0039 0.1533
40 H -0.0566 0.0041 0.0119
41 H -0.0875 0.0114 0.0761
42 H -0.0591 0.0323 -0.0100
43 H -0.0689 0.0506 -0.0346
44 H -0.0636 -0.2500 -0.3465
45 H 0.0648 0.1570 0.0327
46 H -0.0632 0.0221 0.1140
47 H -0.0048 0.0300 0.1220
48 H -0.0055 -0.0022 0.0490
49 H -0.0054 0.0275 0.0581
Vibrational Mode 29
----------------------------------------------------
Harmonic frequency: 523.94 cm**-1
Reduced mass: 3.4461 amu
Force constant: 0.5574 mdyne/A
IR intensity: 2.6240 km/mol
Normal mode:
X Y Z
1 O 0.0708 0.0693 0.0253
2 O -0.0147 -0.0003 0.0117
3 O 0.0700 -0.0015 0.0826
4 C -0.0314 0.0375 0.0683
5 C -0.0755 0.0177 -0.0809
6 C 0.0101 -0.1139 -0.0106
7 C 0.0307 -0.0629 0.0866
8 C 0.0245 0.0075 0.0086
9 C -0.0849 -0.0267 -0.0544
10 C 0.0308 0.0434 -0.0454
11 C -0.0617 0.0683 0.0448
12 C 0.0074 0.0576 -0.0458
13 C 0.0071 -0.1468 -0.0768
14 C 0.0007 -0.0204 0.0440
15 C -0.0348 -0.0421 0.0049
16 C 0.0384 0.0190 -0.0891
17 C -0.0318 0.0311 -0.0385
18 C -0.0669 0.0380 0.1466
19 C 0.0101 0.0644 0.0763
20 C -0.0664 -0.0482 -0.1096
21 C 0.0618 0.0288 -0.0354
22 C 0.0117 0.0054 -0.0367
23 C 0.0388 -0.0444 -0.0041
24 H -0.0951 -0.0743 -0.0822
25 H -0.0370 -0.0466 -0.0431
26 H 0.0135 0.0551 0.0652
27 H 0.1521 -0.1021 0.1124
28 H 0.0867 -0.0335 -0.0121
29 H 0.0174 -0.0024 0.0448
30 H -0.0772 0.1081 0.0395
31 H -0.0911 0.0706 0.0050
32 H 0.1005 0.3131 -0.0426
33 H 0.0630 -0.0407 0.2202
34 H 0.0445 -0.1460 -0.0962
35 H -0.0375 -0.1869 0.0028
36 H 0.0672 -0.0761 0.0431
37 H 0.0237 -0.1483 0.0089
38 H -0.1010 -0.0449 -0.1222
39 H 0.0782 0.0345 -0.2552
40 H 0.0905 0.0398 -0.0400
41 H 0.1343 0.0510 0.0169
42 H -0.0597 -0.0281 -0.0035
43 H -0.0781 0.0448 -0.1053
44 H -0.0486 0.1409 0.3560
45 H 0.1004 0.1212 0.0324
46 H -0.1555 0.0073 -0.1139
47 H -0.0277 -0.0091 -0.1116
48 H -0.0355 0.0102 -0.0439
49 H 0.0307 -0.0975 -0.0204
Vibrational Mode 30
----------------------------------------------------
Harmonic frequency: 527.58 cm**-1
Reduced mass: 2.8355 amu
Force constant: 0.4650 mdyne/A
IR intensity: 3.9740 km/mol
Normal mode:
X Y Z
1 O -0.0105 0.0085 0.0128
2 O -0.0255 0.0147 -0.0025
3 O 0.0285 -0.0063 -0.0441
4 C -0.0151 -0.0970 0.0526
5 C -0.1071 -0.0023 0.0095
6 C 0.0314 -0.0179 -0.0478
7 C 0.0319 -0.0187 0.0815
8 C 0.0246 0.0021 -0.0306
9 C -0.0923 0.1271 0.0004
10 C 0.0120 -0.0246 -0.0383
11 C 0.0142 -0.0766 -0.0311
12 C -0.0249 0.0835 -0.0310
13 C 0.0293 -0.0062 -0.0226
14 C -0.0903 0.0713 0.1170
15 C -0.0097 -0.0368 0.0166
16 C -0.0087 -0.0299 0.0231
17 C -0.0169 0.0043 0.0025
18 C 0.0209 0.0372 -0.1257
19 C 0.0039 -0.0395 -0.0446
20 C 0.0389 0.0095 0.0913
21 C 0.0218 -0.0457 -0.0407
22 C 0.0558 -0.0112 -0.0442
23 C 0.0241 0.0348 0.0827
24 H -0.1134 -0.0691 0.0083
25 H -0.0013 0.0291 -0.0677
26 H 0.0409 0.1452 0.0185
27 H 0.0838 -0.0057 0.2516
28 H 0.1208 0.1674 0.0905
29 H -0.0003 0.0716 -0.2386
30 H 0.0484 0.0414 -0.0315
31 H 0.0286 -0.1191 -0.1372
32 H 0.0208 0.2480 -0.0184
33 H 0.0967 -0.0242 0.0747
34 H 0.1104 -0.0961 -0.0538
35 H 0.0230 -0.0041 0.1241
36 H -0.1396 0.0633 0.1120
37 H -0.0230 -0.2305 0.0130
38 H 0.1020 0.0847 -0.1192
39 H 0.0349 -0.1707 -0.0375
40 H 0.0831 -0.0521 0.2767
41 H 0.0046 -0.1432 -0.1423
42 H -0.0261 0.0316 -0.0066
43 H -0.0109 0.0048 0.0269
44 H 0.1095 0.0567 -0.2324
45 H -0.0352 0.0935 -0.0217
46 H 0.1006 0.0020 0.1537
47 H 0.1006 -0.0092 -0.0129
48 H 0.0239 -0.0116 0.0941
49 H 0.0075 0.0658 0.1682
Vibrational Mode 31
----------------------------------------------------
Harmonic frequency: 561.01 cm**-1
Reduced mass: 3.3221 amu
Force constant: 0.6160 mdyne/A
IR intensity: 3.4850 km/mol
Normal mode:
X Y Z
1 O -0.0694 -0.0182 0.0190
2 O -0.0615 0.0407 -0.0095
3 O 0.0156 0.0337 0.0573
4 C 0.0508 -0.0597 0.0715
5 C -0.0479 0.0519 0.1020
6 C 0.0692 0.0114 -0.0928
7 C 0.0279 -0.0395 0.0650
8 C 0.1179 -0.0246 -0.0332
9 C -0.0009 -0.0552 -0.0055
10 C 0.0324 -0.0912 -0.0663
11 C -0.0106 -0.0948 -0.0508
12 C -0.0582 0.1445 -0.0151
13 C 0.0187 0.0754 0.0066
14 C -0.0157 -0.0421 -0.1398
15 C -0.0494 0.0097 -0.0276
16 C -0.0136 0.0069 -0.0188
17 C -0.0155 -0.0180 0.0486
18 C 0.0317 -0.0457 0.0816
19 C -0.0191 0.0149 -0.0218
20 C 0.0252 -0.0425 -0.0405
21 C 0.0196 0.0103 0.0456
22 C -0.0243 0.0559 0.0799
23 C 0.0317 -0.0096 -0.1022
24 H -0.0739 0.0312 0.0951
25 H 0.0238 0.0430 -0.1198
26 H -0.0101 -0.0202 0.0931
27 H -0.0390 -0.0053 0.1059
28 H 0.2044 0.1403 0.0860
29 H 0.1784 0.0285 -0.2483
30 H -0.0660 -0.0964 -0.0546
31 H -0.0117 -0.0778 -0.0072
32 H -0.0840 0.0347 -0.0238
33 H -0.0714 0.1734 -0.1151
34 H 0.0846 -0.0354 -0.0126
35 H -0.0124 0.0515 0.1678
36 H 0.0002 -0.0201 -0.1321
37 H -0.1966 0.3110 -0.0359
38 H -0.0092 -0.0687 0.2959
39 H -0.0362 0.0885 0.0067
40 H -0.0617 0.0195 -0.1532
41 H -0.0079 0.0716 0.0816
42 H -0.0153 0.0869 0.0033
43 H 0.0173 -0.0199 0.1435
44 H 0.0248 -0.0386 0.1066
45 H -0.0610 0.1373 -0.0124
46 H -0.0125 -0.0387 -0.0808
47 H -0.0192 0.0595 0.1014
48 H 0.0155 0.0024 0.1881
49 H 0.0434 -0.0581 -0.1850
Vibrational Mode 32
----------------------------------------------------
Harmonic frequency: 592.24 cm**-1
Reduced mass: 4.3269 amu
Force constant: 0.8942 mdyne/A
IR intensity: 2.4678 km/mol
Normal mode:
X Y Z
1 O 0.0557 0.0184 -0.0101
2 O 0.0395 -0.0265 0.0062
3 O -0.0793 0.0882 0.1420
4 C -0.0825 -0.0237 -0.0192
5 C -0.0632 -0.0145 -0.0393
6 C -0.0220 -0.0353 0.0443
7 C -0.0002 -0.0094 0.0187
8 C -0.1140 0.0149 -0.0034
9 C -0.0365 -0.0073 0.0356
10 C -0.0274 0.0623 0.0307
11 C 0.0557 0.0497 0.0348
12 C -0.0020 0.0115 -0.0330
13 C 0.0059 -0.0645 -0.0309
14 C -0.0591 0.0161 -0.0244
15 C 0.0711 0.0016 0.0337
16 C -0.0044 0.0071 0.0623
17 C -0.0006 0.0249 -0.0485
18 C 0.1312 -0.0913 -0.0106
19 C -0.0270 -0.0452 -0.1432
20 C 0.1291 -0.0389 0.0307
21 C 0.0069 -0.0621 -0.0492
22 C -0.0622 0.0792 0.1774
23 C 0.0186 0.0066 -0.2469
24 H -0.0302 -0.0412 -0.0327
25 H -0.0078 -0.0060 0.0541
26 H 0.0662 0.1192 -0.0840
27 H 0.1080 -0.0265 0.1414
28 H -0.1090 0.0096 -0.0063
29 H -0.1952 0.0275 0.0151
30 H 0.1449 0.1448 0.0389
31 H 0.0807 -0.0105 -0.0992
32 H 0.0597 0.1865 -0.0289
33 H 0.0806 -0.0751 0.1109
34 H 0.0556 -0.0628 -0.0590
35 H 0.0025 -0.0640 0.0197
36 H -0.1838 0.0504 -0.0217
37 H 0.1912 -0.0555 0.0412
38 H 0.0557 0.0319 -0.0889
39 H 0.0118 0.0000 0.0085
40 H 0.0204 0.0131 0.0938
41 H 0.0282 0.0086 0.0838
42 H -0.0087 -0.0501 -0.0136
43 H -0.0258 0.0268 -0.1148
44 H 0.1709 -0.0630 -0.0250
45 H 0.0253 -0.0675 -0.0079
46 H 0.1584 -0.0277 0.1045
47 H -0.0243 0.1050 0.3057
48 H 0.0088 -0.0134 -0.1052
49 H 0.0476 -0.0194 -0.3723
Vibrational Mode 33
----------------------------------------------------
Harmonic frequency: 604.82 cm**-1
Reduced mass: 5.2629 amu
Force constant: 1.1343 mdyne/A
IR intensity: 1.0874 km/mol
Normal mode:
X Y Z
1 O -0.0302 -0.0130 0.0050
2 O -0.0169 0.0116 -0.0026
3 O 0.0336 -0.0425 0.1940
4 C 0.0661 0.0321 -0.0048
5 C 0.0676 -0.0014 0.0091
6 C -0.0036 0.0298 -0.0172
7 C -0.0034 0.0280 -0.0397
8 C 0.0674 -0.0023 0.0191
9 C 0.0167 0.1007 -0.0122
10 C 0.0103 -0.0270 -0.0065
11 C -0.0507 -0.0355 -0.0286
12 C 0.0226 -0.0553 0.0300
13 C -0.0031 0.0291 0.0183
14 C 0.0155 0.0090 0.0970
15 C -0.0623 -0.0494 0.0088
16 C -0.0115 -0.0046 -0.0094
17 C 0.0035 -0.0148 0.0267
18 C -0.1916 0.1624 -0.1209
19 C 0.1167 -0.0445 -0.1287
20 C -0.1789 0.1040 -0.0024
21 C 0.0240 0.0140 -0.1390
22 C 0.0461 -0.0302 0.1883
23 C 0.1282 -0.1427 -0.1537
24 H 0.0542 0.0231 0.0060
25 H 0.0042 -0.0039 -0.0124
26 H -0.0585 -0.0768 0.0444
27 H -0.0715 0.0318 -0.1489
28 H 0.0362 -0.0466 -0.0141
29 H 0.1186 -0.0283 0.0652
30 H -0.0694 -0.0675 -0.0281
31 H -0.1060 0.0085 0.0073
32 H -0.0132 -0.1482 0.0287
33 H -0.0324 -0.0035 -0.0386
34 H -0.0549 0.0444 0.0448
35 H 0.0204 0.0487 -0.0590
36 H -0.0671 -0.0318 0.0949
37 H -0.1200 -0.2007 0.0023
38 H -0.0295 -0.0012 -0.0715
39 H -0.0064 -0.0919 0.0377
40 H 0.0019 -0.0454 0.1360
41 H -0.0471 -0.1091 -0.1892
42 H 0.0106 0.0234 0.0081
43 H 0.0188 -0.0167 0.0611
44 H -0.2168 0.1431 -0.1088
45 H -0.0144 0.0183 0.0068
46 H -0.1982 0.1497 0.0527
47 H -0.0412 0.0043 0.3110
48 H -0.0063 0.0077 0.0453
49 H 0.1517 -0.1414 -0.2312
Vibrational Mode 34
----------------------------------------------------
Harmonic frequency: 628.27 cm**-1
Reduced mass: 3.5925 amu
Force constant: 0.8355 mdyne/A
IR intensity: 2.8255 km/mol
Normal mode:
X Y Z
1 O -0.0278 0.0193 -0.0196
2 O -0.0366 0.0224 -0.0159
3 O -0.0542 0.0137 0.0521
4 C -0.1276 0.0034 0.0114
5 C -0.0197 -0.0563 0.1117
6 C 0.1300 -0.0063 -0.0222
7 C 0.0399 -0.0461 0.0337
8 C -0.0320 -0.0703 -0.1814
9 C 0.0323 -0.0282 -0.0110
10 C 0.0714 -0.0668 -0.0489
11 C -0.0583 0.1715 0.0971
12 C 0.0286 -0.0364 0.0334
13 C 0.0322 0.0695 0.0265
14 C 0.0439 0.0120 0.0503
15 C -0.0143 0.0635 -0.0015
16 C -0.0128 -0.0191 -0.0287
17 C 0.0183 -0.0513 0.0743
18 C 0.0289 -0.0275 -0.0516
19 C -0.0101 0.0181 0.1080
20 C 0.0321 0.0368 0.0014
21 C 0.0065 -0.0293 -0.1456
22 C -0.0059 -0.0275 -0.0320
23 C -0.0264 0.0002 -0.0203
24 H -0.0354 -0.0726 0.1037
25 H 0.0593 0.0049 -0.0718
26 H 0.1721 -0.0238 -0.0995
27 H 0.0252 -0.0188 0.1429
28 H 0.0084 0.0146 -0.1179
29 H 0.0074 -0.0522 -0.2582
30 H -0.0353 0.2167 0.0927
31 H -0.0091 0.1203 0.0376
32 H -0.0627 -0.2679 0.0335
33 H -0.0219 0.0472 -0.2082
34 H -0.1074 0.2298 0.0762
35 H -0.0660 -0.0449 -0.1493
36 H 0.1129 0.0019 0.0454
37 H 0.1883 -0.1287 0.0126
38 H -0.1577 0.0495 -0.2663
39 H -0.0306 -0.0360 0.0550
40 H -0.0301 -0.0164 -0.0733
41 H -0.0643 -0.0198 -0.0610
42 H 0.0244 -0.0475 0.0671
43 H 0.0121 -0.0490 0.0606
44 H 0.0218 0.0543 0.1476
45 H 0.0268 0.1085 -0.0068
46 H 0.0320 0.1157 0.1821
47 H -0.0125 -0.0339 -0.0687
48 H -0.0358 0.0277 0.0738
49 H -0.0088 0.0145 -0.0703
Vibrational Mode 35
----------------------------------------------------
Harmonic frequency: 640.70 cm**-1
Reduced mass: 4.4940 amu
Force constant: 1.0869 mdyne/A
IR intensity: 0.9997 km/mol
Normal mode:
X Y Z
1 O 0.0209 -0.0011 -0.0098
2 O 0.0190 -0.0114 -0.0005
3 O 0.0218 -0.0694 -0.0934
4 C -0.1240 -0.0332 0.0008
5 C -0.0301 -0.0005 0.0996
6 C 0.0706 -0.0346 0.0015
7 C 0.0356 -0.0544 0.0433
8 C -0.1303 -0.0263 -0.0867
9 C 0.0750 0.0133 -0.0014
10 C -0.0143 0.0111 -0.0096
11 C -0.0150 0.0612 0.0544
12 C -0.0063 0.0683 -0.0151
13 C 0.0265 0.0053 -0.0147
14 C 0.0497 -0.0892 -0.0624
15 C 0.0228 -0.0848 0.0550
16 C -0.0174 0.0055 0.0074
17 C -0.0050 0.0161 -0.0284
18 C -0.0273 0.1944 0.0474
19 C 0.0801 -0.0539 -0.1805
20 C -0.0221 0.0856 -0.0799
21 C -0.0788 0.0947 0.2526
22 C 0.0226 -0.0206 0.0785
23 C 0.0267 -0.0362 0.0322
24 H -0.0340 -0.0357 0.0940
25 H 0.0571 0.0086 -0.0048
26 H 0.1544 0.0993 -0.1187
27 H 0.1190 -0.0460 0.2434
28 H -0.0992 0.0133 -0.0560
29 H -0.1744 -0.0053 -0.1168
30 H 0.0990 0.1936 0.0586
31 H -0.0341 0.0068 -0.1342
32 H -0.0136 -0.0233 -0.0331
33 H -0.0387 0.0995 -0.0689
34 H 0.0247 0.0542 -0.0228
35 H -0.0509 -0.0737 0.0060
36 H -0.0751 -0.1577 -0.0538
37 H 0.1686 -0.1353 0.0634
38 H -0.1051 -0.1269 -0.0825
39 H -0.0532 0.0166 0.1401
40 H -0.0566 0.0156 -0.0998
41 H -0.0910 0.0250 -0.0065
42 H -0.0146 -0.0061 -0.0163
43 H -0.0136 0.0163 -0.0525
44 H -0.0931 0.0159 -0.2456
45 H 0.0018 -0.0254 -0.0151
46 H 0.0681 -0.0843 -0.3364
47 H -0.0253 -0.0080 0.1159
48 H 0.0131 -0.0102 -0.0421
49 H 0.0162 0.0224 0.1206
Vibrational Mode 36
----------------------------------------------------
Harmonic frequency: 654.46 cm**-1
Reduced mass: 3.9097 amu
Force constant: 0.9866 mdyne/A
IR intensity: 5.7871 km/mol
Normal mode:
X Y Z
1 O -0.0276 0.0576 -0.0184
2 O -0.0578 0.0329 -0.0191
3 O 0.0613 0.0282 -0.0450
4 C 0.0208 0.0446 -0.0041
5 C -0.0017 -0.0539 -0.1203
6 C -0.0239 0.0411 0.0150
7 C -0.0545 0.0549 -0.0236
8 C 0.1169 -0.0387 -0.0768
9 C -0.0890 0.0285 -0.0111
10 C 0.1093 -0.0567 -0.0062
11 C -0.0232 0.0818 0.0359
12 C 0.0350 -0.1543 0.0369
13 C -0.0132 0.0156 0.0346
14 C -0.1082 0.0371 0.0097
15 C -0.0358 -0.0163 0.0252
16 C 0.0169 0.0121 -0.0381
17 C 0.0472 -0.0965 0.1430
18 C 0.0191 -0.0008 0.0860
19 C -0.0242 -0.0188 -0.1512
20 C 0.0185 -0.1197 0.0070
21 C 0.0140 0.0016 0.1679
22 C -0.0251 0.0708 0.0290
23 C 0.0133 0.0317 -0.0113
24 H -0.0080 -0.0280 -0.1158
25 H -0.0918 0.0065 -0.0405
26 H -0.0588 -0.1207 0.0390
27 H -0.1463 0.0574 -0.1841
28 H 0.0969 -0.0170 -0.0625
29 H 0.1673 -0.0443 -0.0904
30 H 0.0510 0.2086 0.0351
31 H -0.0782 0.0596 -0.1206
32 H -0.0023 -0.0686 0.0807
33 H 0.1034 -0.1959 0.0212
34 H -0.1116 0.0866 0.0778
35 H 0.0354 0.0542 -0.1465
36 H -0.2281 0.0183 0.0108
37 H 0.0844 -0.1857 0.0310
38 H -0.0503 0.0440 -0.1878
39 H 0.0665 -0.0064 -0.2164
40 H 0.0805 0.0137 0.0875
41 H 0.1134 0.0003 0.0024
42 H 0.0768 -0.1315 0.1430
43 H 0.0323 -0.0939 0.1005
44 H 0.1083 -0.0033 -0.0694
45 H 0.0553 0.1475 0.0108
46 H 0.0328 -0.1822 -0.1159
47 H 0.0031 0.0771 0.0758
48 H -0.0694 0.0493 0.1106
49 H -0.0230 -0.0140 0.0766
Vibrational Mode 37
----------------------------------------------------
Harmonic frequency: 685.03 cm**-1
Reduced mass: 3.3685 amu
Force constant: 0.9313 mdyne/A
IR intensity: 11.5036 km/mol
Normal mode:
X Y Z
1 O 0.0392 0.1269 -0.0414
2 O -0.0383 0.0183 -0.0276
3 O -0.0470 -0.0313 0.0043
4 C -0.0379 -0.0227 -0.0392
5 C -0.0171 0.0198 -0.0410
6 C -0.1114 0.0001 0.0299
7 C -0.1033 0.0591 0.0174
8 C 0.0089 -0.0343 -0.0753
9 C 0.0490 -0.0222 0.0229
10 C 0.1162 0.0053 0.0548
11 C 0.0171 -0.0385 -0.0299
12 C -0.0532 0.0577 -0.0477
13 C -0.0431 -0.0764 -0.0383
14 C 0.0766 -0.0448 -0.0274
15 C 0.0220 -0.0092 -0.0228
16 C 0.0007 0.0083 0.0835
17 C 0.0858 -0.1307 0.1855
18 C 0.0107 0.0187 -0.0313
19 C 0.0331 -0.0050 0.0429
20 C 0.0118 0.1104 -0.0177
21 C -0.0298 0.0213 -0.0473
22 C 0.0215 -0.0477 0.0051
23 C -0.0043 -0.0212 0.0096
24 H 0.0272 0.0106 -0.0310
25 H -0.1853 0.0439 -0.0285
26 H -0.0747 0.1525 -0.0362
27 H -0.0340 0.0496 0.1102
28 H -0.0188 -0.0382 -0.0784
29 H -0.0716 -0.0269 -0.0345
30 H -0.0111 -0.0605 -0.0310
31 H 0.0467 -0.0427 0.0072
32 H 0.0801 0.2978 -0.0733
33 H -0.0007 -0.0352 0.2297
34 H 0.1566 -0.2748 -0.1140
35 H 0.0539 0.0401 0.2186
36 H 0.1251 -0.0162 -0.0266
37 H -0.0656 0.1390 -0.0265
38 H 0.0080 -0.0720 0.1411
39 H -0.0203 0.0390 0.1400
40 H -0.0261 0.0139 0.0128
41 H -0.0279 0.0295 0.0998
42 H 0.1340 -0.3201 0.2403
43 H 0.0333 -0.1305 0.0161
44 H -0.0763 -0.0586 -0.0703
45 H 0.0921 0.1319 -0.0091
46 H 0.0152 0.0915 -0.0499
47 H -0.0031 -0.0473 -0.0085
48 H -0.0791 0.0518 0.0622
49 H 0.0221 0.0330 -0.0367
Vibrational Mode 38
----------------------------------------------------
Harmonic frequency: 744.40 cm**-1
Reduced mass: 2.6426 amu
Force constant: 0.8628 mdyne/A
IR intensity: 7.3244 km/mol
Normal mode:
X Y Z
1 O 0.0238 0.0387 -0.0141
2 O 0.0079 -0.0045 -0.0137
3 O 0.0613 0.0409 -0.0004
4 C -0.0103 -0.0016 -0.0291
5 C 0.0586 0.0381 0.1960
6 C -0.0371 -0.0141 -0.0481
7 C -0.0277 0.0180 -0.0433
8 C -0.0585 0.0300 0.0007
9 C 0.0410 0.0318 -0.0149
10 C 0.0016 0.0318 0.0417
11 C 0.0165 -0.0426 -0.0198
12 C 0.0304 0.0354 0.0145
13 C 0.0138 -0.0601 -0.0706
14 C -0.0402 0.0302 0.0173
15 C 0.0114 -0.0075 0.0224
16 C -0.0039 -0.0094 -0.0978
17 C 0.0409 -0.0466 0.0705
18 C -0.0464 -0.0125 -0.0514
19 C -0.0330 0.0161 -0.0084
20 C -0.0467 -0.1478 0.0029
21 C 0.0313 -0.0207 0.0517
22 C -0.0396 0.0451 -0.0126
23 C -0.0049 0.0073 -0.0517
24 H 0.0585 0.0623 0.1838
25 H -0.0184 0.0420 -0.0310
26 H -0.0216 0.1908 -0.1023
27 H 0.0748 0.0089 0.1101
28 H -0.1199 -0.0919 -0.0862
29 H -0.0999 -0.0105 0.1597
30 H 0.0495 -0.0670 -0.0129
31 H 0.0063 -0.0335 -0.0137
32 H 0.0571 -0.1876 -0.0625
33 H -0.1343 0.1593 -0.0460
34 H 0.0383 -0.1610 -0.0627
35 H 0.0488 -0.0157 0.0170
36 H -0.0740 0.0005 0.0176
37 H 0.0120 -0.0878 0.0204
38 H 0.0783 0.0569 -0.0330
39 H -0.0439 -0.0269 0.0683
40 H -0.0631 -0.0467 -0.1069
41 H -0.0825 -0.0728 -0.2367
42 H 0.0706 -0.1432 0.0962
43 H 0.0272 -0.0525 -0.0126
44 H 0.0442 0.2216 0.3569
45 H 0.0023 -0.0109 -0.0149
46 H -0.0185 -0.0322 0.2908
47 H -0.0185 0.0400 -0.0238
48 H -0.0091 0.0073 -0.0061
49 H -0.1159 0.0008 0.3344
Vibrational Mode 39
----------------------------------------------------
Harmonic frequency: 749.04 cm**-1
Reduced mass: 1.3390 amu
Force constant: 0.4426 mdyne/A
IR intensity: 7.9187 km/mol
Normal mode:
X Y Z
1 O -0.0043 -0.0066 0.0026
2 O -0.0022 0.0012 0.0028
3 O -0.0258 -0.0047 -0.0019
4 C 0.0021 -0.0029 0.0055
5 C -0.0102 -0.0073 -0.0416
6 C 0.0059 0.0024 0.0094
7 C 0.0047 -0.0058 0.0147
8 C 0.0134 -0.0068 0.0018
9 C 0.0001 -0.0145 0.0016
10 C 0.0010 -0.0069 -0.0089
11 C -0.0045 0.0046 -0.0066
12 C -0.0106 0.0027 -0.0052
13 C -0.0062 0.0138 0.0155
14 C 0.0326 0.0041 0.0383
15 C -0.0039 0.0208 -0.0139
16 C 0.0018 -0.0012 0.0081
17 C -0.0081 0.0087 -0.0137
18 C 0.0147 -0.0247 0.0015
19 C 0.0216 -0.0006 0.0052
20 C 0.0127 0.0274 -0.0146
21 C -0.0154 0.0208 0.0185
22 C -0.0032 -0.0179 0.0164
23 C 0.0137 -0.0335 -0.1283
24 H -0.0112 -0.0130 -0.0389
25 H -0.0011 -0.0079 0.0037
26 H 0.0000 -0.0359 0.0282
27 H -0.0170 -0.0027 -0.0119
28 H 0.0290 0.0213 0.0219
29 H 0.0205 0.0030 -0.0346
30 H -0.0465 -0.0338 -0.0092
31 H 0.0203 0.0096 0.0514
32 H -0.0115 0.0504 0.0084
33 H 0.0190 -0.0217 0.0144
34 H -0.0004 0.0241 0.0096
35 H -0.0132 0.0057 0.0142
36 H 0.0518 0.0135 0.0335
37 H -0.0404 0.0082 -0.0159
38 H -0.0072 0.0136 -0.0011
39 H 0.0094 -0.0011 -0.0199
40 H 0.0121 0.0035 0.0139
41 H 0.0161 0.0058 0.0264
42 H -0.0142 0.0279 -0.0187
43 H -0.0054 0.0099 0.0033
44 H -0.0090 -0.0158 0.0574
45 H 0.0014 0.0046 0.0033
46 H -0.0004 0.0490 0.0208
47 H -0.0224 -0.0139 0.0221
48 H 0.0011 -0.0010 0.0025
49 H -0.2097 0.2569 0.9077
Vibrational Mode 40
----------------------------------------------------
Harmonic frequency: 778.30 cm**-1
Reduced mass: 2.7938 amu
Force constant: 0.9971 mdyne/A
IR intensity: 3.3164 km/mol
Normal mode:
X Y Z
1 O -0.0069 -0.0109 0.0021
2 O -0.0015 0.0002 0.0037
3 O 0.0648 -0.0036 0.0111
4 C 0.0011 0.0136 0.0082
5 C -0.0342 -0.0236 -0.0281
6 C 0.0199 0.0114 0.0163
7 C 0.0193 0.0177 -0.0285
8 C 0.0117 -0.0092 -0.0074
9 C -0.0182 0.0390 -0.0066
10 C -0.0009 -0.0046 -0.0034
11 C -0.0069 0.0298 0.0535
12 C -0.0097 -0.0492 -0.0064
13 C 0.0026 0.0066 0.0039
14 C -0.0427 -0.0740 -0.1936
15 C 0.0075 -0.0895 0.0177
16 C 0.0017 0.0296 0.1877
17 C -0.0099 0.0127 -0.0186
18 C -0.0402 0.0701 -0.1478
19 C -0.0750 0.0249 0.0867
20 C -0.0138 -0.0432 -0.0334
21 C 0.0103 -0.0215 0.0057
22 C 0.0114 0.0534 -0.0117
23 C 0.0574 -0.0152 -0.0056
24 H -0.0233 -0.0295 -0.0246
25 H 0.0473 -0.0248 0.0348
26 H 0.0337 -0.0721 -0.0152
27 H -0.0203 0.0186 -0.0944
28 H 0.0164 0.0136 0.0086
29 H 0.0244 -0.0033 -0.0338
30 H 0.1101 0.2058 0.0542
31 H -0.1023 0.0066 -0.1771
32 H 0.0289 0.0910 0.0042
33 H 0.0764 -0.1251 0.0751
34 H 0.0248 0.0223 -0.0122
35 H 0.0146 0.0175 -0.0039
36 H 0.0085 -0.0603 -0.1744
37 H 0.1322 0.1598 0.0291
38 H -0.0982 -0.1943 0.1284
39 H -0.0070 0.0045 0.2284
40 H -0.0000 0.0189 0.2108
41 H -0.0391 0.0137 0.1375
42 H -0.0178 0.0277 -0.0210
43 H -0.0065 0.0128 -0.0054
44 H 0.0577 0.3376 0.3145
45 H -0.0047 -0.0082 0.0024
46 H 0.0808 0.0739 0.3486
47 H 0.0655 0.0148 -0.1473
48 H 0.0034 -0.0035 -0.0036
49 H -0.0055 -0.0381 0.1964
Vibrational Mode 41
----------------------------------------------------
Harmonic frequency: 779.34 cm**-1
Reduced mass: 1.9039 amu
Force constant: 0.6813 mdyne/A
IR intensity: 6.1737 km/mol
Normal mode:
X Y Z
1 O 0.0041 0.0058 -0.0022
2 O 0.0038 -0.0017 -0.0036
3 O 0.0199 0.0019 0.0276
4 C -0.0001 0.0063 -0.0015
5 C 0.0038 0.0093 0.0512
6 C -0.0036 -0.0036 -0.0207
7 C -0.0049 0.0065 -0.0262
8 C -0.0151 0.0138 0.0037
9 C -0.0235 0.0153 -0.0008
10 C -0.0047 0.0094 0.0097
11 C 0.0068 -0.0032 0.0217
12 C 0.0177 -0.0124 0.0127
13 C 0.0117 -0.0183 -0.0189
14 C -0.0545 -0.0173 -0.0880
15 C 0.0076 -0.0406 0.0170
16 C -0.0034 -0.0041 -0.0270
17 C 0.0096 -0.0101 0.0169
18 C -0.0095 0.0813 0.0832
19 C -0.0645 0.0144 0.0858
20 C -0.0064 0.0121 0.1061
21 C 0.0333 -0.0588 -0.1103
22 C 0.0132 0.0309 -0.0238
23 C 0.0411 -0.0051 -0.0340
24 H 0.0003 0.0124 0.0464
25 H 0.0080 0.0083 -0.0109
26 H 0.0018 0.0416 -0.0430
27 H 0.0224 0.0015 0.0008
28 H -0.0354 -0.0262 -0.0249
29 H -0.0214 -0.0001 0.0521
30 H 0.0846 0.0846 0.0249
31 H -0.0426 -0.0153 -0.1005
32 H -0.0021 -0.0902 0.0042
33 H -0.0083 0.0187 -0.0453
34 H -0.0219 -0.0175 0.0009
35 H 0.0149 -0.0154 -0.0456
36 H -0.0015 -0.0431 -0.0787
37 H 0.0873 0.0347 0.0228
38 H 0.0002 -0.0462 0.0235
39 H -0.0054 0.0283 -0.0449
40 H -0.0050 0.0156 -0.0814
41 H 0.0048 0.0383 0.0425
42 H 0.0181 -0.0305 0.0211
43 H 0.0071 -0.0116 -0.0015
44 H -0.0028 -0.2336 -0.6529
45 H -0.0050 -0.0084 -0.0043
46 H -0.0010 -0.2336 -0.4468
47 H 0.0422 0.0370 0.0235
48 H -0.0004 0.0011 -0.0035
49 H -0.0408 0.0452 0.2968
Vibrational Mode 42
----------------------------------------------------
Harmonic frequency: 789.81 cm**-1
Reduced mass: 3.0161 amu
Force constant: 1.1085 mdyne/A
IR intensity: 2.6437 km/mol
Normal mode:
X Y Z
1 O -0.0044 -0.0102 0.0102
2 O -0.0031 0.0015 0.0031
3 O 0.0309 0.0292 0.0045
4 C -0.0272 0.0217 -0.0273
5 C 0.0951 0.1140 -0.1371
6 C -0.0555 -0.0353 0.1483
7 C -0.0784 -0.0447 0.1753
8 C -0.0367 -0.0485 -0.1416
9 C 0.0320 0.0344 -0.0057
10 C 0.0162 -0.0221 -0.0229
11 C 0.0276 -0.0661 -0.0441
12 C 0.0792 0.0681 -0.0026
13 C 0.0581 -0.0070 0.0516
14 C 0.0077 -0.0061 -0.0066
15 C 0.0341 -0.0410 -0.0038
16 C 0.0143 0.0126 0.0371
17 C -0.0112 0.0257 -0.0247
18 C -0.0375 0.0091 -0.0140
19 C -0.0114 0.0080 0.0080
20 C -0.0448 -0.0730 0.0421
21 C 0.0246 -0.0219 -0.0138
22 C -0.0279 0.0135 -0.0144
23 C -0.0227 0.0104 -0.0108
24 H 0.1040 0.1360 -0.1265
25 H -0.2033 0.1042 0.0340
26 H -0.0640 0.0764 0.1263
27 H 0.0553 -0.0815 0.2231
28 H -0.0529 -0.0154 -0.1192
29 H -0.0307 -0.0418 -0.1568
30 H 0.0085 -0.1128 -0.0413
31 H 0.0205 -0.0458 0.0011
32 H -0.1493 -0.1989 0.0809
33 H -0.1206 0.2469 -0.2185
34 H -0.2051 0.3389 0.1361
35 H -0.0663 -0.1500 -0.3189
36 H 0.0176 -0.0109 -0.0058
37 H -0.0862 0.0572 -0.0117
38 H 0.1033 -0.0379 0.1395
39 H 0.0112 -0.0136 0.0637
40 H 0.0043 -0.0032 0.0574
41 H -0.0099 -0.0175 -0.0229
42 H -0.0289 0.0450 -0.0261
43 H -0.0306 0.0352 -0.0266
44 H 0.0067 0.0281 -0.0305
45 H 0.0099 0.0383 0.0072
46 H -0.0325 -0.0911 0.0089
47 H -0.0244 0.0210 0.0185
48 H 0.0129 -0.0101 -0.0092
49 H -0.0440 -0.0487 0.0152
Vibrational Mode 43
----------------------------------------------------
Harmonic frequency: 819.16 cm**-1
Reduced mass: 2.9630 amu
Force constant: 1.1715 mdyne/A
IR intensity: 6.2881 km/mol
Normal mode:
X Y Z
1 O -0.0059 -0.0080 0.0032
2 O -0.0072 0.0019 0.0083
3 O 0.0553 0.1166 -0.0035
4 C -0.0063 -0.0150 0.0037
5 C 0.0079 -0.0592 0.0037
6 C 0.0050 0.0106 0.0606
7 C 0.0345 0.0092 -0.0189
8 C 0.0289 -0.0330 -0.0217
9 C 0.0856 0.0255 -0.0166
10 C 0.0095 -0.0118 -0.0049
11 C -0.0322 0.0289 0.0025
12 C -0.0628 0.0060 -0.0513
13 C -0.0634 0.0441 0.0201
14 C 0.1393 -0.0369 0.0419
15 C -0.0167 -0.0094 -0.0044
16 C 0.0143 0.0091 0.0182
17 C -0.0187 0.0202 -0.0367
18 C -0.0358 0.0934 0.0016
19 C 0.0555 -0.0032 -0.0111
20 C -0.0334 -0.1520 0.0846
21 C 0.0704 -0.0284 -0.0160
22 C -0.0985 -0.0511 -0.0430
23 C -0.1557 0.0531 -0.0465
24 H 0.0331 -0.1119 0.0063
25 H 0.0544 -0.0943 0.0906
26 H 0.0817 -0.1111 -0.0258
27 H -0.0388 0.0254 -0.0645
28 H 0.0607 0.0199 0.0173
29 H 0.0379 -0.0162 -0.0780
30 H -0.0797 0.0048 -0.0020
31 H -0.0085 0.0292 0.0490
32 H 0.1252 0.2775 -0.1018
33 H 0.0970 -0.1519 0.1923
34 H 0.1995 -0.1023 -0.1031
35 H -0.0015 0.1159 0.2861
36 H 0.1935 -0.1046 0.0371
37 H -0.0623 -0.0405 -0.0078
38 H -0.1125 -0.0770 -0.0028
39 H -0.0316 0.0160 0.1859
40 H -0.0454 -0.0154 -0.0303
41 H -0.0689 -0.0181 -0.0711
42 H -0.0381 0.0375 -0.0343
43 H -0.0110 0.0191 -0.0114
44 H 0.0747 0.0566 -0.2436
45 H 0.0108 0.0088 0.0090
46 H 0.0754 -0.2593 -0.0266
47 H -0.1620 0.0308 0.2678
48 H 0.0005 -0.0036 -0.0009
49 H -0.1879 -0.1043 -0.0571
Vibrational Mode 44
----------------------------------------------------
Harmonic frequency: 832.40 cm**-1
Reduced mass: 1.4856 amu
Force constant: 0.6065 mdyne/A
IR intensity: 8.9459 km/mol
Normal mode:
X Y Z
1 O 0.0111 0.0150 -0.0094
2 O 0.0066 -0.0012 -0.0080
3 O -0.0045 0.0094 0.0129
4 C 0.0007 0.0057 0.0151
5 C -0.0230 0.0089 -0.0349
6 C 0.0295 -0.0011 -0.1011
7 C 0.0000 -0.0110 0.0107
8 C -0.0416 0.0245 0.0503
9 C -0.0251 0.0023 -0.0080
10 C -0.0111 0.0067 -0.0037
11 C 0.0025 0.0078 -0.0028
12 C 0.0084 -0.0103 0.0338
13 C 0.0176 -0.0135 -0.0000
14 C 0.0205 -0.0143 -0.0039
15 C 0.0153 -0.0175 -0.0060
16 C -0.0023 0.0064 0.0374
17 C 0.0145 -0.0261 0.0334
18 C 0.0037 0.0095 -0.0099
19 C 0.0065 0.0039 0.0114
20 C 0.0072 -0.0052 -0.0136
21 C -0.0025 0.0085 0.0200
22 C 0.0216 -0.0375 -0.1158
23 C -0.0126 0.0024 -0.0007
24 H -0.0577 0.0226 -0.0365
25 H -0.0071 0.0340 -0.1197
26 H -0.0658 0.0320 0.0564
27 H -0.0081 -0.0069 0.0093
28 H -0.0444 0.0288 0.0519
29 H -0.0529 0.0300 0.0364
30 H -0.0349 -0.0232 -0.0050
31 H -0.0019 0.0229 0.0383
32 H -0.0951 -0.1035 0.0767
33 H -0.0282 0.0386 -0.0881
34 H -0.1204 0.0084 0.0738
35 H -0.0201 -0.0559 -0.1003
36 H 0.0424 -0.0146 -0.0042
37 H -0.0359 0.0501 -0.0082
38 H 0.0014 -0.0486 0.0650
39 H 0.0174 -0.0094 -0.0269
40 H 0.0256 0.0114 0.0770
41 H 0.0262 0.0070 0.0544
42 H 0.0331 -0.0242 0.0239
43 H 0.0208 -0.0284 0.0327
44 H 0.0255 0.0382 0.0190
45 H 0.0015 -0.0066 -0.0091
46 H 0.0266 0.0124 0.0526
47 H -0.1950 0.2014 0.8544
48 H -0.0090 0.0102 0.0111
49 H -0.0099 0.0004 -0.0111
Vibrational Mode 45
----------------------------------------------------
Harmonic frequency: 834.17 cm**-1
Reduced mass: 2.2241 amu
Force constant: 0.9119 mdyne/A
IR intensity: 4.6988 km/mol
Normal mode:
X Y Z
1 O -0.0203 -0.0275 0.0169
2 O -0.0108 0.0017 0.0132
3 O -0.0151 -0.0441 0.0196
4 C -0.0004 -0.0045 -0.0272
5 C 0.0318 -0.0056 0.0669
6 C -0.0526 0.0012 0.1734
7 C -0.0063 0.0219 -0.0223
8 C 0.0732 -0.0370 -0.0881
9 C 0.0236 -0.0136 0.0147
10 C 0.0184 -0.0093 0.0085
11 C 0.0028 -0.0169 0.0119
12 C -0.0025 0.0117 -0.0500
13 C -0.0155 0.0129 -0.0076
14 C -0.0789 0.0294 -0.0217
15 C -0.0226 0.0282 0.0101
16 C 0.0018 -0.0139 -0.0548
17 C -0.0229 0.0451 -0.0542
18 C 0.0030 -0.0381 0.0053
19 C -0.0465 0.0092 0.0218
20 C -0.0021 0.0377 -0.0250
21 C -0.0275 0.0026 0.0158
22 C 0.0603 0.0190 -0.0971
23 C 0.0758 -0.0214 0.0220
24 H 0.0891 -0.0189 0.0692
25 H 0.0102 -0.0415 0.2057
26 H 0.1076 -0.0383 -0.1053
27 H 0.0217 0.0108 -0.0144
28 H 0.0712 -0.0590 -0.1011
29 H 0.0943 -0.0521 -0.0478
30 H 0.0906 0.0615 0.0168
31 H -0.0036 -0.0462 -0.0942
32 H 0.1515 0.1351 -0.1182
33 H 0.0355 -0.0492 0.1282
34 H 0.1812 0.0078 -0.1171
35 H 0.0412 0.0761 0.1161
36 H -0.1051 0.0388 -0.0188
37 H 0.0834 -0.0543 0.0160
38 H 0.0314 0.1009 -0.1075
39 H -0.0261 0.0188 0.0287
40 H -0.0391 -0.0148 -0.1312
41 H -0.0366 0.0010 -0.0544
42 H -0.0534 0.0356 -0.0362
43 H -0.0371 0.0496 -0.0608
44 H -0.0596 -0.0334 0.1164
45 H -0.0065 0.0078 0.0151
46 H -0.0460 0.0709 -0.0043
47 H -0.1119 0.2021 0.6617
48 H 0.0177 -0.0190 -0.0215
49 H 0.0809 0.0202 0.0335
Vibrational Mode 46
----------------------------------------------------
Harmonic frequency: 857.90 cm**-1
Reduced mass: 2.1369 amu
Force constant: 0.9266 mdyne/A
IR intensity: 1.8707 km/mol
Normal mode:
X Y Z
1 O -0.0324 -0.0389 0.0190
2 O 0.0002 -0.0006 0.0007
3 O -0.0067 0.0048 -0.0037
4 C -0.0037 0.0671 -0.0177
5 C -0.0963 -0.0207 0.1176
6 C -0.0206 0.0019 0.0737
7 C -0.0230 -0.0032 -0.0028
8 C 0.0580 0.0589 -0.0693
9 C -0.1171 0.0002 0.0078
10 C 0.0110 0.0119 -0.0061
11 C 0.0531 0.0601 -0.0590
12 C 0.0177 -0.0489 0.0021
13 C 0.0501 -0.0122 -0.0389
14 C 0.0277 -0.0416 0.0259
15 C 0.0597 -0.0700 -0.0367
16 C -0.0409 -0.0014 -0.0122
17 C -0.0016 0.0110 -0.0049
18 C 0.0195 0.0520 -0.0078
19 C 0.0169 -0.0028 -0.0126
20 C 0.0381 0.0072 -0.0222
21 C 0.0003 0.0055 0.0048
22 C -0.0050 -0.0146 0.0048
23 C -0.0186 0.0052 -0.0037
24 H -0.1006 -0.0444 0.1060
25 H -0.0083 0.0683 0.0784
26 H 0.0448 0.0680 -0.0844
27 H 0.0528 -0.0172 0.0629
28 H -0.0027 -0.1736 -0.2264
29 H 0.1147 -0.0428 0.2103
30 H -0.1820 -0.2952 -0.0629
31 H 0.0057 0.2089 0.3350
32 H 0.0523 -0.0410 -0.0203
33 H 0.0829 -0.0920 -0.0101
34 H 0.0394 0.0917 -0.0510
35 H 0.0511 -0.0134 -0.1218
36 H 0.0368 0.0008 0.0227
37 H -0.2572 0.1663 -0.0546
38 H 0.0430 -0.1845 0.3075
39 H 0.0292 -0.0517 -0.2305
40 H 0.0726 0.0215 0.1495
41 H 0.0839 0.0025 0.0671
42 H -0.0068 -0.0014 0.0018
43 H -0.0078 0.0114 -0.0240
44 H 0.0907 0.0632 -0.1067
45 H -0.0204 0.0144 0.0117
46 H 0.0995 0.0244 0.1000
47 H 0.0025 -0.0159 -0.0036
48 H 0.0117 -0.0091 -0.0135
49 H -0.0129 0.0122 -0.0162
Vibrational Mode 47
----------------------------------------------------
Harmonic frequency: 883.70 cm**-1
Reduced mass: 1.9069 amu
Force constant: 0.8774 mdyne/A
IR intensity: 2.0286 km/mol
Normal mode:
X Y Z
1 O 0.0151 0.0144 -0.0147
2 O -0.0024 -0.0010 0.0023
3 O -0.0256 -0.0363 0.0047
4 C 0.0175 0.0349 0.0041
5 C 0.0076 0.0216 0.0169
6 C 0.0645 0.0438 -0.0225
7 C 0.0487 0.0470 0.0984
8 C -0.0976 -0.0609 -0.0434
9 C 0.0237 0.0232 0.0098
10 C 0.0025 -0.0228 0.0086
11 C 0.0388 0.0430 -0.0375
12 C -0.0456 -0.0806 0.0287
13 C -0.0197 -0.0274 -0.0412
14 C -0.0112 -0.0180 0.0214
15 C 0.0298 -0.0260 -0.0190
16 C 0.0102 0.0123 -0.0307
17 C -0.0058 0.0213 -0.0161
18 C -0.0022 -0.0267 -0.0289
19 C -0.0290 0.0161 -0.0152
20 C -0.0085 0.0032 0.0674
21 C -0.0139 -0.0157 -0.0017
22 C 0.0042 0.0703 -0.0145
23 C 0.0521 -0.0114 0.0140
24 H 0.0237 0.0796 0.0207
25 H 0.0333 0.0821 -0.0380
26 H -0.0545 -0.2431 0.2790
27 H -0.1998 0.0926 -0.1345
28 H -0.1252 0.1233 0.0762
29 H -0.0733 -0.0053 -0.2230
30 H -0.1197 -0.1622 -0.0414
31 H 0.0234 0.1271 0.2063
32 H 0.0673 0.0643 -0.0111
33 H -0.0250 -0.1214 0.1919
34 H 0.0904 -0.1255 -0.0818
35 H 0.0424 0.0414 0.0710
36 H -0.0457 -0.0054 0.0199
37 H -0.1605 0.0500 -0.0313
38 H 0.0541 -0.0569 0.1412
39 H -0.0099 -0.0084 0.0578
40 H -0.0228 -0.0266 0.0158
41 H -0.0307 -0.0482 -0.1440
42 H -0.0255 -0.0138 0.0047
43 H -0.0183 0.0201 -0.0521
44 H 0.0058 0.1093 0.2728
45 H 0.0033 -0.0311 -0.0097
46 H -0.0206 -0.2022 -0.4104
47 H -0.0658 0.0710 -0.0277
48 H 0.0170 -0.0150 -0.0206
49 H 0.0552 0.0037 0.0060
Vibrational Mode 48
----------------------------------------------------
Harmonic frequency: 891.86 cm**-1
Reduced mass: 1.3866 amu
Force constant: 0.6498 mdyne/A
IR intensity: 2.1836 km/mol
Normal mode:
X Y Z
1 O -0.0226 -0.0262 0.0129
2 O 0.0062 -0.0011 -0.0050
3 O 0.0013 0.0149 -0.0024
4 C -0.0085 -0.0047 0.0024
5 C -0.0121 -0.0161 -0.0105
6 C -0.0080 -0.0123 -0.0035
7 C -0.0139 -0.0314 -0.0189
8 C 0.0362 0.0693 -0.0074
9 C -0.0063 0.0180 -0.0034
10 C 0.0006 0.0258 -0.0179
11 C -0.0204 -0.0220 0.0145
12 C 0.0048 0.0121 -0.0122
13 C 0.0074 0.0228 0.0186
14 C 0.0171 0.0041 -0.0174
15 C -0.0024 -0.0042 0.0100
16 C -0.0043 0.0088 0.0025
17 C 0.0040 -0.0175 0.0173
18 C 0.0050 -0.0477 -0.0601
19 C 0.0185 -0.0053 -0.0002
20 C 0.0017 0.0491 0.0858
21 C 0.0084 0.0098 0.0046
22 C 0.0002 -0.0357 0.0085
23 C -0.0275 0.0060 -0.0075
24 H -0.0221 -0.0417 -0.0113
25 H -0.0136 -0.0196 -0.0093
26 H 0.0252 0.0924 -0.0922
27 H 0.0788 -0.0436 0.0854
28 H 0.0367 -0.1480 -0.1493
29 H 0.0201 -0.0045 0.2280
30 H 0.0496 0.0691 0.0165
31 H -0.0259 -0.0532 -0.0967
32 H -0.0064 0.0254 0.0001
33 H 0.0324 -0.0058 -0.0279
34 H -0.0183 0.0578 0.0248
35 H -0.0175 -0.0051 -0.0121
36 H 0.0164 0.0222 -0.0158
37 H 0.0640 0.0063 0.0151
38 H -0.0209 -0.0101 -0.0128
39 H 0.0058 -0.0212 -0.0136
40 H 0.0121 -0.0019 0.0645
41 H 0.0081 -0.0199 -0.0323
42 H 0.0184 0.0003 0.0042
43 H 0.0191 -0.0210 0.0334
44 H 0.0421 0.2156 0.4838
45 H -0.0111 0.0162 0.0086
46 H -0.0510 -0.2512 -0.6614
47 H 0.0218 -0.0303 0.0342
48 H -0.0087 0.0092 0.0085
49 H -0.0214 0.0151 -0.0169
Vibrational Mode 49
----------------------------------------------------
Harmonic frequency: 893.83 cm**-1
Reduced mass: 2.4473 amu
Force constant: 1.1520 mdyne/A
IR intensity: 4.8610 km/mol
Normal mode:
X Y Z
1 O 0.0166 0.0075 -0.0051
2 O -0.0260 0.0022 0.0175
3 O 0.0408 0.0573 -0.0059
4 C -0.0031 -0.0270 -0.0043
5 C -0.0363 0.0589 0.0502
6 C -0.0025 0.0200 0.0387
7 C -0.0387 0.0931 -0.0833
8 C 0.0410 -0.1409 0.0311
9 C -0.0526 0.0077 0.0099
10 C 0.0188 -0.0271 0.0681
11 C -0.0172 -0.0105 0.0286
12 C 0.0197 0.0018 0.0468
13 C 0.0624 -0.1036 -0.0659
14 C 0.0053 0.0054 -0.0214
15 C -0.0190 -0.0174 -0.0103
16 C -0.0196 0.0004 -0.0134
17 C 0.0124 0.0874 -0.0305
18 C 0.0106 -0.0186 -0.0193
19 C 0.0406 -0.0302 0.0158
20 C 0.0148 0.0556 0.0040
21 C 0.0232 0.0246 0.0067
22 C -0.0011 -0.1168 0.0264
23 C -0.0849 0.0195 -0.0228
24 H -0.0431 0.1373 0.0470
25 H 0.0926 0.0760 0.1090
26 H -0.0119 0.0051 -0.0773
27 H -0.0967 0.1020 -0.1233
28 H 0.0908 0.2597 0.2960
29 H 0.0440 0.0081 -0.4241
30 H 0.0212 0.1218 0.0234
31 H -0.0458 -0.0353 -0.1004
32 H -0.0677 -0.1452 0.0628
33 H -0.1253 0.1159 -0.0293
34 H -0.0646 0.0110 -0.0102
35 H 0.0342 -0.1319 -0.2202
36 H 0.0209 0.0656 -0.0202
37 H 0.0093 0.0575 -0.0058
38 H -0.0473 -0.0504 0.0345
39 H 0.0170 -0.0248 -0.1295
40 H 0.0325 0.0071 0.0707
41 H 0.0443 -0.0038 0.0180
42 H -0.0436 -0.1164 0.0706
43 H -0.1066 0.1060 -0.2483
44 H 0.0037 0.0493 0.1405
45 H -0.0381 -0.0881 -0.0157
46 H -0.0314 -0.0006 -0.1851
47 H 0.1386 -0.1151 0.0704
48 H 0.0730 -0.0682 -0.0915
49 H -0.0867 -0.0034 -0.0189
Vibrational Mode 50
----------------------------------------------------
Harmonic frequency: 900.73 cm**-1
Reduced mass: 2.2914 amu
Force constant: 1.0953 mdyne/A
IR intensity: 3.4867 km/mol
Normal mode:
X Y Z
1 O -0.0611 -0.0923 0.0305
2 O -0.0000 -0.0064 -0.0052
3 O 0.0373 0.0421 -0.0035
4 C 0.0170 0.0265 0.0115
5 C 0.0154 0.0226 -0.0111
6 C 0.0813 0.0359 -0.0485
7 C 0.0279 0.0395 0.1029
8 C -0.0307 0.0824 -0.1159
9 C 0.0058 0.0108 0.0043
10 C 0.0318 0.0566 -0.0093
11 C -0.0271 -0.0714 0.0142
12 C -0.0531 -0.0518 0.0316
13 C -0.0125 -0.0299 -0.0310
14 C -0.0239 0.0346 -0.0341
15 C 0.0027 -0.0039 0.0200
16 C 0.0034 0.0067 -0.0101
17 C 0.0278 0.0550 0.0340
18 C 0.0040 -0.0308 0.0358
19 C 0.0197 -0.0247 0.0254
20 C -0.0020 0.0277 -0.0436
21 C 0.0162 0.0182 0.0025
22 C 0.0062 -0.0945 0.0212
23 C -0.0588 0.0125 -0.0161
24 H 0.0047 0.0789 -0.0081
25 H 0.0148 0.1008 -0.0898
26 H -0.0442 -0.1813 0.2328
27 H -0.1812 0.0866 -0.0613
28 H -0.0623 -0.2279 -0.3182
29 H -0.0696 -0.0239 0.2482
30 H 0.1123 0.1297 0.0189
31 H -0.0668 -0.1183 -0.2173
32 H 0.0236 0.0714 0.0124
33 H -0.0277 -0.0919 0.1726
34 H 0.0527 -0.1040 -0.0519
35 H -0.0008 -0.0144 0.0553
36 H -0.0223 0.0670 -0.0305
37 H 0.1117 0.0132 0.0273
38 H 0.0669 0.0505 -0.0131
39 H -0.0005 0.0038 0.0053
40 H -0.0067 -0.0075 0.0103
41 H -0.0055 -0.0155 -0.0501
42 H -0.0131 -0.2062 0.1452
43 H -0.0626 0.0507 -0.2513
44 H -0.0723 -0.1450 -0.1102
45 H -0.0850 -0.0711 0.0080
46 H -0.0747 0.1697 0.1794
47 H 0.1531 -0.0966 0.0573
48 H 0.0865 -0.0703 -0.1213
49 H -0.0633 -0.0061 -0.0018
Vibrational Mode 51
----------------------------------------------------
Harmonic frequency: 910.02 cm**-1
Reduced mass: 2.1306 amu
Force constant: 1.0396 mdyne/A
IR intensity: 14.0051 km/mol
Normal mode:
X Y Z
1 O -0.0482 -0.0884 0.0363
2 O -0.0287 -0.0031 0.0086
3 O -0.0555 -0.0489 0.0011
4 C -0.0056 -0.0406 -0.0035
5 C -0.0166 -0.0169 -0.0341
6 C 0.0067 -0.0035 -0.0607
7 C -0.0517 -0.0056 -0.0370
8 C 0.0708 -0.0079 0.0009
9 C 0.0299 -0.0069 -0.0226
10 C 0.0463 0.0539 0.0419
11 C 0.0044 0.0311 -0.0011
12 C -0.0065 0.0307 0.0066
13 C 0.0077 -0.0102 0.0104
14 C 0.0475 -0.0243 0.0326
15 C -0.0199 0.0296 -0.0084
16 C 0.0149 0.0022 0.0320
17 C 0.0628 0.1235 0.0410
18 C -0.0054 0.0198 -0.0032
19 C -0.0069 0.0344 -0.0274
20 C -0.0070 -0.0571 0.0280
21 C -0.0151 -0.0158 -0.0058
22 C -0.0109 0.1055 -0.0236
23 C 0.0644 -0.0150 0.0179
24 H -0.0383 -0.0299 -0.0346
25 H -0.0128 0.0160 -0.0733
26 H -0.0388 0.1614 -0.0983
27 H 0.0024 0.0068 0.1190
28 H 0.1327 -0.0025 0.0100
29 H 0.0026 0.0137 -0.0151
30 H -0.0468 -0.0269 -0.0044
31 H 0.0645 0.0219 0.0809
32 H -0.0785 0.0009 0.0490
33 H -0.0063 0.0403 -0.0543
34 H -0.0650 -0.0245 0.0527
35 H -0.0397 -0.0612 -0.0190
36 H 0.0703 -0.0968 0.0284
37 H -0.0355 -0.0692 -0.0109
38 H -0.0776 0.0113 -0.0840
39 H -0.0080 0.0184 0.1018
40 H -0.0169 0.0008 -0.0279
41 H -0.0245 0.0086 0.0199
42 H -0.0351 -0.3847 0.2677
43 H -0.1434 0.1278 -0.5068
44 H 0.0458 0.0421 -0.0280
45 H -0.1251 -0.1377 -0.0022
46 H 0.0549 -0.1085 -0.0051
47 H -0.2208 0.1079 -0.0823
48 H 0.1646 -0.1428 -0.2179
49 H 0.0760 0.0305 -0.0019
Vibrational Mode 52
----------------------------------------------------
Harmonic frequency: 931.63 cm**-1
Reduced mass: 2.2071 amu
Force constant: 1.1286 mdyne/A
IR intensity: 2.9231 km/mol
Normal mode:
X Y Z
1 O 0.0009 -0.0065 0.0052
2 O -0.0119 0.0001 0.0035
3 O 0.1152 0.0533 0.0119
4 C 0.0180 0.0006 -0.0317
5 C 0.0114 -0.0177 -0.0249
6 C -0.0368 -0.0053 -0.0362
7 C 0.0042 -0.0729 0.0434
8 C -0.0044 -0.0136 0.0231
9 C 0.0179 -0.0772 0.0105
10 C 0.0096 0.0026 0.0105
11 C 0.0772 0.0775 -0.0424
12 C 0.0113 -0.0085 -0.0103
13 C -0.0323 0.0599 0.0164
14 C -0.1169 -0.0174 0.0075
15 C -0.0207 0.0399 0.0049
16 C 0.0087 -0.0616 0.0176
17 C 0.0219 0.0347 0.0141
18 C -0.0160 0.0577 -0.0534
19 C -0.0629 -0.0605 0.0201
20 C 0.0045 0.0754 -0.0154
21 C -0.0043 -0.0242 0.0072
22 C 0.0059 -0.0506 0.0105
23 C -0.0400 0.0158 -0.0132
24 H 0.0357 -0.0059 -0.0204
25 H -0.1280 0.0179 -0.1034
26 H 0.0001 0.0486 0.0087
27 H 0.0775 -0.0812 0.1172
28 H -0.0279 0.0623 0.0695
29 H -0.0042 0.0111 -0.0542
30 H -0.0214 -0.2668 -0.0319
31 H 0.0941 0.1678 0.2873
32 H 0.0267 0.0025 -0.0180
33 H 0.0339 -0.0203 -0.0265
34 H 0.0231 -0.0672 0.0069
35 H 0.0369 0.1299 0.0929
36 H -0.1880 -0.0954 0.0110
37 H -0.0473 -0.1404 -0.0032
38 H 0.0272 0.1205 -0.1507
39 H -0.0218 0.1067 0.0055
40 H -0.0287 0.0339 -0.3195
41 H -0.0038 0.1413 0.3088
42 H -0.0203 -0.1236 0.0882
43 H -0.0285 0.0281 -0.1548
44 H -0.0996 0.0611 0.0986
45 H -0.0242 -0.0291 -0.0046
46 H 0.0715 0.0006 -0.0947
47 H 0.3234 -0.0606 0.0873
48 H 0.0550 -0.0479 -0.0669
49 H -0.1005 -0.1953 0.0380
Vibrational Mode 53
----------------------------------------------------
Harmonic frequency: 942.77 cm**-1
Reduced mass: 1.9628 amu
Force constant: 1.0279 mdyne/A
IR intensity: 3.3826 km/mol
Normal mode:
X Y Z
1 O -0.0182 -0.0101 -0.0040
2 O 0.0159 0.0016 -0.0026
3 O 0.0059 0.0018 0.0015
4 C -0.0192 -0.0239 -0.0360
5 C -0.0845 0.0445 -0.0056
6 C 0.0627 0.0657 0.0275
7 C -0.0473 0.0382 0.0124
8 C 0.0624 0.0377 0.0166
9 C 0.0523 -0.0396 -0.0369
10 C 0.0040 0.0083 -0.0015
11 C 0.0207 -0.0165 -0.0331
12 C -0.0733 -0.0369 0.0528
13 C 0.0835 -0.1002 -0.0634
14 C 0.0042 -0.0136 0.0167
15 C -0.0309 0.0664 0.0247
16 C 0.0397 -0.0302 0.0493
17 C -0.0362 -0.0491 -0.0297
18 C -0.0077 0.0251 -0.0108
19 C -0.0036 -0.0006 -0.0070
20 C -0.0041 -0.0128 0.0105
21 C -0.0026 -0.0117 -0.0017
22 C -0.0076 0.0205 -0.0043
23 C 0.0061 -0.0004 0.0013
24 H -0.1334 0.1585 -0.0071
25 H 0.1018 0.2427 0.0659
26 H 0.0477 0.0153 -0.0668
27 H -0.1478 0.0964 0.1123
28 H 0.0721 -0.0688 -0.0532
29 H 0.0459 0.0050 0.1216
30 H 0.0550 -0.1150 -0.0212
31 H 0.0849 -0.0236 0.0560
32 H -0.0861 0.0925 0.0922
33 H -0.0718 -0.0535 0.1575
34 H -0.0413 0.1269 -0.0293
35 H -0.0178 -0.2009 -0.2326
36 H 0.0133 -0.1885 0.0172
37 H 0.0506 -0.2048 0.0228
38 H -0.0055 0.1590 -0.2347
39 H -0.0416 0.1079 0.2398
40 H -0.0741 0.0044 -0.2675
41 H -0.0764 0.0873 0.1551
42 H 0.0407 0.2092 -0.1513
43 H 0.0198 -0.0234 0.2497
44 H -0.0356 0.0049 -0.0068
45 H 0.0036 0.0124 0.0025
46 H 0.0523 -0.0600 -0.0209
47 H -0.0387 0.0192 -0.0191
48 H -0.0938 0.0805 0.1134
49 H -0.0029 -0.0322 0.0040
Vibrational Mode 54
----------------------------------------------------
Harmonic frequency: 949.41 cm**-1
Reduced mass: 1.7155 amu
Force constant: 0.9111 mdyne/A
IR intensity: 9.0019 km/mol
Normal mode:
X Y Z
1 O 0.0006 0.0010 -0.0004
2 O -0.0028 0.0005 0.0016
3 O 0.0171 0.0111 -0.0006
4 C 0.0089 -0.0095 -0.0236
5 C -0.0401 0.0114 -0.0017
6 C -0.0061 0.0060 -0.0138
7 C -0.0325 -0.0581 -0.0191
8 C 0.0190 -0.0128 0.0263
9 C 0.0528 0.0861 -0.0330
10 C -0.0027 0.0031 0.0105
11 C 0.0366 0.0571 -0.0159
12 C -0.0260 -0.0474 -0.0097
13 C 0.0316 0.0297 0.0070
14 C -0.0343 -0.0276 -0.0484
15 C -0.0130 -0.0149 0.0068
16 C 0.0452 0.0935 -0.0004
17 C 0.0042 0.0018 0.0017
18 C 0.0067 -0.0884 0.0808
19 C -0.0125 -0.0113 0.0209
20 C -0.0226 0.0186 -0.0177
21 C 0.0050 0.0119 0.0018
22 C 0.0106 -0.0369 0.0086
23 C -0.0149 0.0016 -0.0043
24 H -0.0501 0.0061 -0.0031
25 H -0.0163 0.0423 -0.0199
26 H -0.0361 0.1935 -0.0892
27 H 0.1160 -0.0705 0.1808
28 H 0.0075 0.0444 0.0617
29 H 0.0201 0.0065 -0.0347
30 H -0.0055 -0.1396 -0.0087
31 H -0.0003 0.1272 0.1543
32 H -0.0080 0.1061 0.0190
33 H 0.0991 -0.1382 0.0380
34 H 0.0007 0.1050 0.0080
35 H 0.0186 0.0119 -0.0664
36 H -0.0666 -0.0855 -0.0406
37 H -0.0353 -0.0536 0.0041
38 H -0.0472 -0.0343 -0.0056
39 H -0.0198 -0.0843 0.3569
40 H -0.0704 -0.0792 0.2520
41 H -0.1300 -0.2053 -0.5364
42 H 0.0027 -0.0070 0.0059
43 H -0.0009 0.0022 -0.0086
44 H -0.0665 -0.1872 -0.0393
45 H -0.0168 -0.0264 -0.0049
46 H -0.2236 0.1455 0.0010
47 H 0.1175 -0.0391 0.0383
48 H 0.0034 -0.0036 -0.0033
49 H -0.0181 -0.0061 0.0061
Vibrational Mode 55
----------------------------------------------------
Harmonic frequency: 968.36 cm**-1
Reduced mass: 1.9913 amu
Force constant: 1.1001 mdyne/A
IR intensity: 12.7951 km/mol
Normal mode:
X Y Z
1 O 0.0064 0.0100 -0.0032
2 O -0.0113 0.0034 0.0084
3 O -0.0401 -0.0067 -0.0071
4 C 0.0071 -0.0085 -0.0769
5 C 0.0239 0.0735 0.0102
6 C -0.0612 0.0452 0.0052
7 C 0.0452 -0.1222 0.0681
8 C 0.0256 -0.0073 0.0302
9 C -0.0451 0.0164 -0.0282
10 C 0.0153 -0.0244 0.0215
11 C -0.0206 -0.0156 -0.0125
12 C 0.0039 -0.0664 0.0737
13 C -0.0349 0.0576 -0.0941
14 C 0.0549 -0.0127 -0.0450
15 C -0.0393 0.0252 0.0455
16 C -0.0465 0.0089 0.0273
17 C 0.0011 0.0143 -0.0207
18 C 0.0064 -0.0105 0.0003
19 C 0.0393 0.0262 0.0038
20 C 0.0036 -0.0259 0.0090
21 C 0.0137 0.0186 -0.0032
22 C -0.0004 -0.0101 0.0021
23 C 0.0030 -0.0042 0.0015
24 H -0.0297 0.2712 0.0064
25 H -0.1737 0.2466 -0.0749
26 H 0.2097 -0.0867 -0.0969
27 H 0.0977 -0.1138 0.1694
28 H -0.0575 0.0321 0.0459
29 H 0.0528 -0.0012 -0.0128
30 H 0.1837 -0.1060 0.0164
31 H -0.0788 0.0091 -0.0399
32 H 0.0542 -0.1588 0.0064
33 H -0.0799 0.0032 0.0501
34 H 0.1208 -0.2153 -0.1250
35 H 0.2129 0.3183 0.0633
36 H 0.0895 -0.0985 -0.0380
37 H 0.2050 -0.1179 0.0575
38 H -0.1563 0.0145 -0.1952
39 H 0.0288 -0.0474 -0.2002
40 H 0.0835 0.0409 0.1880
41 H 0.0927 0.0102 0.1041
42 H -0.0200 0.0025 -0.0065
43 H -0.0086 0.0166 -0.0247
44 H 0.0716 0.0267 -0.0265
45 H 0.0016 0.0159 -0.0071
46 H -0.0109 -0.0134 0.0160
47 H -0.1648 -0.0029 -0.0303
48 H 0.0107 -0.0117 -0.0067
49 H 0.0393 0.1197 -0.0237
Vibrational Mode 56
----------------------------------------------------
Harmonic frequency: 996.12 cm**-1
Reduced mass: 2.0218 amu
Force constant: 1.1820 mdyne/A
IR intensity: 7.4626 km/mol
Normal mode:
X Y Z
1 O -0.0220 -0.0211 0.0041
2 O 0.0182 -0.0031 -0.0049
3 O 0.0255 0.0025 0.0049
4 C -0.0193 -0.0577 -0.0054
5 C 0.1320 0.0464 -0.0551
6 C 0.0889 0.0594 -0.0293
7 C -0.0814 -0.0673 -0.0175
8 C 0.0356 -0.0182 0.0066
9 C 0.0101 0.0054 0.0673
10 C 0.0026 0.0583 0.0286
11 C 0.0045 0.0540 0.0561
12 C -0.0635 -0.0276 0.0227
13 C -0.0239 -0.0105 -0.0008
14 C -0.0069 -0.0128 0.0278
15 C 0.0076 -0.0341 -0.0552
16 C -0.0510 -0.0086 -0.0619
17 C -0.0249 -0.0278 -0.0115
18 C -0.0060 0.0111 -0.0161
19 C -0.0281 -0.0219 -0.0005
20 C -0.0034 0.0102 -0.0078
21 C -0.0150 -0.0197 0.0008
22 C -0.0068 0.0283 -0.0091
23 C 0.0041 0.0034 0.0002
24 H 0.1952 0.0941 -0.0370
25 H 0.1431 0.1042 0.0220
26 H 0.0948 0.1993 -0.2543
27 H -0.0678 0.0134 0.3772
28 H 0.1445 -0.0019 0.0268
29 H -0.0941 0.0208 -0.0211
30 H -0.0544 0.1353 0.0385
31 H 0.0513 0.0080 0.0056
32 H 0.0458 0.0904 -0.0181
33 H -0.1431 -0.0117 0.2550
34 H 0.0671 -0.0027 -0.0515
35 H -0.0765 -0.0607 0.0993
36 H -0.0279 0.2273 0.0211
37 H -0.0617 0.2080 -0.0502
38 H -0.0953 -0.1690 0.1655
39 H 0.0265 -0.0630 -0.2847
40 H 0.0790 0.0224 0.1054
41 H 0.0959 -0.0126 0.0189
42 H 0.0573 0.0811 -0.0766
43 H -0.0189 0.0020 0.1447
44 H -0.0995 -0.0472 0.0086
45 H -0.0703 -0.1196 -0.0054
46 H 0.0497 0.0086 0.0617
47 H 0.0539 0.0268 0.0078
48 H -0.0727 0.0613 0.0679
49 H -0.0322 -0.1172 0.0237
Vibrational Mode 57
----------------------------------------------------
Harmonic frequency: 1000.20 cm**-1
Reduced mass: 1.8326 amu
Force constant: 1.0801 mdyne/A
IR intensity: 11.0213 km/mol
Normal mode:
X Y Z
1 O -0.0128 -0.0127 0.0072
2 O 0.0247 -0.0053 -0.0111
3 O 0.0247 0.0070 0.0028
4 C 0.0046 -0.0676 0.0163
5 C 0.0429 -0.0401 0.0012
6 C 0.0051 -0.0040 0.0220
7 C 0.0217 0.0672 0.0118
8 C 0.0072 0.0138 -0.0443
9 C -0.0298 0.0101 -0.0569
10 C -0.0035 0.0477 0.0093
11 C 0.0713 0.0341 -0.0070
12 C 0.0610 0.0767 0.0153
13 C -0.0584 -0.0451 -0.0147
14 C 0.0018 -0.0861 -0.0221
15 C -0.0605 0.0671 -0.0109
16 C -0.0429 0.0612 0.0473
17 C -0.0271 -0.0210 0.0014
18 C 0.0041 -0.0141 0.0195
19 C -0.0257 -0.0213 0.0032
20 C 0.0028 0.0232 -0.0059
21 C -0.0103 -0.0081 0.0036
22 C -0.0035 0.0141 -0.0048
23 C -0.0023 0.0032 -0.0016
24 H -0.0321 -0.1047 -0.0120
25 H 0.1135 -0.1080 0.0999
26 H 0.0060 -0.1361 0.0871
27 H -0.2023 0.1284 -0.0704
28 H 0.1375 -0.0752 -0.0890
29 H -0.1428 0.0220 0.0340
30 H -0.0022 -0.0856 -0.0076
31 H 0.2615 -0.0233 0.1610
32 H -0.0078 -0.2115 -0.0164
33 H -0.1372 0.2266 -0.1061
34 H -0.0185 -0.1919 -0.0057
35 H -0.0302 -0.0090 0.1216
36 H 0.0204 -0.2609 -0.0175
37 H -0.0345 -0.1561 -0.0137
38 H -0.1573 0.0497 -0.1989
39 H 0.0487 -0.1158 -0.1591
40 H 0.0924 0.0205 0.4082
41 H 0.0864 -0.0846 -0.0951
42 H 0.0484 0.0444 -0.0476
43 H -0.0287 0.0046 0.1110
44 H -0.0156 -0.0350 0.0005
45 H -0.0191 -0.0372 0.0073
46 H -0.0395 0.0259 -0.0538
47 H 0.0594 0.0126 0.0144
48 H -0.0579 0.0524 0.0526
49 H -0.0334 -0.1012 0.0188
Vibrational Mode 58
----------------------------------------------------
Harmonic frequency: 1023.95 cm**-1
Reduced mass: 1.7049 amu
Force constant: 1.0532 mdyne/A
IR intensity: 6.3203 km/mol
Normal mode:
X Y Z
1 O -0.0096 -0.0149 0.0053
2 O 0.0054 -0.0004 -0.0042
3 O 0.0200 -0.0104 0.0070
4 C -0.0851 -0.0113 0.0336
5 C -0.0523 0.0109 -0.0574
6 C -0.0525 0.0237 0.0223
7 C 0.0795 -0.0237 -0.0252
8 C 0.0312 0.0183 0.0117
9 C 0.0368 -0.0022 -0.0272
10 C 0.0179 0.0114 -0.0145
11 C -0.0318 0.0014 0.0297
12 C 0.0420 0.0074 0.0635
13 C -0.0328 -0.0021 -0.0747
14 C -0.0144 0.0143 0.0636
15 C 0.0665 -0.0236 -0.0418
16 C 0.0433 0.0057 0.0110
17 C -0.0035 -0.0005 0.0068
18 C -0.0020 -0.0050 0.0144
19 C -0.0283 -0.0211 -0.0150
20 C -0.0057 0.0144 -0.0045
21 C -0.0205 -0.0200 0.0022
22 C -0.0038 0.0356 -0.0081
23 C -0.0051 0.0064 -0.0022
24 H -0.1388 0.0263 -0.0613
25 H -0.0714 0.1032 0.0034
26 H 0.3313 -0.2412 -0.1959
27 H 0.0243 -0.0145 -0.0747
28 H 0.1815 -0.0478 -0.0184
29 H -0.0446 0.0231 0.0422
30 H -0.1908 0.1788 -0.0010
31 H 0.0522 -0.0681 -0.0436
32 H -0.0810 -0.1874 0.0844
33 H -0.1182 0.1450 -0.0818
34 H -0.0321 -0.2420 -0.0212
35 H 0.1783 0.2202 -0.0176
36 H -0.0033 0.0773 0.0520
37 H -0.1213 0.1645 -0.0474
38 H 0.1752 -0.0290 0.2349
39 H -0.0266 0.0015 0.2625
40 H -0.0703 -0.0416 -0.0774
41 H -0.0868 -0.0247 -0.1064
42 H 0.0182 -0.0082 0.0020
43 H -0.0430 0.0225 0.0096
44 H -0.0815 -0.0670 0.0065
45 H 0.0230 0.0613 0.0067
46 H -0.0056 0.0048 -0.0220
47 H 0.1996 0.0282 0.0339
48 H -0.0179 0.0160 0.0168
49 H -0.0575 -0.1711 0.0287
Vibrational Mode 59
----------------------------------------------------
Harmonic frequency: 1030.90 cm**-1
Reduced mass: 2.1268 amu
Force constant: 1.3317 mdyne/A
IR intensity: 27.2999 km/mol
Normal mode:
X Y Z
1 O -0.0225 -0.0253 0.0242
2 O 0.0738 -0.0184 -0.0437
3 O -0.0117 0.0103 -0.0047
4 C 0.0929 -0.0399 0.0334
5 C -0.0435 0.0543 0.0122
6 C -0.0808 -0.0165 0.0028
7 C 0.0658 -0.0295 -0.0158
8 C -0.0097 -0.0626 -0.0757
9 C -0.0119 0.0020 0.0170
10 C -0.0320 0.1548 0.0090
11 C 0.0322 0.0198 0.0520
12 C -0.0004 -0.0294 -0.0019
13 C 0.0329 0.0286 -0.0224
14 C -0.0075 0.0055 0.0074
15 C -0.0584 -0.0089 -0.0340
16 C 0.0243 -0.0256 -0.0080
17 C -0.0556 -0.0375 0.0674
18 C -0.0005 0.0065 -0.0081
19 C 0.0241 0.0106 -0.0012
20 C -0.0003 -0.0119 0.0035
21 C 0.0126 0.0136 -0.0014
22 C 0.0013 -0.0257 0.0069
23 C 0.0061 -0.0055 0.0030
24 H -0.0076 0.1551 0.0189
25 H -0.0805 -0.0511 -0.0061
26 H -0.2063 0.0568 0.2087
27 H 0.2354 -0.1287 -0.1934
28 H 0.3353 -0.0091 -0.0093
29 H -0.3725 0.0597 -0.1802
30 H -0.0099 0.0813 0.0398
31 H 0.0262 0.0076 0.0083
32 H -0.0127 0.0414 0.0218
33 H 0.0703 -0.0726 -0.0047
34 H 0.0023 0.0701 -0.0142
35 H 0.1301 0.1254 -0.1371
36 H 0.0106 0.0553 0.0047
37 H -0.0880 0.0144 -0.0335
38 H -0.1331 -0.0646 -0.0211
39 H -0.0193 0.0692 0.0759
40 H -0.0451 -0.0075 -0.1847
41 H -0.0432 0.0437 0.0548
42 H 0.1271 -0.0104 -0.0094
43 H -0.1279 0.0332 0.1735
44 H 0.0496 0.0421 -0.0109
45 H -0.1345 -0.2229 -0.0042
46 H -0.0000 -0.0054 0.0151
47 H -0.1687 -0.0206 -0.0322
48 H -0.1117 0.1096 0.0806
49 H 0.0456 0.1251 -0.0227
Vibrational Mode 60
----------------------------------------------------
Harmonic frequency: 1043.36 cm**-1
Reduced mass: 2.0260 amu
Force constant: 1.2994 mdyne/A
IR intensity: 11.5665 km/mol
Normal mode:
X Y Z
1 O 0.0261 0.0291 -0.0102
2 O -0.0217 0.0038 0.0094
3 O 0.0359 0.0008 0.0073
4 C 0.0550 0.0155 0.0506
5 C -0.0159 0.0525 0.0264
6 C -0.0298 -0.0137 0.0113
7 C 0.0128 -0.0229 -0.0320
8 C -0.0448 0.0165 -0.0336
9 C 0.0158 -0.0188 -0.0207
10 C -0.0587 -0.0524 -0.0187
11 C 0.0205 -0.0621 0.0648
12 C -0.0590 -0.0415 0.0126
13 C 0.0724 0.0455 -0.0173
14 C 0.0479 -0.0776 0.0600
15 C -0.0364 0.0758 -0.0693
16 C -0.0348 0.0205 0.0105
17 C 0.0324 0.0084 0.0139
18 C -0.0041 0.0016 0.0056
19 C -0.0300 -0.0523 -0.0178
20 C -0.0005 0.0308 -0.0095
21 C -0.0468 -0.0372 0.0023
22 C -0.0171 0.0808 -0.0212
23 C -0.0003 0.0082 -0.0016
24 H -0.0935 0.1191 0.0174
25 H 0.0095 0.0218 0.0397
26 H -0.1264 0.1001 0.0617
27 H 0.2254 -0.1209 -0.1265
28 H -0.1563 -0.0166 -0.0627
29 H 0.0941 -0.0330 0.0248
30 H -0.0011 0.2129 0.0416
31 H 0.2468 -0.2349 -0.1018
32 H 0.0108 0.1070 0.0060
33 H 0.0247 -0.1102 0.1090
34 H 0.0779 0.2077 -0.0549
35 H 0.0799 0.0493 -0.1486
36 H 0.1565 -0.0894 0.0470
37 H -0.0286 0.0604 -0.0624
38 H -0.0374 0.0626 -0.0600
39 H 0.0242 -0.0435 -0.1487
40 H 0.0644 0.0197 0.1973
41 H 0.0677 -0.0260 0.0009
42 H -0.0852 -0.0461 0.0683
43 H 0.1416 -0.0814 -0.1246
44 H -0.1170 -0.0713 0.0237
45 H 0.0390 0.0706 -0.0087
46 H 0.0762 -0.0199 -0.0108
47 H 0.1217 0.0764 0.0096
48 H 0.1142 -0.0929 -0.1025
49 H -0.1197 -0.3933 0.0666
Vibrational Mode 61
----------------------------------------------------
Harmonic frequency: 1048.28 cm**-1
Reduced mass: 1.9102 amu
Force constant: 1.2367 mdyne/A
IR intensity: 6.4515 km/mol
Normal mode:
X Y Z
1 O -0.0299 -0.0492 0.0078
2 O -0.0103 0.0051 0.0011
3 O 0.0190 0.0126 0.0008
4 C -0.0398 -0.0217 -0.0867
5 C -0.0165 -0.0219 0.0294
6 C -0.0568 0.0144 0.0092
7 C 0.0633 0.0355 0.0324
8 C -0.0114 0.0137 0.0945
9 C -0.0059 -0.0201 0.1006
10 C 0.0956 0.0205 -0.0659
11 C 0.0122 0.0022 -0.0031
12 C -0.0213 0.0302 -0.0471
13 C 0.0420 -0.0405 0.0415
14 C 0.0353 -0.0366 -0.0015
15 C -0.0219 0.0149 -0.0016
16 C -0.0069 0.0242 -0.0684
17 C 0.0114 -0.0052 0.0019
18 C -0.0047 0.0193 -0.0127
19 C -0.0127 -0.0245 0.0058
20 C 0.0082 0.0199 -0.0082
21 C -0.0248 -0.0204 -0.0017
22 C -0.0074 0.0320 -0.0105
23 C 0.0069 -0.0011 0.0015
24 H 0.1671 0.0384 0.0504
25 H -0.4063 0.1432 -0.2599
26 H 0.1232 -0.2334 0.0506
27 H -0.0108 0.0163 -0.1867
28 H 0.0666 0.0412 0.1143
29 H -0.1501 0.0592 0.0388
30 H 0.2013 -0.1097 0.0241
31 H 0.0316 -0.0100 -0.0026
32 H -0.0427 0.1322 -0.0003
33 H 0.0500 -0.0332 0.0107
34 H -0.1092 0.1278 0.0901
35 H -0.0319 -0.1179 -0.1498
36 H 0.0105 0.1939 -0.0027
37 H 0.2051 0.0935 0.0184
38 H -0.2351 -0.1220 -0.0456
39 H 0.0070 -0.0695 -0.0433
40 H -0.0050 -0.0339 0.0962
41 H -0.0111 -0.0771 -0.2149
42 H 0.0215 0.0151 -0.0085
43 H -0.0796 0.0486 0.0173
44 H -0.0492 -0.0050 0.0058
45 H 0.0600 0.1340 0.0189
46 H 0.1249 -0.0325 0.0360
47 H -0.0342 0.0342 -0.0093
48 H -0.0517 0.0357 0.0514
49 H -0.0484 -0.1840 0.0361
Vibrational Mode 62
----------------------------------------------------
Harmonic frequency: 1063.16 cm**-1
Reduced mass: 1.8032 amu
Force constant: 1.2009 mdyne/A
IR intensity: 22.3545 km/mol
Normal mode:
X Y Z
1 O 0.0190 0.0285 -0.0106
2 O -0.0376 0.0088 0.0269
3 O 0.0143 0.0748 -0.0151
4 C 0.0091 -0.0343 -0.0158
5 C -0.0313 -0.0161 -0.0401
6 C 0.0013 0.0002 0.0027
7 C 0.0029 0.0024 0.0177
8 C 0.0086 0.0536 -0.0171
9 C -0.0072 -0.0088 0.0464
10 C -0.0339 -0.0630 0.0258
11 C 0.0934 0.0141 0.0719
12 C 0.0188 0.0172 0.0268
13 C -0.0142 -0.0013 -0.0232
14 C -0.0495 -0.0074 0.0152
15 C -0.0791 -0.0242 -0.0699
16 C 0.0390 -0.0028 -0.0275
17 C 0.0339 0.0038 -0.0378
18 C -0.0018 0.0145 -0.0068
19 C 0.0138 -0.0172 0.0108
20 C 0.0035 0.0084 -0.0023
21 C -0.0003 -0.0028 -0.0008
22 C -0.0022 -0.0581 0.0125
23 C 0.0454 -0.0292 0.0175
24 H -0.0220 0.0248 -0.0328
25 H 0.1055 0.0269 0.0859
26 H 0.0098 -0.0317 0.0222
27 H -0.0622 0.0316 0.0508
28 H -0.1323 -0.0641 -0.1036
29 H 0.1038 -0.0192 0.1306
30 H 0.1480 0.0447 0.0696
31 H 0.2290 -0.0787 0.0353
32 H -0.0551 -0.0714 0.0500
33 H -0.0124 0.0589 -0.0872
34 H -0.0374 -0.1148 0.0158
35 H 0.0328 0.0496 0.0258
36 H -0.0827 0.2509 0.0095
37 H -0.0886 0.1101 -0.0618
38 H -0.2746 -0.1885 0.0229
39 H -0.0208 0.0190 0.1658
40 H -0.0704 -0.0406 -0.1277
41 H -0.0771 -0.0191 -0.1124
42 H -0.0556 0.0165 -0.0083
43 H 0.1483 -0.0701 -0.0638
44 H -0.0221 0.0020 -0.0007
45 H 0.0342 0.0546 -0.0046
46 H 0.0614 -0.0206 0.0107
47 H -0.5707 -0.0435 -0.1128
48 H 0.0781 -0.0718 -0.0575
49 H 0.1028 0.1623 -0.0159
Vibrational Mode 63
----------------------------------------------------
Harmonic frequency: 1066.85 cm**-1
Reduced mass: 1.7578 amu
Force constant: 1.1788 mdyne/A
IR intensity: 36.2387 km/mol
Normal mode:
X Y Z
1 O -0.0111 -0.0378 -0.0031
2 O -0.0300 0.0079 0.0088
3 O -0.0058 0.0215 -0.0067
4 C -0.0380 -0.0256 0.0716
5 C 0.0617 0.0721 0.0126
6 C -0.0115 0.0181 0.0298
7 C 0.0182 0.0101 -0.0468
8 C -0.0669 0.0126 0.0516
9 C 0.0124 0.0381 -0.0337
10 C 0.0163 0.0026 -0.1174
11 C 0.0310 0.0121 0.0019
12 C -0.0230 -0.0325 -0.0273
13 C 0.0260 -0.0077 0.0277
14 C -0.0530 -0.0187 -0.0256
15 C 0.0082 0.0005 -0.0029
16 C -0.0273 -0.0175 0.0156
17 C 0.0453 -0.0116 0.0279
18 C 0.0038 -0.0119 0.0019
19 C 0.0083 0.0180 0.0109
20 C -0.0085 -0.0188 0.0064
21 C 0.0293 0.0175 0.0017
22 C 0.0037 -0.0590 0.0146
23 C 0.0133 -0.0108 0.0056
24 H 0.0569 -0.0001 0.0108
25 H -0.0894 -0.0534 -0.0398
26 H 0.2455 -0.1197 -0.2172
27 H -0.0451 0.0353 -0.0243
28 H 0.1745 -0.0025 0.0610
29 H -0.2083 0.0542 0.0119
30 H -0.2207 0.0735 -0.0292
31 H 0.2203 -0.0692 0.0882
32 H 0.0680 0.0945 -0.0524
33 H -0.0682 -0.0304 0.1582
34 H 0.0345 0.1534 -0.0112
35 H 0.0324 -0.0044 -0.1062
36 H -0.0985 -0.2429 -0.0161
37 H -0.2147 -0.1204 -0.0233
38 H 0.1154 0.0696 0.0128
39 H 0.0027 0.0016 -0.0988
40 H 0.0422 0.0356 0.0013
41 H 0.0510 0.0487 0.1516
42 H -0.0742 -0.0345 0.0694
43 H 0.1325 -0.0837 -0.0997
44 H 0.0203 -0.0017 -0.0022
45 H 0.1381 0.2644 0.0201
46 H -0.1114 0.0418 -0.0020
47 H -0.2309 -0.0541 -0.0415
48 H 0.0785 -0.0689 -0.0683
49 H 0.1040 0.2914 -0.0480
Vibrational Mode 64
----------------------------------------------------
Harmonic frequency: 1078.75 cm**-1
Reduced mass: 1.9201 amu
Force constant: 1.3165 mdyne/A
IR intensity: 68.6185 km/mol
Normal mode:
X Y Z
1 O -0.0004 0.0015 0.0035
2 O 0.0578 -0.0136 -0.0405
3 O 0.0315 0.1306 -0.0260
4 C -0.0217 0.0514 0.0199
5 C 0.0008 -0.0171 0.0065
6 C 0.0055 0.0027 -0.0077
7 C -0.0049 -0.0089 -0.0117
8 C 0.0125 -0.0304 -0.0031
9 C 0.0249 -0.0241 -0.0230
10 C 0.0037 0.0112 0.0086
11 C -0.0561 -0.0214 -0.0475
12 C -0.0066 -0.0006 -0.0011
13 C 0.0003 0.0045 0.0021
14 C -0.0201 -0.0055 -0.0033
15 C 0.0713 0.0281 0.0329
16 C -0.0230 0.0235 0.0120
17 C -0.0638 0.0205 0.0477
18 C 0.0003 -0.0081 0.0085
19 C -0.0062 -0.0552 0.0180
20 C 0.0044 0.0398 -0.0095
21 C -0.0548 -0.0453 -0.0012
22 C -0.0023 -0.0311 0.0067
23 C 0.0776 -0.0586 0.0317
24 H -0.0520 -0.0964 -0.0031
25 H -0.0322 0.0201 -0.0350
26 H 0.0321 0.0181 -0.0537
27 H 0.0537 -0.0298 -0.0175
28 H -0.0394 0.0455 0.0413
29 H 0.1324 -0.0335 -0.0680
30 H -0.0944 -0.0130 -0.0477
31 H -0.1374 0.0319 -0.0388
32 H -0.0017 0.0090 -0.0011
33 H -0.0234 0.0010 0.0441
34 H 0.0093 0.0134 -0.0054
35 H -0.0073 -0.0039 0.0042
36 H -0.0417 -0.0834 0.0002
37 H 0.0727 0.0059 0.0304
38 H 0.2533 0.1577 0.0206
39 H 0.0181 -0.0503 -0.0783
40 H 0.0399 0.0086 0.1650
41 H 0.0430 -0.0333 -0.0332
42 H 0.0209 -0.0278 0.0293
43 H -0.1909 0.0943 0.0351
44 H -0.1816 -0.1205 0.0505
45 H -0.0406 -0.0742 -0.0045
46 H 0.1586 -0.0583 -0.0073
47 H -0.6896 -0.0168 -0.1493
48 H -0.0471 0.0577 0.0288
49 H 0.0281 -0.2199 0.0637
Vibrational Mode 65
----------------------------------------------------
Harmonic frequency: 1085.88 cm**-1
Reduced mass: 1.5010 amu
Force constant: 1.0428 mdyne/A
IR intensity: 3.9098 km/mol
Normal mode:
X Y Z
1 O -0.0006 0.0161 0.0021
2 O 0.0304 -0.0066 -0.0172
3 O -0.0046 -0.0224 0.0047
4 C 0.0442 0.0310 0.0300
5 C 0.0289 -0.0580 0.0164
6 C -0.0243 0.0608 -0.0173
7 C 0.0296 -0.0230 -0.0201
8 C -0.0118 -0.0033 -0.0375
9 C 0.0182 0.0200 -0.0361
10 C 0.0400 -0.0191 0.0628
11 C 0.0443 -0.0113 0.0479
12 C -0.0343 0.0374 -0.0145
13 C 0.0078 -0.0407 0.0255
14 C -0.0236 0.0008 0.0042
15 C -0.0402 -0.0070 -0.0400
16 C -0.0126 -0.0079 0.0159
17 C -0.0421 0.0195 -0.0067
18 C 0.0024 -0.0047 0.0043
19 C -0.0002 0.0119 -0.0041
20 C -0.0033 -0.0147 0.0051
21 C 0.0254 0.0137 0.0033
22 C -0.0028 -0.0115 0.0025
23 C -0.0115 0.0127 -0.0055
24 H -0.0437 -0.2462 0.0010
25 H -0.3145 0.3307 -0.2268
26 H 0.2213 -0.0852 -0.1788
27 H 0.1073 -0.0562 -0.0586
28 H -0.4012 0.0615 -0.0271
29 H 0.2124 -0.0632 0.0042
30 H -0.0289 0.1134 0.0282
31 H 0.1314 -0.0772 -0.0076
32 H -0.0562 0.0870 0.0210
33 H -0.0173 0.0086 0.0515
34 H -0.1137 -0.0030 0.0865
35 H -0.0770 -0.1244 -0.0301
36 H -0.0011 -0.0536 0.0021
37 H -0.1772 -0.0324 -0.0480
38 H 0.0187 0.0125 0.0224
39 H 0.0029 -0.0127 -0.0316
40 H 0.0218 0.0174 0.0095
41 H 0.0256 0.0250 0.0829
42 H 0.0398 0.0241 -0.0340
43 H -0.1892 0.1149 0.0628
44 H 0.0201 0.0056 0.0021
45 H -0.1053 -0.1914 -0.0157
46 H -0.0987 0.0345 -0.0186
47 H 0.0524 -0.0131 0.0128
48 H -0.0742 0.0673 0.0628
49 H 0.0400 0.1824 -0.0373
Vibrational Mode 66
----------------------------------------------------
Harmonic frequency: 1089.81 cm**-1
Reduced mass: 1.9983 amu
Force constant: 1.3983 mdyne/A
IR intensity: 28.2245 km/mol
Normal mode:
X Y Z
1 O -0.0363 -0.0639 -0.0019
2 O -0.0051 0.0015 0.0048
3 O 0.0029 0.0071 -0.0010
4 C 0.0241 0.0049 -0.0124
5 C -0.0185 0.0312 0.0086
6 C 0.0393 -0.0670 0.0160
7 C -0.0730 0.0135 -0.0221
8 C -0.0605 0.0201 0.0464
9 C -0.0093 -0.0029 -0.0369
10 C 0.2087 -0.0212 -0.0057
11 C 0.0136 -0.0115 0.0444
12 C 0.0367 -0.0281 0.0153
13 C -0.0234 0.0470 -0.0329
14 C -0.0122 -0.0096 0.0266
15 C -0.0076 0.0067 -0.0389
16 C 0.0103 -0.0031 0.0216
17 C -0.0043 0.0189 -0.0643
18 C 0.0017 -0.0019 0.0053
19 C 0.0009 -0.0076 -0.0067
20 C -0.0019 0.0030 -0.0004
21 C -0.0031 0.0003 0.0011
22 C -0.0006 -0.0028 0.0012
23 C 0.0044 -0.0021 0.0019
24 H -0.0949 0.1412 -0.0001
25 H 0.0276 -0.1913 -0.0031
26 H -0.2274 0.2059 0.0671
27 H 0.0339 -0.0163 0.0262
28 H -0.3471 0.0851 0.0615
29 H -0.1609 0.0400 0.0517
30 H -0.0350 0.1325 0.0262
31 H 0.0799 -0.0744 -0.0445
32 H 0.0593 -0.1008 -0.0241
33 H 0.0644 -0.0304 -0.0663
34 H 0.1371 -0.0399 -0.1058
35 H 0.0186 0.0855 0.1144
36 H 0.0347 -0.0444 0.0207
37 H -0.0934 0.0374 -0.0412
38 H 0.0308 0.0143 0.0231
39 H -0.0058 0.0351 0.0452
40 H -0.0100 0.0023 -0.0306
41 H -0.0115 0.0125 0.0332
42 H 0.1267 0.0385 -0.1016
43 H -0.3687 0.2472 0.0961
44 H 0.0357 0.0170 -0.0098
45 H 0.1397 0.2536 0.0310
46 H -0.0412 0.0168 -0.0226
47 H -0.0455 -0.0022 -0.0108
48 H -0.2313 0.1664 0.2110
49 H 0.0035 -0.0068 0.0011
Vibrational Mode 67
----------------------------------------------------
Harmonic frequency: 1090.06 cm**-1
Reduced mass: 3.0670 amu
Force constant: 2.1471 mdyne/A
IR intensity: 41.8326 km/mol
Normal mode:
X Y Z
1 O 0.0241 0.0053 -0.0154
2 O 0.1653 -0.0345 -0.1313
3 O -0.0052 -0.0364 0.0081
4 C -0.0488 -0.0035 -0.0217
5 C -0.0031 0.0380 -0.0098
6 C 0.0225 -0.0354 0.0189
7 C -0.0335 0.0240 0.0223
8 C 0.0064 0.0148 0.0574
9 C -0.0092 0.0063 0.0352
10 C -0.0467 -0.0760 -0.0773
11 C 0.0451 -0.0136 0.0125
12 C 0.0121 -0.0208 -0.0053
13 C -0.0017 0.0199 -0.0028
14 C 0.0062 -0.0157 0.0010
15 C -0.0402 -0.0061 -0.0199
16 C 0.0089 -0.0065 -0.0210
17 C -0.1991 0.0855 0.1972
18 C -0.0019 0.0080 -0.0074
19 C 0.0025 0.0148 -0.0027
20 C -0.0020 -0.0118 0.0022
21 C 0.0176 0.0143 -0.0001
22 C -0.0013 0.0064 -0.0021
23 C -0.0220 0.0182 -0.0094
24 H 0.0399 0.0955 -0.0008
25 H 0.1551 -0.1759 0.1183
26 H 0.1134 -0.0683 -0.0843
27 H -0.1920 0.1025 0.1324
28 H -0.1186 0.0245 0.0503
29 H 0.3177 -0.0566 0.0485
30 H 0.1393 -0.0456 0.0237
31 H 0.1719 -0.0829 0.0247
32 H -0.0089 0.0162 0.0172
33 H 0.0500 -0.0462 0.0010
34 H 0.0356 0.0055 -0.0204
35 H 0.0643 0.0839 -0.0163
36 H -0.0051 0.0855 -0.0002
37 H 0.0113 0.0152 -0.0138
38 H -0.1715 -0.0984 -0.0240
39 H -0.0097 0.0069 0.0384
40 H -0.0208 -0.0116 -0.0589
41 H -0.0228 0.0007 -0.0268
42 H -0.1937 -0.0972 0.2182
43 H -0.3100 0.1124 -0.0909
44 H 0.0581 0.0467 -0.0176
45 H 0.1607 0.3019 0.0062
46 H -0.0339 0.0148 0.0134
47 H 0.1577 0.0038 0.0356
48 H 0.1179 -0.0150 -0.1380
49 H 0.0023 0.0978 -0.0243
Vibrational Mode 68
----------------------------------------------------
Harmonic frequency: 1113.32 cm**-1
Reduced mass: 1.9475 amu
Force constant: 1.4222 mdyne/A
IR intensity: 11.4235 km/mol
Normal mode:
X Y Z
1 O -0.0048 -0.0328 -0.0147
2 O 0.0085 0.0018 -0.0150
3 O -0.0110 0.0053 -0.0037
4 C 0.1238 -0.0366 0.0347
5 C 0.0080 -0.0978 -0.0204
6 C -0.0228 0.0387 0.0579
7 C -0.0016 -0.0168 -0.0379
8 C -0.0624 0.0042 -0.0018
9 C 0.0515 0.0146 -0.0418
10 C 0.0244 -0.0195 -0.0633
11 C 0.0131 0.0436 -0.0099
12 C -0.0430 0.0594 0.0691
13 C 0.0252 -0.0122 -0.0470
14 C 0.0194 0.0974 -0.0161
15 C -0.0383 -0.0578 0.0316
16 C -0.0138 -0.0108 0.0203
17 C -0.0156 0.0062 0.0354
18 C 0.0017 -0.0171 0.0091
19 C 0.0048 -0.0045 0.0013
20 C 0.0009 -0.0007 0.0017
21 C -0.0085 -0.0130 0.0000
22 C 0.0026 0.0152 -0.0029
23 C 0.0053 -0.0044 0.0021
24 H -0.1938 -0.2357 -0.0463
25 H 0.0799 0.2104 0.1379
26 H -0.0713 0.0918 -0.0007
27 H 0.0675 -0.0319 0.0141
28 H -0.0742 0.0022 -0.0060
29 H -0.2432 0.0458 -0.0093
30 H 0.1022 -0.1299 0.0127
31 H -0.3193 0.2403 0.0399
32 H -0.0611 -0.0392 0.0532
33 H -0.2109 0.1658 0.0978
34 H 0.0591 0.0452 -0.0713
35 H 0.0070 -0.0210 -0.0204
36 H 0.0288 0.2659 -0.0189
37 H 0.0196 -0.0332 0.0330
38 H -0.1149 -0.0920 -0.0082
39 H 0.0088 0.0119 -0.0717
40 H 0.0365 0.0251 0.0128
41 H 0.0425 0.0242 0.0992
42 H -0.1117 0.0227 0.0496
43 H 0.0460 -0.0401 -0.0533
44 H -0.1565 -0.1176 0.0465
45 H 0.1763 0.3506 0.0107
46 H 0.0410 -0.0262 0.0042
47 H 0.0248 0.0152 0.0027
48 H 0.0844 -0.0548 -0.0722
49 H -0.0379 -0.1451 0.0279
Vibrational Mode 69
----------------------------------------------------
Harmonic frequency: 1116.48 cm**-1
Reduced mass: 1.6241 amu
Force constant: 1.1928 mdyne/A
IR intensity: 3.3589 km/mol
Normal mode:
X Y Z
1 O -0.0006 -0.0069 -0.0028
2 O 0.0035 -0.0007 -0.0037
3 O 0.0353 -0.0529 0.0217
4 C -0.0269 0.0189 -0.0136
5 C 0.0113 -0.0659 -0.0287
6 C 0.0040 -0.0044 0.0130
7 C 0.0021 -0.0087 -0.0095
8 C 0.0080 -0.0023 0.0073
9 C 0.0164 -0.1039 0.0364
10 C 0.0013 -0.0044 -0.0075
11 C -0.0254 -0.0023 -0.0109
12 C -0.0413 0.0283 0.0606
13 C 0.0230 0.0244 -0.0424
14 C -0.0421 -0.0456 0.0045
15 C 0.0343 0.0395 0.0004
16 C -0.0064 0.0511 -0.0111
17 C -0.0021 0.0029 0.0068
18 C -0.0233 0.0534 -0.0176
19 C -0.0180 0.0290 0.0054
20 C 0.0096 -0.0073 0.0027
21 C 0.0451 0.0088 0.0011
22 C -0.0204 -0.0260 0.0002
23 C -0.0287 0.0394 -0.0166
24 H 0.0150 0.0430 -0.0214
25 H 0.0438 0.1454 0.0486
26 H -0.2021 0.1140 0.1424
27 H 0.2173 -0.1170 -0.1522
28 H 0.0695 -0.0040 0.0110
29 H 0.0219 -0.0020 -0.0040
30 H -0.0803 0.0383 -0.0192
31 H 0.0657 -0.0514 -0.0115
32 H -0.0284 -0.0365 0.0318
33 H -0.1246 0.0804 0.0779
34 H 0.1003 0.0614 -0.0907
35 H -0.0204 -0.0146 0.0343
36 H -0.1916 0.0029 0.0083
37 H 0.0473 0.0367 0.0022
38 H 0.1105 0.0919 -0.0025
39 H 0.0167 -0.0727 -0.0068
40 H 0.0099 -0.0225 0.2079
41 H 0.0063 -0.0972 -0.2125
42 H -0.0027 -0.0046 0.0081
43 H -0.0218 0.0112 -0.0084
44 H -0.1653 -0.0377 0.0228
45 H 0.0341 0.0789 -0.0013
46 H 0.2678 -0.1495 0.0296
47 H 0.0144 -0.0265 0.0084
48 H -0.0008 0.0022 0.0018
49 H 0.1388 0.5942 -0.1129
Vibrational Mode 70
----------------------------------------------------
Harmonic frequency: 1140.80 cm**-1
Reduced mass: 1.3799 amu
Force constant: 1.0581 mdyne/A
IR intensity: 0.8962 km/mol
Normal mode:
X Y Z
1 O -0.0032 -0.0083 -0.0048
2 O 0.0111 0.0026 -0.0125
3 O -0.0390 0.0425 -0.0193
4 C -0.0099 0.0110 -0.0474
5 C 0.0378 -0.0536 -0.0067
6 C 0.0331 0.0203 0.0277
7 C -0.0110 -0.0032 -0.0002
8 C 0.0081 -0.0089 0.0211
9 C -0.0752 -0.0058 0.0018
10 C 0.0006 0.0079 -0.0162
11 C -0.0074 0.0036 0.0242
12 C -0.0023 0.0157 0.0416
13 C -0.0208 -0.0028 -0.0150
14 C -0.0116 -0.0141 -0.0015
15 C 0.0137 0.0073 -0.0146
16 C 0.0304 0.0023 0.0042
17 C -0.0367 -0.0024 0.0264
18 C 0.0145 0.0006 0.0011
19 C 0.0118 0.0207 -0.0035
20 C 0.0037 -0.0072 0.0031
21 C -0.0081 0.0226 -0.0044
22 C 0.0239 -0.0173 0.0094
23 C 0.0197 -0.0476 0.0163
24 H 0.1247 0.1451 0.0088
25 H -0.0913 0.3721 -0.0500
26 H -0.1217 0.0864 0.0759
27 H 0.2223 -0.1162 -0.1411
28 H 0.0848 0.0080 0.0365
29 H -0.0341 0.0129 -0.0178
30 H -0.0945 0.1032 0.0044
31 H 0.0978 -0.0687 -0.0204
32 H 0.0737 -0.1002 -0.0428
33 H 0.0258 0.0140 -0.0641
34 H 0.1423 0.0292 -0.1103
35 H -0.2483 -0.2268 0.2542
36 H -0.0114 -0.1505 0.0010
37 H -0.0964 -0.0208 -0.0219
38 H 0.0568 0.0266 0.0202
39 H -0.0154 0.0328 0.1291
40 H -0.0523 -0.0236 -0.0782
41 H -0.0613 -0.0053 -0.0560
42 H -0.1271 0.0562 0.0259
43 H 0.1479 -0.1034 -0.0100
44 H 0.2902 0.1652 -0.0888
45 H 0.0241 0.0811 -0.0083
46 H -0.1495 0.0706 -0.0345
47 H 0.0601 -0.0199 0.0167
48 H 0.1226 -0.0801 -0.1044
49 H -0.0479 -0.2675 0.0562
Vibrational Mode 71
----------------------------------------------------
Harmonic frequency: 1144.20 cm**-1
Reduced mass: 1.7485 amu
Force constant: 1.3487 mdyne/A
IR intensity: 8.2571 km/mol
Normal mode:
X Y Z
1 O 0.0047 0.0036 -0.0033
2 O -0.0024 -0.0038 0.0049
3 O -0.0649 0.0398 -0.0241
4 C -0.0152 -0.0115 0.0520
5 C 0.0151 0.0044 -0.0311
6 C -0.0185 0.0019 -0.0146
7 C 0.0131 -0.0120 -0.0194
8 C -0.0107 0.0174 -0.0230
9 C -0.0263 -0.0300 0.0962
10 C 0.0044 -0.0361 0.0239
11 C 0.0120 0.0030 -0.0102
12 C -0.0298 0.0073 0.0118
13 C 0.0186 0.0110 -0.0122
14 C 0.0267 0.0303 -0.0872
15 C -0.0171 -0.0147 0.0555
16 C 0.0040 0.0156 -0.0369
17 C 0.0218 0.0134 -0.0203
18 C -0.0084 0.0005 -0.0104
19 C 0.0406 0.0918 0.0126
20 C 0.0024 -0.0238 0.0090
21 C -0.0057 0.0429 -0.0211
22 C 0.0419 -0.0224 0.0138
23 C 0.0084 -0.0742 0.0191
24 H 0.1208 -0.0564 -0.0110
25 H 0.1109 -0.0489 0.0818
26 H -0.0102 0.0133 -0.0044
27 H 0.0331 -0.0208 -0.0187
28 H -0.0472 -0.0236 -0.0487
29 H 0.0622 -0.0232 0.0506
30 H -0.1963 0.0458 -0.0334
31 H 0.2011 -0.0806 0.0720
32 H -0.1002 0.0774 0.0794
33 H -0.0631 0.0183 0.0857
34 H -0.0916 -0.0412 0.0619
35 H 0.1504 0.1440 -0.1372
36 H -0.1884 -0.0598 -0.0644
37 H -0.2427 -0.3055 0.0269
38 H -0.0035 0.0278 -0.0552
39 H 0.0020 -0.0300 0.0015
40 H -0.0150 -0.0101 -0.0041
41 H -0.0126 -0.0412 -0.1257
42 H 0.1040 -0.0501 -0.0175
43 H -0.1765 0.1191 -0.0027
44 H -0.0154 0.0018 0.0051
45 H 0.0270 0.0368 0.0033
46 H 0.3623 -0.2057 0.0976
47 H 0.2427 -0.0282 0.0582
48 H -0.1078 0.0740 0.0960
49 H -0.0748 -0.3378 0.0714
Vibrational Mode 72
----------------------------------------------------
Harmonic frequency: 1152.23 cm**-1
Reduced mass: 1.9650 amu
Force constant: 1.5370 mdyne/A
IR intensity: 2.3891 km/mol
Normal mode:
X Y Z
1 O 0.0051 0.0000 -0.0152
2 O 0.0094 0.0011 -0.0060
3 O 0.0209 -0.0038 0.0050
4 C 0.1067 -0.0774 0.0277
5 C 0.0253 -0.0026 -0.0092
6 C -0.0439 0.0415 -0.0043
7 C 0.0206 -0.0292 -0.0206
8 C -0.0141 0.0196 -0.0389
9 C -0.0720 0.0337 0.0797
10 C 0.0280 -0.0689 0.0475
11 C -0.0847 0.0333 -0.0382
12 C -0.0304 0.0287 -0.0096
13 C 0.0082 -0.0239 0.0011
14 C -0.0031 -0.1016 0.0203
15 C 0.0736 0.0702 0.0147
16 C 0.0256 -0.0118 -0.0340
17 C -0.0264 0.0221 -0.0084
18 C 0.0088 0.0066 -0.0111
19 C -0.0197 -0.0309 0.0021
20 C 0.0035 0.0116 -0.0067
21 C -0.0017 -0.0065 0.0064
22 C -0.0098 0.0005 -0.0028
23 C -0.0000 0.0169 -0.0040
24 H 0.1877 -0.0619 0.0144
25 H 0.1657 0.0945 0.1599
26 H -0.0262 0.0189 0.0116
27 H -0.0624 0.0300 0.1257
28 H -0.1192 -0.0452 -0.0858
29 H -0.2114 0.0244 0.0775
30 H -0.2517 0.0958 -0.0580
31 H -0.0995 0.0583 0.0033
32 H -0.1628 0.1282 0.1122
33 H 0.0529 -0.0280 -0.0165
34 H -0.2372 -0.1496 0.1681
35 H 0.1414 0.1139 -0.1523
36 H 0.0029 -0.2312 0.0242
37 H 0.2748 0.1539 0.0317
38 H 0.0103 0.0363 -0.0232
39 H -0.0108 0.0239 0.0609
40 H -0.0565 -0.0329 -0.1179
41 H -0.0657 0.0075 -0.0527
42 H -0.0437 0.0361 -0.0088
43 H -0.0496 0.0321 -0.0136
44 H 0.2259 0.1466 -0.0599
45 H 0.0963 0.1885 0.0002
46 H -0.2243 0.1452 -0.0242
47 H -0.0876 0.0026 -0.0173
48 H 0.0351 -0.0184 -0.0256
49 H 0.0213 0.0834 -0.0172
Vibrational Mode 73
----------------------------------------------------
Harmonic frequency: 1155.31 cm**-1
Reduced mass: 1.5926 amu
Force constant: 1.2524 mdyne/A
IR intensity: 3.3321 km/mol
Normal mode:
X Y Z
1 O -0.0094 -0.0018 0.0013
2 O 0.0050 0.0091 -0.0073
3 O -0.0005 -0.0023 0.0014
4 C 0.0999 -0.0147 0.0092
5 C -0.0231 0.0606 0.0150
6 C 0.0109 0.0274 0.0092
7 C -0.0143 -0.0145 -0.0065
8 C -0.0103 -0.0072 -0.0049
9 C -0.0660 -0.0811 -0.0235
10 C 0.0455 0.0194 -0.0001
11 C -0.0053 -0.0015 0.0277
12 C 0.0535 -0.0245 -0.0522
13 C -0.0336 -0.0312 0.0395
14 C -0.0119 -0.0039 0.0165
15 C -0.0115 0.0254 -0.0241
16 C 0.0285 0.0357 0.0109
17 C -0.0516 -0.0106 0.0130
18 C -0.0218 0.0082 0.0045
19 C 0.0230 0.0460 -0.0146
20 C 0.0060 0.0051 -0.0012
21 C -0.0101 0.0220 -0.0139
22 C 0.0117 -0.0125 0.0061
23 C -0.0093 -0.0159 0.0021
24 H -0.0267 0.0268 0.0094
25 H -0.1190 -0.0687 -0.0946
26 H 0.1410 -0.0239 -0.1423
27 H -0.1610 0.0851 0.2035
28 H -0.1879 0.0381 0.0073
29 H -0.1754 0.0354 -0.0184
30 H -0.0144 0.0894 0.0187
31 H -0.1872 0.0782 -0.0560
32 H 0.1106 -0.0544 -0.0981
33 H 0.0089 -0.0004 -0.0257
34 H -0.0292 -0.0307 0.0357
35 H -0.0284 -0.0280 0.0321
36 H 0.0503 -0.0645 0.0120
37 H -0.0204 0.0316 -0.0224
38 H 0.0954 0.0880 0.0093
39 H -0.0080 -0.0372 0.1769
40 H -0.0541 -0.0433 0.0657
41 H -0.0571 -0.0528 -0.1569
42 H -0.1954 0.1244 0.0024
43 H 0.2418 -0.1614 0.0183
44 H -0.1415 -0.0640 0.0380
45 H -0.0391 -0.0606 -0.0038
46 H 0.4758 -0.2595 0.0563
47 H 0.0880 -0.0146 0.0212
48 H 0.1833 -0.1229 -0.1633
49 H 0.0038 0.0275 -0.0055
Vibrational Mode 74
----------------------------------------------------
Harmonic frequency: 1167.54 cm**-1
Reduced mass: 1.8817 amu
Force constant: 1.5113 mdyne/A
IR intensity: 12.7496 km/mol
Normal mode:
X Y Z
1 O -0.0033 -0.0259 0.0019
2 O -0.0149 -0.0097 0.0085
3 O 0.0038 -0.0049 0.0024
4 C 0.0045 0.0815 0.0777
5 C 0.0365 0.0145 -0.0139
6 C 0.0197 0.0598 0.0172
7 C -0.0050 -0.0309 -0.0221
8 C -0.0517 -0.0336 -0.0020
9 C -0.1369 -0.0128 -0.0076
10 C -0.0553 0.0583 -0.0839
11 C 0.0237 -0.0469 -0.0358
12 C 0.0145 -0.0146 0.0078
13 C -0.0237 -0.0189 0.0181
14 C -0.0069 -0.0369 0.0317
15 C -0.0234 0.0278 -0.0177
16 C 0.0588 0.0056 0.0048
17 C 0.0829 -0.0132 0.0094
18 C 0.0003 0.0038 0.0022
19 C 0.0093 -0.0036 -0.0169
20 C 0.0154 0.0120 -0.0055
21 C -0.0066 0.0071 -0.0013
22 C 0.0036 -0.0001 0.0012
23 C -0.0056 0.0064 -0.0022
24 H 0.0665 -0.3154 -0.0160
25 H 0.1181 0.0414 0.0920
26 H 0.0735 0.0435 -0.1129
27 H 0.0691 -0.0369 0.0570
28 H -0.0015 0.0594 0.0603
29 H 0.2419 -0.0570 -0.1161
30 H 0.2386 -0.1717 0.0012
31 H -0.0074 -0.0148 -0.0047
32 H 0.1052 -0.0751 -0.0689
33 H -0.2239 0.1133 0.1953
34 H 0.0523 0.0356 -0.0320
35 H 0.0008 0.0079 0.0283
36 H 0.1801 -0.0216 0.0220
37 H 0.2538 0.1454 0.0083
38 H -0.1215 -0.0392 -0.0427
39 H -0.0286 0.0406 0.2623
40 H -0.1026 -0.0601 -0.1124
41 H -0.1214 -0.0085 -0.1089
42 H 0.1820 -0.1462 0.0297
43 H -0.1736 0.1156 -0.0264
44 H 0.0749 0.0503 -0.0249
45 H 0.0484 0.0636 0.0123
46 H 0.0656 -0.0217 -0.0115
47 H -0.0069 0.0010 0.0008
48 H -0.1978 0.1263 0.1695
49 H -0.0098 -0.0115 -0.0013
Vibrational Mode 75
----------------------------------------------------
Harmonic frequency: 1174.26 cm**-1
Reduced mass: 1.4625 amu
Force constant: 1.1881 mdyne/A
IR intensity: 17.5323 km/mol
Normal mode:
X Y Z
1 O 0.0030 -0.0216 -0.0115
2 O -0.0029 -0.0049 0.0025
3 O 0.0155 -0.0190 0.0080
4 C 0.0367 0.0563 0.0348
5 C -0.0566 0.0346 -0.0032
6 C 0.0124 0.0382 0.0165
7 C -0.0174 -0.0179 -0.0044
8 C -0.0283 -0.0161 -0.0098
9 C 0.0617 -0.0279 0.0537
10 C -0.0442 0.0084 -0.0203
11 C 0.0310 -0.0288 0.0146
12 C 0.0426 -0.0231 -0.0307
13 C -0.0553 -0.0256 0.0078
14 C 0.0247 -0.0067 -0.0275
15 C -0.0349 0.0085 0.0005
16 C -0.0262 0.0111 -0.0220
17 C 0.0321 0.0006 0.0029
18 C -0.0085 0.0045 -0.0067
19 C 0.0037 -0.0143 0.0127
20 C -0.0109 0.0051 -0.0022
21 C -0.0061 -0.0033 -0.0015
22 C -0.0050 0.0135 -0.0045
23 C -0.0096 0.0239 -0.0084
24 H -0.0056 0.1043 0.0054
25 H 0.1634 0.3513 0.1520
26 H 0.1151 0.0037 -0.1281
27 H 0.0518 -0.0323 0.0303
28 H 0.1451 -0.0088 0.0090
29 H -0.0697 0.0108 -0.0573
30 H 0.0474 0.0049 0.0151
31 H -0.0900 0.0285 -0.0294
32 H -0.1064 0.0096 0.0785
33 H 0.3700 -0.1966 -0.3217
34 H -0.1378 -0.2397 0.0994
35 H -0.1788 -0.1495 0.1976
36 H -0.0344 0.0562 -0.0222
37 H -0.1285 -0.1219 -0.0099
38 H 0.1462 0.1375 -0.0284
39 H 0.0154 -0.0594 -0.1026
40 H 0.0370 0.0172 0.0784
41 H 0.0517 -0.0273 -0.0323
42 H 0.0946 -0.0723 0.0115
43 H -0.0860 0.0577 -0.0218
44 H 0.0396 0.0392 -0.0035
45 H 0.1221 0.2358 0.0045
46 H 0.0227 -0.0068 0.0171
47 H -0.0578 0.0158 -0.0175
48 H -0.0769 0.0502 0.0743
49 H -0.0086 0.0256 -0.0077
Vibrational Mode 76
----------------------------------------------------
Harmonic frequency: 1178.95 cm**-1
Reduced mass: 2.4201 amu
Force constant: 1.9818 mdyne/A
IR intensity: 1.8886 km/mol
Normal mode:
X Y Z
1 O -0.0100 -0.0020 0.0002
2 O 0.0049 0.0087 -0.0042
3 O 0.0745 -0.0704 0.0329
4 C -0.0441 0.0247 0.0388
5 C -0.0149 0.0188 -0.0254
6 C 0.0333 0.0125 0.0028
7 C -0.0088 -0.0051 -0.0262
8 C 0.0001 -0.0009 -0.0044
9 C -0.0963 0.0873 0.0833
10 C 0.0679 0.0025 0.0275
11 C 0.0117 -0.0205 -0.0188
12 C 0.0340 -0.0205 0.0097
13 C -0.0272 0.0033 0.0012
14 C 0.0970 0.0674 -0.0888
15 C -0.0077 -0.0321 0.0770
16 C 0.0308 -0.0219 -0.0273
17 C -0.0493 -0.0039 -0.0028
18 C -0.0098 -0.0385 0.0006
19 C -0.0029 -0.0900 0.0151
20 C 0.0165 0.0595 -0.0262
21 C -0.0409 -0.0660 0.0090
22 C -0.0181 0.0497 -0.0152
23 C -0.0334 0.1136 -0.0359
24 H 0.1454 -0.1121 -0.0022
25 H -0.0399 0.0660 -0.0504
26 H -0.0956 0.0968 0.0240
27 H 0.1711 -0.0893 -0.1198
28 H -0.0204 0.0113 0.0038
29 H -0.0047 -0.0015 0.0065
30 H -0.1578 0.0060 -0.0346
31 H 0.2008 -0.1056 0.0558
32 H 0.0568 -0.0706 -0.0240
33 H -0.1049 0.0692 0.0493
34 H 0.0150 -0.0480 -0.0093
35 H -0.0106 0.0198 0.0650
36 H 0.2528 -0.1020 -0.0719
37 H -0.2591 -0.3651 0.0445
38 H -0.0714 -0.0362 -0.0595
39 H -0.0192 0.0696 0.0699
40 H -0.0517 -0.0067 -0.2096
41 H -0.0723 0.0187 -0.0284
42 H -0.1337 0.1104 -0.0199
43 H 0.1430 -0.0958 0.0316
44 H -0.2027 -0.1317 0.0888
45 H -0.0385 -0.0470 -0.0083
46 H 0.1244 0.0172 0.0382
47 H -0.2899 0.0619 -0.0751
48 H 0.1334 -0.0867 -0.1167
49 H -0.0336 0.0964 -0.0345
Vibrational Mode 77
----------------------------------------------------
Harmonic frequency: 1188.70 cm**-1
Reduced mass: 1.9406 amu
Force constant: 1.6156 mdyne/A
IR intensity: 24.6405 km/mol
Normal mode:
X Y Z
1 O 0.0208 0.0115 -0.0087
2 O 0.0034 -0.0159 -0.0007
3 O 0.0321 -0.0162 0.0113
4 C 0.0249 -0.0728 -0.1140
5 C 0.0578 -0.0375 0.0379
6 C -0.0333 -0.0126 -0.0139
7 C 0.0222 0.0046 0.0409
8 C 0.0168 0.0238 0.0150
9 C -0.0825 0.0162 -0.0710
10 C -0.1046 -0.0634 -0.0010
11 C -0.0114 0.0443 0.0696
12 C -0.0225 0.0217 -0.0143
13 C 0.0293 0.0089 0.0217
14 C 0.0003 0.0652 -0.0022
15 C 0.0093 -0.0286 -0.0262
16 C 0.0199 0.0028 0.0291
17 C 0.0488 0.0277 0.0011
18 C -0.0099 -0.0301 0.0170
19 C 0.0019 -0.0145 -0.0178
20 C 0.0074 0.0293 -0.0114
21 C -0.0307 -0.0175 -0.0025
22 C -0.0010 0.0040 0.0003
23 C -0.0083 0.0251 -0.0075
24 H 0.1786 0.2155 0.0501
25 H 0.0032 0.1068 0.0248
26 H 0.0026 -0.0921 0.0858
27 H -0.1220 0.0605 0.0746
28 H 0.1446 -0.0696 -0.0352
29 H -0.0380 0.0140 0.0623
30 H -0.0484 0.1522 0.0484
31 H -0.1673 0.0897 -0.0516
32 H 0.0302 0.0439 -0.0374
33 H 0.0270 -0.0242 0.0392
34 H 0.0080 0.0928 0.0133
35 H -0.0726 -0.0945 0.0056
36 H 0.2904 -0.2488 -0.0066
37 H -0.2701 -0.0711 -0.0471
38 H 0.1426 0.0402 0.0721
39 H -0.0089 0.0259 0.0938
40 H -0.0364 -0.0121 -0.0387
41 H -0.0448 0.0144 0.0068
42 H 0.1871 -0.1676 0.0321
43 H -0.2436 0.1655 -0.0603
44 H -0.1503 -0.1075 0.0614
45 H 0.0893 0.1439 0.0065
46 H 0.2431 -0.1079 0.0167
47 H -0.0740 0.0059 -0.0198
48 H -0.1907 0.1276 0.1742
49 H 0.0062 0.0648 -0.0171
Vibrational Mode 78
----------------------------------------------------
Harmonic frequency: 1196.78 cm**-1
Reduced mass: 1.4679 amu
Force constant: 1.2387 mdyne/A
IR intensity: 10.1399 km/mol
Normal mode:
X Y Z
1 O 0.0047 0.0041 -0.0081
2 O 0.0068 -0.0028 -0.0034
3 O 0.0018 0.0146 -0.0027
4 C -0.0152 -0.0319 0.0263
5 C -0.0021 0.0489 0.0146
6 C 0.0031 0.0222 -0.0050
7 C 0.0075 0.0090 -0.0200
8 C -0.0012 0.0124 -0.0153
9 C 0.0178 0.0891 -0.0517
10 C -0.0015 -0.0488 0.0378
11 C -0.0339 0.0062 -0.0552
12 C 0.0407 -0.0306 -0.0325
13 C -0.0247 -0.0036 0.0280
14 C -0.0265 -0.0044 0.0345
15 C 0.0360 -0.0034 0.0172
16 C -0.0019 -0.0301 0.0125
17 C -0.0113 0.0175 -0.0065
18 C -0.0091 -0.0365 0.0182
19 C -0.0042 0.0438 -0.0134
20 C -0.0117 -0.0050 0.0019
21 C -0.0116 0.0126 -0.0102
22 C 0.0042 -0.0177 0.0055
23 C 0.0032 -0.0358 0.0098
24 H -0.0804 0.0108 0.0010
25 H -0.0013 0.2451 0.0065
26 H -0.0861 0.0383 0.0566
27 H 0.1468 -0.0683 -0.1389
28 H 0.1211 -0.0560 -0.0462
29 H -0.0463 0.0064 0.0290
30 H -0.0900 -0.0608 -0.0527
31 H 0.2395 -0.1108 0.0650
32 H 0.0463 -0.0196 -0.0329
33 H 0.1041 -0.0655 -0.0735
34 H -0.0118 -0.0407 0.0266
35 H -0.1673 -0.1501 0.1499
36 H -0.0407 -0.0302 0.0305
37 H 0.3159 0.1904 0.0419
38 H -0.3446 -0.2540 -0.0105
39 H -0.0042 0.0558 -0.0385
40 H 0.0142 0.0159 -0.0699
41 H 0.0064 0.0550 0.1341
42 H 0.0211 -0.0169 -0.0022
43 H -0.0609 0.0428 -0.0088
44 H -0.2604 -0.1840 0.0977
45 H 0.0355 0.0812 -0.0057
46 H 0.2899 -0.1666 0.0657
47 H 0.1294 -0.0216 0.0358
48 H -0.0165 0.0142 0.0210
49 H 0.0353 0.0706 -0.0107
Vibrational Mode 79
----------------------------------------------------
Harmonic frequency: 1198.38 cm**-1
Reduced mass: 1.5693 amu
Force constant: 1.3279 mdyne/A
IR intensity: 9.0668 km/mol
Normal mode:
X Y Z
1 O -0.0088 -0.0232 -0.0141
2 O 0.0038 0.0089 0.0003
3 O -0.0146 0.0134 -0.0061
4 C -0.1002 0.0679 -0.0518
5 C 0.0178 -0.0003 0.0378
6 C 0.0037 0.0437 -0.0342
7 C 0.0191 -0.0339 0.0152
8 C 0.0209 -0.0157 0.0109
9 C 0.0030 0.0104 -0.0438
10 C 0.0532 0.0008 0.0561
11 C 0.0354 -0.0194 0.0396
12 C -0.0135 -0.0053 -0.0507
13 C -0.0148 -0.0176 0.0353
14 C -0.0266 0.0012 0.0182
15 C -0.0263 -0.0143 -0.0552
16 C -0.0074 -0.0014 0.0193
17 C -0.0416 0.0014 -0.0092
18 C 0.0019 -0.0031 0.0047
19 C 0.0053 0.0139 -0.0160
20 C 0.0027 -0.0090 0.0040
21 C 0.0101 0.0110 -0.0003
22 C -0.0014 -0.0073 0.0025
23 C 0.0066 -0.0217 0.0074
24 H 0.1235 -0.2396 0.0368
25 H 0.2510 0.0644 0.1638
26 H -0.2054 0.1084 0.1756
27 H 0.1329 -0.0894 -0.0721
28 H 0.1784 -0.0099 0.0281
29 H -0.1049 0.0223 -0.0139
30 H 0.2803 -0.0844 0.0655
31 H -0.0553 -0.0024 -0.0697
32 H -0.0462 0.1057 0.0075
33 H -0.0856 0.0116 0.1211
34 H -0.2902 -0.1989 0.2277
35 H 0.1878 0.1864 -0.1622
36 H 0.1771 -0.1217 0.0090
37 H -0.0823 0.0583 -0.0542
38 H 0.1553 0.0834 0.0333
39 H 0.0098 0.0014 -0.0506
40 H 0.0128 0.0147 0.0097
41 H 0.0159 0.0099 0.0454
42 H -0.0604 0.0747 -0.0284
43 H 0.0920 -0.0615 0.0284
44 H -0.0607 -0.0415 0.0229
45 H 0.0989 0.2197 -0.0022
46 H 0.0260 -0.0269 -0.0035
47 H 0.0552 -0.0104 0.0120
48 H 0.1143 -0.0732 -0.0931
49 H 0.0118 -0.0027 0.0019
Vibrational Mode 80
----------------------------------------------------
Harmonic frequency: 1216.86 cm**-1
Reduced mass: 1.9767 amu
Force constant: 1.7245 mdyne/A
IR intensity: 17.2781 km/mol
Normal mode:
X Y Z
1 O 0.0159 0.0426 -0.0073
2 O 0.0149 -0.0034 -0.0052
3 O -0.0041 -0.0123 0.0022
4 C -0.0180 0.0252 0.0850
5 C 0.0320 0.0452 -0.0051
6 C 0.0199 -0.0264 -0.0290
7 C -0.0293 0.0241 -0.0314
8 C -0.0278 0.0409 -0.0243
9 C -0.0336 -0.1062 -0.0379
10 C 0.0167 -0.1649 0.1021
11 C 0.0358 -0.0143 -0.0254
12 C -0.0093 -0.0255 -0.0041
13 C 0.0132 0.0271 0.0187
14 C -0.0025 0.0235 0.0040
15 C -0.0354 -0.0074 0.0131
16 C 0.0128 0.0291 0.0156
17 C -0.0402 0.0457 -0.0262
18 C 0.0033 0.0300 -0.0063
19 C 0.0096 -0.0189 0.0021
20 C 0.0072 0.0015 0.0004
21 C 0.0059 -0.0030 0.0045
22 C 0.0067 0.0063 -0.0006
23 C -0.0049 0.0247 -0.0071
24 H -0.0027 -0.0560 -0.0102
25 H -0.1731 0.0344 -0.1692
26 H 0.1017 -0.0240 -0.1355
27 H 0.0598 -0.0213 -0.0748
28 H 0.5263 -0.1791 -0.1102
29 H -0.4293 0.0890 0.0868
30 H 0.1223 -0.1497 -0.0016
31 H -0.0787 0.0617 0.0199
32 H 0.0762 -0.0170 -0.0560
33 H -0.0600 -0.0121 0.1235
34 H 0.0787 0.0957 -0.0364
35 H -0.0406 -0.0340 0.0189
36 H 0.0343 0.2297 -0.0026
37 H 0.0570 -0.0123 0.0193
38 H 0.0184 0.0381 -0.0215
39 H -0.0021 -0.0370 0.1061
40 H -0.0266 -0.0286 0.0874
41 H -0.0209 -0.0358 -0.0887
42 H 0.0336 -0.0134 -0.0225
43 H -0.1258 0.0870 -0.0219
44 H 0.1485 0.1082 -0.0672
45 H -0.0298 -0.0545 -0.0113
46 H -0.1273 0.0630 -0.0544
47 H -0.0717 0.0097 -0.0173
48 H -0.0084 0.0133 0.0177
49 H -0.0322 -0.0668 0.0107
Vibrational Mode 81
----------------------------------------------------
Harmonic frequency: 1221.93 cm**-1
Reduced mass: 2.1326 amu
Force constant: 1.8760 mdyne/A
IR intensity: 5.2993 km/mol
Normal mode:
X Y Z
1 O -0.0055 -0.0226 -0.0082
2 O -0.0003 -0.0015 -0.0009
3 O 0.0003 -0.0124 0.0029
4 C -0.0122 -0.0513 -0.0195
5 C 0.0193 0.0577 0.0355
6 C -0.0370 0.0924 -0.0196
7 C 0.0593 -0.0429 -0.0047
8 C 0.0213 -0.0063 -0.0069
9 C -0.0114 -0.1561 -0.0987
10 C -0.0036 0.0289 0.0007
11 C -0.0430 0.0177 -0.0158
12 C 0.0202 -0.0147 -0.0819
13 C -0.0191 -0.0501 0.0680
14 C 0.0119 0.0944 -0.0022
15 C 0.0327 -0.0280 0.0655
16 C 0.0057 0.0388 0.0441
17 C 0.0080 -0.0009 0.0059
18 C 0.0068 0.0430 -0.0063
19 C -0.0075 -0.0321 0.0124
20 C 0.0104 0.0068 -0.0002
21 C 0.0063 -0.0298 0.0123
22 C 0.0043 0.0082 -0.0023
23 C -0.0004 0.0337 -0.0087
24 H -0.1284 0.1782 0.0119
25 H 0.1600 0.0413 0.1339
26 H -0.1656 0.0640 0.1679
27 H 0.0190 -0.0224 0.0142
28 H -0.2193 0.0413 0.0020
29 H 0.1684 -0.0384 -0.0081
30 H -0.2415 0.0893 -0.0410
31 H 0.2580 -0.1301 0.0611
32 H 0.1456 0.0014 -0.1540
33 H -0.0585 0.0035 0.0951
34 H -0.1140 -0.0092 0.1098
35 H 0.0034 -0.0270 -0.0518
36 H -0.1661 0.3566 -0.0047
37 H -0.0669 -0.1244 0.0510
38 H -0.1978 -0.1580 -0.0014
39 H 0.0134 -0.0489 0.0611
40 H -0.0110 -0.0159 0.1391
41 H -0.0009 -0.0445 -0.0766
42 H 0.0054 -0.0195 0.0136
43 H -0.0048 0.0065 0.0034
44 H 0.1229 0.0948 -0.0758
45 H 0.0765 0.1509 0.0036
46 H -0.2273 0.1153 -0.0934
47 H -0.0941 0.0127 -0.0216
48 H -0.0170 0.0107 0.0153
49 H -0.0286 -0.0599 0.0117
Vibrational Mode 82
----------------------------------------------------
Harmonic frequency: 1240.31 cm**-1
Reduced mass: 2.3785 amu
Force constant: 2.1558 mdyne/A
IR intensity: 19.7090 km/mol
Normal mode:
X Y Z
1 O 0.0041 0.0061 -0.0042
2 O 0.0042 -0.0078 -0.0051
3 O -0.0345 0.0935 -0.0314
4 C 0.0115 -0.0341 -0.0079
5 C -0.0976 0.0419 -0.0264
6 C -0.0035 0.0562 -0.0035
7 C 0.0332 -0.0231 -0.0194
8 C 0.0084 0.0212 -0.0019
9 C 0.0077 0.0127 0.0846
10 C -0.0223 -0.0448 0.0091
11 C -0.0010 -0.0038 0.0242
12 C 0.0749 -0.0300 -0.0259
13 C -0.0267 -0.0241 0.0486
14 C 0.0822 -0.0191 -0.0126
15 C -0.0032 0.0167 0.0030
16 C -0.0036 -0.0041 -0.0237
17 C 0.0063 0.0181 0.0025
18 C 0.0060 0.0408 -0.0280
19 C -0.0740 -0.0362 -0.0157
20 C 0.0292 0.0038 -0.0013
21 C 0.0800 -0.0494 0.0323
22 C -0.1234 0.0185 -0.0310
23 C 0.0706 -0.1281 0.0474
24 H -0.0116 -0.0634 -0.0143
25 H -0.0652 0.0237 -0.0480
26 H -0.1158 0.0533 0.0950
27 H -0.0188 0.0074 0.0369
28 H 0.0081 -0.0415 -0.0422
29 H -0.0117 0.0113 0.0286
30 H 0.0127 0.0359 0.0214
31 H -0.1538 0.0632 -0.0374
32 H 0.2055 -0.1599 -0.1537
33 H -0.2379 0.1484 0.1549
34 H 0.1262 0.1339 -0.0708
35 H -0.0749 -0.0747 0.0613
36 H 0.1568 -0.0230 -0.0139
37 H -0.1581 -0.1381 -0.0125
38 H 0.0936 0.0870 -0.0205
39 H -0.0008 0.0151 -0.0382
40 H 0.0108 0.0087 -0.0285
41 H -0.0008 -0.0129 -0.0354
42 H 0.0139 -0.0422 0.0218
43 H -0.0626 0.0481 -0.0289
44 H -0.2334 -0.0984 0.0550
45 H 0.0174 0.0249 0.0007
46 H -0.2483 0.1660 -0.0363
47 H 0.3758 -0.0036 0.0842
48 H -0.0620 0.0413 0.0569
49 H 0.1980 0.3180 -0.0374
Vibrational Mode 83
----------------------------------------------------
Harmonic frequency: 1242.42 cm**-1
Reduced mass: 2.0671 amu
Force constant: 1.8800 mdyne/A
IR intensity: 11.1124 km/mol
Normal mode:
X Y Z
1 O -0.0030 -0.0018 0.0057
2 O -0.0044 0.0073 0.0048
3 O 0.0356 0.0701 -0.0097
4 C 0.0163 0.0495 0.0262
5 C 0.0785 -0.0248 0.0235
6 C 0.0168 -0.0627 0.0116
7 C -0.0483 0.0237 0.0158
8 C -0.0210 -0.0206 -0.0009
9 C -0.0467 -0.0584 -0.0530
10 C 0.0208 0.0369 -0.0148
11 C -0.0049 -0.0179 -0.0266
12 C -0.0664 0.0228 0.0226
13 C 0.0204 0.0264 -0.0494
14 C 0.0457 0.0821 0.0116
15 C 0.0023 -0.0182 0.0393
16 C 0.0108 0.0182 0.0175
17 C -0.0046 -0.0175 -0.0025
18 C -0.0046 -0.0086 0.0094
19 C -0.0836 -0.0205 -0.0386
20 C -0.0017 0.0348 -0.0119
21 C -0.0359 -0.0373 0.0045
22 C -0.0854 -0.0010 -0.0178
23 C 0.0548 -0.1039 0.0384
24 H 0.1000 0.1060 0.0264
25 H 0.0392 -0.0056 0.0270
26 H 0.1668 -0.0540 -0.1564
27 H 0.0123 -0.0035 -0.0141
28 H 0.0244 0.0349 0.0391
29 H -0.0223 -0.0049 -0.0324
30 H -0.0417 -0.0224 -0.0256
31 H 0.1456 -0.0810 0.0360
32 H -0.2085 0.1508 0.1574
33 H 0.2653 -0.1661 -0.1734
34 H -0.1363 -0.1616 0.0789
35 H 0.0773 0.0846 -0.0479
36 H 0.3398 0.0763 -0.0013
37 H 0.0018 -0.0939 0.0360
38 H -0.2054 -0.1467 -0.0057
39 H 0.0015 -0.0330 0.0752
40 H -0.0310 -0.0216 0.0405
41 H -0.0197 -0.0127 -0.0395
42 H -0.0156 0.0417 -0.0207
43 H 0.0539 -0.0430 0.0239
44 H 0.0967 0.0525 -0.0290
45 H -0.0323 -0.0569 -0.0011
46 H 0.0241 0.0074 -0.0302
47 H 0.2924 -0.0182 0.0716
48 H 0.0572 -0.0382 -0.0530
49 H 0.1938 0.3631 -0.0496
Vibrational Mode 84
----------------------------------------------------
Harmonic frequency: 1267.06 cm**-1
Reduced mass: 2.0718 amu
Force constant: 1.9597 mdyne/A
IR intensity: 39.1943 km/mol
Normal mode:
X Y Z
1 O -0.0036 0.0086 0.0040
2 O 0.0004 -0.0005 -0.0059
3 O 0.0970 0.0068 0.0194
4 C -0.0617 -0.0471 0.0334
5 C 0.0023 0.0331 -0.0180
6 C -0.0518 0.0805 -0.0040
7 C 0.0751 -0.0364 -0.0439
8 C -0.0111 0.0223 -0.0014
9 C 0.0552 0.0137 0.0169
10 C 0.0400 -0.0299 -0.0029
11 C 0.0026 0.0188 0.0053
12 C 0.0034 -0.0003 0.0182
13 C -0.0041 -0.0369 0.0031
14 C 0.0185 -0.0059 -0.0004
15 C -0.0138 -0.0156 -0.0021
16 C -0.0137 -0.0046 -0.0025
17 C 0.0048 0.0023 0.0049
18 C 0.0008 -0.0723 0.0348
19 C -0.0357 0.0304 -0.0227
20 C -0.0570 0.0159 -0.0048
21 C -0.1694 0.0347 -0.0462
22 C 0.0064 -0.0161 0.0060
23 C -0.0028 -0.0386 0.0090
24 H -0.0808 -0.0435 -0.0233
25 H 0.0208 -0.1209 0.0372
26 H -0.1851 0.0679 0.1614
27 H -0.0664 0.0319 0.0478
28 H -0.0054 -0.0265 -0.0288
29 H 0.1275 -0.0244 0.0376
30 H 0.1451 -0.0836 0.0292
31 H -0.0941 0.0720 0.0166
32 H 0.0290 -0.0387 -0.0108
33 H 0.0158 0.0000 -0.0266
34 H 0.1502 0.1550 -0.1218
35 H -0.0730 -0.1020 0.0284
36 H 0.1655 0.0984 -0.0080
37 H -0.0519 -0.0237 -0.0059
38 H 0.1403 0.0894 0.0053
39 H 0.0079 0.0108 -0.0808
40 H 0.0321 0.0262 -0.0073
41 H 0.0313 -0.0094 0.0082
42 H -0.0937 0.0492 0.0151
43 H -0.0519 0.0305 -0.0157
44 H 0.5797 0.2651 -0.1671
45 H -0.0486 -0.1022 0.0000
46 H 0.2130 -0.1360 0.0579
47 H 0.0598 -0.0202 0.0165
48 H -0.0077 0.0057 0.0022
49 H 0.0774 0.2133 -0.0370
Vibrational Mode 85
----------------------------------------------------
Harmonic frequency: 1271.31 cm**-1
Reduced mass: 1.7411 amu
Force constant: 1.6580 mdyne/A
IR intensity: 22.2562 km/mol
Normal mode:
X Y Z
1 O -0.0018 -0.0444 -0.0271
2 O 0.0031 0.0084 0.0177
3 O 0.0130 0.0041 0.0017
4 C -0.0152 0.0402 0.0150
5 C 0.0062 -0.0071 -0.0146
6 C -0.0890 -0.0212 -0.1086
7 C -0.0012 0.0130 0.0513
8 C 0.0750 -0.0422 -0.0315
9 C -0.0091 0.0206 0.0146
10 C -0.0242 0.0611 0.1135
11 C 0.0091 -0.0154 -0.0078
12 C -0.0162 -0.0080 0.0301
13 C 0.0513 0.0301 0.0051
14 C 0.0207 -0.0191 -0.0036
15 C -0.0077 0.0068 0.0037
16 C 0.0045 -0.0055 -0.0098
17 C -0.0513 0.0103 -0.0249
18 C -0.0003 -0.0124 0.0049
19 C -0.0073 0.0016 0.0007
20 C -0.0055 0.0044 -0.0018
21 C -0.0206 0.0031 -0.0056
22 C -0.0061 0.0007 -0.0016
23 C 0.0017 -0.0105 0.0028
24 H -0.0945 -0.0435 -0.0235
25 H 0.1343 -0.1726 0.0637
26 H 0.2541 -0.1834 -0.1314
27 H 0.0104 -0.0113 -0.0431
28 H -0.2455 0.0628 0.0073
29 H -0.1925 0.0269 -0.0414
30 H -0.0124 -0.0094 -0.0085
31 H 0.0177 -0.0167 -0.0040
32 H 0.1358 -0.0676 -0.0910
33 H -0.0381 0.0107 0.0275
34 H 0.1445 0.2000 -0.0940
35 H -0.1865 -0.2109 0.0593
36 H -0.0488 0.0589 -0.0022
37 H 0.0136 -0.0270 0.0053
38 H 0.0085 0.0142 0.0027
39 H -0.0116 0.0058 0.0463
40 H -0.0032 -0.0053 -0.0196
41 H -0.0051 0.0093 0.0068
42 H 0.3022 -0.1402 -0.0629
43 H 0.1472 -0.0760 0.0892
44 H 0.0630 0.0254 -0.0181
45 H 0.2342 0.4762 0.0068
46 H 0.0354 -0.0165 0.0124
47 H 0.0248 -0.0005 0.0062
48 H 0.0818 -0.0506 -0.0453
49 H 0.0185 0.0444 -0.0072
Vibrational Mode 86
----------------------------------------------------
Harmonic frequency: 1278.75 cm**-1
Reduced mass: 1.3551 amu
Force constant: 1.3055 mdyne/A
IR intensity: 9.9375 km/mol
Normal mode:
X Y Z
1 O -0.0001 -0.0280 -0.0191
2 O 0.0011 0.0138 0.0218
3 O 0.0141 0.0005 0.0028
4 C 0.0320 -0.0222 -0.0042
5 C -0.0125 0.0008 -0.0009
6 C 0.0638 -0.0538 0.0363
7 C 0.0060 0.0053 -0.0446
8 C -0.0693 0.0023 -0.0087
9 C 0.0088 0.0010 -0.0015
10 C -0.0243 0.0409 0.0644
11 C -0.0094 0.0012 0.0037
12 C 0.0022 0.0042 -0.0093
13 C -0.0188 -0.0009 0.0065
14 C 0.0101 -0.0052 0.0013
15 C 0.0127 0.0096 0.0010
16 C -0.0012 -0.0012 0.0029
17 C -0.0531 0.0046 -0.0255
18 C 0.0016 -0.0082 0.0045
19 C -0.0069 0.0056 0.0006
20 C -0.0069 0.0010 -0.0000
21 C -0.0247 0.0028 -0.0061
22 C 0.0024 -0.0019 0.0009
23 C -0.0013 -0.0047 0.0007
24 H -0.0243 -0.0101 -0.0037
25 H -0.3357 0.1300 -0.2578
26 H -0.1213 0.0994 0.0454
27 H -0.1654 0.0973 0.0953
28 H 0.2680 -0.0574 -0.0182
29 H 0.4388 -0.0940 -0.0592
30 H -0.0115 0.0383 0.0000
31 H -0.0419 0.0191 -0.0013
32 H 0.0139 0.0046 -0.0180
33 H -0.0494 0.0330 0.0281
34 H -0.0330 -0.0345 0.0241
35 H 0.1474 0.1650 -0.0751
36 H -0.0698 0.0263 0.0016
37 H -0.0401 -0.0183 -0.0043
38 H -0.0306 -0.0153 -0.0115
39 H 0.0033 0.0060 -0.0199
40 H 0.0058 0.0068 -0.0070
41 H 0.0032 -0.0032 0.0010
42 H 0.3620 -0.1702 -0.0731
43 H 0.1260 -0.0733 0.0918
44 H 0.0788 0.0350 -0.0263
45 H 0.1538 0.3082 0.0048
46 H 0.0149 -0.0121 0.0051
47 H 0.0067 -0.0022 0.0019
48 H 0.1078 -0.0663 -0.0699
49 H 0.0090 0.0277 -0.0047
Vibrational Mode 87
----------------------------------------------------
Harmonic frequency: 1283.39 cm**-1
Reduced mass: 1.7282 amu
Force constant: 1.6771 mdyne/A
IR intensity: 18.6047 km/mol
Normal mode:
X Y Z
1 O 0.0063 0.0088 0.0021
2 O 0.0020 -0.0166 -0.0093
3 O 0.0505 0.0029 0.0096
4 C 0.0471 0.0572 -0.0709
5 C -0.0357 -0.0238 0.0150
6 C 0.0774 -0.0279 0.0132
7 C -0.0653 0.0187 0.0459
8 C 0.0495 -0.0140 0.0153
9 C 0.0135 0.0312 -0.0129
10 C -0.0576 -0.0202 -0.0282
11 C -0.0109 -0.0351 0.0212
12 C 0.0059 0.0003 -0.0299
13 C -0.0209 -0.0029 0.0357
14 C 0.0650 -0.0205 0.0044
15 C 0.0477 0.0471 -0.0078
16 C -0.0007 -0.0104 0.0025
17 C 0.0235 0.0146 0.0093
18 C 0.0078 -0.0328 0.0180
19 C -0.0289 0.0197 0.0102
20 C -0.0196 0.0055 -0.0009
21 C -0.0871 0.0018 -0.0198
22 C 0.0064 -0.0039 0.0019
23 C -0.0051 -0.0176 0.0024
24 H -0.1584 -0.1468 -0.0105
25 H -0.0164 0.1123 -0.0443
26 H 0.0808 -0.0031 -0.0792
27 H 0.1179 -0.0670 -0.0708
28 H -0.0217 0.0103 0.0186
29 H -0.3568 0.0860 -0.0173
30 H -0.0507 0.0714 0.0031
31 H -0.0269 -0.0582 -0.1042
32 H 0.1557 -0.0515 -0.1422
33 H -0.2036 0.1069 0.1639
34 H -0.1052 -0.0802 0.0996
35 H 0.1828 0.1971 -0.1206
36 H -0.4476 0.0651 0.0115
37 H -0.1838 -0.1170 -0.0273
38 H -0.2060 -0.1273 -0.0186
39 H -0.0017 0.0080 -0.0020
40 H 0.0118 0.0126 -0.0341
41 H 0.0110 0.0117 0.0386
42 H -0.0733 -0.0058 0.0411
43 H 0.0024 0.0100 -0.0621
44 H 0.2297 0.0895 -0.0774
45 H -0.0115 -0.0299 -0.0004
46 H 0.0456 -0.0342 0.0163
47 H 0.0296 -0.0048 0.0081
48 H -0.1147 0.0702 0.0958
49 H 0.0335 0.1051 -0.0181
Vibrational Mode 88
----------------------------------------------------
Harmonic frequency: 1294.96 cm**-1
Reduced mass: 1.5744 amu
Force constant: 1.5555 mdyne/A
IR intensity: 2.8627 km/mol
Normal mode:
X Y Z
1 O 0.0037 -0.0049 -0.0019
2 O 0.0009 -0.0135 -0.0039
3 O 0.0038 -0.0013 0.0017
4 C -0.0080 -0.0789 0.0001
5 C -0.0293 0.0382 -0.0087
6 C 0.0634 -0.0733 0.0148
7 C -0.0396 0.0523 0.0101
8 C 0.0622 -0.0007 -0.0028
9 C 0.0394 -0.0177 0.0256
10 C -0.0536 0.0250 -0.0118
11 C 0.0169 0.0403 -0.0225
12 C 0.0087 -0.0175 -0.0156
13 C -0.0125 0.0238 0.0187
14 C -0.0537 -0.0038 -0.0087
15 C -0.0687 -0.0539 0.0372
16 C -0.0106 0.0074 -0.0079
17 C 0.0102 0.0108 0.0027
18 C 0.0024 -0.0019 -0.0001
19 C 0.0120 0.0034 -0.0205
20 C -0.0092 -0.0096 0.0037
21 C -0.0170 0.0247 -0.0096
22 C 0.0048 -0.0052 0.0028
23 C -0.0010 -0.0031 0.0012
24 H 0.0408 -0.0773 0.0012
25 H -0.0623 0.2615 -0.0609
26 H 0.0201 -0.0304 -0.0191
27 H 0.1614 -0.0688 -0.1909
28 H -0.2987 0.0416 -0.0078
29 H -0.0776 0.0168 0.0309
30 H -0.2149 0.0401 -0.0422
31 H 0.0910 0.0328 0.1133
32 H 0.0930 -0.0223 -0.0754
33 H -0.1296 0.0615 0.0957
34 H -0.0820 -0.0819 0.0789
35 H 0.1024 0.1336 -0.0421
36 H 0.5150 0.1416 -0.0206
37 H 0.2636 0.1044 0.0627
38 H 0.2668 0.1885 0.0047
39 H 0.0082 -0.0295 -0.0393
40 H 0.0076 -0.0009 0.0414
41 H 0.0230 -0.0134 -0.0174
42 H -0.0037 -0.0326 0.0219
43 H 0.0767 -0.0320 -0.0259
44 H 0.1145 0.0625 -0.0350
45 H 0.0322 0.0645 0.0006
46 H 0.0053 -0.0167 0.0084
47 H -0.0058 -0.0055 -0.0003
48 H -0.0879 0.0525 0.0781
49 H -0.0006 -0.0044 0.0001
Vibrational Mode 89
----------------------------------------------------
Harmonic frequency: 1301.17 cm**-1
Reduced mass: 1.4125 amu
Force constant: 1.4090 mdyne/A
IR intensity: 0.6669 km/mol
Normal mode:
X Y Z
1 O -0.0042 0.0008 0.0018
2 O -0.0007 0.0057 0.0035
3 O 0.0017 0.0042 -0.0008
4 C 0.0097 -0.0454 0.0372
5 C 0.0105 -0.0733 -0.0018
6 C 0.0065 0.0497 -0.0156
7 C -0.0576 0.0182 0.0382
8 C -0.0578 0.0202 0.0000
9 C -0.0263 0.0521 -0.0224
10 C 0.0373 -0.0046 -0.0008
11 C 0.0140 0.0203 -0.0058
12 C -0.0004 0.0189 -0.0421
13 C 0.0058 -0.0165 0.0183
14 C 0.0365 -0.0546 -0.0020
15 C -0.0357 -0.0067 0.0021
16 C 0.0093 -0.0117 0.0010
17 C -0.0123 -0.0013 -0.0042
18 C -0.0046 -0.0168 0.0092
19 C 0.0045 -0.0097 0.0091
20 C -0.0019 0.0070 -0.0030
21 C 0.0015 0.0072 -0.0024
22 C -0.0176 0.0085 -0.0060
23 C 0.0036 -0.0096 0.0029
24 H -0.0537 0.5889 0.0063
25 H 0.1203 -0.1800 0.0618
26 H 0.1461 -0.0666 -0.1205
27 H 0.3171 -0.1716 -0.2156
28 H 0.1025 -0.0434 -0.0249
29 H 0.2546 -0.0495 -0.0074
30 H 0.0107 -0.0358 0.0004
31 H -0.1046 0.0948 0.0390
32 H 0.1264 -0.0187 -0.1335
33 H -0.0919 0.0558 0.0799
34 H -0.1335 -0.0936 0.1104
35 H 0.0359 0.0137 -0.0596
36 H -0.1275 0.2164 -0.0033
37 H 0.0587 0.0104 0.0103
38 H 0.1909 0.1446 0.0037
39 H -0.0081 0.0170 0.0405
40 H -0.0126 -0.0088 -0.0346
41 H -0.0190 0.0300 0.0468
42 H 0.0418 -0.0166 -0.0123
43 H -0.0048 0.0005 0.0247
44 H -0.0092 -0.0217 -0.0029
45 H -0.0296 -0.0517 -0.0032
46 H 0.0619 -0.0267 0.0134
47 H 0.0255 0.0069 0.0041
48 H 0.0343 -0.0205 -0.0317
49 H 0.0146 0.0291 -0.0045
Vibrational Mode 90
----------------------------------------------------
Harmonic frequency: 1302.70 cm**-1
Reduced mass: 1.7898 amu
Force constant: 1.7895 mdyne/A
IR intensity: 0.7782 km/mol
Normal mode:
X Y Z
1 O 0.0014 0.0016 0.0035
2 O -0.0011 -0.0034 -0.0040
3 O -0.0125 0.0063 -0.0047
4 C -0.0564 -0.0436 0.0032
5 C 0.0972 0.0561 0.0210
6 C -0.0090 -0.0743 0.0165
7 C 0.0428 0.0149 -0.0374
8 C 0.0323 -0.0045 0.0013
9 C -0.0880 0.0470 -0.0564
10 C -0.0056 0.0185 -0.0115
11 C -0.0072 0.0214 0.0053
12 C -0.0188 -0.0178 0.0033
13 C 0.0122 0.0379 -0.0143
14 C 0.0686 -0.1149 -0.0079
15 C -0.0182 0.0114 0.0111
16 C 0.0280 -0.0172 0.0178
17 C 0.0129 -0.0058 0.0035
18 C -0.0137 -0.0114 0.0093
19 C 0.0107 -0.0265 0.0227
20 C 0.0023 0.0170 -0.0072
21 C 0.0366 0.0031 0.0062
22 C -0.0351 0.0181 -0.0125
23 C 0.0082 -0.0091 0.0034
24 H -0.2097 -0.2096 -0.0274
25 H -0.0320 0.2818 0.0115
26 H -0.1223 0.0197 0.1092
27 H -0.1483 0.0932 0.0402
28 H -0.1316 0.0210 0.0032
29 H -0.0332 0.0052 0.0132
30 H 0.0922 -0.0454 0.0218
31 H -0.0421 0.0399 0.0116
32 H -0.0905 0.0905 0.0834
33 H 0.1128 -0.0900 -0.0584
34 H -0.0425 -0.0515 0.0347
35 H -0.0445 -0.0205 0.0597
36 H -0.3206 0.5503 -0.0135
37 H -0.0933 -0.1038 0.0020
38 H 0.2755 0.2154 -0.0148
39 H -0.0107 0.0667 0.0684
40 H -0.0356 -0.0152 -0.0875
41 H -0.0698 0.0393 0.0423
42 H -0.0879 0.0461 0.0128
43 H 0.0042 -0.0032 -0.0114
44 H -0.0773 -0.0466 0.0239
45 H -0.0127 -0.0232 -0.0003
46 H 0.0934 -0.0329 0.0061
47 H 0.0373 0.0160 0.0048
48 H -0.0229 0.0129 0.0171
49 H 0.0161 0.0241 -0.0026
Vibrational Mode 91
----------------------------------------------------
Harmonic frequency: 1310.24 cm**-1
Reduced mass: 1.6465 amu
Force constant: 1.6654 mdyne/A
IR intensity: 0.3998 km/mol
Normal mode:
X Y Z
1 O 0.0012 0.0012 -0.0031
2 O 0.0012 -0.0014 -0.0021
3 O -0.0065 0.0011 -0.0015
4 C 0.0500 0.1336 -0.0721
5 C -0.0256 -0.0787 0.0134
6 C -0.0003 0.0557 0.0060
7 C 0.0212 -0.0591 0.0029
8 C -0.0103 -0.0164 0.0069
9 C 0.0019 0.0464 -0.0206
10 C -0.0122 -0.0257 0.0084
11 C 0.0019 -0.0146 -0.0095
12 C 0.0015 0.0293 0.0054
13 C -0.0140 -0.0420 0.0057
14 C -0.0100 -0.0542 -0.0109
15 C -0.0570 -0.0339 0.0475
16 C 0.0023 -0.0125 0.0032
17 C 0.0053 0.0036 0.0022
18 C -0.0059 -0.0142 0.0092
19 C 0.0133 -0.0081 -0.0012
20 C -0.0019 0.0015 -0.0010
21 C 0.0102 0.0187 -0.0031
22 C -0.0102 0.0037 -0.0031
23 C 0.0026 -0.0047 0.0018
24 H -0.0034 0.0485 0.0119
25 H -0.0672 -0.1880 -0.0544
26 H -0.0556 0.0941 0.0261
27 H -0.2296 0.0987 0.2558
28 H 0.2306 0.0043 0.0388
29 H -0.1775 0.0367 -0.0261
30 H -0.3146 0.2101 -0.0549
31 H 0.3994 -0.1945 0.0884
32 H -0.0052 -0.0290 -0.0051
33 H 0.0143 0.0191 -0.0296
34 H 0.0692 0.0833 -0.0634
35 H 0.0578 0.0354 -0.0550
36 H 0.1692 0.3654 -0.0205
37 H 0.2139 0.0271 0.0665
38 H 0.1977 0.1541 -0.0116
39 H -0.0069 0.0393 0.0012
40 H 0.0086 0.0055 -0.0216
41 H -0.0126 0.0218 0.0411
42 H -0.0228 0.0095 0.0069
43 H -0.0348 0.0201 -0.0193
44 H -0.0134 -0.0181 0.0085
45 H 0.0184 0.0245 0.0033
46 H 0.0643 -0.0347 0.0118
47 H 0.0130 0.0031 0.0031
48 H -0.0078 0.0054 0.0077
49 H 0.0026 -0.0032 0.0004
Vibrational Mode 92
----------------------------------------------------
Harmonic frequency: 1322.32 cm**-1
Reduced mass: 1.3009 amu
Force constant: 1.3402 mdyne/A
IR intensity: 68.9666 km/mol
Normal mode:
X Y Z
1 O 0.0056 0.0191 0.0102
2 O -0.0059 0.0690 0.0397
3 O 0.0027 -0.0000 0.0006
4 C 0.0092 -0.0154 -0.0313
5 C -0.0049 0.0216 0.0107
6 C 0.0109 0.0194 0.0135
7 C -0.0143 0.0052 0.0180
8 C 0.0174 0.0063 0.0145
9 C 0.0008 -0.0021 -0.0054
10 C -0.0160 -0.0335 -0.0754
11 C -0.0078 0.0081 0.0059
12 C 0.0212 -0.0080 -0.0083
13 C -0.0170 -0.0067 0.0013
14 C -0.0013 -0.0104 -0.0014
15 C 0.0016 -0.0001 0.0056
16 C -0.0005 -0.0004 0.0002
17 C -0.0260 -0.0666 -0.0061
18 C 0.0018 0.0001 0.0006
19 C 0.0002 0.0023 -0.0013
20 C -0.0009 -0.0023 0.0011
21 C -0.0062 0.0031 -0.0020
22 C 0.0017 -0.0007 0.0005
23 C -0.0010 -0.0013 0.0001
24 H 0.0030 -0.1429 0.0045
25 H 0.0689 0.0167 0.0566
26 H 0.0050 -0.0169 0.0086
27 H 0.0950 -0.0500 -0.0511
28 H -0.0795 0.0236 0.0155
29 H -0.1299 0.0264 0.0443
30 H -0.0313 0.0173 0.0008
31 H 0.0242 -0.0148 -0.0060
32 H -0.0921 0.0157 0.0755
33 H -0.0191 0.0063 0.0448
34 H 0.0392 0.0282 -0.0350
35 H -0.0034 0.0065 0.0260
36 H 0.0213 0.0722 -0.0039
37 H -0.0193 -0.0170 0.0030
38 H 0.0295 0.0222 -0.0024
39 H -0.0017 0.0030 0.0036
40 H 0.0024 -0.0008 0.0078
41 H -0.0001 0.0040 0.0073
42 H 0.4545 -0.1923 -0.1034
43 H -0.3787 0.1604 0.1949
44 H 0.0145 0.0066 -0.0042
45 H -0.0897 -0.1505 -0.0110
46 H -0.0042 -0.0016 -0.0014
47 H -0.0004 -0.0007 -0.0001
48 H 0.4243 -0.2518 -0.3728
49 H 0.0005 0.0034 -0.0008
Vibrational Mode 93
----------------------------------------------------
Harmonic frequency: 1332.62 cm**-1
Reduced mass: 1.4912 amu
Force constant: 1.5603 mdyne/A
IR intensity: 10.0081 km/mol
Normal mode:
X Y Z
1 O -0.0003 0.0194 0.0163
2 O -0.0016 0.0078 0.0067
3 O 0.0066 -0.0001 0.0014
4 C -0.0050 0.0511 0.0227
5 C -0.0225 -0.0397 -0.0214
6 C -0.0701 -0.1082 -0.0200
7 C 0.0427 -0.0074 -0.0330
8 C -0.0261 0.0025 0.0081
9 C 0.0131 0.0080 0.0193
10 C 0.0678 -0.0059 -0.0473
11 C 0.0193 -0.0215 -0.0063
12 C -0.0253 -0.0031 -0.0079
13 C 0.0454 0.0513 0.0313
14 C -0.0015 0.0108 0.0001
15 C -0.0113 -0.0036 -0.0009
16 C -0.0016 0.0005 -0.0008
17 C -0.0270 0.0030 -0.0035
18 C 0.0063 0.0008 -0.0016
19 C -0.0037 0.0100 -0.0040
20 C 0.0018 -0.0071 0.0030
21 C -0.0171 0.0005 -0.0038
22 C 0.0085 -0.0029 0.0026
23 C -0.0038 -0.0027 -0.0001
24 H -0.0462 0.2092 -0.0136
25 H 0.2391 0.4814 0.2374
26 H -0.0148 -0.0029 0.0140
27 H -0.3064 0.1640 0.1865
28 H 0.0492 0.0148 0.0235
29 H -0.0012 0.0023 -0.0040
30 H -0.0360 0.0449 -0.0132
31 H 0.0160 -0.0049 0.0229
32 H 0.3366 -0.1202 -0.2835
33 H -0.1190 0.0450 0.1045
34 H -0.1479 -0.0420 0.1524
35 H -0.0412 -0.0359 0.0201
36 H 0.0284 -0.0785 0.0021
37 H 0.0610 0.0284 0.0054
38 H -0.0307 -0.0189 -0.0014
39 H 0.0082 -0.0101 -0.0306
40 H -0.0024 0.0028 -0.0154
41 H 0.0035 -0.0092 -0.0146
42 H 0.1264 -0.0852 -0.0128
43 H 0.0293 -0.0168 0.0495
44 H 0.0083 -0.0007 -0.0078
45 H -0.1261 -0.2311 -0.0096
46 H -0.0277 0.0097 0.0012
47 H -0.0018 -0.0025 0.0002
48 H 0.0403 -0.0238 -0.0424
49 H -0.0005 0.0067 -0.0019
Vibrational Mode 94
----------------------------------------------------
Harmonic frequency: 1339.90 cm**-1
Reduced mass: 1.4538 amu
Force constant: 1.5378 mdyne/A
IR intensity: 19.6969 km/mol
Normal mode:
X Y Z
1 O 0.0033 -0.0176 -0.0129
2 O -0.0015 0.0315 -0.0054
3 O 0.0064 0.0013 0.0010
4 C -0.0343 0.0198 0.0344
5 C 0.0032 -0.0300 -0.0031
6 C 0.0113 -0.0392 -0.0075
7 C 0.0194 0.0005 -0.0221
8 C 0.0220 -0.0118 -0.0205
9 C -0.0025 0.0017 0.0056
10 C -0.0517 0.0412 0.0661
11 C 0.0590 -0.0306 -0.0072
12 C 0.0152 -0.0060 -0.0268
13 C 0.0028 0.0184 0.0074
14 C 0.0182 0.0200 0.0052
15 C -0.0281 -0.0029 -0.0043
16 C 0.0011 -0.0002 -0.0028
17 C 0.0801 -0.0906 -0.0026
18 C 0.0032 -0.0020 0.0003
19 C -0.0032 0.0022 0.0011
20 C 0.0011 -0.0003 0.0003
21 C -0.0133 -0.0037 -0.0019
22 C -0.0005 0.0013 -0.0004
23 C -0.0007 -0.0035 0.0006
24 H -0.0330 0.2272 0.0015
25 H -0.1088 0.0611 -0.0901
26 H -0.0261 0.0150 0.0119
27 H -0.1022 0.0577 0.0441
28 H -0.0255 0.0114 -0.0047
29 H 0.0151 -0.0120 -0.0048
30 H -0.1500 0.0562 -0.0331
31 H -0.1486 0.0888 0.0021
32 H -0.0268 0.0085 0.0096
33 H -0.0884 0.0344 0.1215
34 H -0.0084 0.0003 0.0155
35 H -0.0054 0.0067 0.0142
36 H -0.0776 -0.1138 0.0104
37 H 0.1240 0.0663 0.0094
38 H -0.0494 -0.0188 -0.0118
39 H -0.0011 0.0041 0.0023
40 H -0.0004 -0.0037 0.0058
41 H -0.0055 0.0022 -0.0018
42 H -0.4621 0.3010 0.0123
43 H -0.4871 0.2362 0.0845
44 H 0.0170 0.0048 -0.0079
45 H 0.1034 0.2025 0.0046
46 H -0.0112 0.0061 -0.0005
47 H 0.0064 0.0012 0.0016
48 H 0.1967 -0.1167 -0.1800
49 H 0.0050 0.0148 -0.0027
Vibrational Mode 95
----------------------------------------------------
Harmonic frequency: 1342.43 cm**-1
Reduced mass: 1.7613 amu
Force constant: 1.8701 mdyne/A
IR intensity: 4.9825 km/mol
Normal mode:
X Y Z
1 O -0.0022 -0.0066 -0.0015
2 O -0.0010 0.0073 -0.0083
3 O 0.0143 -0.0013 0.0035
4 C 0.0873 -0.0256 -0.0689
5 C -0.0439 0.0404 0.0035
6 C -0.0455 -0.0373 -0.0345
7 C -0.0365 0.0235 0.0504
8 C -0.0770 0.0080 0.0051
9 C 0.0093 -0.0035 -0.0032
10 C 0.0307 0.0295 0.0397
11 C -0.0766 0.0281 0.0114
12 C 0.0090 -0.0231 0.0090
13 C 0.0410 0.0547 -0.0147
14 C -0.0365 -0.0550 -0.0085
15 C 0.0519 0.0260 0.0216
16 C -0.0040 0.0002 -0.0061
17 C 0.0340 -0.0372 -0.0010
18 C 0.0246 0.0208 -0.0086
19 C -0.0089 0.0432 -0.0210
20 C 0.0111 -0.0350 0.0150
21 C -0.0438 0.0091 -0.0106
22 C 0.0400 -0.0147 0.0122
23 C -0.0172 -0.0074 -0.0017
24 H 0.0843 -0.3339 0.0049
25 H 0.1218 -0.0049 0.0952
26 H 0.1517 -0.1161 -0.0783
27 H 0.0539 -0.0290 -0.0307
28 H 0.2949 -0.0587 -0.0077
29 H 0.2893 -0.0542 -0.0528
30 H 0.1027 0.0110 0.0291
31 H 0.1579 -0.1025 0.0028
32 H 0.0434 -0.0610 -0.0250
33 H -0.0994 0.0326 0.1154
34 H -0.0521 -0.0708 0.0555
35 H -0.1513 -0.1447 0.0873
36 H 0.2436 0.2969 -0.0227
37 H -0.2212 -0.1552 -0.0059
38 H 0.0170 0.0157 -0.0116
39 H -0.0096 -0.0145 0.0403
40 H 0.0150 0.0024 0.0276
41 H 0.0266 0.0106 0.0305
42 H -0.3043 0.2032 0.0119
43 H -0.1642 0.0785 0.0167
44 H -0.0739 -0.0436 0.0184
45 H 0.0091 0.0267 -0.0017
46 H -0.1150 0.0292 -0.0156
47 H -0.0213 -0.0125 -0.0027
48 H 0.0448 -0.0270 -0.0466
49 H -0.0135 0.0015 -0.0031
Vibrational Mode 96
----------------------------------------------------
Harmonic frequency: 1349.38 cm**-1
Reduced mass: 1.4221 amu
Force constant: 1.5256 mdyne/A
IR intensity: 0.4107 km/mol
Normal mode:
X Y Z
1 O -0.0028 0.0032 0.0043
2 O -0.0005 -0.0066 -0.0009
3 O 0.0055 0.0012 0.0008
4 C -0.0263 -0.0141 -0.0556
5 C -0.0184 0.0426 0.0084
6 C -0.0247 0.0048 -0.0143
7 C -0.0059 0.0050 0.0226
8 C -0.0332 0.0135 0.0150
9 C -0.0001 -0.0069 -0.0413
10 C 0.0351 -0.0009 -0.0087
11 C 0.1286 -0.0621 0.0129
12 C 0.0031 -0.0152 0.0187
13 C 0.0107 0.0177 -0.0149
14 C 0.0248 -0.0005 0.0110
15 C -0.0403 0.0155 0.0156
16 C -0.0009 -0.0022 0.0013
17 C -0.0138 0.0145 0.0014
18 C 0.0015 -0.0035 0.0045
19 C 0.0006 -0.0023 0.0049
20 C -0.0027 0.0008 0.0002
21 C -0.0097 0.0005 -0.0022
22 C -0.0056 0.0045 -0.0025
23 C 0.0003 -0.0047 0.0011
24 H 0.2859 -0.2884 0.0310
25 H 0.0960 -0.0349 0.0743
26 H 0.0066 -0.0594 0.0312
27 H 0.0804 -0.0412 -0.0237
28 H 0.1117 -0.0172 0.0051
29 H 0.1844 -0.0363 0.0078
30 H -0.5789 0.2676 -0.0923
31 H -0.3602 0.1896 -0.0482
32 H -0.0679 0.0037 0.0696
33 H -0.0029 -0.0083 0.0127
34 H 0.0183 -0.0078 -0.0162
35 H -0.0754 -0.0703 0.0479
36 H -0.1482 0.0710 0.0110
37 H 0.1680 0.0643 0.0296
38 H -0.1230 -0.0212 -0.0684
39 H -0.0090 0.0142 0.0266
40 H 0.0095 -0.0111 0.0570
41 H -0.0054 0.0275 0.0463
42 H 0.0381 -0.0143 -0.0032
43 H 0.0989 -0.0502 -0.0249
44 H 0.0164 0.0026 -0.0070
45 H -0.0351 -0.0637 -0.0025
46 H 0.0049 -0.0074 -0.0052
47 H 0.0156 0.0042 0.0037
48 H -0.0419 0.0244 0.0360
49 H 0.0067 0.0170 -0.0028
Vibrational Mode 97
----------------------------------------------------
Harmonic frequency: 1352.30 cm**-1
Reduced mass: 1.9581 amu
Force constant: 2.1098 mdyne/A
IR intensity: 16.7136 km/mol
Normal mode:
X Y Z
1 O -0.0010 -0.0021 -0.0002
2 O -0.0004 0.0074 -0.0023
3 O -0.0333 -0.0010 -0.0069
4 C 0.0508 -0.0264 -0.0428
5 C -0.0274 0.0423 -0.0084
6 C -0.0153 -0.0252 -0.0311
7 C -0.0251 0.0181 0.0299
8 C -0.0385 0.0068 0.0042
9 C 0.0274 -0.0095 -0.0088
10 C 0.0169 0.0122 0.0175
11 C -0.0432 0.0460 0.0053
12 C -0.0277 0.0155 0.0372
13 C -0.0057 -0.0144 0.0234
14 C 0.0310 0.0673 0.0019
15 C -0.0399 -0.0592 -0.0228
16 C -0.0055 0.0024 0.0038
17 C 0.0154 -0.0220 -0.0023
18 C -0.0582 -0.0513 0.0247
19 C 0.0293 -0.1036 0.0446
20 C -0.0399 0.0757 -0.0323
21 C 0.0997 0.0057 0.0177
22 C -0.0913 0.0271 -0.0263
23 C 0.0428 0.0227 0.0034
24 H 0.0962 -0.3086 -0.0029
25 H 0.1067 0.0984 0.0702
26 H 0.0836 -0.0681 -0.0438
27 H 0.0464 -0.0214 -0.0288
28 H 0.1461 -0.0288 -0.0042
29 H 0.1401 -0.0251 -0.0209
30 H 0.0733 -0.0452 0.0166
31 H 0.2316 -0.1115 0.0108
32 H 0.1979 -0.1274 -0.1547
33 H 0.0773 -0.0387 -0.0570
34 H 0.0757 0.0927 -0.0482
35 H 0.0699 0.0592 -0.0810
36 H -0.3238 -0.2738 0.0171
37 H 0.1687 0.1535 0.0012
38 H 0.1570 0.0477 0.0846
39 H 0.0053 -0.0108 -0.0228
40 H 0.0009 0.0043 0.0003
41 H 0.0094 -0.0112 -0.0107
42 H -0.1347 0.0843 0.0046
43 H -0.1119 0.0550 0.0289
44 H 0.2947 0.1667 -0.0843
45 H -0.0047 -0.0005 -0.0029
46 H 0.2827 -0.0913 0.0401
47 H 0.0362 0.0219 0.0038
48 H 0.0441 -0.0260 -0.0431
49 H 0.0270 -0.0240 0.0117
Vibrational Mode 98
----------------------------------------------------
Harmonic frequency: 1358.76 cm**-1
Reduced mass: 1.9337 amu
Force constant: 2.1034 mdyne/A
IR intensity: 16.0232 km/mol
Normal mode:
X Y Z
1 O 0.0001 -0.0004 -0.0007
2 O 0.0004 0.0040 -0.0001
3 O -0.0486 -0.0086 -0.0076
4 C -0.0293 0.0076 0.0041
5 C 0.0225 -0.0135 -0.0087
6 C 0.0051 0.0042 -0.0051
7 C 0.0011 -0.0005 0.0007
8 C 0.0053 0.0004 0.0025
9 C 0.0363 0.0238 0.0130
10 C -0.0020 -0.0002 0.0047
11 C 0.0316 -0.0460 -0.0002
12 C -0.0228 0.0150 0.0073
13 C -0.0037 -0.0172 0.0134
14 C -0.1248 -0.0634 -0.0088
15 C 0.1025 0.0873 0.0267
16 C -0.0058 0.0004 0.0154
17 C 0.0053 -0.0078 -0.0013
18 C -0.0504 -0.0347 0.0188
19 C 0.0259 -0.0710 0.0083
20 C -0.0328 0.0442 -0.0204
21 C 0.1166 0.0468 0.0117
22 C -0.0463 -0.0003 -0.0101
23 C 0.0328 0.0316 -0.0001
24 H -0.2050 0.0567 -0.0315
25 H 0.0106 0.0069 0.0005
26 H 0.0035 0.0012 -0.0026
27 H 0.0206 -0.0139 -0.0273
28 H 0.0059 -0.0186 -0.0113
29 H 0.0048 0.0052 -0.0199
30 H -0.1134 0.0697 -0.0199
31 H -0.2653 0.1116 -0.0418
32 H 0.1349 -0.0414 -0.1138
33 H 0.0518 -0.0240 -0.0511
34 H -0.0003 0.0193 0.0038
35 H 0.0605 0.0481 -0.0588
36 H 0.4481 0.2394 -0.0210
37 H -0.2873 -0.2109 -0.0135
38 H -0.3354 -0.1788 -0.0856
39 H 0.0217 -0.0118 -0.0882
40 H -0.0064 0.0314 -0.0988
41 H 0.0147 -0.0449 -0.0568
42 H -0.0330 0.0127 0.0033
43 H -0.0576 0.0299 0.0194
44 H 0.2054 0.1231 -0.0566
45 H 0.0028 0.0058 -0.0001
46 H 0.2540 -0.1017 0.0432
47 H 0.0099 -0.0024 0.0056
48 H 0.0234 -0.0134 -0.0221
49 H -0.0066 -0.0979 0.0223
Vibrational Mode 99
----------------------------------------------------
Harmonic frequency: 1359.88 cm**-1
Reduced mass: 1.3892 amu
Force constant: 1.5136 mdyne/A
IR intensity: 2.5669 km/mol
Normal mode:
X Y Z
1 O -0.0011 -0.0020 -0.0011
2 O -0.0004 0.0030 -0.0009
3 O 0.0083 0.0009 0.0015
4 C -0.0098 -0.0111 0.0323
5 C -0.0196 -0.0027 -0.0116
6 C 0.0027 -0.0285 -0.0461
7 C -0.0044 0.0075 0.0035
8 C 0.0023 0.0010 -0.0074
9 C -0.0075 0.0067 -0.0368
10 C 0.0115 0.0088 0.0148
11 C 0.0007 -0.0029 -0.0035
12 C -0.0217 0.0500 0.0386
13 C -0.0756 -0.0851 0.0686
14 C 0.0019 -0.0263 0.0027
15 C 0.0132 0.0157 0.0049
16 C -0.0030 -0.0058 -0.0103
17 C 0.0067 -0.0090 -0.0036
18 C 0.0168 0.0157 -0.0056
19 C -0.0086 0.0320 -0.0103
20 C 0.0122 -0.0217 0.0094
21 C -0.0242 -0.0074 -0.0028
22 C 0.0269 -0.0061 0.0072
23 C -0.0139 -0.0075 -0.0012
24 H 0.3792 0.0424 0.0393
25 H 0.1271 0.2622 0.0684
26 H 0.0446 0.0024 -0.0458
27 H -0.0018 -0.0058 -0.0679
28 H -0.0553 0.0153 0.0011
29 H 0.0164 -0.0105 0.0230
30 H 0.0466 -0.0311 0.0057
31 H -0.0640 0.0331 -0.0028
32 H 0.1131 -0.1010 -0.0953
33 H 0.2468 -0.0924 -0.2202
34 H 0.2934 0.3362 -0.2255
35 H 0.2761 0.2686 -0.2269
36 H 0.0005 0.1505 -0.0039
37 H -0.0727 -0.0484 -0.0045
38 H -0.0217 0.0002 -0.0240
39 H -0.0310 0.0300 0.0944
40 H 0.0404 0.0010 0.0798
41 H 0.0170 0.0393 0.0752
42 H -0.0695 0.0386 0.0030
43 H -0.0433 0.0222 0.0181
44 H -0.1522 -0.0855 0.0488
45 H -0.0022 0.0014 -0.0026
46 H -0.0787 0.0229 -0.0162
47 H -0.0107 -0.0044 -0.0018
48 H 0.0176 -0.0106 -0.0176
49 H -0.0087 0.0090 -0.0039
Vibrational Mode 100
----------------------------------------------------
Harmonic frequency: 1374.10 cm**-1
Reduced mass: 1.3733 amu
Force constant: 1.5277 mdyne/A
IR intensity: 0.2718 km/mol
Normal mode:
X Y Z
1 O 0.0011 -0.0013 -0.0022
2 O 0.0014 0.0080 0.0010
3 O 0.0066 0.0018 0.0008
4 C -0.0192 -0.0126 -0.0064
5 C 0.0233 0.0336 -0.0269
6 C 0.0245 0.0506 0.0405
7 C 0.0023 -0.0058 -0.0078
8 C 0.0127 0.0027 0.0054
9 C -0.0116 -0.0020 0.0175
10 C -0.0256 -0.0083 -0.0047
11 C 0.0158 -0.0009 0.0021
12 C -0.0985 0.0441 0.0697
13 C 0.0467 -0.0100 -0.0267
14 C 0.0123 0.0004 -0.0005
15 C -0.0131 -0.0042 0.0002
16 C 0.0059 0.0013 0.0052
17 C 0.0081 -0.0118 -0.0008
18 C 0.0139 0.0179 -0.0108
19 C -0.0089 0.0277 -0.0087
20 C 0.0120 -0.0169 0.0073
21 C -0.0191 -0.0146 0.0001
22 C 0.0230 -0.0030 0.0057
23 C -0.0132 -0.0077 -0.0010
24 H -0.0890 -0.1807 -0.0402
25 H -0.1321 -0.2398 -0.0966
26 H -0.0624 0.0200 0.0486
27 H 0.0539 -0.0231 0.0085
28 H -0.0132 -0.0310 -0.0232
29 H -0.0204 0.0178 -0.0331
30 H -0.0579 0.0112 -0.0080
31 H -0.0403 0.0245 -0.0087
32 H 0.4773 -0.2708 -0.4001
33 H 0.3070 -0.1823 -0.2083
34 H -0.2130 -0.2160 0.1621
35 H 0.0122 -0.0406 -0.0453
36 H -0.0020 -0.0230 0.0007
37 H 0.0346 0.0207 0.0040
38 H 0.0223 0.0214 -0.0061
39 H 0.0145 -0.0075 -0.0392
40 H -0.0264 -0.0067 -0.0395
41 H -0.0217 -0.0109 -0.0311
42 H -0.0137 -0.0250 0.0120
43 H -0.1109 0.0609 0.0487
44 H -0.1334 -0.0661 0.0469
45 H 0.0214 0.0341 0.0036
46 H -0.0793 0.0323 -0.0103
47 H -0.0103 -0.0016 -0.0025
48 H 0.0475 -0.0267 -0.0413
49 H -0.0071 0.0131 -0.0047
Vibrational Mode 101
----------------------------------------------------
Harmonic frequency: 1376.68 cm**-1
Reduced mass: 1.3535 amu
Force constant: 1.5113 mdyne/A
IR intensity: 0.9987 km/mol
Normal mode:
X Y Z
1 O 0.0019 -0.0006 -0.0016
2 O 0.0007 0.0010 0.0010
3 O -0.0059 -0.0019 -0.0007
4 C -0.0039 0.0052 0.0571
5 C -0.0848 -0.0114 -0.0145
6 C 0.0092 0.0101 0.0342
7 C 0.0182 -0.0123 -0.0282
8 C 0.0299 -0.0060 -0.0141
9 C 0.0262 0.0109 -0.0775
10 C -0.0237 -0.0019 -0.0079
11 C -0.0224 0.0027 -0.0081
12 C -0.0036 -0.0048 0.0184
13 C 0.0400 0.0369 -0.0364
14 C -0.0069 -0.0173 0.0059
15 C 0.0240 0.0102 0.0018
16 C -0.0120 -0.0054 0.0008
17 C 0.0005 0.0000 0.0010
18 C -0.0117 -0.0187 0.0145
19 C 0.0086 -0.0226 0.0106
20 C -0.0129 0.0132 -0.0053
21 C 0.0179 0.0137 -0.0002
22 C -0.0193 0.0033 -0.0050
23 C 0.0106 0.0059 0.0007
24 H 0.7858 0.0978 0.0976
25 H -0.1212 -0.1073 -0.0746
26 H -0.0559 0.0602 0.0189
27 H -0.0697 0.0346 0.0335
28 H -0.1642 0.0590 0.0187
29 H -0.1000 0.0041 0.0588
30 H 0.1470 -0.0750 0.0197
31 H 0.0245 -0.0172 0.0044
32 H 0.0485 -0.0896 -0.0406
33 H -0.0797 0.0304 0.1018
34 H -0.1500 -0.1657 0.1135
35 H -0.1005 -0.1026 0.0882
36 H -0.0903 0.1652 -0.0016
37 H -0.0904 -0.0382 -0.0096
38 H -0.0324 -0.0202 -0.0181
39 H -0.0216 0.0127 0.0585
40 H 0.0521 0.0161 0.0822
41 H 0.0446 0.0368 0.0993
42 H 0.0334 -0.0295 0.0015
43 H -0.0107 0.0070 0.0080
44 H 0.0736 0.0273 -0.0307
45 H 0.0201 0.0301 0.0038
46 H 0.0767 -0.0392 0.0045
47 H 0.0110 0.0022 0.0023
48 H 0.0072 -0.0038 -0.0041
49 H 0.0054 -0.0114 0.0040
Vibrational Mode 102
----------------------------------------------------
Harmonic frequency: 1403.85 cm**-1
Reduced mass: 1.2301 amu
Force constant: 1.4283 mdyne/A
IR intensity: 5.9170 km/mol
Normal mode:
X Y Z
1 O -0.0001 -0.0007 -0.0004
2 O -0.0000 0.0003 -0.0001
3 O 0.0030 0.0014 0.0003
4 C 0.0058 -0.0012 0.0071
5 C -0.0185 -0.0002 0.0020
6 C -0.0024 -0.0034 -0.0067
7 C -0.0052 0.0046 0.0008
8 C -0.0007 -0.0008 -0.0042
9 C 0.0006 0.0013 -0.0238
10 C 0.0002 0.0020 0.0030
11 C -0.0012 0.0008 -0.0021
12 C 0.0051 -0.0072 0.0079
13 C -0.0045 -0.0022 0.0019
14 C 0.0097 -0.0078 0.0051
15 C -0.0088 0.0061 -0.0072
16 C -0.0009 0.0183 0.1322
17 C 0.0008 -0.0016 0.0001
18 C 0.0018 0.0050 0.0002
19 C -0.0063 0.0122 -0.0025
20 C 0.0069 -0.0056 0.0031
21 C -0.0081 -0.0059 0.0003
22 C 0.0129 0.0005 0.0026
23 C -0.0083 -0.0059 -0.0003
24 H 0.1793 0.0076 0.0248
25 H 0.0093 0.0078 0.0028
26 H 0.0434 -0.0415 -0.0283
27 H 0.0227 -0.0051 0.0144
28 H -0.0097 0.0205 0.0120
29 H 0.0008 -0.0086 0.0265
30 H 0.0073 0.0041 0.0001
31 H 0.0029 0.0035 0.0104
32 H 0.0024 0.0596 0.0211
33 H -0.0233 0.0283 -0.0382
34 H 0.0428 0.0346 -0.0313
35 H 0.0034 0.0045 -0.0048
36 H -0.0512 0.0554 0.0018
37 H 0.0616 -0.0430 0.0042
38 H 0.0220 -0.0096 0.0863
39 H 0.1537 -0.0742 -0.5283
40 H -0.1380 0.1046 -0.5482
41 H -0.0467 -0.2875 -0.4286
42 H -0.0056 0.0102 -0.0026
43 H -0.0064 0.0023 -0.0032
44 H -0.0321 -0.0171 0.0053
45 H 0.0027 0.0052 0.0001
46 H -0.0254 0.0078 -0.0094
47 H -0.0073 0.0016 -0.0020
48 H 0.0019 -0.0011 -0.0013
49 H -0.0041 0.0097 -0.0035
Vibrational Mode 103
----------------------------------------------------
Harmonic frequency: 1446.49 cm**-1
Reduced mass: 7.1726 amu
Force constant: 8.8421 mdyne/A
IR intensity: 7.5150 km/mol
Normal mode:
X Y Z
1 O -0.0000 0.0001 0.0000
2 O -0.0002 0.0002 0.0002
3 O -0.0586 0.0358 -0.0242
4 C 0.0003 -0.0013 -0.0017
5 C -0.0106 0.0010 -0.0009
6 C -0.0005 -0.0017 -0.0002
7 C -0.0004 0.0006 0.0004
8 C -0.0003 0.0004 -0.0000
9 C 0.0366 0.0244 -0.0074
10 C 0.0002 0.0001 -0.0002
11 C 0.0009 -0.0057 -0.0000
12 C 0.0049 -0.0057 -0.0046
13 C 0.0022 0.0064 0.0001
14 C 0.0831 -0.0263 0.0306
15 C -0.0221 -0.0038 -0.0011
16 C -0.0074 -0.0141 -0.0105
17 C -0.0001 -0.0007 0.0008
18 C -0.1105 -0.0683 0.0327
19 C -0.2324 0.2214 -0.1135
20 C -0.0751 0.1972 -0.0848
21 C 0.2536 -0.3722 0.1498
22 C 0.3005 -0.0007 0.0656
23 C -0.1788 -0.0582 -0.0243
24 H 0.0138 0.0015 0.0023
25 H 0.0013 0.0037 0.0015
26 H 0.0006 -0.0028 0.0004
27 H 0.0031 -0.0014 -0.0011
28 H 0.0009 0.0022 0.0017
29 H 0.0025 -0.0013 0.0038
30 H 0.0065 0.0369 0.0010
31 H -0.0121 0.0157 0.0325
32 H -0.0101 0.0094 0.0095
33 H -0.0539 0.0273 0.0409
34 H -0.0031 -0.0163 0.0062
35 H -0.0125 -0.0109 -0.0015
36 H 0.1402 0.1105 0.0113
37 H 0.0331 -0.0099 0.0051
38 H 0.0560 0.0406 0.0138
39 H -0.0301 0.0825 0.0361
40 H 0.0869 0.0388 0.0534
41 H 0.0039 0.0146 0.0314
42 H 0.0046 0.0070 -0.0044
43 H -0.0016 -0.0008 -0.0079
44 H 0.3503 0.2212 -0.1337
45 H -0.0000 -0.0004 0.0001
46 H 0.2562 0.0412 0.0329
47 H -0.2759 0.0195 -0.0730
48 H 0.0009 -0.0006 -0.0004
49 H -0.1532 0.0784 -0.0494
Vibrational Mode 104
----------------------------------------------------
Harmonic frequency: 1459.27 cm**-1
Reduced mass: 1.0833 amu
Force constant: 1.3591 mdyne/A
IR intensity: 0.9733 km/mol
Normal mode:
X Y Z
1 O 0.0002 0.0006 -0.0008
2 O -0.0049 0.0015 0.0094
3 O 0.0001 -0.0002 0.0001
4 C -0.0016 0.0009 0.0028
5 C -0.0001 -0.0018 -0.0016
6 C 0.0005 -0.0022 0.0005
7 C -0.0003 0.0036 -0.0077
8 C 0.0030 0.0006 0.0031
9 C -0.0008 -0.0004 0.0005
10 C -0.0016 0.0010 0.0066
11 C -0.0002 -0.0004 -0.0010
12 C -0.0030 0.0036 0.0008
13 C -0.0048 0.0184 0.0208
14 C -0.0007 0.0002 -0.0003
15 C -0.0006 0.0009 -0.0010
16 C -0.0030 -0.0037 -0.0003
17 C -0.0152 -0.0387 0.0628
18 C 0.0002 0.0003 -0.0001
19 C 0.0005 -0.0006 0.0002
20 C 0.0005 -0.0007 0.0003
21 C -0.0008 0.0014 -0.0006
22 C -0.0013 -0.0006 -0.0002
23 C 0.0011 0.0008 0.0000
24 H -0.0003 0.0103 -0.0009
25 H -0.0060 0.0092 -0.0032
26 H -0.0138 -0.0465 0.0262
27 H 0.0263 0.0006 0.0538
28 H -0.0246 -0.0305 -0.0230
29 H 0.0039 0.0116 -0.0409
30 H 0.0049 0.0041 0.0002
31 H 0.0026 0.0014 0.0074
32 H 0.0340 -0.0126 -0.0260
33 H 0.0047 -0.0009 -0.0025
34 H 0.1221 -0.1741 -0.0355
35 H -0.0495 -0.0636 -0.1895
36 H 0.0012 -0.0011 -0.0001
37 H 0.0068 -0.0103 0.0003
38 H 0.0012 -0.0035 0.0128
39 H -0.0127 0.0367 0.0182
40 H 0.0415 0.0286 0.0003
41 H 0.0130 -0.0156 -0.0142
42 H 0.3406 0.5027 -0.3192
43 H -0.0875 -0.0715 -0.6345
44 H -0.0008 -0.0004 0.0000
45 H 0.0014 0.0042 -0.0002
46 H -0.0007 -0.0001 -0.0002
47 H 0.0005 -0.0007 0.0005
48 H -0.0021 -0.0008 0.0123
49 H 0.0007 -0.0013 0.0005
Vibrational Mode 105
----------------------------------------------------
Harmonic frequency: 1467.69 cm**-1
Reduced mass: 1.0956 amu
Force constant: 1.3905 mdyne/A
IR intensity: 1.2247 km/mol
Normal mode:
X Y Z
1 O -0.0006 -0.0015 -0.0002
2 O 0.0005 -0.0007 -0.0009
3 O -0.0000 0.0003 -0.0001
4 C -0.0001 0.0071 0.0013
5 C -0.0028 -0.0039 -0.0011
6 C 0.0069 0.0012 -0.0025
7 C -0.0235 0.0387 -0.0543
8 C -0.0041 -0.0147 -0.0425
9 C 0.0082 -0.0009 0.0007
10 C -0.0017 0.0007 0.0004
11 C -0.0030 -0.0023 -0.0014
12 C -0.0002 -0.0059 0.0050
13 C -0.0055 0.0012 0.0095
14 C -0.0030 0.0003 -0.0014
15 C 0.0049 -0.0018 0.0032
16 C 0.0202 -0.0044 -0.0030
17 C 0.0012 0.0024 -0.0037
18 C 0.0006 0.0001 0.0001
19 C 0.0021 -0.0008 0.0009
20 C -0.0013 -0.0003 0.0002
21 C -0.0002 0.0004 -0.0001
22 C 0.0014 0.0024 -0.0002
23 C -0.0025 -0.0025 0.0001
24 H -0.0141 0.0145 -0.0026
25 H -0.0064 0.0100 -0.0136
26 H -0.0752 -0.4682 0.2013
27 H 0.3188 -0.0364 0.4493
28 H 0.0842 0.2997 0.2079
29 H -0.0124 -0.1054 0.3512
30 H 0.0269 0.0040 0.0035
31 H 0.0076 -0.0033 0.0109
32 H 0.0418 0.0591 -0.0155
33 H -0.0332 0.0387 -0.0692
34 H 0.0434 -0.0464 -0.0177
35 H -0.0067 -0.0121 -0.0769
36 H 0.0065 0.0040 -0.0015
37 H -0.0455 0.0140 -0.0037
38 H -0.0086 0.0058 -0.0387
39 H 0.0219 0.0651 -0.1235
40 H -0.0977 -0.1168 0.1259
41 H -0.2078 0.1070 0.0393
42 H -0.0251 -0.0245 0.0184
43 H 0.0090 0.0028 0.0350
44 H 0.0037 0.0005 -0.0022
45 H 0.0023 0.0086 -0.0016
46 H -0.0006 -0.0006 0.0005
47 H 0.0008 0.0028 -0.0008
48 H -0.0025 0.0017 0.0022
49 H -0.0012 0.0032 -0.0010
Vibrational Mode 106
----------------------------------------------------
Harmonic frequency: 1475.44 cm**-1
Reduced mass: 1.0937 amu
Force constant: 1.4028 mdyne/A
IR intensity: 2.7580 km/mol
Normal mode:
X Y Z
1 O 0.0000 0.0008 -0.0008
2 O 0.0003 0.0001 -0.0001
3 O -0.0003 -0.0012 0.0003
4 C 0.0041 -0.0113 -0.0091
5 C 0.0060 0.0047 0.0005
6 C -0.0008 0.0047 0.0047
7 C 0.0108 -0.0205 0.0317
8 C -0.0096 -0.0163 -0.0602
9 C -0.0091 -0.0010 0.0035
10 C -0.0043 -0.0057 -0.0024
11 C 0.0026 0.0041 0.0046
12 C -0.0037 -0.0242 0.0183
13 C 0.0022 0.0044 0.0040
14 C 0.0043 -0.0009 0.0014
15 C -0.0029 0.0034 -0.0030
16 C -0.0204 0.0025 -0.0057
17 C 0.0005 -0.0017 0.0029
18 C -0.0001 0.0016 -0.0009
19 C -0.0063 0.0029 -0.0027
20 C 0.0015 -0.0008 0.0002
21 C 0.0005 0.0009 -0.0002
22 C -0.0037 -0.0074 0.0009
23 C 0.0073 0.0074 -0.0002
24 H -0.0543 -0.0216 -0.0082
25 H -0.0071 -0.0193 0.0003
26 H 0.0365 0.2569 -0.1043
27 H -0.1650 0.0130 -0.2565
28 H 0.1278 0.4144 0.2874
29 H 0.0023 -0.1561 0.5069
30 H -0.0280 -0.0253 -0.0012
31 H -0.0282 0.0016 -0.0413
32 H 0.1315 0.2097 -0.0378
33 H -0.0924 0.1085 -0.2139
34 H 0.0175 -0.0669 0.0037
35 H -0.0221 -0.0313 -0.0532
36 H 0.0018 -0.0041 0.0015
37 H 0.0289 -0.0143 0.0018
38 H 0.0049 -0.0041 0.0287
39 H -0.0338 -0.0503 0.1597
40 H 0.1183 0.1145 -0.0803
41 H 0.2179 -0.0922 -0.0134
42 H 0.0097 0.0222 -0.0122
43 H -0.0187 0.0058 -0.0276
44 H -0.0075 -0.0034 0.0005
45 H 0.0067 0.0019 0.0027
46 H -0.0011 0.0011 0.0002
47 H -0.0007 -0.0085 0.0022
48 H 0.0006 0.0001 0.0004
49 H 0.0029 -0.0111 0.0034
Vibrational Mode 107
----------------------------------------------------
Harmonic frequency: 1477.90 cm**-1
Reduced mass: 5.5740 amu
Force constant: 7.1731 mdyne/A
IR intensity: 19.6912 km/mol
Normal mode:
X Y Z
1 O 0.0001 -0.0001 0.0003
2 O 0.0004 -0.0002 -0.0006
3 O -0.0207 -0.0546 0.0095
4 C -0.0007 -0.0005 -0.0001
5 C -0.0047 0.0043 -0.0004
6 C 0.0014 0.0006 0.0005
7 C -0.0046 0.0078 -0.0123
8 C 0.0013 0.0026 0.0083
9 C 0.0024 0.0056 0.0009
10 C 0.0005 0.0004 -0.0008
11 C -0.0036 -0.0093 -0.0046
12 C 0.0012 0.0026 -0.0026
13 C -0.0024 0.0040 0.0045
14 C 0.1282 -0.0063 0.0297
15 C -0.0307 0.0124 -0.0186
16 C -0.0056 -0.0098 -0.0021
17 C 0.0007 0.0031 -0.0046
18 C -0.0179 -0.0005 0.0020
19 C -0.3024 0.1210 -0.1046
20 C 0.0142 -0.0301 0.0086
21 C 0.0341 0.0731 -0.0128
22 C -0.1032 -0.3034 0.0513
23 C 0.2776 0.2881 -0.0102
24 H 0.0073 -0.0020 -0.0001
25 H -0.0004 0.0038 -0.0009
26 H -0.0263 -0.0974 0.0517
27 H 0.0703 -0.0091 0.0954
28 H -0.0155 -0.0548 -0.0382
29 H -0.0004 0.0207 -0.0659
30 H 0.0418 0.0777 0.0020
31 H -0.0066 0.0286 0.0797
32 H -0.0190 -0.0247 0.0066
33 H 0.0146 -0.0149 0.0222
34 H 0.0270 -0.0396 -0.0094
35 H -0.0140 -0.0161 -0.0506
36 H 0.0526 0.0305 0.0097
37 H 0.1423 -0.2024 0.0096
38 H 0.0663 -0.0243 0.2293
39 H -0.0258 0.0840 0.0334
40 H 0.0923 0.0615 0.0028
41 H 0.0216 -0.0232 -0.0181
42 H -0.0225 -0.0384 0.0234
43 H 0.0095 0.0038 0.0491
44 H -0.0273 -0.0087 0.0069
45 H -0.0025 -0.0015 -0.0010
46 H 0.0533 -0.0451 0.0175
47 H -0.0417 -0.3423 0.0736
48 H -0.0003 0.0004 -0.0009
49 H 0.0933 -0.4694 0.1333
Vibrational Mode 108
----------------------------------------------------
Harmonic frequency: 1484.78 cm**-1
Reduced mass: 1.1079 amu
Force constant: 1.4390 mdyne/A
IR intensity: 0.8134 km/mol
Normal mode:
X Y Z
1 O 0.0002 0.0001 0.0007
2 O 0.0010 -0.0001 -0.0017
3 O 0.0024 0.0039 -0.0004
4 C -0.0023 -0.0004 -0.0010
5 C 0.0058 -0.0012 0.0006
6 C 0.0021 0.0023 0.0033
7 C -0.0056 0.0092 -0.0156
8 C 0.0015 0.0026 0.0093
9 C -0.0087 -0.0097 -0.0003
10 C 0.0003 0.0000 -0.0033
11 C -0.0051 -0.0112 -0.0118
12 C -0.0009 0.0028 -0.0008
13 C -0.0065 0.0195 0.0194
14 C -0.0167 0.0038 -0.0038
15 C -0.0151 0.0312 -0.0312
16 C -0.0306 -0.0333 -0.0005
17 C 0.0025 0.0078 -0.0124
18 C 0.0013 0.0027 -0.0005
19 C 0.0296 -0.0124 0.0098
20 C 0.0027 -0.0000 0.0004
21 C -0.0061 -0.0010 -0.0010
22 C 0.0036 0.0225 -0.0050
23 C -0.0186 -0.0205 0.0009
24 H -0.0055 -0.0025 -0.0014
25 H -0.0019 0.0049 0.0005
26 H -0.0328 -0.1224 0.0657
27 H 0.0885 -0.0126 0.1180
28 H -0.0145 -0.0588 -0.0403
29 H -0.0039 0.0226 -0.0696
30 H 0.0732 0.1366 0.0009
31 H 0.0005 0.0518 0.1461
32 H -0.0160 0.0092 0.0112
33 H 0.0028 0.0003 -0.0031
34 H 0.1202 -0.1907 -0.0370
35 H -0.0658 -0.0800 -0.2276
36 H -0.0040 -0.0108 -0.0022
37 H 0.1978 -0.3101 0.0087
38 H 0.0269 -0.1025 0.3654
39 H -0.1144 0.3200 0.1735
40 H 0.4071 0.2882 -0.0165
41 H 0.1418 -0.1504 -0.1302
42 H -0.0626 -0.1033 0.0639
43 H 0.0203 0.0135 0.1366
44 H -0.0026 0.0009 0.0002
45 H -0.0041 -0.0037 -0.0013
46 H -0.0080 0.0046 -0.0048
47 H -0.0001 0.0259 -0.0038
48 H 0.0017 -0.0003 -0.0038
49 H -0.0054 0.0335 -0.0089
Vibrational Mode 109
----------------------------------------------------
Harmonic frequency: 1493.83 cm**-1
Reduced mass: 1.0875 amu
Force constant: 1.4298 mdyne/A
IR intensity: 1.2704 km/mol
Normal mode:
X Y Z
1 O -0.0003 0.0002 -0.0004
2 O -0.0007 -0.0005 0.0010
3 O 0.0004 0.0008 -0.0001
4 C -0.0051 -0.0090 -0.0077
5 C 0.0008 0.0074 -0.0005
6 C 0.0009 0.0008 -0.0020
7 C 0.0006 -0.0041 0.0127
8 C 0.0009 -0.0024 -0.0112
9 C 0.0047 0.0016 0.0005
10 C -0.0005 -0.0019 0.0012
11 C -0.0221 -0.0519 -0.0416
12 C -0.0008 0.0080 -0.0051
13 C 0.0048 -0.0153 -0.0168
14 C -0.0025 0.0052 -0.0021
15 C -0.0053 0.0127 -0.0184
16 C 0.0127 0.0114 -0.0049
17 C -0.0018 -0.0034 0.0073
18 C 0.0015 -0.0008 -0.0003
19 C 0.0058 -0.0041 0.0038
20 C -0.0024 0.0004 -0.0001
21 C -0.0004 0.0004 -0.0001
22 C 0.0017 0.0060 -0.0011
23 C -0.0050 -0.0052 0.0002
24 H -0.0105 -0.0325 -0.0050
25 H 0.0022 -0.0094 -0.0017
26 H 0.0081 0.0725 -0.0269
27 H -0.0199 -0.0116 -0.0924
28 H 0.0265 0.0852 0.0590
29 H -0.0117 -0.0288 0.1065
30 H 0.2877 0.5180 0.0078
31 H -0.0151 0.1902 0.5543
32 H -0.0301 -0.0997 -0.0034
33 H 0.0444 -0.0504 0.0787
34 H -0.1077 0.1509 0.0350
35 H 0.0545 0.0678 0.1837
36 H -0.0282 -0.0217 -0.0023
37 H 0.0850 -0.1651 0.0004
38 H 0.0097 -0.0598 0.1801
39 H 0.0384 -0.1130 -0.0534
40 H -0.1535 -0.1186 0.0341
41 H -0.0664 0.0825 0.0807
42 H 0.0376 0.0593 -0.0373
43 H -0.0034 -0.0123 -0.0846
44 H 0.0026 0.0002 0.0007
45 H 0.0024 0.0007 0.0010
46 H 0.0004 -0.0011 0.0013
47 H -0.0016 0.0071 -0.0014
48 H -0.0038 0.0017 0.0046
49 H -0.0017 0.0086 -0.0025
Vibrational Mode 110
----------------------------------------------------
Harmonic frequency: 1496.95 cm**-1
Reduced mass: 1.0869 amu
Force constant: 1.4350 mdyne/A
IR intensity: 0.6511 km/mol
Normal mode:
X Y Z
1 O -0.0004 -0.0002 -0.0002
2 O -0.0014 -0.0018 0.0012
3 O -0.0002 0.0004 -0.0001
4 C 0.0030 -0.0045 -0.0002
5 C -0.0004 0.0049 -0.0029
6 C -0.0012 -0.0049 -0.0023
7 C 0.0020 -0.0023 0.0072
8 C -0.0044 -0.0070 -0.0236
9 C 0.0046 -0.0031 0.0043
10 C 0.0008 0.0014 0.0003
11 C 0.0102 0.0272 0.0202
12 C 0.0010 0.0425 -0.0314
13 C 0.0064 -0.0151 -0.0201
14 C -0.0075 -0.0008 -0.0010
15 C -0.0103 0.0237 -0.0192
16 C 0.0062 -0.0183 0.0055
17 C -0.0025 -0.0015 0.0076
18 C -0.0005 0.0001 0.0001
19 C 0.0024 -0.0016 0.0001
20 C 0.0006 -0.0009 0.0005
21 C 0.0002 0.0026 -0.0006
22 C 0.0025 0.0019 -0.0000
23 C -0.0028 -0.0026 0.0001
24 H -0.0427 0.0084 -0.0093
25 H 0.0030 0.0034 0.0005
26 H 0.0175 0.0497 -0.0329
27 H -0.0416 0.0082 -0.0502
28 H 0.0440 0.1752 0.1206
29 H 0.0104 -0.0621 0.1967
30 H -0.1319 -0.2382 -0.0030
31 H -0.0102 -0.0767 -0.2599
32 H -0.1762 -0.4154 0.0144
33 H 0.1770 -0.2045 0.3625
34 H -0.1359 0.1815 0.0453
35 H 0.0629 0.0832 0.2173
36 H 0.0264 0.0042 -0.0005
37 H 0.1274 -0.2151 0.0075
38 H 0.0206 -0.0610 0.2414
39 H -0.0209 0.1993 -0.0732
40 H 0.0781 0.0320 0.0517
41 H -0.1529 0.0134 -0.0609
42 H 0.0411 0.0588 -0.0382
43 H 0.0263 -0.0280 -0.0933
44 H 0.0012 0.0034 0.0025
45 H 0.0018 0.0010 0.0005
46 H 0.0001 -0.0008 -0.0006
47 H -0.0048 0.0028 -0.0006
48 H -0.0100 0.0047 0.0101
49 H -0.0017 0.0025 -0.0009
Vibrational Mode 111
----------------------------------------------------
Harmonic frequency: 1502.41 cm**-1
Reduced mass: 1.0932 amu
Force constant: 1.4538 mdyne/A
IR intensity: 0.2373 km/mol
Normal mode:
X Y Z
1 O -0.0001 0.0001 0.0002
2 O -0.0002 -0.0014 -0.0005
3 O 0.0001 -0.0013 0.0003
4 C -0.0014 -0.0014 -0.0015
5 C 0.0038 0.0036 -0.0015
6 C -0.0006 -0.0013 -0.0001
7 C -0.0004 -0.0009 0.0063
8 C -0.0007 -0.0040 -0.0118
9 C -0.0060 -0.0035 0.0047
10 C 0.0003 0.0005 -0.0027
11 C -0.0074 -0.0214 -0.0147
12 C 0.0005 0.0344 -0.0236
13 C -0.0039 0.0152 0.0116
14 C 0.0090 0.0017 0.0014
15 C 0.0191 -0.0414 0.0359
16 C -0.0126 -0.0195 0.0152
17 C 0.0002 0.0051 -0.0045
18 C -0.0020 0.0002 0.0002
19 C -0.0033 0.0046 -0.0015
20 C 0.0036 -0.0000 -0.0000
21 C -0.0025 -0.0021 -0.0000
22 C -0.0074 -0.0070 0.0002
23 C 0.0089 0.0077 0.0000
24 H -0.0356 -0.0038 -0.0078
25 H 0.0037 0.0052 0.0044
26 H 0.0300 0.0167 -0.0306
27 H -0.0096 -0.0043 -0.0384
28 H 0.0237 0.0952 0.0660
29 H -0.0078 -0.0302 0.1061
30 H 0.0988 0.1785 0.0028
31 H -0.0006 0.0589 0.1946
32 H -0.1507 -0.3044 0.0259
33 H 0.1358 -0.1538 0.2761
34 H 0.0849 -0.1118 -0.0305
35 H -0.0418 -0.0472 -0.1390
36 H -0.0166 -0.0166 0.0019
37 H -0.2356 0.4083 -0.0135
38 H -0.0376 0.1174 -0.4442
39 H -0.0464 0.2052 0.0099
40 H 0.2046 0.1627 -0.0655
41 H 0.0188 -0.1197 -0.1617
42 H -0.0212 -0.0380 0.0234
43 H 0.0365 -0.0098 0.0443
44 H -0.0021 0.0029 0.0032
45 H -0.0006 -0.0020 0.0001
46 H -0.0011 0.0022 -0.0017
47 H 0.0107 -0.0088 0.0027
48 H -0.0054 0.0031 0.0046
49 H 0.0046 -0.0107 0.0037
Vibrational Mode 112
----------------------------------------------------
Harmonic frequency: 1512.90 cm**-1
Reduced mass: 1.0754 amu
Force constant: 1.4503 mdyne/A
IR intensity: 2.7469 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0001 0.0005
2 O 0.0001 -0.0011 -0.0008
3 O -0.0003 0.0009 -0.0003
4 C -0.0024 0.0005 -0.0004
5 C 0.0007 -0.0009 -0.0013
6 C 0.0011 0.0001 0.0030
7 C -0.0004 0.0021 -0.0107
8 C -0.0002 -0.0021 -0.0050
9 C -0.0027 0.0142 0.0008
10 C 0.0006 0.0005 -0.0036
11 C 0.0025 0.0047 0.0030
12 C 0.0014 0.0308 -0.0191
13 C -0.0091 0.0254 0.0255
14 C 0.0014 -0.0036 0.0002
15 C -0.0054 0.0095 -0.0081
16 C -0.0111 0.0483 -0.0102
17 C 0.0007 0.0064 -0.0069
18 C 0.0012 -0.0028 -0.0003
19 C -0.0029 -0.0025 -0.0000
20 C -0.0035 0.0018 -0.0010
21 C 0.0040 -0.0005 0.0010
22 C 0.0058 0.0038 0.0003
23 C -0.0055 -0.0044 -0.0001
24 H -0.0176 0.0061 -0.0043
25 H -0.0021 0.0121 0.0021
26 H -0.0438 -0.0407 0.0544
27 H 0.0280 -0.0003 0.0568
28 H 0.0082 0.0463 0.0317
29 H -0.0006 -0.0135 0.0468
30 H -0.0267 -0.0418 -0.0015
31 H -0.0015 -0.0130 -0.0468
32 H -0.1352 -0.2584 0.0286
33 H 0.1172 -0.1303 0.2440
34 H 0.1962 -0.2142 -0.0769
35 H -0.0801 -0.0961 -0.2800
36 H 0.0008 0.0064 0.0004
37 H 0.0664 -0.0937 0.0049
38 H 0.0114 -0.0256 0.1030
39 H 0.0712 -0.5298 0.1360
40 H -0.2539 -0.1023 -0.1904
41 H 0.3572 -0.0096 0.1741
42 H -0.0323 -0.0609 0.0370
43 H 0.0371 -0.0047 0.0774
44 H -0.0035 -0.0028 0.0048
45 H -0.0024 -0.0017 -0.0010
46 H 0.0052 -0.0022 0.0035
47 H -0.0059 0.0044 -0.0022
48 H -0.0033 0.0021 0.0023
49 H -0.0028 0.0069 -0.0026
Vibrational Mode 113
----------------------------------------------------
Harmonic frequency: 1515.31 cm**-1
Reduced mass: 1.0743 amu
Force constant: 1.4533 mdyne/A
IR intensity: 1.9855 km/mol
Normal mode:
X Y Z
1 O 0.0003 0.0006 0.0001
2 O 0.0006 0.0001 -0.0012
3 O -0.0004 -0.0003 0.0000
4 C 0.0018 -0.0042 -0.0003
5 C -0.0037 0.0004 0.0001
6 C -0.0020 0.0023 0.0050
7 C 0.0130 -0.0190 0.0271
8 C -0.0006 0.0003 -0.0020
9 C 0.0124 -0.0040 0.0009
10 C -0.0011 -0.0012 -0.0039
11 C -0.0008 0.0015 0.0012
12 C 0.0001 0.0032 0.0002
13 C -0.0086 0.0351 0.0334
14 C -0.0034 0.0025 -0.0009
15 C -0.0020 0.0080 -0.0079
16 C 0.0402 -0.0123 0.0036
17 C 0.0019 0.0052 -0.0084
18 C 0.0003 -0.0001 0.0004
19 C -0.0020 -0.0008 -0.0001
20 C -0.0013 -0.0011 0.0005
21 C 0.0005 0.0036 -0.0008
22 C 0.0016 -0.0005 0.0005
23 C -0.0002 -0.0001 0.0000
24 H 0.0115 -0.0032 0.0023
25 H -0.0009 0.0011 0.0093
26 H 0.0460 0.2427 -0.1155
27 H -0.1503 0.0191 -0.2134
28 H 0.0014 0.0127 0.0084
29 H 0.0009 -0.0057 0.0187
30 H 0.0044 -0.0023 0.0016
31 H -0.0068 0.0035 -0.0025
32 H -0.0149 -0.0054 0.0100
33 H -0.0014 0.0027 0.0100
34 H 0.2445 -0.3248 -0.0832
35 H -0.1169 -0.1454 -0.3771
36 H 0.0084 -0.0053 -0.0011
37 H 0.0323 -0.0870 0.0008
38 H 0.0046 -0.0254 0.0860
39 H 0.0378 0.1765 -0.2685
40 H -0.1736 -0.1974 0.2051
41 H -0.4662 0.1787 0.0066
42 H -0.0421 -0.0691 0.0438
43 H 0.0149 0.0093 0.0987
44 H 0.0090 0.0035 -0.0028
45 H 0.0006 -0.0024 0.0012
46 H 0.0014 -0.0026 0.0010
47 H -0.0021 -0.0002 -0.0003
48 H 0.0028 -0.0010 -0.0027
49 H -0.0007 -0.0016 0.0002
Vibrational Mode 114
----------------------------------------------------
Harmonic frequency: 1569.10 cm**-1
Reduced mass: 9.8049 amu
Force constant: 14.2231 mdyne/A
IR intensity: 1.6930 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0000 0.0000
2 O 0.0000 -0.0000 -0.0000
3 O 0.0126 -0.0069 0.0025
4 C -0.0012 0.0018 -0.0001
5 C 0.0016 -0.0100 -0.0006
6 C 0.0000 -0.0001 -0.0000
7 C 0.0004 -0.0005 0.0000
8 C 0.0001 -0.0002 0.0001
9 C 0.0003 0.0007 0.0051
10 C 0.0001 -0.0000 -0.0001
11 C 0.0060 0.0012 0.0026
12 C 0.0004 0.0021 -0.0001
13 C 0.0000 0.0010 0.0010
14 C -0.1351 -0.0568 -0.0268
15 C -0.0044 0.0153 -0.0096
16 C 0.0007 0.0046 0.0042
17 C 0.0000 0.0001 -0.0001
18 C 0.0881 -0.0016 -0.0068
19 C 0.4232 0.3511 0.0219
20 C -0.1190 0.1159 -0.0430
21 C -0.1454 -0.4883 0.0978
22 C -0.3232 -0.0789 -0.0549
23 C 0.1640 0.1632 -0.0040
24 H 0.0025 0.0034 0.0005
25 H 0.0003 0.0002 0.0003
26 H -0.0017 0.0040 0.0001
27 H -0.0024 0.0009 -0.0007
28 H 0.0001 0.0002 0.0003
29 H -0.0006 0.0002 -0.0003
30 H -0.0079 -0.0107 0.0011
31 H -0.0063 0.0020 -0.0162
32 H -0.0026 -0.0113 -0.0004
33 H -0.0054 0.0024 0.0169
34 H 0.0083 -0.0066 -0.0034
35 H -0.0022 -0.0029 -0.0102
36 H -0.0364 0.0705 0.0059
37 H 0.0179 -0.0328 -0.0054
38 H -0.0043 -0.0007 0.0323
39 H 0.0178 -0.0505 -0.0415
40 H -0.0466 -0.0260 -0.0101
41 H -0.0054 0.0125 0.0169
42 H -0.0005 -0.0008 0.0006
43 H 0.0004 0.0000 0.0013
44 H 0.0887 0.0109 -0.0046
45 H 0.0000 0.0000 0.0000
46 H -0.1235 0.1028 -0.0169
47 H 0.3225 -0.0941 0.0943
48 H -0.0001 0.0000 0.0000
49 H 0.1052 -0.1098 0.0594
Vibrational Mode 115
----------------------------------------------------
Harmonic frequency: 1637.48 cm**-1
Reduced mass: 8.9585 amu
Force constant: 14.1526 mdyne/A
IR intensity: 8.8792 km/mol
Normal mode:
X Y Z
1 O 0.0000 -0.0000 0.0000
2 O 0.0000 -0.0000 0.0000
3 O -0.0080 -0.0098 -0.0006
4 C 0.0013 0.0045 -0.0009
5 C -0.0069 -0.0130 0.0060
6 C -0.0001 0.0001 -0.0000
7 C -0.0000 -0.0004 0.0002
8 C -0.0002 -0.0007 0.0001
9 C -0.0659 -0.0332 0.0134
10 C 0.0001 0.0001 -0.0000
11 C -0.0008 -0.0007 -0.0002
12 C 0.0008 0.0001 -0.0005
13 C 0.0003 -0.0004 -0.0003
14 C 0.0295 0.0120 0.0000
15 C -0.0019 -0.0005 0.0040
16 C 0.0033 -0.0030 0.0121
17 C -0.0000 0.0001 -0.0000
18 C 0.5792 0.0817 -0.0536
19 C -0.0818 -0.0744 0.0009
20 C -0.5831 0.0331 -0.0120
21 C 0.1154 0.0488 0.0060
22 C 0.0287 0.0103 0.0035
23 C -0.0101 -0.0042 -0.0008
24 H 0.0119 0.0067 0.0064
25 H -0.0001 -0.0010 -0.0001
26 H 0.0015 -0.0008 -0.0011
27 H -0.0015 0.0010 0.0025
28 H 0.0014 -0.0002 0.0002
29 H -0.0031 0.0010 -0.0021
30 H -0.0009 0.0006 -0.0004
31 H 0.0028 -0.0023 0.0010
32 H -0.0013 -0.0018 0.0008
33 H -0.0055 0.0053 0.0080
34 H -0.0033 0.0023 0.0015
35 H 0.0007 0.0009 0.0021
36 H 0.0248 -0.0067 0.0015
37 H -0.0085 0.0033 0.0022
38 H 0.0098 0.0137 -0.0139
39 H -0.0046 0.0439 0.0029
40 H 0.0199 0.0221 -0.0201
41 H -0.0123 -0.0272 -0.0404
42 H 0.0000 -0.0004 0.0001
43 H 0.0009 -0.0003 0.0001
44 H -0.0246 -0.2987 0.1610
45 H 0.0000 0.0003 -0.0001
46 H 0.0983 -0.3317 0.1755
47 H -0.0564 0.0106 -0.0176
48 H -0.0002 0.0001 0.0001
49 H -0.0142 -0.0010 0.0026
Vibrational Mode 116
----------------------------------------------------
Harmonic frequency: 2833.72 cm**-1
Reduced mass: 1.0719 amu
Force constant: 5.0713 mdyne/A
IR intensity: 41.9956 km/mol
Normal mode:
X Y Z
1 O -0.0000 0.0000 0.0000
2 O -0.0000 -0.0000 0.0000
3 O -0.0001 0.0001 -0.0001
4 C 0.0001 -0.0001 0.0001
5 C -0.0008 0.0001 0.0044
6 C -0.0001 -0.0000 0.0001
7 C -0.0000 -0.0001 0.0000
8 C 0.0000 -0.0000 0.0001
9 C 0.0004 -0.0008 -0.0032
10 C -0.0000 -0.0000 0.0000
11 C -0.0008 -0.0007 0.0019
12 C -0.0001 0.0000 0.0001
13 C 0.0000 0.0001 0.0000
14 C -0.0001 -0.0013 -0.0758
15 C -0.0006 0.0017 -0.0008
16 C 0.0005 -0.0003 0.0004
17 C 0.0000 0.0000 0.0001
18 C -0.0004 -0.0003 0.0001
19 C -0.0034 -0.0004 -0.0027
20 C 0.0006 -0.0002 0.0002
21 C 0.0002 0.0011 -0.0001
22 C 0.0008 -0.0004 0.0001
23 C -0.0001 -0.0003 0.0001
24 H 0.0093 0.0005 -0.0529
25 H 0.0009 0.0001 -0.0014
26 H -0.0007 -0.0002 -0.0001
27 H 0.0004 0.0014 -0.0000
28 H 0.0001 0.0006 -0.0010
29 H -0.0001 -0.0002 -0.0001
30 H 0.0048 0.0001 -0.0149
31 H 0.0040 0.0077 -0.0013
32 H 0.0009 -0.0001 0.0013
33 H -0.0006 -0.0002 -0.0003
34 H -0.0006 -0.0000 -0.0005
35 H 0.0002 -0.0003 0.0003
36 H 0.0229 0.0216 0.9945
37 H 0.0023 0.0046 -0.0249
38 H 0.0094 -0.0123 -0.0033
39 H -0.0031 -0.0001 -0.0002
40 H -0.0001 -0.0005 -0.0017
41 H 0.0002 0.0017 -0.0001
42 H -0.0005 -0.0008 -0.0017
43 H 0.0001 0.0002 0.0000
44 H 0.0009 -0.0022 -0.0000
45 H -0.0000 -0.0000 -0.0001
46 H -0.0010 -0.0022 0.0006
47 H 0.0001 -0.0007 0.0001
48 H 0.0000 0.0001 -0.0001
49 H -0.0013 0.0004 -0.0002
Vibrational Mode 117
----------------------------------------------------
Harmonic frequency: 2884.04 cm**-1
Reduced mass: 1.0745 amu
Force constant: 5.2657 mdyne/A
IR intensity: 40.9378 km/mol
Normal mode:
X Y Z
1 O 0.0000 -0.0000 -0.0001
2 O 0.0001 -0.0000 -0.0002
3 O -0.0000 -0.0000 -0.0000
4 C -0.0010 -0.0009 0.0030
5 C -0.0100 -0.0019 0.0764
6 C 0.0011 0.0000 -0.0014
7 C -0.0001 -0.0006 -0.0005
8 C 0.0005 0.0012 -0.0014
9 C 0.0010 0.0002 0.0034
10 C -0.0001 0.0000 -0.0001
11 C 0.0007 0.0008 -0.0028
12 C -0.0046 0.0009 -0.0030
13 C -0.0000 0.0002 -0.0009
14 C -0.0002 0.0001 0.0037
15 C -0.0000 0.0001 -0.0004
16 C 0.0006 0.0001 -0.0005
17 C -0.0014 -0.0017 -0.0033
18 C -0.0005 0.0004 -0.0005
19 C 0.0003 0.0000 0.0001
20 C -0.0000 0.0000 -0.0000
21 C -0.0001 -0.0001 0.0000
22 C -0.0001 0.0000 -0.0000
23 C -0.0000 0.0000 -0.0000
24 H 0.1210 0.0266 -0.9825
25 H -0.0134 -0.0008 0.0171
26 H 0.0022 0.0010 0.0015
27 H 0.0001 0.0028 0.0006
28 H -0.0041 -0.0112 0.0135
29 H -0.0011 -0.0025 -0.0021
30 H -0.0035 -0.0007 0.0302
31 H -0.0049 -0.0096 0.0016
32 H 0.0449 -0.0131 0.0601
33 H 0.0016 0.0070 -0.0006
34 H 0.0036 0.0006 0.0042
35 H -0.0016 0.0007 0.0004
36 H 0.0006 -0.0027 -0.0531
37 H -0.0012 0.0004 0.0073
38 H 0.0012 -0.0018 -0.0011
39 H -0.0008 0.0002 0.0011
40 H -0.0022 0.0028 0.0005
41 H -0.0017 -0.0037 0.0017
42 H 0.0146 0.0193 0.0451
43 H 0.0003 0.0022 -0.0017
44 H 0.0056 -0.0054 0.0027
45 H -0.0001 0.0003 0.0007
46 H 0.0006 0.0013 -0.0001
47 H -0.0000 0.0001 0.0000
48 H 0.0002 -0.0004 0.0003
49 H 0.0000 -0.0000 0.0000
Vibrational Mode 118
----------------------------------------------------
Harmonic frequency: 2891.29 cm**-1
Reduced mass: 1.0721 amu
Force constant: 5.2803 mdyne/A
IR intensity: 59.8580 km/mol
Normal mode:
X Y Z
1 O -0.0002 -0.0001 -0.0003
2 O -0.0023 0.0009 0.0031
3 O 0.0000 0.0000 -0.0000
4 C -0.0000 -0.0000 0.0001
5 C -0.0005 -0.0001 0.0039
6 C 0.0014 -0.0003 -0.0021
7 C -0.0001 -0.0001 -0.0002
8 C -0.0002 0.0005 -0.0018
9 C 0.0000 0.0000 0.0002
10 C 0.0021 0.0011 0.0029
11 C 0.0000 -0.0000 -0.0005
12 C -0.0001 -0.0003 0.0002
13 C 0.0002 -0.0004 -0.0003
14 C -0.0000 0.0000 0.0003
15 C 0.0000 -0.0001 -0.0001
16 C 0.0001 0.0000 -0.0000
17 C 0.0259 0.0312 0.0642
18 C -0.0000 0.0000 -0.0000
19 C 0.0000 0.0000 0.0000
20 C 0.0000 0.0000 -0.0000
21 C -0.0000 -0.0000 0.0000
22 C -0.0000 -0.0000 -0.0000
23 C 0.0000 -0.0000 0.0000
24 H 0.0063 0.0012 -0.0496
25 H -0.0183 -0.0008 0.0233
26 H 0.0021 0.0004 0.0016
27 H 0.0005 0.0015 -0.0002
28 H 0.0003 -0.0138 0.0173
29 H 0.0025 0.0063 0.0006
30 H -0.0006 0.0000 0.0056
31 H 0.0002 0.0002 -0.0003
32 H -0.0012 0.0009 -0.0013
33 H 0.0022 0.0033 0.0006
34 H 0.0028 0.0002 0.0040
35 H -0.0057 0.0037 -0.0015
36 H 0.0000 -0.0002 -0.0044
37 H -0.0002 0.0000 0.0008
38 H -0.0006 0.0008 0.0002
39 H -0.0005 -0.0001 -0.0001
40 H -0.0005 0.0005 0.0001
41 H -0.0003 -0.0005 0.0002
42 H -0.2855 -0.3756 -0.8749
43 H -0.0045 -0.0203 0.0396
44 H 0.0003 -0.0004 0.0003
45 H 0.0002 0.0008 0.0014
46 H -0.0001 -0.0001 0.0001
47 H 0.0000 0.0001 -0.0000
48 H -0.0033 0.0027 -0.0045
49 H -0.0000 0.0000 -0.0000
Vibrational Mode 119
----------------------------------------------------
Harmonic frequency: 2925.13 cm**-1
Reduced mass: 1.0756 amu
Force constant: 5.4222 mdyne/A
IR intensity: 75.6487 km/mol
Normal mode:
X Y Z
1 O 0.0002 0.0002 -0.0003
2 O 0.0001 0.0001 -0.0002
3 O -0.0000 -0.0000 -0.0000
4 C 0.0001 0.0003 -0.0003
5 C -0.0002 0.0000 0.0016
6 C -0.0475 -0.0027 0.0619
7 C 0.0004 0.0036 0.0003
8 C 0.0002 0.0005 -0.0008
9 C 0.0001 0.0000 0.0001
10 C -0.0018 -0.0003 0.0019
11 C 0.0001 -0.0001 -0.0010
12 C -0.0001 0.0000 -0.0012
13 C -0.0012 -0.0035 -0.0021
14 C -0.0000 0.0000 0.0002
15 C -0.0001 0.0001 0.0001
16 C 0.0000 -0.0000 0.0000
17 C 0.0007 0.0012 0.0020
18 C -0.0000 0.0000 -0.0000
19 C 0.0000 -0.0000 0.0000
20 C -0.0000 -0.0000 0.0000
21 C 0.0000 0.0000 0.0000
22 C -0.0000 0.0000 -0.0000
23 C -0.0000 0.0000 -0.0000
24 H 0.0028 0.0005 -0.0213
25 H 0.6034 0.0385 -0.7855
26 H 0.0078 0.0064 0.0083
27 H -0.0224 -0.0533 0.0080
28 H -0.0012 -0.0066 0.0093
29 H -0.0003 0.0011 0.0002
30 H -0.0020 0.0006 0.0135
31 H 0.0002 -0.0003 -0.0006
32 H 0.0062 -0.0015 0.0081
33 H -0.0022 -0.0019 0.0001
34 H 0.0328 0.0055 0.0516
35 H -0.0362 0.0306 -0.0059
36 H 0.0001 -0.0001 -0.0026
37 H 0.0000 0.0000 -0.0007
38 H 0.0013 -0.0019 -0.0007
39 H -0.0006 -0.0001 -0.0002
40 H -0.0001 -0.0002 0.0004
41 H -0.0003 0.0005 0.0001
42 H -0.0079 -0.0107 -0.0241
43 H -0.0025 -0.0037 0.0019
44 H 0.0004 -0.0002 0.0001
45 H 0.0047 0.0029 0.0002
46 H 0.0003 0.0006 -0.0002
47 H -0.0000 -0.0002 0.0000
48 H 0.0004 -0.0003 0.0010
49 H 0.0002 -0.0001 0.0001
Vibrational Mode 120
----------------------------------------------------
Harmonic frequency: 2954.30 cm**-1
Reduced mass: 1.0875 amu
Force constant: 5.5925 mdyne/A
IR intensity: 34.6790 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0000 0.0001
2 O -0.0000 -0.0000 0.0000
3 O 0.0000 0.0000 0.0000
4 C 0.0012 0.0006 -0.0027
5 C 0.0002 0.0001 -0.0021
6 C 0.0003 -0.0001 -0.0005
7 C -0.0017 -0.0047 0.0010
8 C -0.0001 0.0002 0.0024
9 C -0.0000 -0.0001 -0.0003
10 C 0.0000 0.0000 -0.0000
11 C 0.0221 0.0210 -0.0779
12 C 0.0009 0.0001 0.0009
13 C 0.0006 -0.0000 0.0007
14 C 0.0001 0.0002 -0.0004
15 C -0.0055 0.0078 0.0092
16 C 0.0000 0.0002 -0.0000
17 C -0.0001 -0.0001 -0.0002
18 C 0.0000 -0.0000 0.0000
19 C -0.0001 0.0000 -0.0000
20 C 0.0000 0.0000 -0.0000
21 C 0.0000 0.0000 0.0000
22 C 0.0000 -0.0001 0.0000
23 C 0.0000 -0.0000 0.0000
24 H -0.0037 -0.0029 0.0299
25 H -0.0044 -0.0005 0.0064
26 H -0.0014 -0.0019 -0.0002
27 H 0.0208 0.0558 -0.0081
28 H 0.0038 0.0162 -0.0218
29 H -0.0032 -0.0192 -0.0048
30 H -0.1135 0.0388 0.9088
31 H -0.1623 -0.2960 0.0700
32 H -0.0076 0.0027 -0.0107
33 H -0.0027 -0.0039 -0.0004
34 H -0.0051 -0.0013 -0.0082
35 H -0.0020 0.0018 0.0003
36 H -0.0021 -0.0021 0.0157
37 H 0.0061 0.0032 -0.1200
38 H 0.0616 -0.0909 -0.0268
39 H -0.0007 -0.0001 -0.0001
40 H 0.0008 -0.0009 -0.0002
41 H -0.0002 -0.0008 0.0011
42 H 0.0009 0.0011 0.0026
43 H 0.0002 0.0003 -0.0002
44 H -0.0003 0.0005 -0.0003
45 H -0.0001 -0.0001 -0.0005
46 H -0.0002 -0.0005 0.0002
47 H 0.0001 0.0006 -0.0002
48 H -0.0001 0.0001 -0.0001
49 H -0.0001 0.0001 -0.0000
Vibrational Mode 121
----------------------------------------------------
Harmonic frequency: 2962.50 cm**-1
Reduced mass: 1.0911 amu
Force constant: 5.6422 mdyne/A
IR intensity: 6.8413 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0001 -0.0000
2 O 0.0000 -0.0000 -0.0000
3 O -0.0000 -0.0000 -0.0000
4 C 0.0000 -0.0000 0.0001
5 C 0.0007 0.0001 0.0030
6 C -0.0009 0.0001 -0.0029
7 C 0.0020 0.0026 0.0006
8 C 0.0002 0.0014 -0.0010
9 C 0.0000 -0.0001 0.0000
10 C -0.0000 0.0001 0.0002
11 C -0.0003 -0.0006 -0.0002
12 C 0.0298 0.0109 0.0307
13 C -0.0538 0.0175 -0.0486
14 C 0.0000 0.0001 0.0001
15 C -0.0006 0.0008 0.0008
16 C -0.0014 0.0005 0.0001
17 C 0.0001 0.0000 -0.0003
18 C 0.0003 -0.0004 0.0001
19 C 0.0000 0.0000 0.0000
20 C -0.0000 0.0000 0.0000
21 C -0.0000 0.0000 0.0000
22 C -0.0000 -0.0000 0.0000
23 C -0.0000 -0.0000 0.0000
24 H 0.0030 0.0011 -0.0310
25 H -0.0120 -0.0012 0.0165
26 H -0.0096 -0.0045 -0.0117
27 H -0.0106 -0.0265 0.0056
28 H -0.0019 -0.0097 0.0140
29 H -0.0014 -0.0061 -0.0026
30 H -0.0009 -0.0002 0.0053
31 H 0.0043 0.0076 -0.0031
32 H -0.2440 0.0888 -0.3600
33 H -0.1445 -0.2175 -0.0341
34 H 0.3564 0.1109 0.6120
35 H 0.3244 -0.3218 0.0035
36 H 0.0000 -0.0001 -0.0011
37 H 0.0004 0.0003 -0.0059
38 H 0.0075 -0.0110 -0.0036
39 H 0.0089 0.0021 0.0023
40 H 0.0051 -0.0083 -0.0023
41 H 0.0000 0.0005 0.0001
42 H 0.0007 0.0008 0.0024
43 H 0.0009 -0.0010 0.0001
44 H -0.0020 0.0034 -0.0020
45 H -0.0004 0.0004 0.0002
46 H -0.0001 -0.0002 0.0000
47 H 0.0000 0.0003 -0.0001
48 H 0.0002 -0.0000 0.0000
49 H -0.0000 -0.0000 -0.0000
Vibrational Mode 122
----------------------------------------------------
Harmonic frequency: 2971.26 cm**-1
Reduced mass: 1.0699 amu
Force constant: 5.5652 mdyne/A
IR intensity: 17.1843 km/mol
Normal mode:
X Y Z
1 O 0.0000 0.0000 -0.0000
2 O 0.0000 -0.0000 -0.0000
3 O 0.0001 0.0001 -0.0000
4 C 0.0002 0.0017 0.0003
5 C -0.0002 0.0000 0.0002
6 C -0.0009 -0.0005 0.0010
7 C -0.0062 -0.0154 0.0022
8 C -0.0006 -0.0033 -0.0004
9 C -0.0002 0.0001 0.0001
10 C -0.0001 -0.0001 0.0000
11 C 0.0245 0.0443 -0.0026
12 C 0.0016 0.0009 0.0013
13 C -0.0005 -0.0000 -0.0009
14 C 0.0007 -0.0016 -0.0016
15 C 0.0282 -0.0372 -0.0239
16 C -0.0006 -0.0001 0.0003
17 C 0.0001 0.0002 0.0000
18 C 0.0000 -0.0000 -0.0000
19 C -0.0001 0.0000 -0.0000
20 C 0.0001 0.0000 0.0000
21 C -0.0000 -0.0000 0.0000
22 C 0.0001 0.0004 -0.0001
23 C 0.0000 -0.0001 0.0000
24 H 0.0003 -0.0005 -0.0006
25 H 0.0096 0.0006 -0.0124
26 H 0.0001 -0.0065 0.0052
27 H 0.0765 0.1955 -0.0317
28 H 0.0002 0.0021 -0.0058
29 H 0.0058 0.0371 0.0096
30 H 0.0375 0.0175 -0.1961
31 H -0.3311 -0.5827 0.2193
32 H -0.0095 0.0039 -0.0143
33 H -0.0100 -0.0139 -0.0027
34 H 0.0073 0.0022 0.0125
35 H 0.0007 -0.0015 -0.0003
36 H 0.0003 0.0005 0.0155
37 H 0.0020 -0.0193 0.1198
38 H -0.3422 0.4969 0.1732
39 H 0.0024 0.0003 0.0009
40 H 0.0029 -0.0043 -0.0012
41 H 0.0019 0.0052 -0.0022
42 H -0.0001 -0.0001 -0.0004
43 H -0.0010 -0.0018 0.0002
44 H -0.0002 -0.0001 -0.0001
45 H -0.0000 0.0001 0.0003
46 H -0.0005 -0.0006 0.0003
47 H -0.0005 -0.0040 0.0011
48 H -0.0001 0.0000 0.0000
49 H -0.0007 0.0003 -0.0003
Vibrational Mode 123
----------------------------------------------------
Harmonic frequency: 2976.75 cm**-1
Reduced mass: 1.0975 amu
Force constant: 5.7299 mdyne/A
IR intensity: 59.9382 km/mol
Normal mode:
X Y Z
1 O -0.0000 0.0001 -0.0001
2 O 0.0001 0.0000 -0.0001
3 O -0.0000 0.0000 -0.0000
4 C -0.0004 -0.0007 0.0004
5 C 0.0010 0.0010 0.0047
6 C 0.0002 -0.0010 0.0018
7 C -0.0081 -0.0167 0.0010
8 C 0.0015 0.0083 -0.0053
9 C 0.0000 -0.0001 0.0001
10 C -0.0000 0.0000 -0.0001
11 C -0.0035 -0.0066 0.0010
12 C 0.0514 0.0234 0.0474
13 C 0.0358 -0.0156 0.0250
14 C 0.0000 0.0000 0.0001
15 C 0.0009 -0.0014 -0.0021
16 C 0.0012 -0.0011 -0.0003
17 C 0.0016 0.0030 -0.0008
18 C 0.0006 -0.0009 0.0002
19 C -0.0000 -0.0000 -0.0000
20 C 0.0000 0.0001 -0.0000
21 C 0.0000 -0.0000 0.0000
22 C 0.0000 0.0000 -0.0000
23 C 0.0000 -0.0000 0.0000
24 H 0.0053 0.0010 -0.0500
25 H 0.0110 0.0015 -0.0144
26 H 0.0159 0.0002 0.0213
27 H 0.0818 0.2067 -0.0363
28 H -0.0086 -0.0554 0.0796
29 H -0.0098 -0.0449 -0.0165
30 H -0.0041 -0.0027 0.0184
31 H 0.0486 0.0867 -0.0316
32 H -0.3407 0.1315 -0.4988
33 H -0.2952 -0.4304 -0.0795
34 H -0.1623 -0.0572 -0.2799
35 H -0.2716 0.2614 -0.0130
36 H 0.0002 -0.0001 -0.0024
37 H -0.0021 -0.0001 0.0213
38 H -0.0111 0.0160 0.0046
39 H -0.0036 -0.0015 -0.0015
40 H -0.0111 0.0140 0.0029
41 H 0.0001 -0.0008 -0.0003
42 H 0.0027 0.0045 0.0050
43 H -0.0219 -0.0385 0.0039
44 H -0.0043 0.0072 -0.0041
45 H 0.0006 -0.0005 0.0003
46 H -0.0008 -0.0015 0.0007
47 H -0.0000 -0.0001 0.0000
48 H -0.0002 0.0003 -0.0000
49 H -0.0001 0.0001 -0.0001
Vibrational Mode 124
----------------------------------------------------
Harmonic frequency: 2977.67 cm**-1
Reduced mass: 1.0751 amu
Force constant: 5.6165 mdyne/A
IR intensity: 40.3264 km/mol
Normal mode:
X Y Z
1 O -0.0000 0.0001 -0.0001
2 O -0.0000 0.0000 -0.0000
3 O -0.0000 -0.0000 0.0000
4 C -0.0005 -0.0003 0.0006
5 C -0.0001 0.0001 0.0000
6 C -0.0019 -0.0011 0.0018
7 C -0.0161 -0.0363 0.0039
8 C -0.0002 -0.0009 -0.0014
9 C 0.0002 -0.0001 -0.0001
10 C -0.0000 -0.0002 0.0001
11 C 0.0108 0.0276 0.0133
12 C -0.0023 -0.0001 -0.0027
13 C -0.0024 0.0012 -0.0019
14 C -0.0007 0.0012 0.0020
15 C -0.0291 0.0418 0.0288
16 C -0.0001 0.0001 -0.0001
17 C 0.0000 -0.0001 0.0002
18 C -0.0001 0.0001 0.0000
19 C 0.0001 0.0001 -0.0000
20 C -0.0000 0.0000 -0.0000
21 C -0.0000 -0.0001 0.0000
22 C -0.0001 -0.0005 0.0001
23 C 0.0000 0.0001 -0.0000
24 H -0.0002 -0.0003 0.0009
25 H 0.0183 0.0013 -0.0234
26 H 0.0173 -0.0095 0.0283
27 H 0.1819 0.4612 -0.0785
28 H -0.0017 -0.0090 0.0113
29 H 0.0029 0.0206 0.0051
30 H 0.0458 0.0042 -0.2935
31 H -0.1920 -0.3343 0.1330
32 H 0.0223 -0.0077 0.0319
33 H 0.0062 0.0103 0.0010
34 H 0.0132 0.0048 0.0226
35 H 0.0186 -0.0189 0.0010
36 H -0.0002 -0.0003 -0.0157
37 H 0.0025 0.0191 -0.1638
38 H 0.3683 -0.5333 -0.1841
39 H 0.0022 0.0005 0.0003
40 H -0.0006 0.0010 0.0002
41 H -0.0007 -0.0020 0.0001
42 H -0.0008 -0.0008 -0.0020
43 H 0.0006 0.0011 -0.0000
44 H 0.0006 -0.0004 0.0003
45 H 0.0006 0.0003 0.0008
46 H -0.0000 -0.0002 0.0001
47 H 0.0006 0.0048 -0.0012
48 H 0.0001 -0.0002 0.0001
49 H 0.0005 -0.0003 0.0002
Vibrational Mode 125
----------------------------------------------------
Harmonic frequency: 2979.68 cm**-1
Reduced mass: 1.0740 amu
Force constant: 5.6181 mdyne/A
IR intensity: 86.4704 km/mol
Normal mode:
X Y Z
1 O -0.0000 0.0002 -0.0002
2 O -0.0000 0.0000 -0.0000
3 O 0.0000 0.0000 0.0000
4 C -0.0012 -0.0026 0.0004
5 C 0.0001 0.0002 -0.0013
6 C -0.0030 -0.0016 0.0024
7 C -0.0241 -0.0565 0.0074
8 C 0.0018 0.0090 -0.0001
9 C -0.0001 0.0000 0.0000
10 C -0.0001 -0.0000 0.0002
11 C -0.0135 -0.0283 -0.0026
12 C -0.0122 -0.0039 -0.0123
13 C -0.0108 0.0057 -0.0070
14 C 0.0002 -0.0004 -0.0007
15 C 0.0109 -0.0162 -0.0132
16 C -0.0013 -0.0003 0.0012
17 C -0.0005 -0.0008 0.0001
18 C -0.0002 0.0003 -0.0001
19 C -0.0000 -0.0001 0.0000
20 C -0.0000 -0.0001 0.0001
21 C 0.0000 0.0001 -0.0000
22 C 0.0001 0.0002 -0.0000
23 C -0.0000 0.0000 -0.0000
24 H -0.0022 -0.0012 0.0160
25 H 0.0266 0.0019 -0.0331
26 H 0.0124 -0.0209 0.0304
27 H 0.2875 0.7255 -0.1240
28 H -0.0019 -0.0153 0.0285
29 H -0.0189 -0.0941 -0.0261
30 H -0.0289 -0.0084 0.1649
31 H 0.2033 0.3600 -0.1353
32 H 0.0930 -0.0342 0.1351
33 H 0.0573 0.0852 0.0140
34 H 0.0445 0.0173 0.0781
35 H 0.0911 -0.0885 0.0054
36 H 0.0003 0.0004 0.0040
37 H -0.0053 -0.0071 0.0935
38 H -0.1392 0.2018 0.0680
39 H -0.0019 -0.0002 0.0006
40 H 0.0101 -0.0136 -0.0032
41 H 0.0063 0.0170 -0.0100
42 H -0.0003 -0.0004 0.0001
43 H 0.0049 0.0092 -0.0011
44 H 0.0015 -0.0023 0.0011
45 H 0.0008 0.0000 0.0008
46 H 0.0007 0.0014 -0.0006
47 H -0.0004 -0.0026 0.0006
48 H 0.0003 -0.0000 0.0001
49 H 0.0000 0.0001 -0.0000
Vibrational Mode 126
----------------------------------------------------
Harmonic frequency: 2986.27 cm**-1
Reduced mass: 1.0519 amu
Force constant: 5.5269 mdyne/A
IR intensity: 44.2256 km/mol
Normal mode:
X Y Z
1 O -0.0001 -0.0001 -0.0000
2 O 0.0000 0.0001 0.0001
3 O 0.0000 0.0000 0.0000
4 C -0.0001 -0.0002 0.0001
5 C -0.0002 0.0008 -0.0021
6 C 0.0034 -0.0017 -0.0051
7 C -0.0000 -0.0006 0.0009
8 C -0.0004 -0.0025 0.0040
9 C 0.0001 0.0000 -0.0001
10 C 0.0002 -0.0000 0.0002
11 C -0.0003 -0.0006 -0.0003
12 C -0.0038 0.0128 -0.0154
13 C 0.0188 -0.0441 -0.0348
14 C -0.0000 0.0000 -0.0001
15 C 0.0001 -0.0002 0.0002
16 C -0.0006 0.0003 -0.0002
17 C 0.0004 0.0007 -0.0005
18 C 0.0002 -0.0003 0.0001
19 C -0.0000 0.0000 -0.0000
20 C 0.0000 0.0001 -0.0000
21 C -0.0000 -0.0000 -0.0000
22 C 0.0000 -0.0000 0.0000
23 C 0.0000 -0.0000 0.0000
24 H -0.0021 -0.0006 0.0240
25 H -0.0428 -0.0038 0.0549
26 H -0.0075 -0.0037 -0.0078
27 H 0.0060 0.0117 -0.0019
28 H 0.0054 0.0353 -0.0490
29 H -0.0009 -0.0050 0.0012
30 H -0.0010 -0.0000 0.0069
31 H 0.0048 0.0088 -0.0033
32 H 0.1550 -0.0424 0.2152
33 H -0.0969 -0.1249 -0.0393
34 H 0.3169 0.0593 0.4892
35 H -0.5439 0.4939 -0.0657
36 H -0.0001 0.0001 0.0016
37 H 0.0003 -0.0002 -0.0034
38 H -0.0016 0.0024 0.0010
39 H 0.0070 0.0016 0.0016
40 H 0.0007 -0.0018 0.0004
41 H -0.0017 -0.0032 0.0023
42 H 0.0023 0.0032 0.0057
43 H -0.0075 -0.0111 0.0021
44 H -0.0022 0.0025 -0.0019
45 H -0.0006 -0.0003 0.0004
46 H -0.0005 -0.0009 0.0004
47 H 0.0000 0.0001 -0.0000
48 H -0.0000 -0.0003 -0.0004
49 H -0.0001 0.0000 -0.0000
Vibrational Mode 127
----------------------------------------------------
Harmonic frequency: 2996.29 cm**-1
Reduced mass: 1.0733 amu
Force constant: 5.6772 mdyne/A
IR intensity: 4.4984 km/mol
Normal mode:
X Y Z
1 O 0.0001 -0.0003 -0.0002
2 O -0.0000 0.0000 0.0000
3 O 0.0000 0.0000 0.0000
4 C 0.0008 0.0017 -0.0006
5 C 0.0001 -0.0014 0.0013
6 C 0.0008 0.0000 -0.0011
7 C 0.0005 0.0022 -0.0007
8 C 0.0116 0.0576 -0.0143
9 C -0.0002 -0.0001 -0.0001
10 C 0.0001 0.0016 -0.0000
11 C 0.0014 0.0031 0.0004
12 C -0.0103 -0.0416 0.0132
13 C 0.0065 -0.0128 -0.0056
14 C 0.0000 -0.0000 0.0000
15 C -0.0003 0.0004 0.0012
16 C -0.0008 0.0006 -0.0004
17 C -0.0008 -0.0016 -0.0004
18 C -0.0008 0.0010 -0.0003
19 C -0.0000 -0.0000 -0.0000
20 C -0.0000 -0.0000 0.0000
21 C -0.0001 -0.0000 -0.0000
22 C -0.0000 -0.0000 0.0000
23 C 0.0000 -0.0000 0.0000
24 H -0.0006 -0.0010 -0.0120
25 H -0.0102 -0.0014 0.0132
26 H 0.0039 0.0022 0.0028
27 H -0.0115 -0.0301 0.0060
28 H -0.0292 -0.2096 0.3288
29 H -0.1121 -0.5034 -0.1559
30 H 0.0036 0.0010 -0.0208
31 H -0.0224 -0.0420 0.0155
32 H -0.2156 0.0458 -0.2932
33 H 0.3508 0.4666 0.1235
34 H 0.0622 0.0102 0.0957
35 H -0.1507 0.1363 -0.0165
36 H -0.0001 0.0000 0.0006
37 H 0.0013 0.0001 -0.0136
38 H 0.0028 -0.0041 -0.0009
39 H 0.0099 0.0024 0.0024
40 H 0.0023 -0.0027 -0.0005
41 H -0.0029 -0.0056 0.0034
42 H 0.0032 0.0036 0.0093
43 H 0.0101 0.0165 -0.0037
44 H 0.0072 -0.0092 0.0057
45 H -0.0016 0.0011 0.0030
46 H 0.0006 0.0012 -0.0005
47 H 0.0000 0.0003 -0.0001
48 H -0.0003 -0.0006 0.0000
49 H -0.0002 0.0001 -0.0000
Vibrational Mode 128
----------------------------------------------------
Harmonic frequency: 2999.40 cm**-1
Reduced mass: 1.0753 amu
Force constant: 5.6994 mdyne/A
IR intensity: 72.5337 km/mol
Normal mode:
X Y Z
1 O -0.0001 0.0003 0.0002
2 O 0.0001 0.0001 -0.0001
3 O 0.0000 0.0000 0.0000
4 C -0.0006 -0.0018 0.0006
5 C -0.0000 -0.0011 0.0028
6 C 0.0001 -0.0004 0.0001
7 C -0.0027 -0.0065 0.0010
8 C -0.0122 -0.0609 0.0157
9 C -0.0001 -0.0002 0.0000
10 C -0.0000 -0.0018 0.0002
11 C -0.0019 -0.0039 -0.0002
12 C -0.0040 -0.0370 0.0181
13 C 0.0072 -0.0107 -0.0023
14 C 0.0001 0.0000 -0.0000
15 C 0.0002 0.0000 -0.0035
16 C -0.0010 0.0004 -0.0001
17 C 0.0022 0.0041 -0.0009
18 C -0.0009 0.0011 -0.0004
19 C -0.0000 -0.0000 -0.0000
20 C -0.0000 -0.0001 0.0000
21 C -0.0001 -0.0000 -0.0000
22 C -0.0000 0.0000 -0.0000
23 C -0.0000 0.0000 -0.0000
24 H 0.0039 0.0009 -0.0365
25 H 0.0011 -0.0003 -0.0018
26 H 0.0014 -0.0018 0.0040
27 H 0.0330 0.0836 -0.0156
28 H 0.0310 0.2286 -0.3558
29 H 0.1182 0.5297 0.1645
30 H -0.0041 -0.0013 0.0219
31 H 0.0287 0.0534 -0.0195
32 H -0.2448 0.0602 -0.3385
33 H 0.2999 0.3955 0.1081
34 H 0.0357 0.0043 0.0534
35 H -0.1315 0.1198 -0.0130
36 H 0.0002 -0.0000 -0.0008
37 H -0.0048 0.0006 0.0457
38 H 0.0012 -0.0014 -0.0025
39 H 0.0096 0.0024 0.0024
40 H 0.0037 -0.0049 -0.0011
41 H -0.0013 -0.0010 0.0004
42 H 0.0018 0.0036 0.0020
43 H -0.0291 -0.0518 0.0065
44 H 0.0087 -0.0106 0.0061
45 H 0.0018 -0.0010 -0.0023
46 H 0.0008 0.0015 -0.0006
47 H -0.0000 -0.0001 0.0000
48 H 0.0003 -0.0003 -0.0001
49 H -0.0001 0.0001 -0.0000
Vibrational Mode 129
----------------------------------------------------
Harmonic frequency: 3007.88 cm**-1
Reduced mass: 1.0546 amu
Force constant: 5.6215 mdyne/A
IR intensity: 10.1395 km/mol
Normal mode:
X Y Z
1 O 0.0001 -0.0001 -0.0003
2 O 0.0000 0.0001 -0.0000
3 O -0.0000 -0.0000 0.0000
4 C 0.0003 0.0003 0.0012
5 C -0.0002 -0.0001 0.0006
6 C -0.0005 -0.0000 0.0005
7 C 0.0009 0.0011 0.0001
8 C 0.0041 0.0084 0.0511
9 C 0.0001 -0.0001 -0.0001
10 C 0.0000 0.0003 0.0012
11 C 0.0016 0.0037 -0.0021
12 C 0.0025 0.0014 0.0022
13 C 0.0000 0.0015 0.0021
14 C 0.0001 0.0004 -0.0016
15 C -0.0020 0.0086 -0.0373
16 C -0.0012 -0.0031 0.0013
17 C 0.0017 0.0027 0.0007
18 C 0.0001 -0.0001 0.0000
19 C -0.0000 0.0001 -0.0000
20 C 0.0000 -0.0000 0.0000
21 C -0.0000 0.0000 -0.0000
22 C -0.0001 -0.0003 0.0001
23 C -0.0000 0.0000 -0.0000
24 H 0.0020 0.0013 -0.0096
25 H 0.0058 0.0003 -0.0076
26 H -0.0042 -0.0014 -0.0042
27 H -0.0055 -0.0126 0.0026
28 H 0.0572 0.3881 -0.5157
29 H -0.1068 -0.4897 -0.1131
30 H 0.0016 0.0006 -0.0020
31 H -0.0269 -0.0472 0.0167
32 H -0.0153 0.0064 -0.0236
33 H -0.0169 -0.0242 -0.0048
34 H -0.0168 -0.0039 -0.0265
35 H 0.0167 -0.0150 0.0024
36 H 0.0010 0.0009 0.0079
37 H -0.0544 0.0093 0.5258
38 H 0.0789 -0.1120 -0.0610
39 H 0.0078 0.0002 0.0025
40 H -0.0043 0.0037 0.0021
41 H 0.0119 0.0310 -0.0199
42 H -0.0053 -0.0040 -0.0145
43 H -0.0159 -0.0292 0.0043
44 H -0.0004 0.0006 -0.0003
45 H -0.0027 0.0004 0.0042
46 H -0.0001 -0.0002 0.0000
47 H 0.0008 0.0033 -0.0009
48 H -0.0001 -0.0001 0.0002
49 H 0.0003 -0.0002 0.0001
Vibrational Mode 130
----------------------------------------------------
Harmonic frequency: 3009.03 cm**-1
Reduced mass: 1.0598 amu
Force constant: 5.6535 mdyne/A
IR intensity: 69.9235 km/mol
Normal mode:
X Y Z
1 O -0.0001 0.0000 0.0002
2 O -0.0000 -0.0000 0.0001
3 O -0.0000 -0.0001 0.0000
4 C 0.0001 0.0000 -0.0013
5 C 0.0001 0.0002 -0.0014
6 C 0.0004 0.0001 -0.0004
7 C 0.0022 0.0016 0.0020
8 C -0.0021 -0.0021 -0.0350
9 C 0.0000 -0.0003 -0.0002
10 C 0.0000 -0.0001 -0.0009
11 C 0.0024 0.0030 -0.0085
12 C -0.0018 0.0002 -0.0025
13 C -0.0005 -0.0008 -0.0018
14 C 0.0001 0.0006 -0.0027
15 C -0.0024 0.0119 -0.0563
16 C -0.0021 -0.0043 0.0022
17 C -0.0014 -0.0024 -0.0004
18 C 0.0001 -0.0001 0.0001
19 C -0.0001 0.0001 -0.0000
20 C -0.0000 -0.0001 0.0001
21 C 0.0000 0.0001 -0.0000
22 C -0.0001 -0.0004 0.0001
23 C -0.0000 0.0001 -0.0000
24 H -0.0024 -0.0020 0.0190
25 H -0.0050 -0.0004 0.0068
26 H -0.0236 -0.0092 -0.0261
27 H -0.0047 -0.0126 0.0035
28 H -0.0397 -0.2731 0.3656
29 H 0.0649 0.2972 0.0659
30 H -0.0119 0.0043 0.0774
31 H -0.0227 -0.0393 0.0113
32 H 0.0214 -0.0074 0.0314
33 H 0.0022 0.0044 -0.0003
34 H 0.0135 0.0033 0.0218
35 H -0.0072 0.0062 -0.0014
36 H 0.0009 0.0012 0.0168
37 H -0.0807 0.0135 0.7877
38 H 0.1099 -0.1555 -0.0869
39 H 0.0089 -0.0000 0.0035
40 H -0.0017 -0.0006 0.0016
41 H 0.0188 0.0482 -0.0304
42 H 0.0036 0.0024 0.0099
43 H 0.0146 0.0265 -0.0039
44 H -0.0016 0.0019 -0.0009
45 H 0.0015 -0.0001 -0.0028
46 H 0.0005 0.0006 -0.0004
47 H 0.0011 0.0043 -0.0012
48 H 0.0000 -0.0000 -0.0002
49 H 0.0007 -0.0004 0.0002
Vibrational Mode 131
----------------------------------------------------
Harmonic frequency: 3030.18 cm**-1
Reduced mass: 1.0704 amu
Force constant: 5.7906 mdyne/A
IR intensity: 24.4918 km/mol
Normal mode:
X Y Z
1 O 0.0003 0.0001 -0.0001
2 O -0.0016 -0.0000 0.0010
3 O 0.0000 0.0000 -0.0000
4 C -0.0000 -0.0002 0.0001
5 C 0.0002 -0.0001 0.0004
6 C -0.0001 -0.0001 0.0003
7 C -0.0006 -0.0006 -0.0003
8 C -0.0007 -0.0041 0.0039
9 C -0.0000 -0.0000 -0.0000
10 C -0.0011 -0.0022 0.0003
11 C -0.0002 -0.0002 0.0002
12 C 0.0020 -0.0001 0.0027
13 C 0.0020 -0.0016 0.0006
14 C 0.0000 -0.0000 0.0000
15 C 0.0000 -0.0001 0.0002
16 C -0.0012 -0.0003 -0.0001
17 C -0.0333 -0.0660 0.0119
18 C -0.0001 0.0001 -0.0000
19 C 0.0000 0.0000 0.0000
20 C -0.0000 -0.0000 0.0000
21 C -0.0000 -0.0000 0.0000
22 C -0.0000 0.0000 -0.0000
23 C -0.0000 0.0000 -0.0000
24 H 0.0006 0.0004 -0.0040
25 H 0.0023 -0.0001 -0.0036
26 H 0.0045 0.0017 0.0049
27 H 0.0021 0.0064 -0.0015
28 H 0.0066 0.0366 -0.0545
29 H 0.0028 0.0152 0.0063
30 H 0.0003 -0.0002 -0.0018
31 H 0.0015 0.0032 -0.0010
32 H -0.0281 0.0102 -0.0357
33 H -0.0050 -0.0081 -0.0007
34 H -0.0032 -0.0018 -0.0062
35 H -0.0242 0.0240 -0.0034
36 H 0.0000 -0.0000 -0.0005
37 H 0.0003 -0.0001 -0.0033
38 H -0.0010 0.0013 0.0006
39 H 0.0135 0.0026 0.0032
40 H 0.0003 -0.0014 -0.0004
41 H 0.0003 0.0025 -0.0017
42 H -0.0252 -0.0466 -0.0305
43 H 0.4707 0.8630 -0.1253
44 H 0.0009 -0.0011 0.0005
45 H -0.0016 0.0006 0.0013
46 H 0.0001 0.0001 -0.0001
47 H -0.0000 -0.0001 0.0000
48 H -0.0041 -0.0096 0.0021
49 H 0.0000 -0.0000 0.0000
Vibrational Mode 132
----------------------------------------------------
Harmonic frequency: 3036.14 cm**-1
Reduced mass: 1.0860 amu
Force constant: 5.8981 mdyne/A
IR intensity: 33.7073 km/mol
Normal mode:
X Y Z
1 O 0.0000 0.0000 0.0000
2 O -0.0000 -0.0000 0.0000
3 O -0.0000 0.0000 -0.0000
4 C 0.0001 -0.0000 -0.0000
5 C -0.0003 -0.0001 -0.0001
6 C -0.0000 0.0000 0.0000
7 C 0.0026 0.0005 0.0030
8 C -0.0000 -0.0001 0.0002
9 C 0.0024 0.0004 0.0007
10 C -0.0001 -0.0001 0.0000
11 C 0.0001 0.0002 -0.0002
12 C -0.0002 -0.0007 0.0002
13 C -0.0006 -0.0002 -0.0010
14 C 0.0001 0.0001 0.0001
15 C 0.0001 0.0002 -0.0018
16 C 0.0828 0.0139 0.0035
17 C -0.0005 -0.0010 0.0001
18 C -0.0004 0.0004 -0.0002
19 C -0.0001 -0.0002 -0.0001
20 C -0.0000 0.0001 -0.0003
21 C 0.0001 0.0001 -0.0002
22 C -0.0000 0.0000 -0.0000
23 C 0.0001 -0.0001 0.0000
24 H 0.0007 -0.0000 0.0028
25 H -0.0001 -0.0001 0.0002
26 H -0.0349 -0.0147 -0.0361
27 H 0.0047 0.0081 0.0006
28 H 0.0002 0.0016 -0.0024
29 H -0.0001 -0.0003 -0.0001
30 H -0.0002 0.0001 0.0002
31 H -0.0016 -0.0026 0.0008
32 H -0.0037 0.0008 -0.0049
33 H 0.0065 0.0088 0.0021
34 H 0.0078 0.0029 0.0138
35 H -0.0002 0.0001 -0.0002
36 H 0.0004 -0.0000 -0.0021
37 H -0.0020 0.0011 0.0258
38 H 0.0016 -0.0025 -0.0019
39 H -0.9058 -0.1772 -0.2175
40 H -0.0825 0.1853 0.0553
41 H -0.0253 -0.1775 0.1147
42 H -0.0001 -0.0005 0.0001
43 H 0.0070 0.0129 -0.0020
44 H 0.0051 -0.0059 0.0028
45 H -0.0002 -0.0001 -0.0001
46 H -0.0005 -0.0007 0.0009
47 H 0.0001 0.0002 0.0001
48 H -0.0002 -0.0000 -0.0000
49 H -0.0006 0.0003 -0.0001
Vibrational Mode 133
----------------------------------------------------
Harmonic frequency: 3056.33 cm**-1
Reduced mass: 1.0796 amu
Force constant: 5.9416 mdyne/A
IR intensity: 23.9799 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0000 0.0000
2 O 0.0000 -0.0000 -0.0000
3 O -0.0001 -0.0000 -0.0000
4 C 0.0002 0.0001 0.0002
5 C -0.0001 0.0000 0.0003
6 C 0.0001 0.0002 0.0004
7 C 0.0072 0.0024 0.0080
8 C 0.0001 0.0003 0.0002
9 C -0.0005 0.0020 0.0007
10 C -0.0000 -0.0000 -0.0000
11 C 0.0000 0.0002 0.0001
12 C 0.0007 0.0006 0.0005
13 C 0.0009 -0.0003 0.0010
14 C 0.0001 -0.0002 0.0001
15 C -0.0003 0.0007 -0.0012
16 C -0.0228 0.0760 0.0087
17 C -0.0000 -0.0001 0.0001
18 C 0.0013 -0.0017 0.0006
19 C 0.0001 -0.0000 -0.0000
20 C -0.0001 0.0002 -0.0001
21 C 0.0001 0.0001 -0.0001
22 C -0.0000 -0.0002 0.0001
23 C -0.0000 0.0000 -0.0000
24 H 0.0004 0.0003 -0.0032
25 H 0.0015 -0.0001 -0.0018
26 H -0.0927 -0.0338 -0.0992
27 H 0.0039 0.0033 0.0029
28 H 0.0003 0.0014 -0.0016
29 H -0.0012 -0.0049 -0.0014
30 H 0.0004 -0.0001 -0.0022
31 H -0.0010 -0.0020 0.0008
32 H -0.0033 0.0015 -0.0051
33 H -0.0061 -0.0101 -0.0032
34 H -0.0099 -0.0041 -0.0141
35 H -0.0063 0.0060 -0.0003
36 H -0.0003 0.0008 -0.0003
37 H -0.0030 -0.0001 0.0207
38 H 0.0062 -0.0092 -0.0041
39 H -0.1467 0.0124 -0.0291
40 H 0.5328 -0.7300 -0.2356
41 H -0.1047 -0.2065 0.1536
42 H -0.0003 -0.0004 -0.0008
43 H 0.0009 0.0014 -0.0002
44 H -0.0143 0.0194 -0.0083
45 H 0.0001 -0.0000 -0.0001
46 H -0.0011 -0.0023 0.0013
47 H 0.0003 0.0021 -0.0005
48 H -0.0000 0.0001 0.0000
49 H 0.0010 -0.0004 0.0003
Vibrational Mode 134
----------------------------------------------------
Harmonic frequency: 3063.95 cm**-1
Reduced mass: 1.0484 amu
Force constant: 5.7990 mdyne/A
IR intensity: 8.1835 km/mol
Normal mode:
X Y Z
1 O 0.0000 -0.0000 -0.0000
2 O -0.0000 0.0000 0.0000
3 O 0.0000 0.0000 0.0000
4 C -0.0005 -0.0001 -0.0002
5 C -0.0001 -0.0000 0.0002
6 C 0.0000 -0.0003 -0.0010
7 C -0.0209 -0.0055 -0.0243
8 C -0.0000 -0.0002 -0.0002
9 C -0.0004 -0.0006 0.0016
10 C 0.0000 0.0001 -0.0000
11 C 0.0001 0.0002 0.0001
12 C 0.0001 -0.0002 0.0002
13 C 0.0000 -0.0005 -0.0005
14 C 0.0001 -0.0001 0.0004
15 C -0.0002 -0.0003 0.0027
16 C -0.0104 -0.0286 0.0409
17 C 0.0001 0.0001 -0.0001
18 C -0.0003 0.0006 0.0000
19 C -0.0001 -0.0001 -0.0000
20 C 0.0009 0.0013 -0.0006
21 C 0.0001 -0.0000 -0.0000
22 C -0.0000 0.0001 -0.0001
23 C 0.0000 0.0000 0.0000
24 H 0.0003 0.0000 -0.0033
25 H -0.0054 -0.0001 0.0062
26 H 0.2821 0.1091 0.2984
27 H -0.0246 -0.0383 -0.0055
28 H -0.0005 -0.0013 0.0014
29 H 0.0008 0.0038 0.0012
30 H 0.0002 -0.0002 -0.0024
31 H -0.0019 -0.0030 0.0008
32 H -0.0023 0.0007 -0.0032
33 H 0.0006 0.0010 -0.0001
34 H 0.0055 0.0006 0.0079
35 H -0.0056 0.0047 -0.0010
36 H -0.0005 -0.0001 -0.0029
37 H 0.0056 -0.0012 -0.0399
38 H -0.0019 0.0028 0.0029
39 H -0.2700 -0.0666 -0.0447
40 H 0.1507 -0.2381 -0.0524
41 H 0.2471 0.6543 -0.4009
42 H 0.0003 0.0006 0.0010
43 H -0.0011 -0.0020 0.0003
44 H 0.0032 -0.0047 0.0019
45 H -0.0001 0.0001 0.0002
46 H -0.0098 -0.0160 0.0067
47 H -0.0001 -0.0013 0.0003
48 H 0.0001 -0.0001 -0.0000
49 H -0.0001 0.0002 -0.0001
Vibrational Mode 135
----------------------------------------------------
Harmonic frequency: 3076.54 cm**-1
Reduced mass: 1.0686 amu
Force constant: 5.9594 mdyne/A
IR intensity: 37.8712 km/mol
Normal mode:
X Y Z
1 O -0.0001 0.0000 0.0000
2 O 0.0000 -0.0000 -0.0000
3 O 0.0000 0.0000 0.0000
4 C 0.0012 0.0003 0.0008
5 C -0.0001 0.0000 0.0005
6 C 0.0004 0.0006 0.0020
7 C 0.0430 0.0133 0.0493
8 C -0.0000 0.0003 0.0007
9 C -0.0004 -0.0008 0.0008
10 C -0.0000 -0.0001 0.0000
11 C -0.0001 -0.0000 0.0010
12 C 0.0005 -0.0004 0.0008
13 C 0.0011 -0.0002 0.0012
14 C -0.0000 -0.0001 0.0004
15 C -0.0004 -0.0001 0.0045
16 C -0.0055 -0.0257 0.0181
17 C -0.0001 -0.0001 0.0003
18 C -0.0004 0.0006 -0.0001
19 C -0.0001 -0.0001 -0.0000
20 C 0.0010 0.0014 -0.0006
21 C 0.0001 -0.0000 0.0000
22 C 0.0000 0.0002 -0.0001
23 C 0.0000 -0.0000 0.0000
24 H 0.0010 0.0002 -0.0067
25 H 0.0084 0.0001 -0.0097
26 H -0.5890 -0.2183 -0.6194
27 H 0.0353 0.0442 0.0152
28 H 0.0011 0.0048 -0.0060
29 H -0.0023 -0.0100 -0.0031
30 H 0.0017 -0.0002 -0.0106
31 H 0.0010 0.0011 0.0004
32 H -0.0072 0.0026 -0.0105
33 H 0.0012 0.0015 0.0006
34 H -0.0095 -0.0046 -0.0162
35 H -0.0070 0.0067 -0.0005
36 H -0.0001 -0.0001 -0.0034
37 H 0.0055 -0.0014 -0.0625
38 H -0.0002 0.0003 0.0022
39 H -0.0561 -0.0249 -0.0043
40 H -0.0048 -0.0125 0.0072
41 H 0.1469 0.3613 -0.2258
42 H -0.0008 -0.0014 -0.0026
43 H 0.0017 0.0031 -0.0004
44 H 0.0040 -0.0056 0.0024
45 H 0.0006 -0.0003 -0.0007
46 H -0.0102 -0.0166 0.0071
47 H -0.0003 -0.0021 0.0005
48 H -0.0001 0.0004 0.0001
49 H -0.0003 0.0002 -0.0001
Vibrational Mode 136
----------------------------------------------------
Harmonic frequency: 3086.53 cm**-1
Reduced mass: 1.0763 amu
Force constant: 6.0415 mdyne/A
IR intensity: 11.6436 km/mol
Normal mode:
X Y Z
1 O -0.0000 -0.0000 0.0000
2 O -0.0000 -0.0000 0.0000
3 O 0.0001 0.0001 -0.0000
4 C 0.0000 0.0001 0.0000
5 C -0.0001 -0.0000 -0.0004
6 C 0.0001 0.0000 -0.0000
7 C 0.0004 0.0002 0.0004
8 C 0.0000 0.0001 -0.0000
9 C -0.0007 0.0013 -0.0005
10 C 0.0000 0.0000 -0.0000
11 C 0.0000 0.0001 -0.0000
12 C 0.0005 0.0009 0.0002
13 C 0.0001 -0.0000 0.0001
14 C 0.0002 -0.0001 -0.0002
15 C -0.0001 0.0001 0.0001
16 C -0.0009 0.0007 0.0010
17 C 0.0000 0.0001 -0.0000
18 C -0.0270 0.0356 -0.0153
19 C 0.0004 0.0005 0.0000
20 C -0.0324 -0.0494 0.0220
21 C -0.0019 -0.0015 0.0004
22 C 0.0000 0.0000 -0.0000
23 C -0.0002 -0.0001 -0.0000
24 H 0.0001 0.0005 0.0060
25 H -0.0005 -0.0001 0.0006
26 H -0.0052 -0.0018 -0.0055
27 H -0.0004 -0.0013 0.0004
28 H 0.0000 -0.0006 0.0011
29 H -0.0004 -0.0013 -0.0004
30 H 0.0000 0.0001 0.0000
31 H -0.0007 -0.0013 0.0005
32 H 0.0012 -0.0001 0.0013
33 H -0.0093 -0.0139 -0.0023
34 H -0.0005 -0.0002 -0.0008
35 H -0.0006 0.0005 -0.0000
36 H -0.0001 0.0000 0.0009
37 H 0.0001 -0.0000 -0.0005
38 H 0.0005 -0.0002 -0.0002
39 H -0.0031 -0.0005 -0.0001
40 H 0.0115 -0.0165 -0.0055
41 H 0.0035 0.0094 -0.0060
42 H -0.0000 -0.0000 -0.0001
43 H -0.0004 -0.0009 0.0002
44 H 0.3405 -0.4630 0.1994
45 H 0.0001 0.0000 -0.0000
46 H 0.3900 0.6269 -0.2785
47 H 0.0003 -0.0006 0.0002
48 H 0.0000 0.0000 0.0000
49 H 0.0024 -0.0003 0.0006
Vibrational Mode 137
----------------------------------------------------
Harmonic frequency: 3101.10 cm**-1
Reduced mass: 1.0888 amu
Force constant: 6.1693 mdyne/A
IR intensity: 54.9631 km/mol
Normal mode:
X Y Z
1 O 0.0000 -0.0000 0.0000
2 O 0.0000 -0.0000 -0.0000
3 O -0.0003 0.0001 -0.0001
4 C 0.0000 -0.0000 0.0000
5 C -0.0000 0.0002 -0.0005
6 C -0.0000 -0.0000 0.0000
7 C -0.0003 -0.0001 -0.0004
8 C 0.0000 0.0002 -0.0001
9 C -0.0012 0.0005 0.0001
10 C -0.0000 0.0000 -0.0000
11 C -0.0000 -0.0000 -0.0001
12 C 0.0004 0.0009 0.0001
13 C -0.0000 0.0001 0.0000
14 C 0.0001 -0.0001 -0.0000
15 C -0.0001 0.0001 -0.0002
16 C -0.0007 0.0023 -0.0008
17 C 0.0000 0.0001 -0.0000
18 C -0.0395 0.0497 -0.0217
19 C -0.0000 -0.0003 -0.0000
20 C 0.0275 0.0417 -0.0184
21 C 0.0017 -0.0005 0.0001
22 C 0.0001 -0.0000 0.0001
23 C -0.0002 -0.0000 -0.0001
24 H 0.0000 0.0007 0.0062
25 H 0.0000 -0.0001 -0.0000
26 H 0.0044 0.0017 0.0048
27 H -0.0006 -0.0010 0.0001
28 H -0.0001 -0.0013 0.0019
29 H -0.0002 -0.0006 -0.0002
30 H -0.0001 -0.0000 0.0006
31 H 0.0003 0.0002 -0.0001
32 H 0.0020 -0.0005 0.0023
33 H -0.0092 -0.0141 -0.0023
34 H -0.0004 0.0001 -0.0003
35 H 0.0009 -0.0009 0.0001
36 H -0.0002 -0.0001 -0.0027
37 H -0.0005 -0.0002 0.0043
38 H 0.0006 -0.0004 -0.0004
39 H 0.0092 0.0034 0.0029
40 H 0.0082 -0.0101 -0.0044
41 H -0.0088 -0.0210 0.0130
42 H 0.0001 0.0001 0.0002
43 H -0.0004 -0.0009 0.0001
44 H 0.4405 -0.6021 0.2592
45 H -0.0000 -0.0000 -0.0001
46 H -0.2985 -0.4821 0.2145
47 H -0.0002 0.0003 -0.0000
48 H -0.0000 0.0001 -0.0000
49 H 0.0024 -0.0007 0.0006
Vibrational Mode 138
----------------------------------------------------
Harmonic frequency: 3166.31 cm**-1
Reduced mass: 1.0812 amu
Force constant: 6.3863 mdyne/A
IR intensity: 5.5858 km/mol
Normal mode:
X Y Z
1 O 0.0000 -0.0000 0.0000
2 O 0.0000 -0.0000 -0.0000
3 O 0.0015 -0.0007 0.0005
4 C -0.0000 0.0000 0.0000
5 C 0.0000 -0.0000 -0.0000
6 C -0.0000 0.0000 0.0000
7 C -0.0000 0.0000 -0.0000
8 C 0.0000 0.0000 -0.0000
9 C -0.0000 -0.0000 0.0000
10 C -0.0000 0.0000 0.0000
11 C -0.0000 0.0000 0.0000
12 C 0.0000 0.0000 -0.0000
13 C -0.0000 0.0000 -0.0000
14 C -0.0000 -0.0000 0.0001
15 C -0.0001 0.0002 -0.0001
16 C -0.0001 0.0002 -0.0000
17 C -0.0000 0.0000 0.0000
18 C -0.0001 0.0002 -0.0001
19 C 0.0006 0.0010 -0.0001
20 C 0.0000 0.0000 -0.0000
21 C -0.0012 -0.0001 -0.0002
22 C 0.0013 0.0295 -0.0069
23 C 0.0716 -0.0146 0.0196
24 H 0.0000 0.0000 0.0001
25 H 0.0001 -0.0000 -0.0001
26 H 0.0003 0.0001 0.0004
27 H -0.0001 -0.0002 0.0000
28 H 0.0000 -0.0000 0.0000
29 H -0.0000 -0.0001 -0.0000
30 H 0.0000 -0.0000 -0.0001
31 H -0.0000 -0.0001 0.0000
32 H 0.0001 -0.0000 0.0001
33 H -0.0001 -0.0002 -0.0001
34 H -0.0000 0.0000 0.0000
35 H 0.0001 -0.0001 0.0000
36 H -0.0003 0.0000 -0.0013
37 H -0.0005 0.0001 0.0023
38 H 0.0019 -0.0038 -0.0013
39 H 0.0006 0.0003 0.0002
40 H 0.0009 -0.0013 -0.0004
41 H -0.0006 -0.0010 0.0008
42 H 0.0000 -0.0000 -0.0000
43 H 0.0000 0.0000 -0.0000
44 H 0.0017 -0.0023 0.0010
45 H -0.0000 0.0000 0.0000
46 H -0.0001 0.0002 -0.0001
47 H -0.0304 -0.3861 0.0880
48 H -0.0000 0.0000 -0.0000
49 H -0.8559 0.2094 -0.2432
Vibrational Mode 139
----------------------------------------------------
Harmonic frequency: 3184.04 cm**-1
Reduced mass: 1.0939 amu
Force constant: 6.5343 mdyne/A
IR intensity: 23.7515 km/mol
Normal mode:
X Y Z
1 O 0.0000 0.0000 -0.0000
2 O 0.0000 -0.0000 -0.0000
3 O 0.0007 0.0002 0.0001
4 C -0.0000 -0.0000 0.0000
5 C -0.0000 -0.0000 -0.0000
6 C -0.0000 -0.0000 0.0000
7 C 0.0000 -0.0000 0.0000
8 C -0.0000 -0.0000 -0.0000
9 C 0.0000 -0.0000 0.0000
10 C -0.0000 -0.0000 0.0000
11 C -0.0000 -0.0000 -0.0000
12 C -0.0000 0.0000 -0.0000
13 C -0.0000 0.0000 0.0000
14 C 0.0001 -0.0001 0.0001
15 C 0.0002 -0.0004 0.0001
16 C -0.0000 -0.0002 0.0000
17 C -0.0000 -0.0000 -0.0000
18 C -0.0001 0.0001 -0.0000
19 C -0.0032 0.0007 -0.0008
20 C -0.0000 -0.0001 0.0000
21 C -0.0004 0.0005 -0.0002
22 C -0.0080 -0.0779 0.0173
23 C 0.0353 -0.0057 0.0093
24 H -0.0001 -0.0000 -0.0001
25 H 0.0002 0.0000 -0.0002
26 H -0.0005 -0.0003 -0.0005
27 H 0.0003 0.0006 -0.0001
28 H -0.0000 -0.0001 0.0000
29 H 0.0001 0.0004 0.0001
30 H -0.0001 -0.0001 0.0001
31 H 0.0004 0.0008 -0.0003
32 H 0.0002 -0.0001 0.0002
33 H 0.0000 0.0001 0.0000
34 H -0.0001 -0.0000 -0.0001
35 H 0.0001 -0.0001 0.0000
36 H 0.0000 -0.0002 -0.0002
37 H 0.0008 -0.0002 -0.0035
38 H -0.0033 0.0064 0.0022
39 H 0.0000 -0.0001 0.0005
40 H -0.0006 0.0008 0.0003
41 H 0.0008 0.0016 -0.0011
42 H 0.0000 0.0000 0.0001
43 H 0.0000 0.0001 -0.0000
44 H 0.0010 -0.0011 0.0005
45 H -0.0000 -0.0000 -0.0000
46 H 0.0006 0.0003 -0.0002
47 H 0.0726 0.8897 -0.2023
48 H 0.0000 0.0000 -0.0000
49 H -0.3683 0.0888 -0.1043
Vibrational Mode 140
----------------------------------------------------
Harmonic frequency: 3525.83 cm**-1
Reduced mass: 1.0657 amu
Force constant: 7.8055 mdyne/A
IR intensity: 12.0886 km/mol
Normal mode:
X Y Z
1 O -0.0036 0.0014 0.0057
2 O -0.0335 -0.0516 0.0031
3 O 0.0000 0.0000 -0.0000
4 C 0.0000 -0.0000 0.0000
5 C -0.0000 -0.0000 0.0000
6 C 0.0000 -0.0001 -0.0000
7 C 0.0000 0.0000 -0.0000
8 C -0.0000 -0.0000 0.0001
9 C -0.0000 0.0000 0.0000
10 C -0.0002 0.0000 0.0001
11 C 0.0000 0.0000 0.0000
12 C 0.0000 -0.0000 0.0000
13 C 0.0001 -0.0001 -0.0000
14 C -0.0000 0.0000 0.0000
15 C -0.0000 0.0000 -0.0000
16 C 0.0000 -0.0000 -0.0000
17 C -0.0018 -0.0013 0.0009
18 C 0.0000 -0.0000 0.0000
19 C -0.0000 0.0000 -0.0000
20 C -0.0000 -0.0000 0.0000
21 C -0.0000 -0.0000 0.0000
22 C 0.0000 0.0000 -0.0000
23 C -0.0000 -0.0000 -0.0000
24 H 0.0001 0.0001 -0.0004
25 H -0.0005 -0.0001 -0.0004
26 H 0.0004 0.0001 0.0003
27 H -0.0002 0.0001 0.0000
28 H 0.0000 0.0003 -0.0008
29 H -0.0000 -0.0010 -0.0005
30 H 0.0000 -0.0000 -0.0002
31 H -0.0001 -0.0001 0.0001
32 H -0.0001 0.0002 -0.0007
33 H 0.0002 0.0004 -0.0000
34 H -0.0000 0.0003 0.0002
35 H -0.0003 0.0007 0.0003
36 H 0.0000 -0.0000 -0.0001
37 H -0.0000 0.0000 0.0001
38 H 0.0001 -0.0001 -0.0000
39 H 0.0001 0.0000 0.0000
40 H -0.0000 0.0000 0.0000
41 H -0.0001 -0.0000 0.0000
42 H 0.0007 0.0024 -0.0005
43 H 0.0017 0.0084 0.0024
44 H -0.0000 0.0001 -0.0000
45 H 0.0586 -0.0187 -0.0930
46 H 0.0000 0.0000 -0.0000
47 H -0.0000 -0.0000 0.0000
48 H 0.5515 0.8221 -0.0604
49 H 0.0000 -0.0000 0.0000
Vibrational Mode 141
----------------------------------------------------
Harmonic frequency: 3530.46 cm**-1
Reduced mass: 1.0652 amu
Force constant: 7.8225 mdyne/A
IR intensity: 9.8789 km/mol
Normal mode:
X Y Z
1 O 0.0310 -0.0120 -0.0516
2 O -0.0038 -0.0058 0.0003
3 O 0.0000 0.0000 -0.0000
4 C -0.0000 0.0000 -0.0001
5 C 0.0000 0.0000 -0.0000
6 C -0.0003 0.0000 0.0004
7 C 0.0000 0.0000 -0.0000
8 C 0.0002 -0.0001 -0.0004
9 C -0.0000 0.0000 -0.0000
10 C 0.0014 0.0004 -0.0013
11 C -0.0000 -0.0000 -0.0000
12 C -0.0000 0.0000 -0.0000
13 C -0.0000 -0.0000 0.0000
14 C 0.0000 -0.0000 -0.0000
15 C 0.0000 -0.0000 -0.0000
16 C -0.0000 -0.0000 0.0000
17 C -0.0002 -0.0001 -0.0001
18 C 0.0000 0.0000 0.0000
19 C -0.0000 0.0000 -0.0000
20 C -0.0000 0.0000 -0.0000
21 C -0.0000 -0.0000 0.0000
22 C 0.0000 0.0000 0.0000
23 C 0.0000 -0.0000 0.0000
24 H -0.0001 -0.0001 0.0007
25 H 0.0021 0.0012 0.0002
26 H -0.0008 -0.0002 -0.0006
27 H 0.0010 -0.0005 -0.0000
28 H 0.0010 -0.0010 0.0015
29 H 0.0008 0.0071 0.0024
30 H -0.0002 0.0001 0.0009
31 H 0.0002 0.0005 -0.0002
32 H 0.0002 -0.0001 0.0001
33 H -0.0002 -0.0002 0.0001
34 H -0.0005 -0.0002 -0.0004
35 H 0.0001 0.0000 0.0002
36 H -0.0000 -0.0000 0.0002
37 H 0.0001 0.0000 -0.0000
38 H 0.0000 0.0001 0.0000
39 H -0.0001 -0.0000 0.0000
40 H 0.0001 -0.0001 -0.0000
41 H 0.0001 0.0001 -0.0001
42 H 0.0009 0.0005 0.0011
43 H -0.0003 -0.0010 0.0008
44 H 0.0000 -0.0001 0.0000
45 H -0.5092 0.1807 0.8317
46 H 0.0000 0.0000 -0.0000
47 H 0.0000 0.0000 -0.0000
48 H 0.0603 0.0936 -0.0057
49 H 0.0000 -0.0000 0.0000
Now we have the frequencies, normal modes and IR intensities. In order to animate a specific normal mode, we need to write a routine which selects the mode of interest and returns it in the format required by py3Dmol. We would also like to plot the IR spectrum and, for this, we also need a routine which adds a Gaussian or Lorentzian broadening to the calculated IR spectrum.
# To animate the normal mode we will need both the geometry and the displacements
def get_normal_mode(molecule, normal_mode):
elements = molecule.get_labels()
coords = molecule.get_coordinates() * bohr_in_angstroms() # To transform from au to A
natm = molecule.number_of_atoms()
vib_xyz = "%d\n\n" % natm
nm = normal_mode.reshape(natm, 3)
for i in range(natm):
# add coordinates:
vib_xyz += elements[i] + " %15.7f %15.7f %15.7f " % (coords[i,0], coords[i,1], coords[i,2])
# add displacements:
vib_xyz += "%15.7f %15.7f %15.7f\n" % (nm[i,0], nm[i,1], nm[i,2])
return vib_xyz
# Broadening function
def add_broadening(list_ex_energy, list_osci_strength, line_profile='Lorentzian', line_param=10, step=10):
x_min = np.amin(list_ex_energy) - 50
x_max = np.amax(list_ex_energy) + 50
x = np.arange(x_min, x_max, step)
y = np.zeros((len(x)))
#print(x)
#print(y)
# go through the frames and calculate the spectrum for each frame
for xp in range(len(x)):
for e, f in zip(list_ex_energy, list_osci_strength):
if line_profile == 'Gaussian':
y[xp] += f * np.exp(-(
(e - x[xp]) / line_param)**2)
elif line_profile == 'Lorentzian':
y[xp] += 0.5 * line_param * f / (np.pi * (
(x[xp] - e)**2 + 0.25 * line_param**2))
return x, y
After adding the broadening, we can plot the spectrum and then animate a specific normal mode, selected based on its index.
wvn, ir = xtb_hessian_drv.frequencies, xtb_hessian_drv.ir_intensities
wvng, irg = add_broadening(wvn, ir, line_profile='Gaussian', line_param=10, step=2)
# Plot the IR spectra
plt.figure(figsize=(7,4))
# Plot the IR spectrum
plt.plot(wvng, irg, label='IR spectrum')
plt.xlabel('Wavenumber (cm**-1)')
#plt.axis(xmin=3200, xmax=3500)
#plt.axis(ymin=-0.2, ymax=1.5)
plt.ylabel('IR intensity (km/mol)')
plt.title("Calculated IR sepctrum, Kahweol")
plt.legend()
plt.tight_layout(); plt.show()
# Visualize the vibrational mode
index = 64
print("\n\n\n Normal mode %d: %.2f cm-1, %.2f km/mol." % (index,
xtb_hessian_drv.frequencies[index-1],
xtb_hessian_drv.ir_intensities[index-1]))
normal_mode = get_normal_mode(xtb_opt_kahweol, xtb_hessian_drv.normal_modes[index-1])
view = p3d.view(viewergrid=(1,1), width=600, height=300)
view.addModel(normal_mode, "xyz", {'vibrate': {'frames':10,'amplitude':0.75}})
view.setViewStyle({"style": "outline", "width": 0.05})
view.setStyle({"stick": {}, "sphere": {"scale": 0.25}})
view.animate({'loop': 'backAndForth'})
view.zoomTo()
view.show()
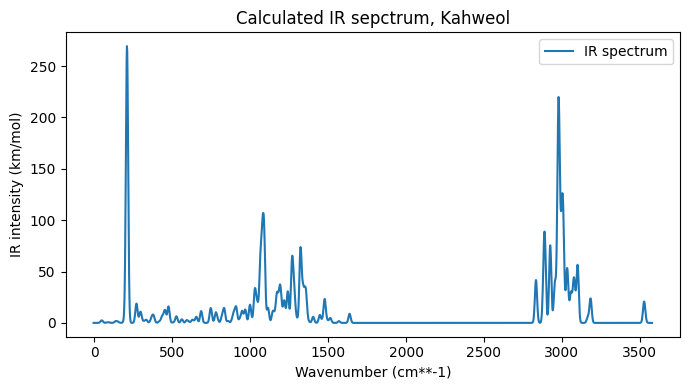
Normal mode 64: 1078.75 cm-1, 68.62 km/mol.
3Dmol.js failed to load for some reason. Please check your browser console for error messages.
To make a more interactive normal mode selection, we can create a slider using the ipywidgets Python module for Jupyter notebooks. To make use of the slider widget, we first need a routine which can plot the IR spectrum and animate a specific normal mode selected based on its index.
def vibration_viewer(index):
freq = xtb_hessian_drv.frequencies[index-1]
ir_intens = xtb_hessian_drv.ir_intensities[index-1]
# Plot the IR spectrum
plt.figure(figsize=(7,4))
plt.plot(wvng, irg)
plt.plot(freq, ir_intens, 'o', markersize=10)
plt.xlabel('Wavenumber (cm**-1)')
plt.ylabel('IR intensity (km/mol)')
plt.title("Calculated IR sepctrum, Kahweol")
plt.tight_layout(); plt.show()
normal_mode = get_normal_mode(xtb_opt_kahweol, xtb_hessian_drv.normal_modes[index-1])
view = p3d.view(viewergrid=(1,1), width=600, height=300)
view.addModel(normal_mode, "xyz", {'vibrate': {'frames':10,'amplitude':0.75}})
view.setViewStyle({"style": "outline", "width": 0.05})
view.setStyle({"stick": {}, "sphere": {"scale": 0.25}})
view.animate({'loop': 'backAndForth'})
view.zoomTo()
view.show()
no_norm_modes = xtb_hessian_drv.frequencies.shape[0] # number of vibrational modes
# Note that the slider only works in a Jupyter notebook.
ipywidgets.interact(vibration_viewer,
index=ipywidgets.IntSlider(min=1, max=no_norm_modes, step=1, value=92))
<function __main__.vibration_viewer(index)>